Step-by-Step Guide to Implementing Typewriter Effect in React

Table of contents
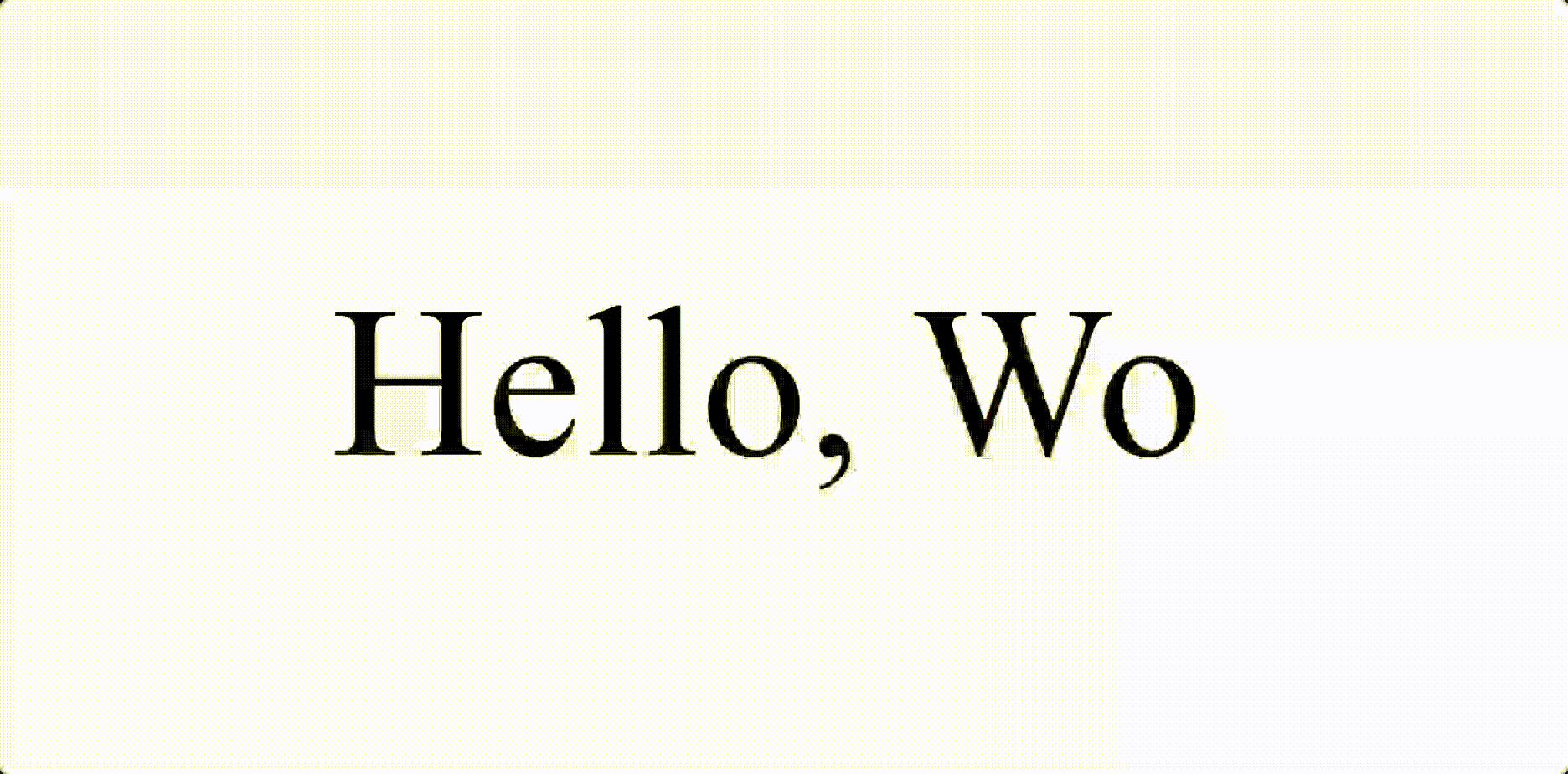
Adding interesting features to your website can grab your audience's attention in today's fast digital world. The typewriter effect, like old typewriters, is a delightful touch that can make your web content more lively. In this guide, we will show you how to create this nostalgic effect using React, making your website more dynamic and engaging for users.
Prerequisites:
- Node.js and npm (or yarn) are installed on your system.
For this walkthrough, you'll be creating a React project using Vite and Tailwind CSS for styling. While these are my preferred choices, you can absolutely tailor the setup to your existing project or preferences.
Setting up the App
Create an empty React project
npm create vite@latest react-typewiter -- --template react
Install Dependencies
cd react-typewiter yarn
Start the development server:
yarn dev
Creating the Typewriter Component
src/components/Typewriter.jsx
import PropTypes from 'prop-types';
const Typewriter = ({ text}) => {
return (
<div>
{text}
</div>
);
};
export default Typewriter;
Typewriter.propTypes = {
text: PropTypes.string.isRequired,
};
Import Typewriter Component
import Typewriter from "./src/components/Typewriter"
function App() {
return (
<div>
<Typewriter text={"Hello World"} />
</div>
)
}
export default App
Typewriter Logic
Initialize a useState index for maintaining the typed words
const [currentIndex, setCurrentIndex] = useState(0);
Now to maintain the currentIndex
to go from 0 to text.length
, Introduce a useEffect hook. What could be an easy way to do that? Of course, a setInterval could do the job.
useEffect(() => {
const interval = setInterval(() => {
setCurrentIndex((prevIndex) => {
if (prevIndex === text.length) {
clearInterval(interval);
return prevIndex;
}
return prevIndex + 1;
});
}, 100);
return () => clearInterval(interval);
}, [text]);
But we're not utilizing the currentIndex in the Component. We could simply slice the string according to the currentIndex.
text.substring(0, currentIndex)
Yay, it's complete!
This is my first article! ๐ฅณ
I would love to hear your feedback. Feel free to share your thoughts! If you found this useful and want more similar content, I'm happy to write about other features or components.
I've also added custom props like speed, color, onComplete, blinkRate, cursorChar (to change the blinking character, currently set as '|'), pauseOnHover to this component. If you'd like explanations on how these are implemented, please let me know in the comments.
I hope you enjoyed reading. ๐ค
Subscribe to my newsletter
Read articles from Pulkit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pulkit
Pulkit
Hi there! ๐ I'm Pulkit, a passionate web and app developer specializing in MERN and React Native technologies. With a keen interest in open-source contributions and competitive programming, I love building innovative solutions and sharing my knowledge with the community. Follow along for insights, tutorials, and updates on my coding journey!