Mastering Higher-Order Functions: A Comprehensive Guide for Developers(lt.38)

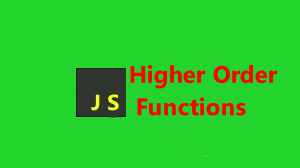
Introduction:
A higher-order function is a function that deals with other functions in a special way. They refer to functions that can take other functions as arguments or return functions as their results . Also called first class functions.
Key points about higher-order functions in JavaScript:
Accepting Functions as Arguments: They can take other functions as parameters. This feature allows for dynamic behavior based on the functions passed as arguments.
Returning Functions: They return functions as their output
Functional Composition: Here smaller functions are combined to create more complex functionality. This promotes code reusability and modularity.
Callback Functions: These functions are executed after certain events or operations are completed.
Coding implementation of Hof
const powertwo =(n)=>
{
return n**2
}
const powercube =(powertwo,n)=>
{
return powertwo(n)*n
}
console.log(powercube(powertwo,3))
// // // TYPE 2
function hello()
{
// return "gopal";
return ()=>
{
console.log("hello ji")
}
}
let guess = hello()
console.log(guess)
// // The code is printing [Function (anonymous)]
//instead of "hello ji" because of how JavaScript handles function definitions
// and references.
// // When you define a function in JavaScript, such as the arrow function
//returned by hello, what is stored in a variable like guess is not
// the result of executing that function immediately. Instead,
//it stores a reference to the function itself.
guess();
// so to print the actual matter inside the function we have to call the guess
// variable
// // // HIGHER ORDER complex ex
const higheroder=(x)=>
{
const fun1=(y)=>
{
const fun2=(z)=>
{
return x+y+z;
}
return fun2
}
return fun1
}
let ans = higheroder(1)(2)(3)
console.log(ans)
// usee of higher order
const my =[1,2,3,4]
const sum = (my1)=>
{
let total =0;
my1.forEach(function(elements) {
total+=elements
});
return total;
}
console.log(sum(my));
// TO SET INTERVAL AND TIMEOUT
// function print()
// {
// console.log("himanshu ", Math.random())
// }
// // setInterval(print,1000)
// setTimeout(print,10000)
Loops to handle array easily
Some of the loops we will discuss here are : For each, map, filter reduce every, find, sort
Below coding implementation will help us to learn these loops easily.
let arr = [1, 2, 3, 45];
arr.forEach(function (e, i, a) {
console.log(e, i, a);
// console.log(e,i)
});
// usimg arrow function
arr.forEach((elemet, indx, arr) => {
console.log("arrw : ", elemet, indx, arr);
});
const heros = ["naagraj", "doga", "dhruva", "maniraj"];
heros.forEach((a) => {
console.log(a.toUpperCase());
});
//USING MAP FUNCTION
arr.map(function (elemet, indx, arr) {
console.log(elemet, indx, arr);
});
//TO CHANGE TO UPEER CASE IN MAP
heros.map((a1) => {
console.log(a1.toUpperCase());
});
//FILTER
console.log("current values inside heroes : ", heros);
const heros1 = heros.filter((h) => {
return h.endsWith("raj");
});
console.log(heros1);
//SHOPPING BILL
const bill = [20, 40, 50];
const sumbill = bill.reduce((prev, curr) => prev + curr, 0);
console.log(sumbill);
//game socre
const gamescore = [290, 700, 210, 300];
const gamescore1 = [290, 700, 210, "gkd"];
console.log(typeof gamescore[1]);
const checkscore = gamescore.every((g) => typeof g === "number");
console.log("result: ", checkscore);
const checkscore1 = gamescore1.every((g) => typeof g === "number");
console.log("result: ", checkscore1);
//FIND SCORE ABOVE 200
const above200 =gamescore.find((s)=> s>200)
console.log(above200)
//finindex
//some
//sort
Subscribe to my newsletter
Read articles from himanshu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
