How to Optimize ReactJS Performance: Strategies for Speed and Efficiency
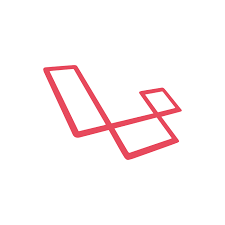
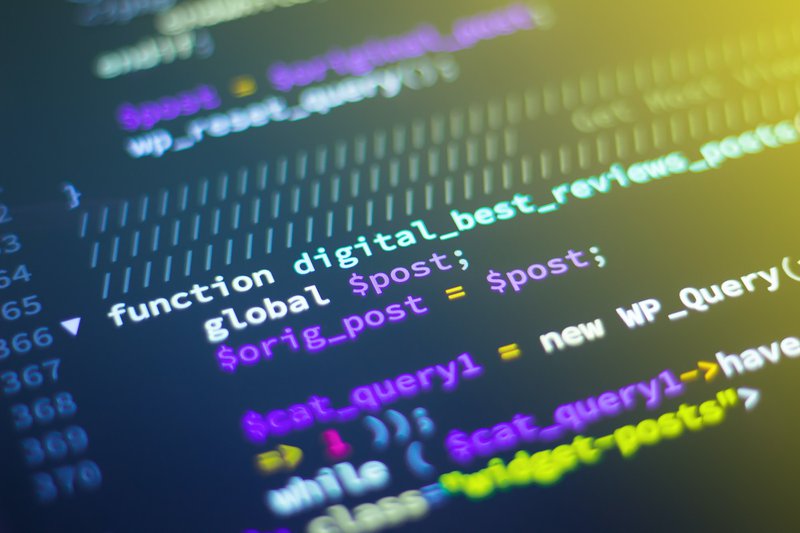
ReactJS has become one of the most popular JavaScript libraries for building user interfaces, thanks to its declarative and component-based approach. However, as applications grow in complexity, ensuring optimal performance becomes crucial for delivering a smooth user experience. In this article, we'll explore various strategies to optimize ReactJS performance, focusing on speed and efficiency.
Introduction to ReactJS Performance Optimization
What is ReactJS?
ReactJS, developed by Facebook, is a JavaScript library for building interactive user interfaces. It allows developers to create reusable UI components and efficiently manage the state of an application.
Importance of Optimizing ReactJS Performance
Optimizing ReactJS performance is essential for several reasons. It directly impacts user experience, with faster load times and smoother interactions leading to higher user satisfaction and engagement. Additionally, improved performance can result in better search engine rankings and reduced server costs.
Understanding the Key Performance Metrics
Before diving into optimization strategies, it's essential to understand the key metrics used to measure ReactJS performance.
Rendering Time
Rendering time refers to the time it takes for React to render components and update the DOM in response to state or prop changes. Minimizing rendering time is crucial for achieving fast and responsive user interfaces.
Time to Interactive
Time to interactive measures how long it takes for a web page to become fully interactive, meaning users can interact with elements and perform actions. A shorter time to interactive enhances user engagement and satisfaction.
Bundle Size
Bundle size refers to the total size of JavaScript files required to run a React application. Smaller bundle sizes result in faster load times, especially on slower network connections.
Strategies for Improving ReactJS Performance
There are several strategies for optimizing ReactJS performance, ranging from component-level optimizations to network request optimization techniques.
Component Optimization
Optimizing individual components is one of the most effective ways to improve ReactJS performance. This involves minimizing unnecessary re-renders by using shouldComponentUpdate or React.memo, and breaking down complex components into smaller, more manageable ones.
Memoization and useMemo
Memoization is a technique used to optimize expensive function calls by caching their results. In React, the useMemo hook can be used to memoize values and prevent unnecessary recalculations, improving performance.
Virtualization Techniques
Virtualization techniques such as windowing and pagination can significantly improve the performance of large lists or tables in React applications. By rendering only the visible items, virtualization reduces the amount of DOM manipulation and improves rendering performance.
Code Splitting
Code splitting involves splitting the codebase into smaller chunks and loading them asynchronously. This can improve initial load times by only loading the code that is necessary for the current view, reducing bundle size and improving perceived performance.
Lazy Loading
Lazy loading is a technique used to defer the loading of non-essential resources until they are needed. In React, lazy loading can be implemented using React.lazy and Suspense, allowing components to be loaded asynchronously when they are rendered on the screen.
Tree Shaking
Tree shaking is a process of eliminating dead code or unused modules from the final bundle. By removing unused code, tree shaking reduces bundle size and improves load times, resulting in better performance.
Optimizing Network Requests
Optimizing network requests is essential for improving the overall performance of ReactJS applications, especially those that rely heavily on data fetching from APIs.
Reducing API Calls
Minimizing the number of API calls by combining multiple requests or caching data locally can significantly reduce network latency and improve application performance.
Data Caching
Caching frequently accessed data locally or using browser caching mechanisms can reduce the need for repeated network requests, resulting in faster data retrieval and improved responsiveness.
Optimizing Data Fetching
Optimizing data fetching involves techniques such as prefetching data, using WebSockets for real-time updates, and implementing server-side rendering to improve initial load times and reduce time to interactive.
Profiling and Debugging Performance Issues
Profiling and debugging tools are invaluable for identifying and resolving performance issues in ReactJS applications.
Using Browser Developer Tools
Modern web browsers offer developer tools that allow developers to profile and debug JavaScript code, analyze network requests, and identify performance bottlenecks.
Profiling with React DevTools
React DevTools is a browser extension that provides insights into the performance of React components, including rendering times, component lifecycles, and state changes.
Identifying and Resolving Performance Bottlenecks
Once performance issues are identified, developers can take steps to address them, such as optimizing critical rendering paths, reducing JavaScript execution time, and eliminating unnecessary re-renders.
Testing and Benchmarking
Testing and benchmarking are essential steps in ensuring the effectiveness of performance optimization efforts.
Setting up Performance Benchmarks
Establishing performance benchmarks allows developers to measure the impact of optimization strategies and track improvements over time.
Automated Testing for Performance
Automated testing frameworks such as Jest and React Testing Library can be used to create performance tests that simulate user interactions and measure key performance metrics.
Conclusion
Optimizing ReactJS performance is crucial for delivering fast and responsive user experiences. By understanding key performance metrics and implementing effective optimization strategies, hire dedicated AngularJS aevelopers can ensure their React applications perform efficiently, leading to improved user satisfaction and engagement.
FAQs
How do I know if my React application needs performance optimization?
- You may need performance optimization if your application experiences slow load times, laggy interactions, or high resource usage.
What are some common performance bottlenecks in React applications?
- Common performance bottlenecks include excessive re-renders, large bundle sizes, inefficient data fetching, and unnecessary API calls.
Does optimizing React performance require sacrificing code quality?
- No, optimizing React performance can actually lead to improved code quality by encouraging modularization, code splitting, and efficient data management.
How often should I monitor and optimize ReactJS performance?
- It's recommended to monitor and optimize ReactJS performance regularly, especially after making significant changes to the codebase or adding new features.
Are there any tools available for automated performance testing in React?
- Yes, tools like Jest, React Testing Library, and Lighthouse can be used for automated performance testing and benchmarking in React applications.
Subscribe to my newsletter
Read articles from Hire Laravel Developers directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
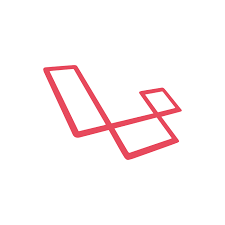
Hire Laravel Developers
Hire Laravel Developers
Discover top Laravel developers for hire on Hashnode.com. Elevate your projects with our skilled professionals. Let's collaborate and bring your ideas to life!