React: Best Practices
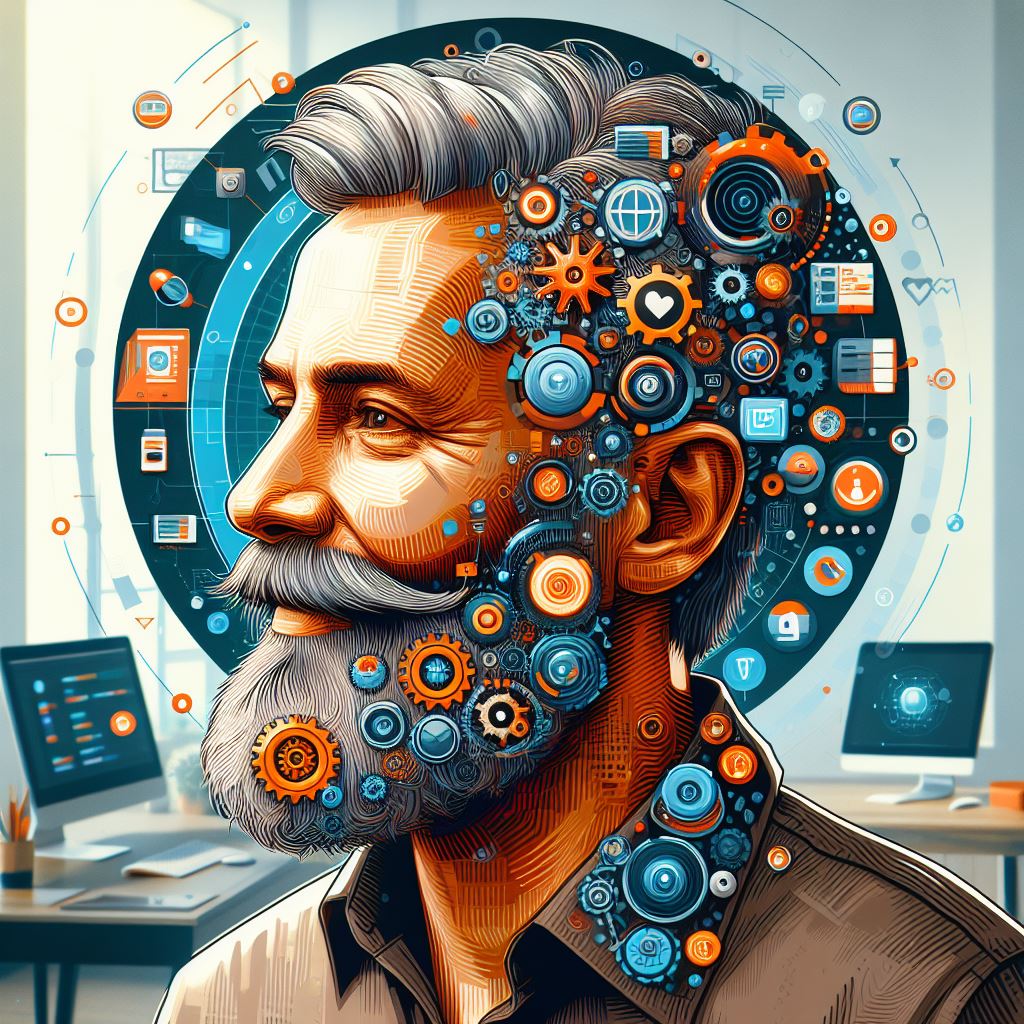
Below are my key take aways from the above video. Kindly note that its recommended to follow the best practices but sometimes you got to do what you got to do.
Following are 4 key best practices in React:
A. Dividing Project into different components:
Making project modular is not only good from organisational purposes. But this also has direct impact on application performance. Dividing a React application into components and sub-components is considered a best practice for several reasons:
Modularity and Reusability: Breaking down a React application into smaller, reusable components promotes modularity and reusability. Each component encapsulates a specific piece of functionality or user interface element, making it easier to understand, maintain, and reuse across different parts of the application or even in other projects.
Separation of Concerns: Component-based architecture encourages separation of concerns, where each component is responsible for a specific aspect of the application's functionality or presentation. This separation makes it easier to reason about the code, debug issues, and make changes without affecting other parts of the application.
Encapsulation: Components in React encapsulate both the UI elements and the associated logic, allowing developers to manage the complexity of the application by breaking it down into smaller, manageable units. This encapsulation helps maintain a clear boundary between different parts of the application, reducing the risk of unintended side effects and making it easier to test and refactor code.
Scalability: Dividing a React application into components and sub-components makes it more scalable, as developers can add, remove, or modify individual components without affecting the entire application. This modular approach enables teams to work on different parts of the application concurrently, improving productivity and reducing development time.
Code Reusability and Maintainability: By promoting code reusability and encapsulation, component-based architecture improves code maintainability. Changes to one component are less likely to have unintended consequences on other parts of the application, making it easier to maintain and update the codebase over time. Additionally, reusable components reduce duplication of code, leading to cleaner, more maintainable codebases.
Improved Collaboration: Component-based architecture facilitates collaboration among team members by providing clear boundaries between different parts of the application. Developers can work on individual components independently, reducing merge conflicts and enabling parallel development. Additionally, reusable components can be shared among team members, fostering a collaborative and efficient development process.
Dividing a React application into components and sub-components itself does not directly improve application performance. However, component-based architecture indirectly contributes to performance optimization in several ways:
Optimized Rendering:
- React's virtual DOM and reconciliation algorithm optimize rendering by only updating the parts of the DOM that have changed. When components are divided into smaller, more granular units, React can efficiently determine which components need to be re-rendered, leading to faster rendering and better performance.
Component Reusability:
- Reusable components reduce code duplication and promote code sharing, which can indirectly improve performance by reducing the overall size of the application bundle. Smaller bundle sizes lead to faster initial load times, especially in scenarios where components are shared across multiple parts of the application.
Granular State Management:
- Component-based architecture encourages a more granular approach to state management, where each component manages its own state and only updates when necessary. This fine-grained control over state reduces unnecessary re-renders and improves performance by minimizing the impact of state changes on the rest of the application.
Code Splitting:
- Dividing a React application into components and sub-components enables developers to implement code splitting techniques, where different parts of the application are loaded asynchronously as needed. Code splitting reduces the initial load time of the application by loading only the essential code upfront and deferring the loading of non-essential code until later.
Memoization and PureComponent:
- React provides optimization techniques such as memoization and PureComponent to prevent unnecessary re-renders of components. By breaking down the application into smaller components, developers can apply these optimization techniques more effectively to individual components, improving performance by minimizing unnecessary re-renders.
Parallel Development and Performance Tuning:
- Component-based architecture facilitates parallel development, allowing multiple team members to work on different components simultaneously. This parallel development approach enables developers to focus on optimizing individual components for performance, leading to overall performance improvements across the application.
Let's dive deeper into each example and provide more context into when to create components vs when to avoid them:
When to Create Components:
UI Reusability:
Best Practice : When building a web application, certain UI elements, such as headers, navigation menus, footers, or sidebars, are used across multiple pages. By creating components for these reusable UI elements, you can encapsulate their functionality and appearance in a single place, making it easier to maintain consistency across different parts of your application.
Example : Imagine you're building a website with a consistent header across all pages, containing a logo, navigation links, and a user profile dropdown. By creating a header component, you can reuse this layout across all pages without duplicating code.
Functional Cohesion:
Best Practice : Components should have a clear purpose and responsibility, encapsulating a single, cohesive piece of functionality. This promotes code organization and maintainability by grouping related functionality together in a modular and reusable way.
Example : Consider building a form component for user registration. This form includes input fields for username, email, password, and a submit button. By creating a form component, you can encapsulate the logic for input validation, submission handling, and error display within a single component.
Separation of Concerns:
Best Practice : Separating concerns in your application involves dividing it into distinct sections, each responsible for a specific aspect of functionality. By creating components, you can isolate different parts of your application, making it easier to understand, maintain, and scale.
Example : Suppose you're building a product catalog for an e-commerce website. Each product displayed on the catalog page consists of various details like name, image, price, and description. By creating a product card component, you can encapsulate the presentation logic and UI elements related to displaying product information, separating it from other parts of your application.
Composition:
Best Practice : Components can be composed together to build complex UI layouts from simpler building blocks. This promotes code reuse and maintainability by breaking down the UI into manageable, reusable components that can be combined to create more complex structures.
Example : Imagine building a dashboard for a data analytics application. The dashboard consists of various widgets, such as charts, tables, and data summaries. Each widget can be implemented as a separate component and composed together to form the complete dashboard layout.
Data Fetching:
Best Practice : Components can fetch and display data from APIs or external sources, encapsulating the logic for data fetching, error handling, and loading states. This promotes code organization and reusability by separating data fetching concerns from UI presentation concerns.
Example : Consider building a user profile component that fetches user data from an API and displays it, including the user's name, email, profile picture, and recent activity. By creating a user profile component, you can encapsulate the logic for fetching and displaying user data within a single component.
Event Handling:
Best Practice : Components can handle user interactions and events, encapsulating the logic for event handling and state management. This promotes code organization and maintainability by separating UI-related logic from business logic.
Example : Suppose you're building a dropdown menu component that allows users to select options from a list. By creating a dropdown menu component, you can encapsulate the logic for opening and closing the menu, selecting options, and triggering actions based on user input.
When Not to Create Components:
Premature Abstraction:
Best Practice : Avoid creating components prematurely for UI elements that are not reused or do not require encapsulation. Premature abstraction can lead to unnecessary complexity and overhead in the codebase.
Example : If you have a simple landing page layout with static content, such as a hero section, features section, and footer, individual components for each section may be unnecessary, and keeping the layout as a single page component may suffice.
Overly Fine-Grained Components:
Best Practice : Avoid creating components that are too small or granular, as this can lead to excessive nesting and complexity in the component hierarchy.
Example : If you have a form with a single input field for collecting user feedback, creating separate components for each input field may be overly fine-grained, as the form is simple and does not require extensive reusability or encapsulation.
Tight Coupling:
Best Practice : Avoid creating components that are tightly coupled to specific implementation details or dependencies, as this limits their reusability and flexibility.
Example : If you have a tooltip component tightly coupled to a specific CSS framework or styling library, its reusability may be limited in different environments. Instead, creating a more generic tooltip component with customizable styling options may be preferable.
Performance Considerations:
Best Practice : Avoid creating components that introduce unnecessary performance overhead, such as excessive re-renders or inefficient data fetching.
Example : If you have a component rendering a large list of items with complex UI elements and interactions, creating separate components for each item without considering performance implications may degrade application performance. Instead, optimizing the list rendering process or implementing virtualization techniques may be more suitable.
Global State Management:
Best Practice : Avoid creating components that manage global application state or have side effects outside their scope, as this violates separation of concerns and makes the codebase harder to maintain.
Example : If you have a component directly managing global state or interacting with browser storage, its responsibilities may be too broad, and its behavior may not be predictable in different contexts. Instead, using a dedicated state management solution or passing down props and callbacks may be more appropriate.
Prop Drilling:
Best Practice : Avoid creating components that rely on prop drilling to access shared data or functionality, as this can lead to prop conflicts and make the codebase harder to maintain.
Example : If you have a deeply nested component hierarchy where data needs to be passed down multiple levels using props, prop drilling may introduce unnecessary complexity and make the code harder to understand. Instead, using context or state management libraries to share data across components may be a better approach.
B. Using Fragments
Using fragments in React is considered a best practice for several reasons:
Avoiding Unnecessary DOM Elements:
- When rendering multiple components in React, each component needs to be wrapped in a single parent element. Without fragments, developers often resort to using
<div>
elements as wrappers, even when they serve no semantic purpose. Fragments allow developers to group multiple components without introducing extra DOM nodes, resulting in cleaner and more efficient code.
- When rendering multiple components in React, each component needs to be wrapped in a single parent element. Without fragments, developers often resort to using
Improved Performance:
- By eliminating unnecessary
<div>
wrappers, fragments reduce the size and complexity of the DOM tree. This can lead to improved rendering performance, especially in large and complex React applications where minimizing the DOM footprint is crucial for optimal performance.
- By eliminating unnecessary
Semantic Clarity:
- Fragments help maintain the semantic clarity of the JSX code by allowing developers to focus on the structure and hierarchy of components without being distracted by wrapper elements. This makes the code easier to read, understand, and maintain, especially for developers new to React or those unfamiliar with the codebase.
Avoiding Style and Layout Issues:
- Using
<div>
wrappers for grouping components can sometimes lead to unintended style and layout issues, especially when dealing with CSS styles or layout constraints. Fragments provide a lightweight and non-intrusive way to group components without affecting their styles or layout properties, ensuring consistent rendering behaviour across different components.
- Using
Reduced HTML Pollution:
- Fragments help reduce HTML pollution by eliminating unnecessary
<div>
elements that serve no purpose other than acting as wrappers for grouped components. This leads to cleaner and more maintainable JSX code, with fewer extraneous elements cluttering the markup.
- Fragments help reduce HTML pollution by eliminating unnecessary
Compatibility with Tooling and Libraries:
- Fragments are fully supported by popular React tooling and libraries, including JSX parsers, syntax highlighters, and code linters. Using fragments ensures compatibility with existing development workflows and tools, making it easier to integrate React components into larger projects or ecosystems.
Overall, using fragments in React helps improve code cleanliness, performance, and maintainability by allowing developers to group components without introducing unnecessary wrapper elements. By embracing fragments as a best practice, developers can write cleaner, more efficient, and semantically clear React code.
While fragments are generally beneficial for grouping components without introducing extra DOM elements, there are scenarios where using them may not be necessary or appropriate:
Single Child Components:
- When a component only renders a single child element, using a fragment is unnecessary. In such cases, it's more concise and readable to return the single child element directly without wrapping it in a fragment.
// Instead of using a fragment:
const MyComponent = () => {
return (
<>
<div>Hello</div>
</>
);
};
// You can directly return the single child:
const MyComponent = () => {
return <div>Hello</div>;
};
Semantic Structure Requirement:
- In situations where the parent-child relationship between components is important for semantic structure or accessibility purposes, using fragments may obscure this relationship. In such cases, it may be preferable to use a meaningful container element that conveys the intended structure.
// Using a fragment may obscure the parent-child relationship:
const PageLayout = () => {
return (
<>
<Header />
<MainContent />
<Footer />
</>
);
};
// Instead, use a meaningful container element:
const PageLayout = () => {
return (
<div>
<Header />
<MainContent />
<Footer />
</div>
);
};
Styling or Layout Requirements:
- Fragments do not contribute to the layout or styling of the page, so if you need a wrapping element for styling purposes, using a fragment may not be appropriate. In such cases, a container element with appropriate styling classes or attributes may be more suitable.
// If a wrapping element is needed for styling:
const StyledSection = () => {
return (
<section className="styled-section">
<>
<div>Hello</div>
<div>World</div>
</>
</section>
);
};
Compatibility with Older Browsers:
- Fragments are a feature introduced in React 16.2. If you need to support older browsers that do not fully support React 16 or JSX fragments, you may need to avoid using fragments or transpile them to a compatible syntax using tools like Babel.
// Avoid using fragments if targeting older browsers without JSX fragment support.
While fragments are a powerful tool for improving code cleanliness and performance in React applications, it's essential to consider the specific requirements and constraints of each use case before deciding whether to use them. In some cases, using fragments may not provide any significant benefit and may even obscure the intended structure or semantics of the components.
C. Relationship between States and Objects
Managing state in React is a fundamental aspect of building interactive and dynamic user interfaces. There are several approaches to managing state in React, each suited to different scenarios and application requirements. Here are some common methods for managing state in React:
Component State (useState Hook):
- React provides the
useState
hook, which allows functional components to have local state. You can declare state variables using array destructuring, where the first element is the state value, and the second element is a function to update that value. This approach is suitable for managing simple local state within a component.
- React provides the
codeimport React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
Props (Parent Component State):
- You can pass state down to child components via props. When the parent component's state changes, it triggers a re-render of the child components with the updated props. This approach is useful for sharing state among multiple components in a component tree.
codeimport React, { useState } from 'react';
const ParentComponent = () => {
const [text, setText] = useState('');
const handleChange = (event) => {
setText(event.target.value);
};
return (
<div>
<ChildComponent text={text} onChange={handleChange} />
</div>
);
};
const ChildComponent = ({ text, onChange }) => {
return (
<div>
<input type="text" value={text} onChange={onChange} />
<p>Value: {text}</p>
</div>
);
};
Context API:
- React's Context API allows you to share state across multiple components without manually passing props through each level of the component tree. It provides a way to pass data through the component tree without having to pass props down manually at every level. Context is primarily used when some data needs to be accessible by many components at different nesting levels.
codeimport React, { createContext, useContext, useState } from 'react';
const CountContext = createContext();
const ParentComponent = () => {
const [count, setCount] = useState(0);
return (
<CountContext.Provider value={{ count, setCount }}>
<ChildComponent />
</CountContext.Provider>
);
};
const ChildComponent = () => {
const { count, setCount } = useContext(CountContext);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
Redux or Other State Management Libraries:
- For larger applications with complex state management requirements, you can use external libraries like Redux, MobX, or Recoil. These libraries provide centralized state management, allowing you to define and update application state in a predictable and scalable way.
// Example with Redux
import { createStore } from 'redux';
import { Provider, useSelector, useDispatch } from 'react-redux';
// Redux store setup
const initialState = { count: 0 };
const reducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
default:
return state;
}
};
const store = createStore(reducer);
// Component using Redux state
const Counter = () => {
const count = useSelector((state) => state.count);
const dispatch = useDispatch();
const increment = () => {
dispatch({ type: 'INCREMENT' });
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
// App component wrapped with Redux provider
const App = () => {
return (
<Provider store={store}>
<Counter />
</Provider>
);
};
These are some of the common methods for managing state in React applications. The choice of state management approach depends on the complexity of your application, the scale of state management requirements, and your preference for simplicity versus flexibility.
In React, the useState
hook is used to manage state in functional components. When you call useState
, it returns an array with two elements: the current state value and a function to update that value.
The relationship between states and objects in useState
is that the current state value can be of any data type, including objects. When using an object as the state value, you can represent complex data structures and update them using the state updater function returned by useState
.
Here's an example demonstrating the relationship between states and objects in useState
:
codeimport React, { useState } from 'react';
const MyComponent = () => {
// Define state with an object
const [person, setPerson] = useState({ name: '', age: 0 });
// Event handler to update state
const handleChange = (event) => {
// Update state with a new object
setPerson({ ...person, [event.target.name]: event.target.value });
};
return (
<div>
<input
type="text"
name="name"
value={person.name}
onChange={handleChange}
placeholder="Name"
/>
<input
type="number"
name="age"
value={person.age}
onChange={handleChange}
placeholder="Age"
/>
<p>Name: {person.name}</p>
<p>Age: {person.age}</p>
</div>
);
};
export default MyComponent;
In this example, we have a component with a state variable person
, which is an object containing name
and age
properties. We use the useState
hook to initialize the state with an empty object { name: '', age: 0 }
.
The handleChange
function updates the person
state whenever the input fields change. It creates a new object by spreading the existing person
object and updating the changed property based on the name
attribute of the input field.
By leveraging objects in useState
, we can manage more complex state structures in React components, enabling us to build richer and more dynamic user interfaces.
let's explore the positives and negatives of using a single object state versus splitting it into multiple single-value states in a React component:
A. Single Object State:
Positives:
Grouping Related Data:
- Using a single object state allows you to group related data together. This can be beneficial when dealing with complex data structures, as it provides a clear organization of state variables.
Consistency and Atomic Updates:
- Updating a single object state ensures consistency in state updates. You can perform atomic updates by spreading the existing state and only modifying the properties that need to change.
Easier to Pass as Props:
- When passing state down to child components, it's often more convenient to pass a single object rather than multiple individual values. This reduces the number of props being passed and simplifies component interfaces.
Negatives:
Performance Concerns:
- If the object state is large or deeply nested, updating it frequently can lead to performance issues. React's shallow comparison for state updates may become less efficient with complex object structures.
Potential for Unintentional Mutations:
- When updating a single object state, it's essential to avoid unintentional mutations. Since objects are passed by reference in JavaScript, directly modifying the state object can lead to unexpected behavior.
B. Multiple Single-Value States:
Positives:
Granular Control and Precision Updates:
- Splitting state into multiple single-value states allows for granular control over each piece of state. You can update individual state variables independently, leading to more precise updates and reducing the risk of unintended side effects.
Improved Performance:
- Managing multiple single-value states may lead to better performance compared to a single large object state, especially if only a subset of state variables needs to be updated frequently. React's efficient state update mechanism works well with smaller state values.
Simpler State Initialization:
- Initializing multiple single-value states is often simpler and more straightforward than initializing a complex object state. Each state variable can have its default value without the need for nested object structures.
Negatives:
Increased Boilerplate:
- Maintaining multiple single-value states can result in increased boilerplate code, especially when dealing with a large number of state variables. Each state variable requires its declaration, initialization, and update logic.
Difficulty in Grouping Related Data:
- Splitting state into multiple single-value states may lead to difficulties in organizing and managing related data. You may need to introduce additional logic to coordinate updates across multiple state variables.
Prop Drilling:
- Passing multiple single-value states as props to deeply nested components can result in prop drilling, where intermediate components need to pass props down to their children. This can make the component hierarchy more complex and reduce code maintainability.
Example:
Consider a form component that manages user input for a user profile:
Using a Single Object State:
codeconst [formData, setFormData] = useState({ firstName: '', lastName: '', email: '', age: 0, });
Using Multiple Single-Value States:
codeconst [firstName, setFirstName] = useState(''); const [lastName, setLastName] = useState(''); const [email, setEmail] = useState(''); const [age, setAge] = useState(0);
In this example, using a single object state (formData
) allows you to group related form fields together, while using multiple single-value states provides granular control over each field. The choice depends on factors such as the complexity of the form, the frequency of state updates, and the need for data organization and consistency.
Type Checking in React Applications
Checking types for variables, especially in React applications, is important for several reasons:
Type Safety and Error Prevention:
- By checking types, you can catch type-related errors early in the development process. This helps prevent runtime errors caused by passing incorrect types of data to components or functions.
Code Robustness and Reliability:
- Ensuring that variables have the expected types improves the robustness and reliability of your code. It reduces the likelihood of unexpected behavior or crashes due to mismatched data types.
Enhanced Code Readability:
- Type annotations provide additional context and clarity to your code, making it easier for other developers (or future you) to understand the purpose and intended usage of variables and functions.
Facilitates Collaboration:
- When working in a team, type annotations serve as a form of documentation, aiding communication between team members. They help clarify the expected input and output types of functions and components, reducing misunderstandings and bugs.
Improved Developer Experience:
- Modern development tools and IDEs often provide features like type checking and autocompletion based on type annotations. These features enhance the developer experience by offering proactive suggestions and catching errors as you write code.
Better Integration with TypeScript/Flow:
- If you're using TypeScript or Flow in your React project, type checking becomes an integral part of the development workflow. Explicit type annotations enable these tools to perform static type analysis, providing additional safety and tooling support.
Prevents Runtime Errors in Production:
- Type checking helps catch potential errors during development, reducing the likelihood of encountering runtime errors in production environments. This leads to a more stable and reliable application for end users.
Overall, checking types for variables in React (or any JavaScript application) contributes to code quality, reliability, and maintainability. It's a proactive approach to writing robust code and avoiding common pitfalls associated with dynamic typing.
In React, there are several ways to check types for variables to ensure type safety and prevent errors. Here are some common approaches:
PropTypes:
- PropTypes is a built-in type-checking mechanism in React that allows you to define the expected types of props passed to components. You can specify prop types such as string, number, boolean, array, object, function, etc. PropTypes will issue warnings in the console if the actual types of props do not match the specified types.
codeimport PropTypes from 'prop-types';
function MyComponent(props) {
// propTypes definition
MyComponent.propTypes = {
name: PropTypes.string,
age: PropTypes.number,
isAdmin: PropTypes.bool,
onClick: PropTypes.func,
items: PropTypes.arrayOf(PropTypes.string),
user: PropTypes.shape({
username: PropTypes.string,
email: PropTypes.string,
}),
};
// Component implementation
return <div>{props.name}</div>;
}
TypeScript/Flow:
- TypeScript and Flow are static type-checking tools that extend JavaScript with type annotations. By using TypeScript or Flow in your React project, you can annotate variables, functions, and components with type information. These tools perform static analysis to catch type-related errors at compile time.
typescriptCopy code// TypeScript example
interface Props {
name: string;
age: number;
isAdmin: boolean;
onClick: () => void;
items: string[];
user: {
username: string;
email: string;
};
}
function MyComponent(props: Props) {
return <div>{props.name}</div>;
}
Runtime Type Checking Libraries:
- There are libraries available, such as
prop-types
for PropTypes ortcomb
for runtime type checking, that enable you to perform type checking at runtime. These libraries allow you to define types and validate them during development or in development builds of your application.
- There are libraries available, such as
codeimport PropTypes from 'prop-types';
import t from 'tcomb';
const Person = t.struct({
name: t.String,
age: t.Number,
});
function MyComponent(props) {
// Validate prop types at runtime
PropTypes.checkPropTypes(MyComponent.propTypes, props, 'prop', 'MyComponent');
// Validate object type at runtime
const person = new Person(props.person);
return <div>{person.name}</div>;
}
Manual Type Checking:
- For simpler cases, you can perform manual type checking using JavaScript's built-in type checking mechanisms such as
typeof
orinstanceof
. While less robust than other approaches, manual type checking can still be effective for basic type validation.
- For simpler cases, you can perform manual type checking using JavaScript's built-in type checking mechanisms such as
codefunction MyComponent(props) {
if (typeof props.name !== 'string') {
console.warn('Expected "name" prop to be a string.');
}
return <div>{props.name}</div>;
}
Each of these approaches has its pros and cons, and the choice depends on factors such as project requirements, developer preferences, and existing tooling in your project. Combining multiple approaches can provide comprehensive type checking and ensure the reliability and robustness of your React applications.
Using PropTypes in React has several advantages and disadvantages compared to other approaches for type checking. Here's a breakdown:
Advantages of PropTypes:
Ease of Use:
- PropTypes is built into React, making it easy to start using without additional setup or configuration. It provides a simple and familiar syntax for defining prop types directly within components.
Clear Documentation and Readability:
- PropTypes offer clear and concise documentation within component definitions, making it easy for developers to understand the expected types of props. This improves code readability and helps maintain consistency across components.
Runtime Validation:
- PropTypes perform runtime validation of props, issuing warnings in the console when prop types do not match the specified types. This helps catch type-related errors early during development and prevents them from reaching production.
Integration with Development Tools:
- PropTypes integrate well with development tools such as ESLint and IDEs, providing additional linting and autocompletion support based on prop type definitions. This enhances the developer experience and improves productivity.
Disadvantages of PropTypes:
Limited Type Support:
- PropTypes support a limited set of basic types (e.g., string, number, boolean, array, object, etc.) and some PropTypes (e.g., shape, arrayOf, oneOfType) for more complex types. However, it lacks support for more advanced type constructs available in TypeScript or Flow.
Runtime Overhead:
- Runtime type checking performed by PropTypes incurs a small performance overhead, especially in large applications with numerous components and prop validations. This overhead may be negligible in most cases but could become noticeable in performance-critical applications.
Less Robust Type Inference:
- PropTypes rely on runtime inference of prop types based on usage within components. While this approach works well for most cases, it may lead to less robust type checking compared to static type systems like TypeScript or Flow, which perform static analysis based on type annotations.
Not Suitable for Large Projects:
- PropTypes may become cumbersome to manage and maintain in large-scale projects with numerous components and complex data structures. In such cases, a more robust type-checking solution like TypeScript or Flow may offer better scalability and maintainability.
In summary, PropTypes provide a convenient and lightweight solution for type checking in React applications, offering runtime validation, ease of use, and integration with development tools. However, they have limitations in terms of type support, runtime overhead, and robustness compared to other approaches like TypeScript or Flow. The choice of type-checking solution depends on the specific requirements and constraints of your project.
Summary
Summary: The content discusses key best practices in React, including dividing projects into components, using fragments, and managing states. It explores when to create components, the benefits of using fragments, and the relationship between states and objects. It also covers type checking in React, various approaches like PropTypes, TypeScript, and runtime type checking, along with their advantages and disadvantages.
Text generated by OpenAI's ChatGPT based on questions and answers with the Sunney Sood.
Subscribe to my newsletter
Read articles from Sunney Sood directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
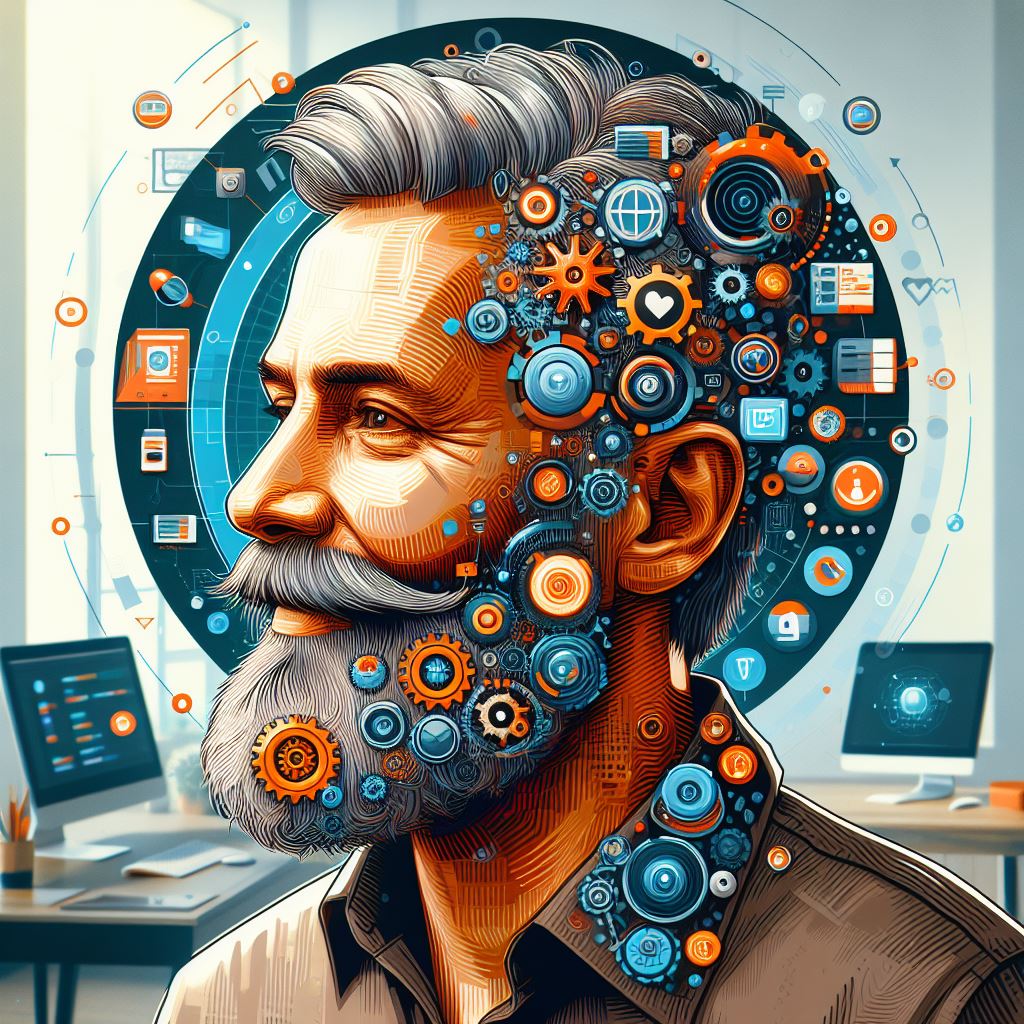
Sunney Sood
Sunney Sood
Profile Summary: Sunney Sood is a Program Manager who in spare time is DevOps enthusiast with exceptional leadership and problem-solving skills. Sunney is adept at managing software development lifecycles and bridging the gap between technical and non-technical team members. With real-world experience from professional projects and internships, he aspire to pursue a career in DevOps and Cloud. Skills: DevOps tools (Jenkins, Docker, Kubernetes, Git, Terraform), scripting (Python, Shell), project management (Agile).