C++ OOP Mastery: Part 1 Overview
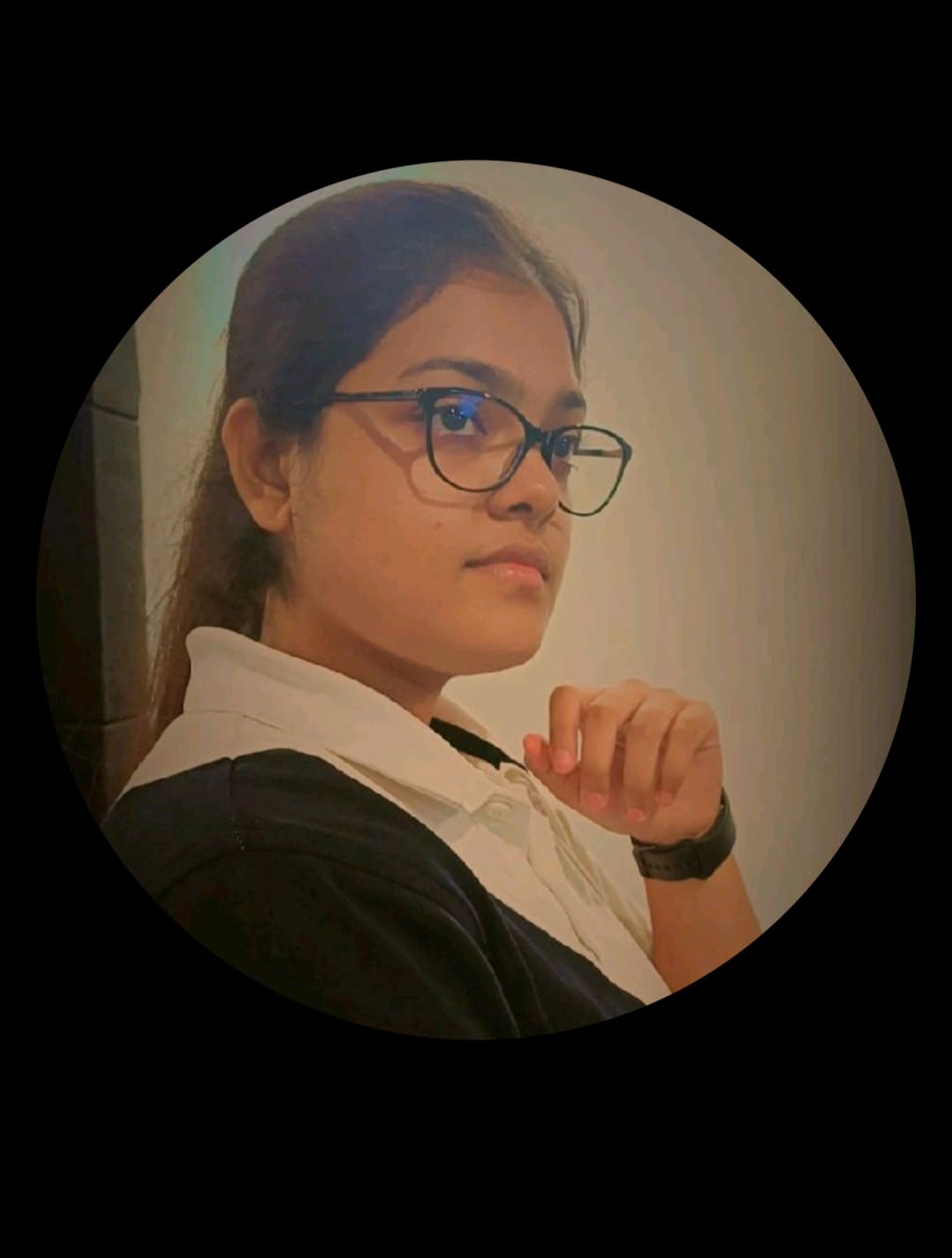
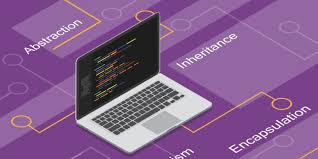
OOPS
OOPS, which stands for "Object Oriented Programming System," is a programming technique centered around objects. An object is an entity that encompasses both state/properties and behavior. This approach operates on the principles of classes and objects..
POPS
It stands for "Procedure Oriented Programming System". It consists of writing a set of instructions for the computer to follow. Its main focus is on functions, where functions can utilize either local or global data.
Characteristics OF OOPS
Object
Class
Inheritance
Polymorphism
Abstraction
Encapsulation
OBJECT (blueprint of class)
It is an entity that has state(data member) and behaviour(member function). It is an instance of class.
In real life entity we can take an example like pen, chair, table.
All the members of class can be accessed by object.
SYNTAX: class_name object_name;
EXAMPLE:
int main(){
age obj;
cout<<"enter age:";
cin>>obj.age;
obj.display();
return 0;
}
CLASS
It is a template from which object are created.
It consist of data members and data function which can be accessed by creating instance of class.
SYNTAX:
class class_name{
//some data
//some function
};
EXAMPLE:
class age{
public:
int age;
void display(){
cout<<"age="<<age;
}
};
CLASS & OBJECT EXAMPLE THROUGH PICTURE:
PROGRAM INCLUDING CLASS, OBJECT
#include <iostream>
using namespace std;
class age {
public:
int age;//data member
void display(){
cout<<"age="<<age;
}
};
int main() {
age obj; //creating an object of age
cout<<"enter age: ";
cin>>obj.age;
obj.display();
return 0;
}
ACCESS MODIFIERS/ACCESS SPECIFIERS
Access Modifiers or Access Specifiers in a class are used to assign the accessibility to the class members, i.e., they set some restrictions on the class members so that they can’t be directly accessed by the outside functions.
1. Public
2. Private
3. Protected
PUBLIC ACCESS SPECIFIER:
It means data member can be accessible inside or outside the class.
#include<iostream>
using namespace std;
class hero{
public:
int health;
char level;
};
int main(){
hero obj;
cout<<"health is: "<<obj.health<<endl;
cout<<"level is: "<<obj.level<<endl;
return 0;
}
PRIVATE ACCESS SPECIFIER:
It means data member can be accessible inside the class.
#include<iostream>
using namespace std;
class hero{
public:
int health;
private:
char level;
void print(){
cout<<level<<endl;
};
int main(){
hero obj;
cout<<"health is: "<<obj.health<<endl;
cout<<"level is: "<<obj.level<<endl;
return 0;
}
PROTECTED ACCESS SPECIFIER:
It is similar to the private access modifier in the sense that it can’t be accessed outside of its class unless with the help of a friend class.
#include <iostream>
using namespace std;
class Hero{
protected:
int id_protected;
};
class Student:public Hero{
public:
void setId(int id){
id_protected=id;
}
void displayId()
{
cout<<"id_protected:"<<id_protected<<endl;
}
};
int main(){
Student obj;
obj.setId(90);
obj.displayId();
return 0;
}
CONSTRUCTOR
It is invoked automatically at the time of object creation.
It has no return type, no input parameter.
It is a special member function with same name as of the class, used to initialize the objects of its class.
It is typically declared in class's public section.
Overloaded constructors are possible.
Declaring a constructor virtual is not permitted.
SYNTAX: <class name>(list_of_parameters);
DEFAULT CONSTRUCTOR
Daily life example: you ask a store to give you a marker, given that you didn't specify the brand name or colour of the marker you wanted, simply asking for one amount to a request. So, when we just said, "I just need a marker," he would hand us whatever the most popular marker was in the market or his store. The default constructor is exactly what it sounds like!
Definition: Constructor having no arguments is called default constructor.
SYNTAX:
<class-name>::<class-name>(list-of-parameters)
CODE:
#include<iostream>
using namespace std;
class complex{
int a;
int b;
public:
complex(void); //constructor called
void printnumber(){
cout<<"the number is"<<a<<"+"<<b<<"i"<<endl;
}
};
complex::complex(void){ //default constructor called
a=10;
b=20;
}
int main(){
complex c;
c.printnumber();
return 0;
}
PARAMETERIZED CONSTRUCTOR
Daily life example: Go into a store and specify that you want a red marker of the XYZ brand. He will give you that marker since you have brought up the subject. The parameters have been set in this instance thus. And a parameterized constructor is exactly what it sounds like!
Definition: A constructor which has two parameters is called parameterized constructor. It is used to provide different values to distinct object.
CODE:
#include<iostream>
using namespace std;
class complex{
int a;
int b;
public:
complex(int,int);
void printnumber(){
cout<<"the number is "<<a<<"+"<<b<<"i"<<endl;
}
};
complex::complex(int x,int y){ //parameterized constructor called
a=x;
b=y;
}
int main(){
//implicit call
complex a(4,8);
a.printnumber();
//explicit call
complex b=complex(5,6);
b.printnumber();
return 0;
}
COPY CONSTRUCTOR
Real life example: Visit a store and declare that you want a marker that looks like this (a physical marker on your hand). The shopkeeper will thus notice that marker. He will provide you with a new marker when you say all right. Therefore, make a copy of that marker. And that is what a copy constructor does!
Definition: Constructor which makes copy of another object.
SYNTAX:
number(number & obj){
a=obj.a;
}
CODE:
#include<iostream>
using namespace std;
class number{
int a;
public:
number(){}
number(int num){
a=num;
}
number(number & obj){
cout<<"copy constructor called"<<endl;
a=obj.a;
}
void display(){
cout<<"the number for this object is"<<a<<endl;
}
};
int main(){
number x,y,z(45);
x.display();
y.display();
z.display();
number z1(x);
z1.display();
return 0;
}
DESTRUCTOR
It simple free the memory.
It is used for memory de-allocate(when object becomes out of scope).
It has no parameter.
SYNTAX:
~<class_name>(){
}
CODE:
#include<iostream>
using namespace std;
int count=0; //global variable
class num{
public:
num(){
count++;
cout<<"this is the time when constructor is called for object number"<<count<<endl;
}
~num(){
cout<<"this is the time when destructor is called for object number"<<count<<endl;
count--;
}
};
int main(){
cout<<"we are inside the main function"<<endl;
cout<<"creating first object n1"<<endl;
num n1;
{
cout<<"creating two objects more"<<endl;
num n2,n3;
cout<<"entering this block"<<endl;
cout<<"exiting"<<endl;
}
cout<<"back to main function"<<endl;
return 0;
}
Subscribe to my newsletter
Read articles from Kali Baranwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
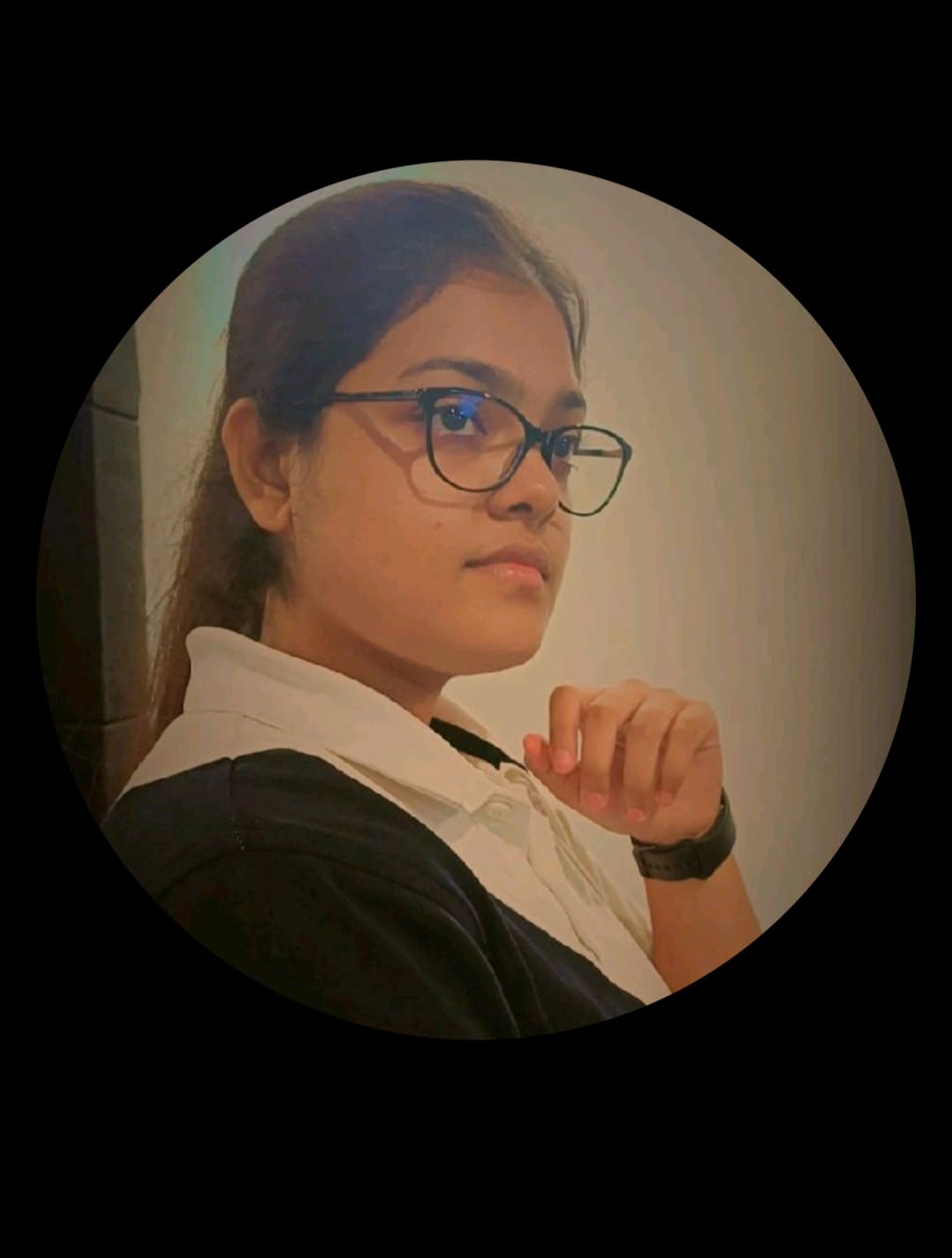
Kali Baranwal
Kali Baranwal
"👋 Hello, fellow developers! I'm Kali Baranwal , a 2nd year student . I've , exploring in another blog post also. Skilled in frontend developer, UI/UX design , C++ Join me as I share insights, of notes here on Hashnode .📚 Beyond technical skills, I'm also passionate about continuous learning and personal development. Let's connect, learn, and grow together!"