All About Python Lists

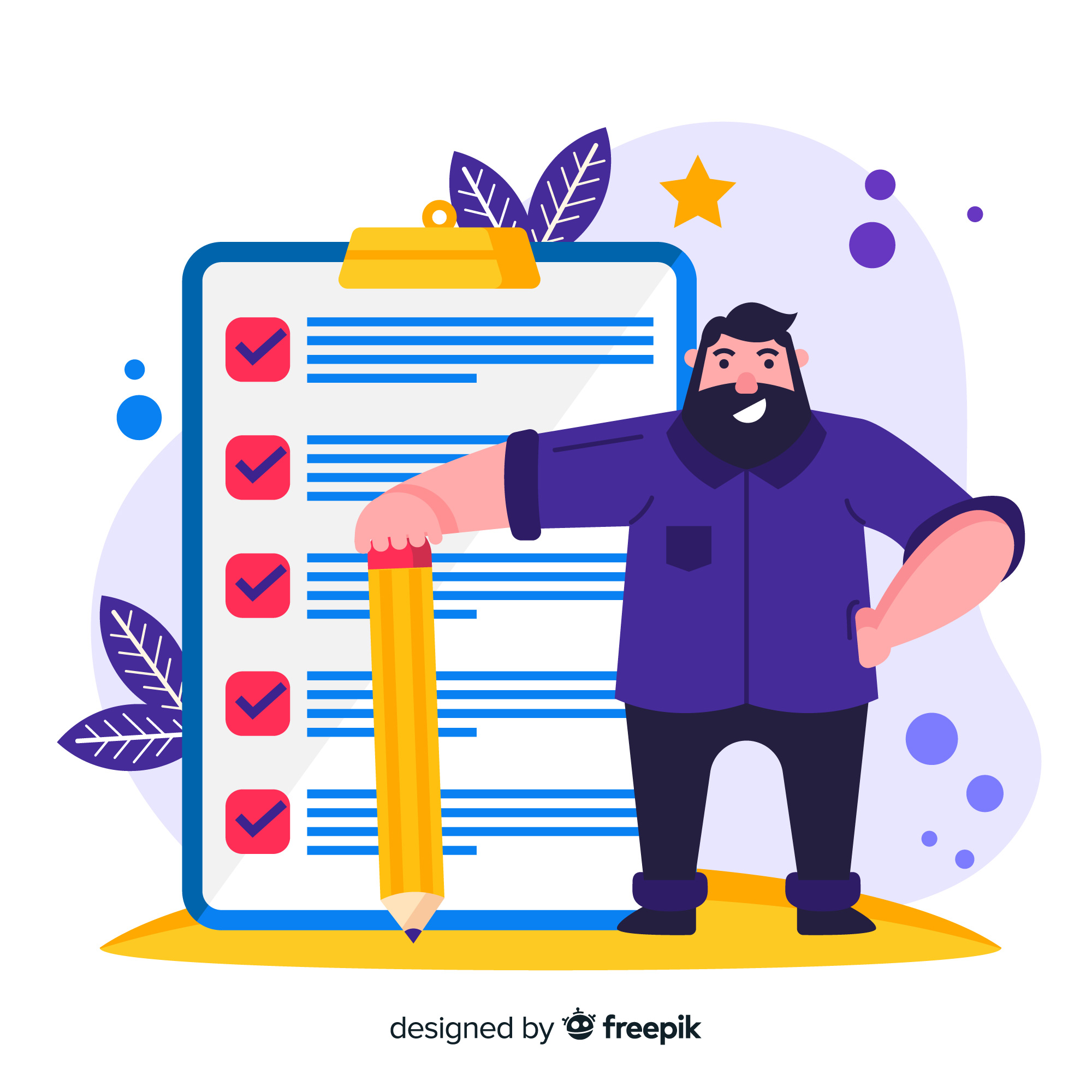
Aloha again Python newbies and non-newbies alike. We would be talking about lists today; perhaps one of the most used data types in programming. You may well be aware that programming languages consists of different data types from the primitive types such as strings and integers to the more "collectable" types that are regarded as iterables (of which dictionaries, tuples and sets are examples); helping to house or contain other data types and use them in a variety of ways in programs.
Lists are a favorite kind of data type in python. They usually appear as square brackets on either end of some data separated by commas. An example of a list is found below;
#Below is a list of numbers (integers)
myList = [1,2,3,4,5,6]
Lists can house any data type, including other lists.
myList = [1,2,3,[4,5],(6,7,8),"words"]
The above example may look a little crazy but it is a valid list and can be used in a program. You're probably wondering what on God's earth we would need to do to access individual items in the list and tuple present in myList. We would get to that soon.
Accessing/Retrieving Items in Lists
Items in lists can be accessed by something known as an index. Whenever we refer to positions of items in collectibles such as tuples, lists or sets in any programming language, we do not start numbering with '1' as we would normally do, but we begin with '0'. Therefore in the second myList above, the first item is at position 0, the second at position 1, the third at 2 an so on. If I asked for the position or index of the number 3 in the list above, you would say it is at index 2 and not 3. If you're totally new to programming, it may take some getting used to but in no time it becomes as easy as knowing what feet your shoes go on. This is how we access items in lists. We type in the variable that holds the list and in square brackets type in the index as in the example below:
myList = [1,2,3,4,5,6]
print(myList[4])
#This would print 5 as the result.
This is all smooth and dandy but there is also a thing called negative indexing. Here we use negative numbers for indexing, and it is generally just the opposite of the usual indexing; meaning that '-1' refers to the position of the last item in the list, '-2' is the second to the last item, '-3' is the third to the...you get the idea.
myList = [1,2,3,4,5,6]
print(myList[-1])
#This would print 6 as the result.
#What do you think print(myList[-3]) would give?
You are probably thinking about the first myList example we gave above where a list was contained in a bigger list. How do we get the items in the mini list? Let's see how.
myList = [1,2,3,[4,5],(6,7,8),"words"]
print(myList[3][1])
#Here, the result would be 5. This is because the mini list is at
#index 3 and within it, 5 is at index 1. Lists like these are
#reffered to as multidimensional lists, becauase they exist in more
#than one dimension.
You can apply the same logic to the tuple in myList. Do say kind person, how would I go about printing 7 in the tuple above?
Slicing Lists like Cakes
A great thing about iterables is that we are not limited to only accessing an item at a time. If we would like to access a chunk of a list cake, we would be able to do so. We do this with something aptly named slicing.
In slicing, we are able to take a range off a list and print it out (if that's what we want to do with it).
myList = [1,2,3,4,5,6]
print(myList[2:5])
#This prints [3,4,5]. Notice the last number indexed with 5 is not
#printed. This is just how slicing works. The number after ':' is
#ignored
The slicing notation for ease of reference can be read as "start: stop: step". The first number (start) is the index it begins with, the second number (end) is the last index that is generally ignored, so the count stops at the item in a position before that and the last number (step) determines in what intervals the result should be printed. Look at the example below:
myList = [1,2,3,4,5,6,7,8,9,10]
print(myList[2:9:2])
#This prints [3,5,7,9] because it steps over a number each time and
#displays the second number after that. By default, step is '1'."""
It should be noted that if we were to leave out the 'start', the default would be the index of the first item on the list and if we leave out the 'end' the default would be the index of the last item on the list so what do you think "[2:]" in the above would print?
Adding Stuff to Lists
There is a method in python that is used to add new items to the end of lists; this is known as append. If you'd rather decide exactly where you want to add an item in your list, you would use the insert method instead. With this, you add an index as an argument and the item would be inserted in that position. Lastly, if you'd like to add a list to another, you could use the extend method. Examples showing each method are shown below:
#Using append
myList = [1,2,3,4,5,6,7,8]
myList.append(9)
print(myList)
#The list would be altered and [1,2,3,4,5,6,7,8,9] would be printed
#(notice 9 added at the end)
#Using insert
myList = [1,2,3,4,5,6,7,8]
myList.insert(2,9)
print(myList)
#[1,2,9,3,4,5,6,7,8] would be printed. The first argument is the index
#in which you wish to insert the item and the second argument is the
#item.
#Using extend
myList1 = [1,2,3,4]
myList2 = [5,6,7,8]
myList1.extend(myList2)
print(myList1)
#myList1 becomes [1,2,3,4,5,6,7,8] because myList 2 has been added
#to it, thereby extending it.
It should be worth noting based on the last example that you could also create a third variable (such as myList3) and add myList1 to myList2 (myList1 + myList2) to achieve the same result as shown in the snippet above but this is not considered to be adding items to an existing list since a completely different list is created.
Altering Lists
If you created a list of the best characters on Star Trek but realize that you added Sulu and you really don't think Sulu should be on the list (for whatever reason) it only makes sense to change the list. Lists are mutable so lucky us in that regard.
#Changing an item on a list
bestOnStarTrek = ["Kirk", "Spock", "Uhura", "Sulu"]
#Nooo Sulu must go!
bestOnStarTrek[3] = "Chekov"
print(bestOnStarTrek)
All you did was figure out the position of Sulu on the list by getting its index, changing the item on the index by equating it to some other value and just like that! Chekov replaced Sulu on the list (for whatever reasons known to you alone).
If you would like to change multiple items at a time, just do what you did but this time with slicing. For instance;
#Changing items on a list
bestOnStarTrek = ["Kirk", "Spock", "Uhura", "Sulu"]
#Sorry Sulu and Uhura...
bestOnStarTrek[2:] = ["Chekov", "McCoy"]
print(bestOnStarTrek)
Getting Rid of Items on Lists
Only four methods/attributes are important when removing items in Python. These are well...remove,pop, del and clear. See how they work with the code snippet below.
#Using remove
toDo = ["brush cat", "read book", "take over the world"]
toDo.remove("take over the world")
print(toDo)
#With remove, we specify the item we wish to remove as an argument.
#Using pop
toDo = ["brush cat", "read book", "take over the world"]
toDo.pop()
print(toDo)
#With pop(), the last item in the list is removed and we are kind of
#happy it's that one.
toDo = ["brush cat", "read book", "take over the world"]
toDo.pop(1)
print(toDo)
#Here however, when an index is specified as an argument, that item
#is removed instead. Well, sorry world.
#Using del
toDo = ["brush cat", "read book", "take over the world"]
del toDo[0]
print(toDo)
#The item indicated (with index 0) is removed.
toDo = ["brush cat", "read book", "take over the world"]
del toDo
print(toDo)
#You would see an angry error here because this deletes the list;
#Hence the list would cease to exist and be undefined.
#Using clear
toDo = ["brush cat", "read book", "take over the world"]
toDo.clear()
print(toDo)
#While the list would still exist, its content would be erased so
#you would be left with an empty list.
Sorting and Copying Lists
If you would like to make sure that your lists are in either alphabetical or numerical order, you could do that easily with the sort method.
#Sorting alphabetically
toDo = ["read book", "brush cat", "take over the world"]
toDo.sort()
print(toDo)
#Sorting numerically
toDo = [9,5,6,2,1,4]
toDo.sort()
print(toDo)
You could also sort lists in descending order.
#Sorting alphabetically
toDo = ["brush cat", "read book", "take over the world"]
toDo.sort(reverse = True)
print(toDo)
#Sorting numerically
toDo = [9,5,6,2,1,4]
toDo.sort(reverse = True)
print(toDo)
Copying Lists
Copying lists can also be done as easily as sorting them, with the copy method.
#Copying lists
toDo = ["brush cat", "read book", "take over the world"]
newTodo = toDo.copy()
print(newTodo)
Getting the Length of Lists
This is easily done by using len. Check the code snippet below.
toDo = ["brush cat", "read book", "take over the world"]
print(len(toDo))
# This prints 3 which is the length (number of items) of toDo.
In the next few posts we would be looking at loops and in doing so, find interesting ways to use and manipulate lists. Try not to take over the world before then...
Subscribe to my newsletter
Read articles from Amanda Ene Adoyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amanda Ene Adoyi
Amanda Ene Adoyi
I am a Software Developer from Nigeria. I love learning about new technologies and talking about coding. I talk about coding so much that I genuinely think I could explode if I don't . I have been freelance writing for years and now I do the two things I love the most: writing and talking about tech.