Solidity basics for beginners — Week 6 of Learning.
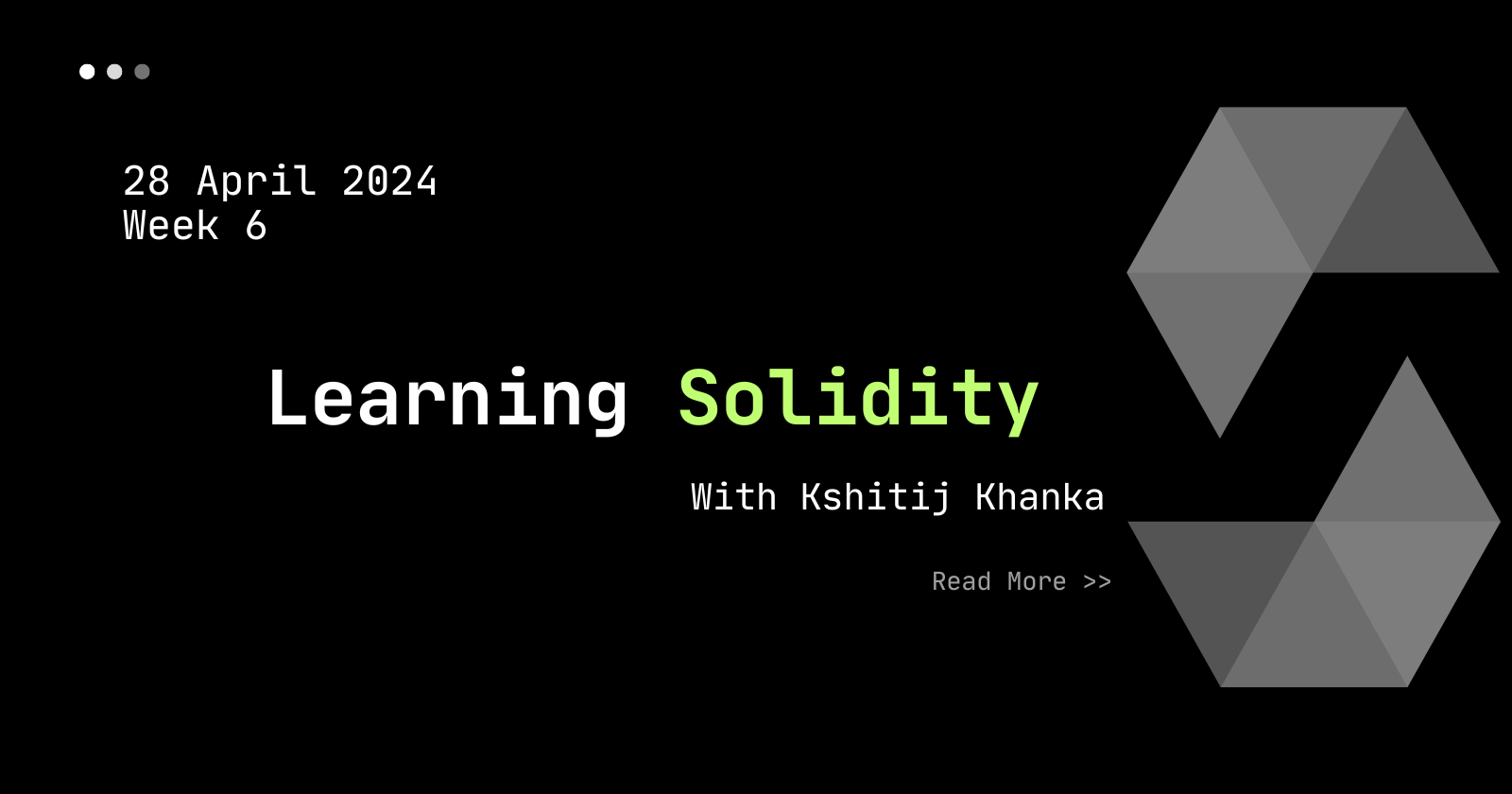
In this blog, we will be learning about the basics of Smart Contracts and Solidity, ranging from knowing datatype, functions, loops, etc to deploying our first ever Solidity smart contract.
ps. I have planned to cover all the tokens and coins in detail but exclusive on my LinkedIn, I would love to connect with fellow like-minded peers. ( My LinkedIn Profile )
Although I have covered about Smart contracts ( here ), but on a high level, it is basically a code deployed on blockchain. Now coming towards Solidity, What is Solidity ? It is a High level Object Oriented programming language specially used to write Smart contracts and Dapps on chains who are Ethereum Virtual Machine compatible. It takes inspiration from languages such as C++, Javascript and Python.
The way Solidity’s program is compiled and executed is :
You write a program / contract, you set the Gas Limit i.e. the program will only execute if the expenditure of Gas is within the limit. The extension of a Solidity Smart contract is .sol . SolC is the solidity compiler.
You compile the code and it decomposes into : Application Binary Interface ( ABI ) and Bytecode. The Bytecode and EVM makes the smart contract platform independent ( just like Java ). The bytecode compiled by a Windows machine will be the same if compiled from any other OS machine. The EVM ensures to run this bytecode specifically to the platform ( OS ). The ABI sets up a way for you to communicate with your smart contract after deployment. It allows you to access all the public functions and variables. It is in the form of JSON.
The contract is then deployed on the blockchain which after this step cannot be changed. The Bytecode part of the contract is deployed and ABI is used to setup a communication between applications so that we can interact with our smart contract. After the contract is deployed you get the contract address which later can be used to identify the contract.
We will be using Remix IDE which is one of the most used environment to write Solidity Smart contracts. ( Link to Remix )
Understanding the components of Remix IDE :
The Workspaces tab consists of all your files related to your project. It is the same as the project folder.
Just below the Workspaces tab we have a Search tab which can be used to locate any file from the project folder.
3. Compiler tab is where you can compile your smart contract and at the bottom you can see the option you view the ABI and the Bytecode file.
4. Deploy tab is used when you want to deploy your smart contract on the chain. It allows you to select an account from which you will transact / deploy the contract ( it gives you dummy accounts with fake ether, as you are just testing your contract ), set Gas Limit and Gas Price per unit, and it also allows you to interact with the contract after it is deployed.
5. Debug tab allows you to debug your code just like other compilers.
Now that we have learned our way around Remix IDE, let's get our hands dirty with some code.
Line 1 : It defines the License we will be using for our contract. Don’t worry about it for now.
Line 2 : Here we define which compiler we will be using, you can select a specific compiler using ^^. So here, I have selected a compiler version greater than 0.8.4.
Line 4 : We start writing our contract.
Line 6 : We have defined a variable tokens and it is a special variable as it is referred to as State variable. Why is it special ? The scope of this variable is the whole contract thus it will be stored on the blockchain permanently. It can be accessed by any function if intended, so modification to it costs Gas ( because you will be writing it on blockchain ).
Line 7 : I have defined a function which modifies the State variable therefore I cannot use view or pure. The function is made public, i.e. it can be accessed after deployment ( remember ABI ? It allows you to access your smart contract after deployment ). If any variable is defined inside the function scope, it is referred to as a local variable and is not stored on blockchain. To easily differentiate State and Local variables apart, we use underscore ( _ ) before naming a Local variable.
Line 10 : I have defined a function which only reads the state variable thus I have to declare the function as view. If there exists a function which neither reads nor modifies the State variable it is declared by using pure. Also, It returns the number of tokens thus I have to mention returns and the datatype it returns. If we were to define any private function, we would use underscore ( _ ) before its name to easily differentiate.
This was a simple program, let's understand data types, functions and loops in detail now so that you can write your own Smart Contracts.
State vs Local variables : State variables define the state of contract, have scope of complete contract, and are stored on blockchain permanently whereas a Local variable has scope of the function which begins with underscore ( _ ) and is not stored on blockchain permanently.
Normal vs View vs Pure Functions : We use keyword pure while defining if we are neither reading nor modifying any State variable throughout the function, whereas if we are just viewing / accessing the State variable we use view ( even if it is once throughout the function ) and if we are modifying the State variable we do not use any keyword ( even if it is once throughout the function ).
Public vs Private Functions / Variables : It is the same principle used in other programming languages. Public functions / variables can be called / accessed by users whereas Private cannot be accessed by users directly. If you declare a State Variable Public solidity automatically creates a getter function for it, which returns the current value of that State variable. Public and Private are also called modifiers. We have many more modifiers which we will discuss later.
Data types in Solidity : To allocate memory to a variable and knowing what type of data it stores, we require Datatype.
Integer : To store numbers we require an integer datatype, it is further divided into Signed and Unsigned i.e. you can store negative numbers in a signed integer. We can also tell the compiler the memory we require by stating the number of bits required, example — uint32 will be given 32 bits i.e. 4 bytes of memory. If you declare only unit, the compiler by default allocates 256 bits i.e. 32 bytes of memory.
Boolean : To store boolean values i.e. True or False.
Bytes : It stores value in the form of Hexadecimal which requires less space and is more efficient. It can be used as replacement of string ( when the size is fixed ), but the size of Bytes is limited to 32 bytes.
Address : The address type is a 160-bit value that does not allow any arithmetic operations. It is used to store addresses.
Loops in Solidity is same as in Javascript, which you can lookup here ( Link ).
In the next blog, we will code and go through some snippets to understand more about it. Stay tuned!
Subscribe to my newsletter
Read articles from Kshitij Khanka directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by