MongoDB Aggregation - II [Class 3 ]
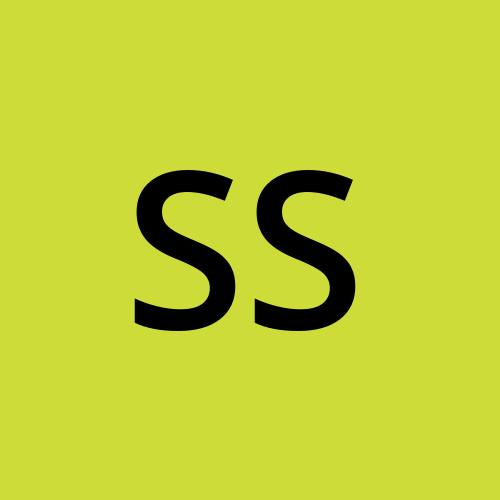
Relationships
Learnings of this class
$lookup Operator
- use to get results from combining 2 or more collections$out Operator
- to store the output in separate collection in that case $out is used.$project Operator
-$count Operator
- It will just count the number of documents so far$set
- is used to add some field in the data
Learn about
1. mongoose populate
2. ref
$Lookup Aggregation Operator
lets suppose we have two clooections
blogs collection
author collection
Now from the queries whtever we have learned till now, Will we be able to access something from blogs collection when we are working inside authors collection?
lets say while doing this `db.authors.find()`. will we be able to find something from blogs collection?
The answer is no.
But $lookup
operator solves that problem. we will be able to access the blogs collection data while working on db.authors.find()
lets use this data
authors collection data
db.authors.insertMany([{
name:"Aman",
email:"aman@gmail.com",
role:"SDE"
},{
name:"Albert",
email:"albert@gmail.com",
role:"Curriculum Director"
}])
blogs collection data
db.blogs.insertMany([{
title:"JS 101",
content:"Intro to JS",
author_email:"albert@gmail.com"
},{
title:"DSA 101",
content:"Intro to Data Structures",
author_email:"aman@gmail.com"
}])
benefits of $lookup operator is while I am reading the blog data, I will be able to get the author details
lets suppose I want to show the role of the author. I want to show that in the blog. I can get that using $lookup operator
Also When I am reading the authors data, at that time I want to acces its blogs. That also Is possible using $lookup operator
Q 1) Query to integrate the authors data with their blogs data
(want to know all the blogs of a particular collection)
Explaination of the below query
lookup operator , currently we are dealing with the authors collection
we want to get the blogs collection data.
from -> means from which collection we need data. [here its blogs]
now what is the field in authors collection the localField which is used in the blogs collection to have a relationships [here local means authors collection and foreign means blogs collection]
so in authors collection `email ` will be the key that is the one that is being used
foreignField -> means what is this email
field in the blogs collection so there it is present by author_email
as -> what should the keyword be for that here it is `myblogs`
nxm201repeat>
db.authors.aggregate([{$lookup : { from : "blogs" ,
localField : "email", foreignField : "author_email",as : "myblogs" }}])
[
{
_id: ObjectId('662df04e7cea397157c934e0'),
name: 'Aman',
email: 'aman@gmail.com',
role: 'SDE',
myblogs: [
{
_id: ObjectId('662df1127cea397157c934e3'),
title: 'DSA 101',
content: 'Intro to Data Structures',
author_email: 'aman@gmail.com'
}
]
},
{
_id: ObjectId('662df04e7cea397157c934e1'),
name: 'Albert',
email: 'albert@gmail.com',
role: 'Curriculum Director',
myblogs: [
{
_id: ObjectId('662df1127cea397157c934e2'),
title: 'JS 101',
content: 'Intro to JS',
author_email: 'albert@gmail.com'
}
]
}
]
solve the assignment of multiple lookup
there you have to perform lookup from 4-5 collections.
How u will be doing that?
You will use the output of one stage to query into second stage and so on.
$out Aggregation Operator [ write the documents result into a new collection]
out means output.
whatever we queried lets suppose we want to store it in separate collections.
In that case this $out will come into picture
we should put the out at the end of the operation
syntax [please verify with mongo db docs]$out : "authors_and_blogs"
What will happen when we will give the collection name here $out : "authors_and_blogs"
that is already existing? -> it will overwrite that collection in same db data even the schema in that collection is different it will overwrite the data
also lets suppose with the $out operator I want to store the data in different database collection. that is also possible
syntax
$out : {db:"different_database_name", coll:"authorandblogs"}
Project Operator
//original data
nxm201repeat> db.authors.aggregate([{$lookup : { from : "blogs" , localField : "email", foreignField : "author_email", as : "myblogs" }}])
[
{
_id: ObjectId('662df04e7cea397157c934e0'),
name: 'Aman',
email: 'aman@gmail.com',
role: 'SDE',
myblogs: [
{
_id: ObjectId('662df1127cea397157c934e3'),
title: 'DSA 101',
content: 'Intro to Data Structures',
author_email: 'aman@gmail.com'
}
]
},
{
_id: ObjectId('662df04e7cea397157c934e1'),
name: 'Albert',
email: 'albert@gmail.com',
role: 'Curriculum Director',
myblogs: [
{
_id: ObjectId('662df1127cea397157c934e2'),
title: 'JS 101',
content: 'Intro to JS',
author_email: 'albert@gmail.com'
}
]
}
]
example
// query
db.authors.aggregate([
{
$lookup: {
from: "blogs",
localField: "email",
foreignField: "author_email",
as: "myblogs"
}
},
{
$project: {
name: 1,
myblogs: 1,
_id: 0
}
}
])
// output
[
{
name: 'Aman',
myblogs: [
{
_id: ObjectId('662df1127cea397157c934e3'),
title: 'DSA 101',
content: 'Intro to Data Structures',
author_email: 'aman@gmail.com'
}
]
},
{
name: 'Albert',
myblogs: [
{
_id: ObjectId('662df1127cea397157c934e2'),
title: 'JS 101',
content: 'Intro to JS',
author_email: 'albert@gmail.com'
}
]
}
]
$count
example
nxm201repeat> db.authors.aggregate([ { $lookup: { from: "blogs", localField: "email", foreignField: "author_email", as: "myblogs" } }, { $count:"myblogs" }] )
[ { myblogs: 2 } ]
$set mongodb aggregation
set is basically add some field at some particular point
aggregation is mostly used when you want to read something. even though you are reading you want to update one key value or want to replace some key value at that time you can use set.
db.heros.agregate([{$match : {health : {$lt : 40}}},
{$set : {is_young : true}}])
Subscribe to my newsletter
Read articles from Surya Prakash Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
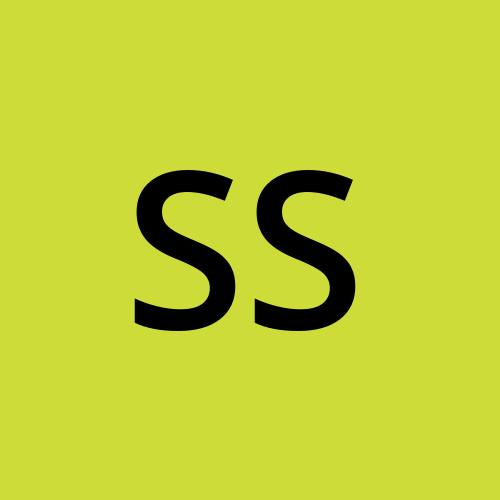
Surya Prakash Singh
Surya Prakash Singh
A full stack developer from India. Html | Css | Javascript | React | Redux | Tailwind | Node | Express | Mongodb | Sql | Nosql | Socket.io