Transitioning from Arrays to Singly Linked Lists: Unlocking Dynamic Flexibility
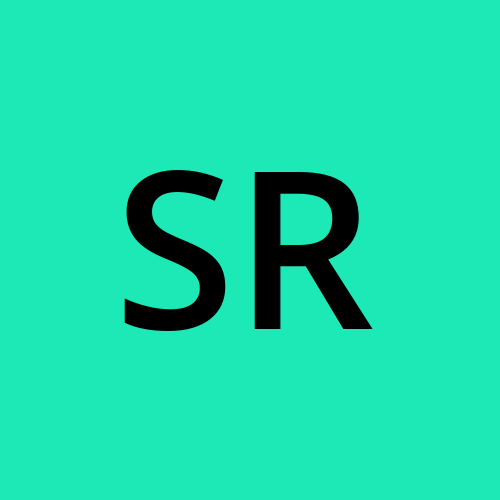
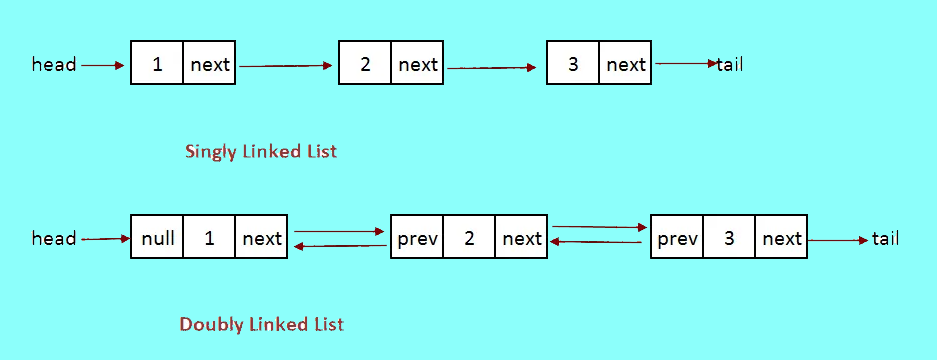
We've delved deep into arrays and learned their ins and outs. Now, it's time to take our journey a step further and explore Singly LinkedList ! ๐๐ก
But wait, why are we transitioning from arrays to LinkedList? Let's take a quick look:
๐ Arrays vs. Lists: What's the Difference?
Arrays:
Fixed-size data structures.
Allocate contiguous memory.
Require manual resizing.
Efficient for random access.
Lists:
Dynamic data structures.
Allocate memory dynamically.
Can grow or shrink as needed.
Efficient for insertions and deletions.
In Java, there are several types of lists, each with its own characteristics and use cases.
ArrayList: Implements a dynamic array that can dynamically resize itself. It's efficient for random access but less efficient for insertions and deletions in the middle of the list.
LinkedList: Implements a doubly linked list, where each element points to the next and previous elements. It's efficient for insertions and deletions, especially in the middle of the list, but less efficient for random access.
Vector: Similar to ArrayList but is synchronized, making it thread-safe. However, it's less efficient due to the synchronization overhead.
Stack: Implements the stack data structure, by following the Last In First Out (LIFO) principle.
Queue: Implements the queue data structure, by following the First In First Out (FIFO) principle.
Singly Linked List is a type of linked list where each element points to the next element in the sequence, forming a linear structure and has following properties :
It Contains sequence of nodes.
A node has data and reference to next node in a list.
First node in the list is the Head node.
This Head node hold the entire list
Last node of the list has only data and reference will be pointed to
null
.
REAL-TIME SCENARIO:
Imagine you're managing a playlist of songs on a music streaming app. Each song in the playlist can be represented as a node in a singly linked list.
Data Structure Representation: Each node contains data(the song) and a reference to the next song in the playlist.
Adding a Song: When you add a new song to the playlist, it becomes the new head of the list, with its reference pointing to the previous head.
Removing a Song: Removing a song form the playlist involves updating the references of the adjacent nodes to bypass the removed song.
Playing the playlist: You can traverse the playlist from the head to the tail, playing each song in sequence.
Implementation of a List Node in Singly Linked List
//Generic Type
public class ListNode<T>{
private T data;
private ListNode<T> next;
}
//Integer Type
public class ListNode{
private int data;
private ListNode next;
}
How to Implement a singly LinkedList
LinkedList Usually, has a head node which actually holds the complete list.
First we will create a instance variable of type ListNode. (i.e. private ListNode head)
As we have created the head of type ListNode, we have to create the ListNode class.
Note: Singly Linked List internally contains ListNode class. so we will create a static class by the name ListNode.
This ListNode has two properties
One is the data property (usually this data type can be any GenericType <T>
Other is the pointer to the next node in the List.
We also provide a constructor to this class and this constructor will takes the data part.
Whenever we create a list node we only provide this data part because when a new ListNode is created. The next by default points to
null
.
public class SinglyLinkedList{
private ListNode head;
private static class ListNode{
// Below are the properties of this static class
private int data; // Generic Type
private ListNode next;
// this is the constructor to the ListNode class, where data is taken and next will be pointed to null
public ListNode(int data){
this.data =data;
this.next = null;
}
}
}
How to create a singly LinkedList in Java and different ways to insert the node into the singly LinkedList
Adding the nodes and creating the Singly Linked List
Below is the Coding implementation to print the Singly Linked List
public void display() {
ListNode current = head;
while(current != null) {
System.out.print(current.data + " --> ");
current = current.next;
}
System.out.print("null");
}
Coding Implementation of Singly Linked List in Eclipse
In short, lists in Java provide a more flexible and efficient way to work with collections of data compared to arrays, making them an essential tool in any Java developer's toolkit! ๐ป๐
By understanding the differences between arrays and lists, we're ready to dive into LinkedLists and explore their power! ๐ช Stay tuned for more insights and tutorials on LinkedLists! ๐๐ป
Subscribe to my newsletter
Read articles from Sri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
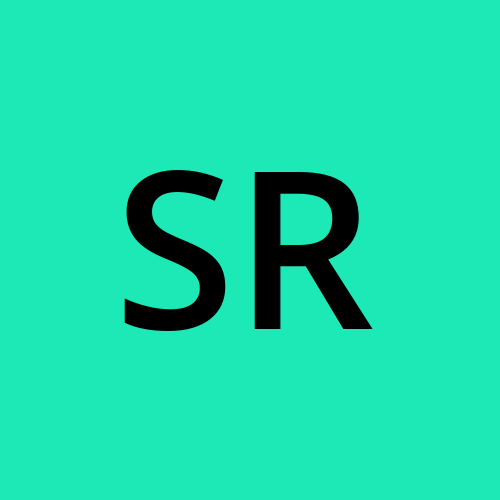