Essential Guide to Angular Library π¦
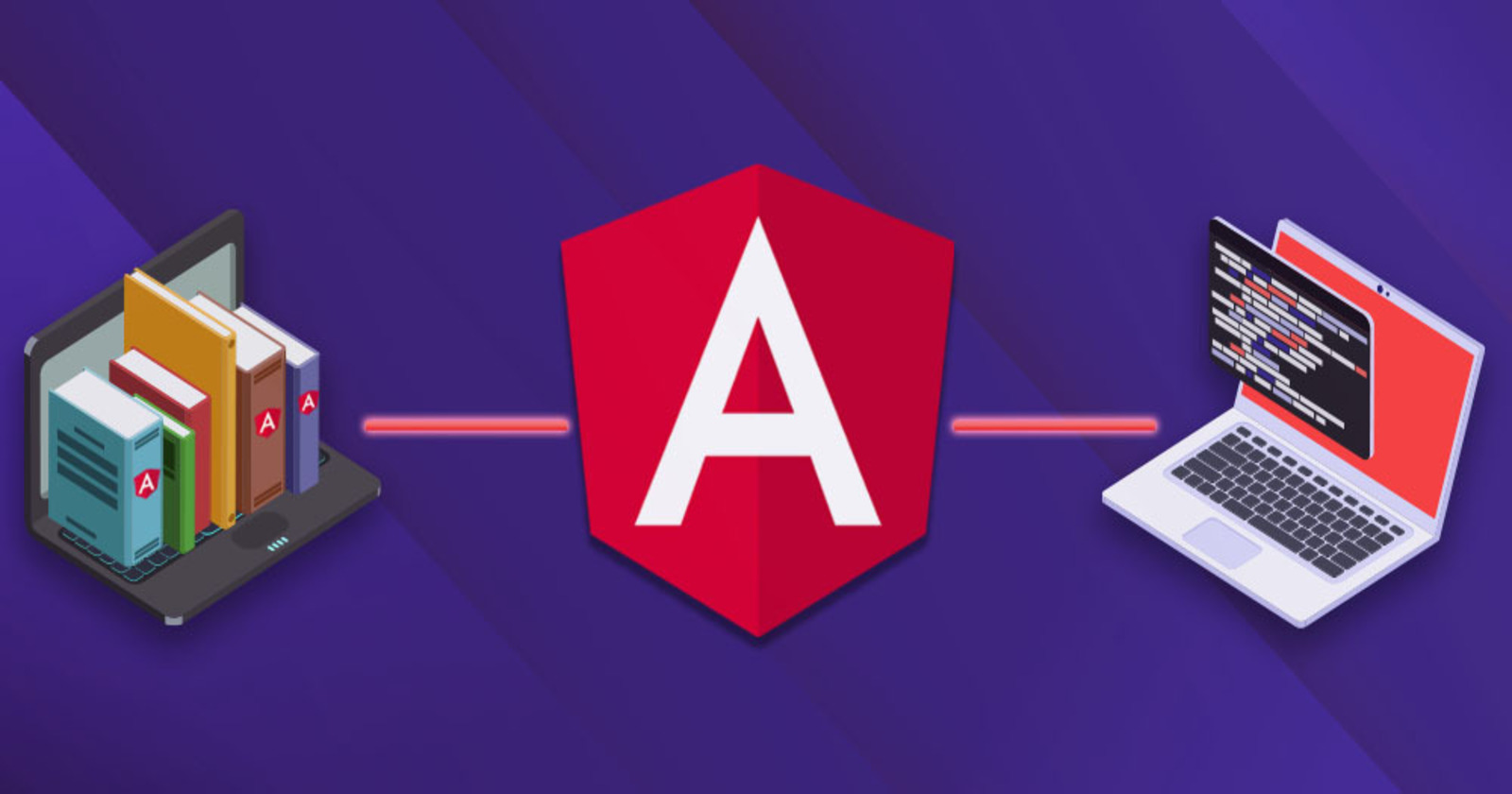
Hello Techies π€
Have you ever created a package and published it on NPM?
Have you ever used your package in a separate project?
Have you ever tried using Angular library?
In this article, weβre going to walk through the steps of creating a generic library, how to build and publish it on NPM, and how to use it in your application.
what is a package/library? π¦
A package (also known as a library
) in programming is a bundle of reusable code that can be used to perform common tasks. Packages allow developers to write code once and then reuse it across different projects, which can greatly improve productivity and code maintainability.
More simply, A library is a place that holds the logic to make a specific task for you that can be reused anywhere.
Think of it like a container that holds your logic/feature. Whenever you want this feature in your project, you only have to install and import this container in your project and use it properly.
A library provides a set of functions, classes, or methods (collectively known as APIs
or Application Programming Interfaces
) that you can use in your code. These APIs define how you can interact with the functionality provided by the library.
What is Angular Library?
An Angular library is an Angular project that differs from an application in that it cannot run on its own. A library must be imported and used in an application. Libraries extend Angular's base features.
Angular library is used for angular applications only.
Naming Convention of Angular Libraries π‘
Avoid using a name that is prefixed with ng-
, such as ng-library
. The ng-
prefix is a reserved keyword used by the Angular framework and its libraries.
The ngx-
prefix is preferred as a convention used to denote that the library is suitable for use with Angular
this is an example of how you should name your Angular library
ngx-package-name
ngx-arkami-arabic-converter
How to create an angular library?
Create a workspace
ng new my-workspace --no-create-application
cd my-workspace
Create the angular library
ng generate library my-lib
Create the feature you will offer
Build and Publish it on NPM
Use it in your application
How to publish it on NPM
First, you need to build it by running
ng build my-lib
This command will build the package and the files that will be uploaded and used in npm in
dist
directory
Second, you need to publish it on npm by running
npm publish dist/my-lib
Here you publish the build version of the package
Time to Practice π¨π»βπ»
Let's try a real-world example
I wanted to create my own Tafqit Library and use it in an Application which responsible of converting arabic numbers into their text representation
β Tafqit is a package for converting Arabic numerals into their equivalent in words
Creating an Angular Library π¦
Creating a new Workspace named
ngx-arkami-arabic-converter
ng new ngx-arkami-arabic-converter --no-create-application
change the directory to the workspace
cd ngx-arkami-arabic-converter
creating
ngx-arkami-arabic-converter
library β started withngx-
to denote that the library is for angular apps βng generate library ngx-arkami-arabic-converter
this command will create a
projects
directory that hasngx-arkami-arabic-converter
directory which hassrc/lib
including component, service, module, and spec files
- Implemented a service
ngx-arkami-arabic-converter.service.ts
that hasconvertToArabicWords
method that does the logic of getting the number as a string value and converting it to text representation and returning the text representation as a result
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class NgxArkamiArabicConverterService {
convertToArabicWords(strNumber: string): string {
let result = '';
let length = strNumber.length;
switch (length) {
case 7:
result = this.sevenDigitsToArabic(strNumber);
break;
case 6:
result = this.sixDigitsToArabic(strNumber);
break;
case 5:
result = this.fiveDigitsToArabic(strNumber);
break;
case 4:
result = this.FourDigitsToArabic(strNumber);
break;
case 3:
result = this.threeDigitsToArabic(strNumber);
break;
case 2:
result = this.twoDigitToArabic(strNumber);
break;
case 1:
result = this.oneDigitToArabic(strNumber);
break;
}
return 'ΩΩΨ· ' + result.trim() + (parseInt(strNumber) === 1 ? ' Ψ¬ΩΩΩ Ω
Ψ΅Ψ±Ω ΩΨ§ ΨΊΩΨ±' : ' Ψ¬ΩΩΩΨ§Ψͺ Ω
Ψ΅Ψ±ΩΨ© ΩΨ§ ΨΊΩΨ±');
}
}
- Implemented a Component
ngx-arkami-arabic-converter.component.ts
that uses the service, gets thestring number
as a component input, and displays thetext representation
in the component after converting it.
import { Component, Input, OnChanges, OnInit, SimpleChanges } from '@angular/core';
import { NgxArkamiArabicConverterService } from './ngx-arkami-arabic-converter.service';
@Component({
selector: 'ngx-arkami-arabic-converter',
template: `
<p *ngIf="arabicText">
{{ arabicText }}
</p>
`,
styles: [
]
})
export class NgxArkamiArabicConverterComponent implements OnInit, OnChanges{
@Input() strNumber: string = '';
arabicText: string = '';
constructor(
private ngxArkamiService: NgxArkamiArabicConverterService
) { }
ngOnInit(): void {
if (this.strNumber){
this.arabicText = this.ngxArkamiService.convertToArabicWords(this.strNumber);
}
}
ngOnChanges(changes: SimpleChanges): void {
if (changes['strNumber']) {
this.arabicText = this.ngxArkamiService.convertToArabicWords(this.strNumber);
}
}
}
- To make your service accessible by your component, you have to add it to the providers besides declaration, imports, and exports in
ngx-arkami-arabic-converter.module.ts
@NgModule({
declarations: [
NgxArkamiArabicConverterComponent
],
providers: [
NgxArkamiArabicConverterService
],
imports: [
CommonModule
],
exports: [
NgxArkamiArabicConverterComponent
]
})
Prepare the Manifest file π¨
The package.json
file is a manifest file that contains metadata about the library. This file is used to give information to npm
that allows it to identify the project as well as handle the project's dependencies. It can also contain other metadata such as a project description, the version of the project, license information, and even configuration data - all of which can be vital to both npm
and the end users of the package.
check the version of your library before you publish it in package.json
β You need to increment the version after changes and publish it againβ
Publishing the library to NPM πͺπ»
- navigate to your workspace directory and run
ng build ngx-arkami-arabic-converter
, this will create adist/
directory with the build artifacts
- To publish the library to your NPM, run
npm publish dist/ngx-arkami-arabic-converter
, this will upload the dist folder on NPM
Using the Library in the Application π―
To install this library in your application, run:
npm i ngx-arkami-arabic-converter
Usage
first Approach: Using Service
// import NgxArkamiArabicConverterService in your component
import { NgxArkamiArabicConverterService } from './ngx-arkami-arabic-converter.service';
// inject your service in the component constructor
constructor(
private ngxArkamiService: NgxArkamiArabicConverterService
) {}
// Use `convertToArabicWords` method and pass the string number to it
// It will return the text representation
const arabicTextRepresentation = this.ngxArkamiService.convertToArabicWords(stringNumber);
Second Approach: using Component
import NgxArkamiArabicConverterModule inapp.module.ts
// import `NgxArkamiArabicConverterModule` in your app.module
import { NgxArkamiArabicConverterModule } from './ngx-arkami-arabic-converter.service';
// add `NgxArkamiArabicConverterModule` to your imports
@NgModule({
imports: [
NgxArkamiArabicConverterModule
]
})
use ngx-arkami-arabic-converter component to render output
<!-- use ngx-arkami-arabic-converter component in your html file -->
<!-- pass the string number you want to convert to arabic text representation -->
<!-- the component will display the arabic text representation for you -->
<ngx-arkami-arabic-converter [strNumber]="inputNumber"></ngx-arkami-arabic-c
After we finished the steps of creating, build, publishing the library β ,
You can check the Demo App, any feedback is welcomeππ».
Demo Application
Live Application: https://mazenaboelanin.github.io/arkami-arabic-converter-app/
NPM: https://www.npmjs.com/package/ngx-arkami-arabic-converter
GitHub: https://github.com/mazenaboelanin/ngx-arkami-arabic-converter
What about Libraries for Non-Angular Applications
How can I create reusable components/services that can be used for non-Angular applications?
Here's where the importance of Angular Elements comes
This is the topic of the next article, Stay Tuned π
Subscribe to my newsletter
Read articles from Mazen Abo Elanin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Mazen Abo Elanin
Mazen Abo Elanin
a Software Engineer with 2+ years of professional software development experience in designing, developing, and maintaining Software solutions that serve end users in their businesses and make their life easier.