Operation in arrays

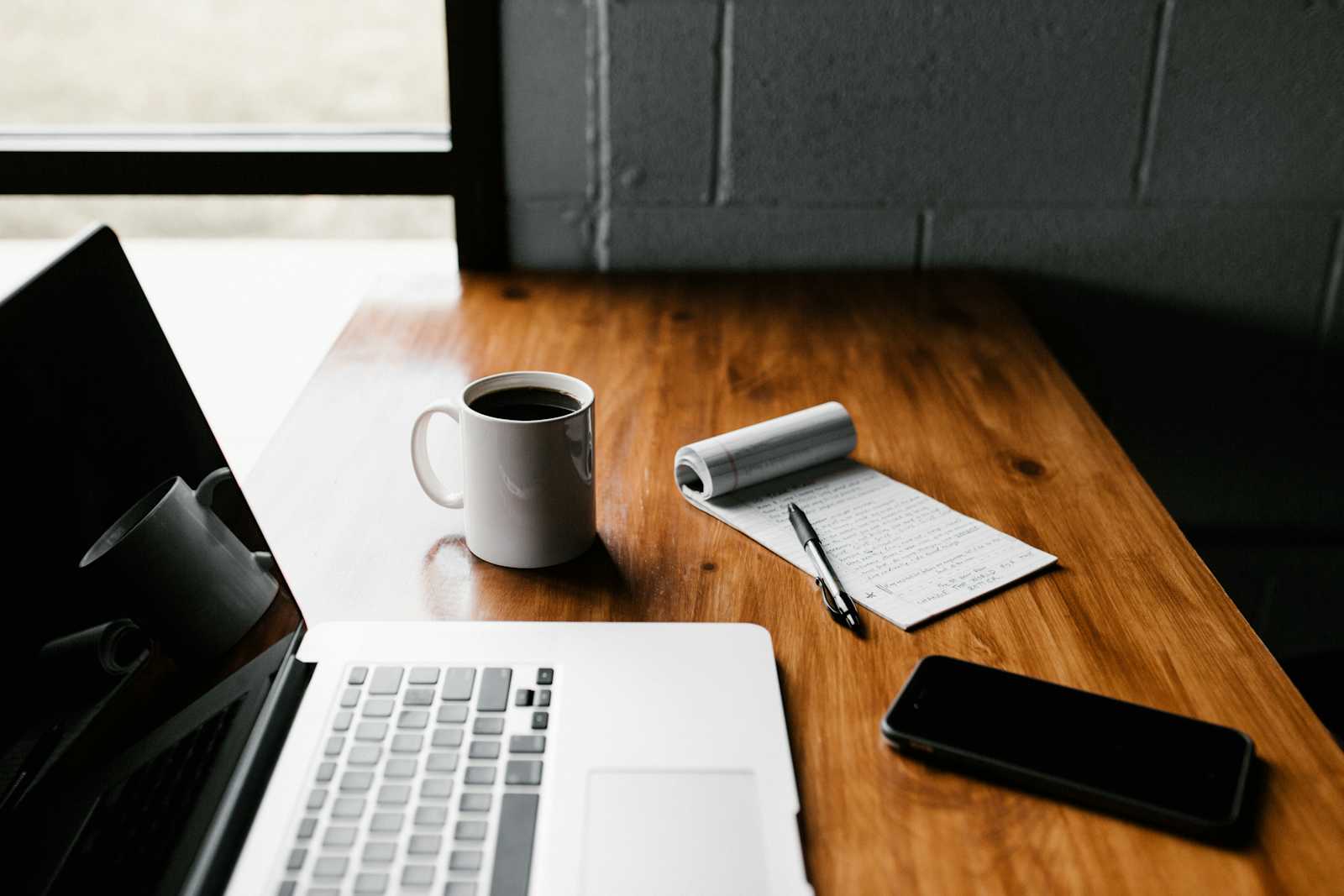
In this blog we are going to learn how to Insert, add, replace, or delete in an array. We have several methods for doing such operations. let us discuss one by one.
//let us take an array
let names = ["jeet", "ayon", "debraj", "souvik", "sonya"];
//we have push() method that adds one or more elemet to the
//end of an array, push method returns the new length
console.log(names.push("jay"));
//we have pop() method to pop out the last element fromthe array
console.log(names.pop());
console.log(names);
//we have unshift method to insert an elemet at the begining of
//of the array
console.log(names.unshift("pritam"));//prints the length
console.log(names);//prints the updated array
//we have shift method that removes the first elemnt from the array
console.log(names.shift());
console.log(names);
what if we want to add or delete element from a specific position, this can be easily done in javaScript with some specific method.
''The splice()
method of array instances changes the contents of an array by removing or replacing existing elements and/or adding new elements in place''.
The basic syntax of splice()
method is :
''The splice()
method of array instances changes the contents of an array by removing or replacing existing elements and/or adding new elements in place''.
The basic syntax of the splice()
method is:
splice(start, deleteCount, item1, item2 ...... itemN);
//start says from where to start
//deleteCount reffers how many elemts need to be deleted
let names = ["jeet", "ayon", "debraj", "souvik", "sonya");
//suppose i want to delete fiest two names thad then enlist another
//name in place of that, the systax would be like...
names.splice(0, 2, "rahul");
console.log(names);
//what of we want to inser at end or begining without deleting
names.splice(-1, 0, "rahul");
names.splice(1, 0, "rahul");
console.log(names);
Searching in arrays
In JavaScript, for searching in arrays, we have three methods:
indexOf()
lastIndexOf()
includes() method
let num = [1, 2, 3, 4, 5, 6, 4, 7, 8];
console.log(num.indexOf(3)); //output 2
console.log(num.lastIndexOf(4)); //output 6
//also indexOf method has this sysntax
//indexOf(searchElement, fromIndex);
console.log(num.indexOf(4, 5)); // output 6
//includes() method returns true or false
const result = num.includes(5);
console.log(result);
We have the find()
method which is used to find the first element in the array that satisfies a provided testing function. It returns the first matching element or undefined if no element is found. The syntax is like :
const num = [1, 2, 3, 4, 5, 6, 4, 7, 8, 10, 15];
const result = num.find((curElem) => {
return curElem > 6;
});
console.log(result);
The findIndex()
method also does the same work, but it returns the index number of the element.
const nums = [3, 10, 18, 12, 20, 43];
let res = nums.findIndex(checkNum);
function checkNum(Num) {
return Num > 18;
}
console.log(res);
We can filter an array with the help of filter method , this method creates a new array with all the elements that pass the test of the provided function.
// the syntax is like
//filter(callbackFunction)
//? filter(callbackFuntion, thisArg)
const num = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const result = num.filter((currElem) => {
return currElem > 3;
});
console.log(result);
//Example: Filtering books by Price
const books = [
{ name: "EASY JS GUIDE", price: 1300 },
{ name: "C++ GUIDE", price: 700 },
{ name: "LEARN PYTHON", price: 300 },
{ name: "START SQL", price: 150 },
];
// Filter books with a price less than or equal to 700
const filterbooks = books.filter((curElem) => {
return curElem.price <= 700;
});
console.log(filterbooks);
"The reduce method in JavaScript is used to accumulate or reduce an array to a single value. It iterates over the elements of an array and applies a callback function to each element, updating an accumulator value with the result. The reduce method takes a callback function as its first argument and an optional initial value for the accumulator as the second argument."
the syntax is like this:
array.reduce(callback fn (accumulator, currentValue, index, array){
// Your logic here
//Return the updated accumulator value
}, initialValue);
// write a code to add the total of the array
const Price = [100, 200, 300, 400, 500];
const totalPrice = Price.reduce((accum, curElem) => {
return accum + curElem;
}, 0);
console.log(totalPrice);
//output : 1500
copy the code ,do research and practice by yourself.
Subscribe to my newsletter
Read articles from Jeet kangsabanik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Jeet kangsabanik
Jeet kangsabanik
I am jeet , experienced full-stack developer proficient in HTML, CSS, JavaScript, React, MongoDB, MySQL, and Express.js. Dedicated to creating innovative and robust web applications with a keen eye for design and a commitment to delivering high-quality, scalable solutions that meet and exceed client expectations.