Understanding let, const, and var in JavaScript Programming
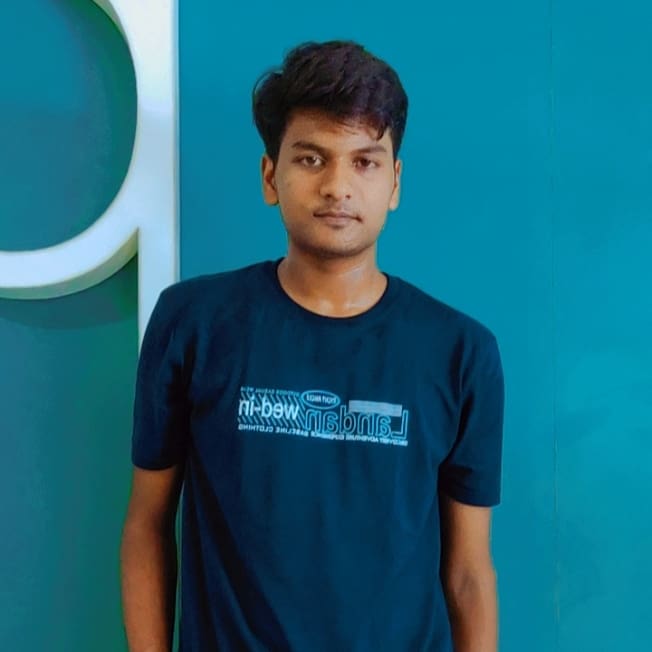
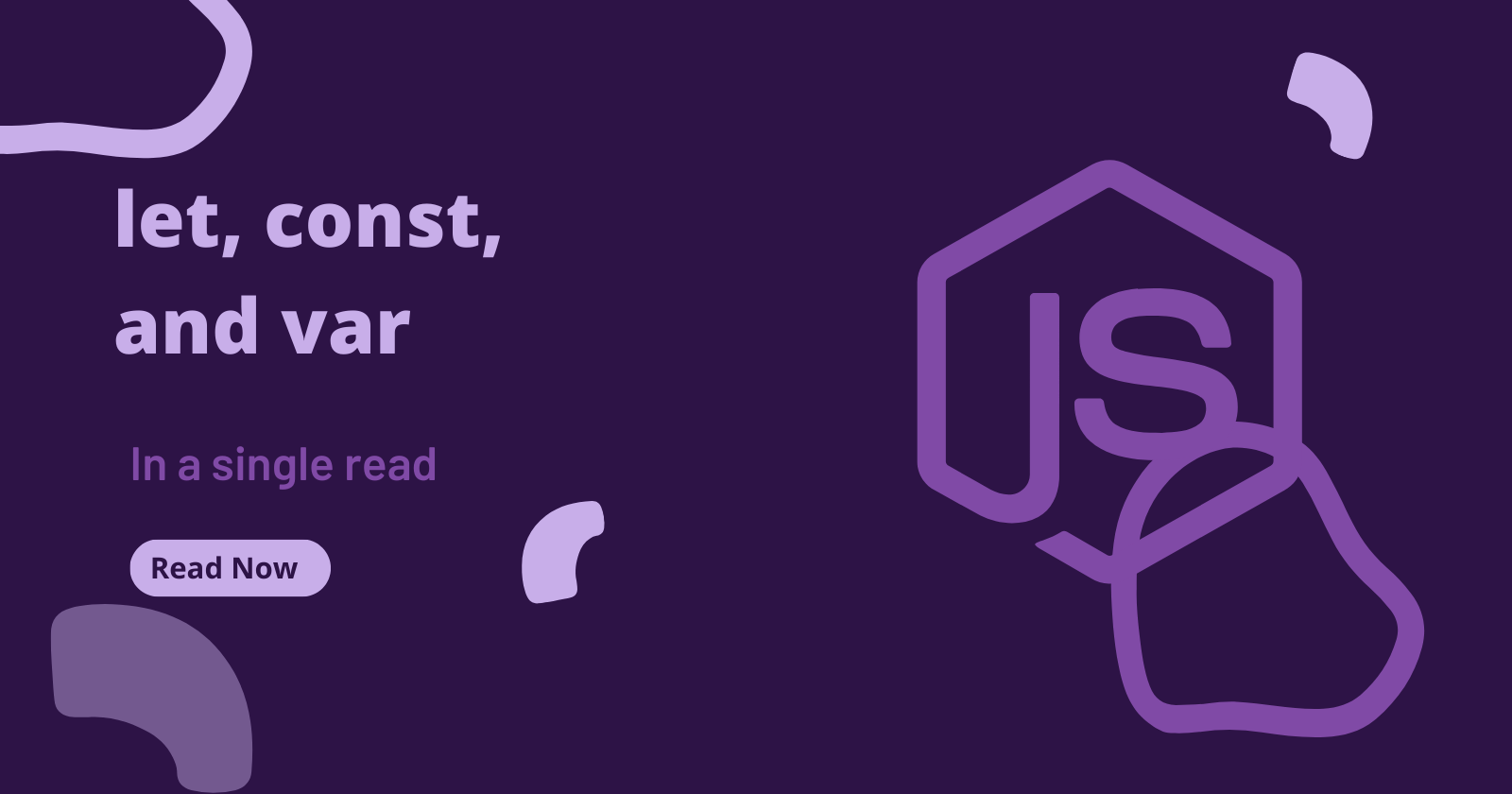
In JavaScript, certain words called keywords have special meanings. Today, let's explore three important ones: let
, const
, and var
. These keywords help us work with data more effectively in our code. Let's break them down!
Const: Keeping Things Steady
When we use const
to make a variable, it means that the value of that variable can't change later on. It's like writing something in pen instead of pencil—it stays put! Here's a quick example:
const accountId = 14435;
accountId = 2334; // Oops! This won't work.
Once we've set accountId
to 14435
, we can't change it to 2334
later. This helps keep our code predictable and less prone to mistakes.
Let vs. Var: Scoping Out the Difference
JavaScript gives us two ways to make variables: let
and var
. They're pretty similar, but they behave a bit differently when it comes to where we can use them.
Var's tricky scope
Imagine we have a message to share in a function:
function example() {
if (true) {
var message = "Hello";
}
console.log(message); // Prints: Hello
}
example();
console.log(message); // Oops! This will give an error.
With var
, message
can sneak out of its little box (the if
statement) and cause confusion elsewhere in our code. Not cool!
Now try using let
Now, let's try the same thing with let
:
function example() {
if (true) {
let message = "Hello";
}
console.log(message); // Uh-oh! This will give an error too.
}
example();
console.log(message); // Oops! Another error here.
With let
, message
stays inside its box, or "block," and doesn't cause trouble elsewhere.
note: always use let instead of var because of block/functional scope issue
Making Variables Without Keywords
Did you know we can make variables without using any special words like let
or const
? It's like saying, "Hey, computer, remember this for me!" Here's how:
personCountry = "India";
console.log(personCountry); // Prints: India
In js, we can declare variables without using keywords.
Getting Started with Undefined
Sometimes, we want to make a variable but don't have a value for it just yet. That's where undefined
comes in handy:
let accountState;
console.log(accountState); // Prints: undefined
Here, accountState
exists, but it doesn't have anything inside it yet. It's like having an empty box waiting to be filled!
Understanding
let
,const
, andvar
helps us write code that's easier to understand and less likely to break. Remember: useconst
when you want things to stay put,let
for keeping things tidy, and always give your variables a clear purpose. Happy coding!
Subscribe to my newsletter
Read articles from Harsh Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
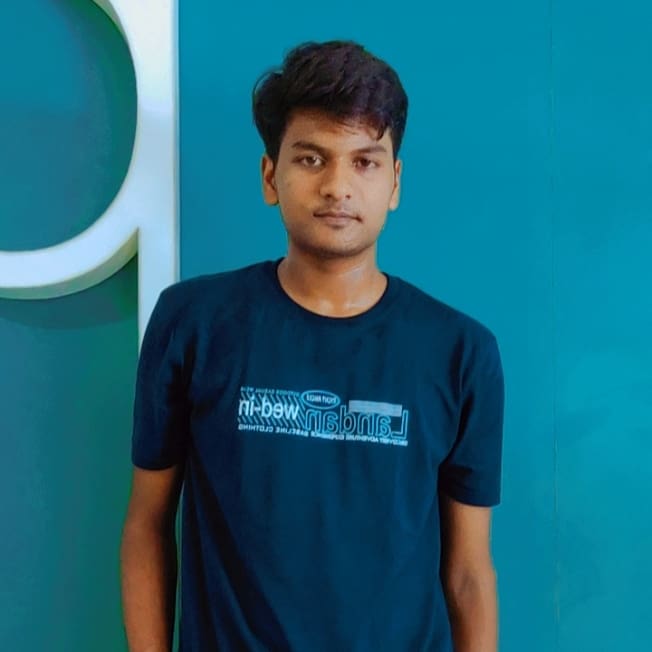
Harsh Gupta
Harsh Gupta
I am a CSE undergrad learning DevOps and sharing what I learn.