Tricky Questions About JavaScript's setTimeout
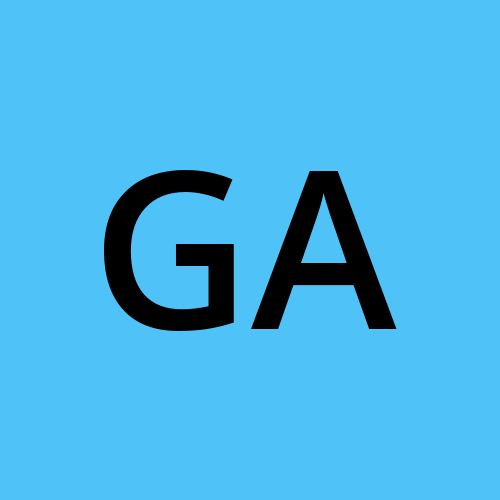
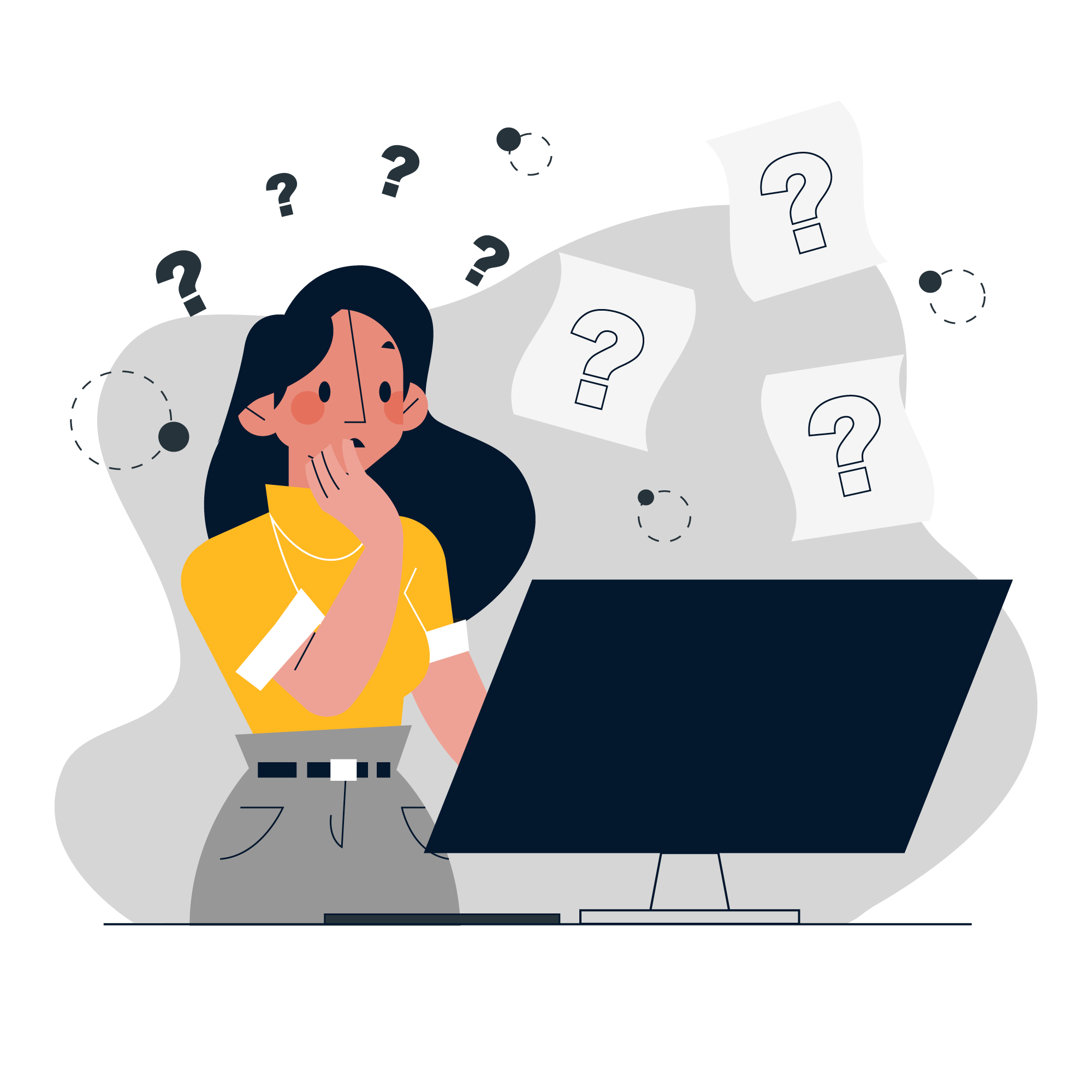
Introduction: While working with JavaScript, setTimeout
often presents some puzzling scenarios due to its asynchronous nature and the way it interacts with JavaScript’s scoping rules. This post explores some of these tricky cases to enhance your understanding of setTimeout
and closures.
Prerequisite Reading: Before diving into these questions, ensure you're familiar with the basics of setTimeout
and closures:
Q1. Initial Challenge: Consider the following code snippet:
for (var i = 0; i < 5; i++) {
setTimeout(() => console.log(i), 1000);
}
Output:
5 5 5 5 5
Explanation: This output occurs because the variable i
is declared with var
, which is function-scoped. The setTimeout
the function is asynchronous, meaning it schedules the callback functions to run after the loop has been completed.
Detailed Breakdown:
Synchronous vs. Asynchronous Execution: JavaScript first executes all synchronous code. Here, the loop is synchronous and completes before any of the
setTimeout
callbacks are executed.Function Scoping with
var
: Sincevar
is not block-scoped but function-scoped, all iterations of the loop share the samei
. This meansi
is incremented to 5 by the time the loop ends, before any of thesetTimeout
callbacks have a chance to execute.Execution of
setTimeout
Callbacks: Each callback is placed in the JavaScript callback queue almost immediately as the loop runs. However, they are not executed until the synchronous code has finished running, which is why they all print5
— the final value ofi
when the loop completes.Closure and Variable Reference: Each callback captures a closure over the same
i
. A closure allows the function to remember and accessi
from its scope even after the scope has been exited. In this case, since there is only onei
and it is shared across all callbacks, they all print the final value ofi
.
This demonstrates a fundamental aspect of how closures work with function-scoped variables in JavaScript, and why understanding the difference between var
and let
can be crucial for managing asynchronous code correctly.
Q2. Revised Question: Now, let's modify the initial code by changing the declaration of i
from var
to let
and see the outcome:
for (let i = 0; i < 5; i++) {
setTimeout(() => console.log(i), 1000);
}
Output:
0 1 2 3 4
Why is the output different now? This time, the code prints each number from 0
to 4
sequentially. The key difference here lies in the scope of the variable i
.
In-depth Analysis:
Block Scoping with
let
: Unlikevar
, which is function-scoped,let
provides block scoping. This means that a newi
is created for each iteration of the loop. Each of these instances ofi
is confined within the block of the loop iteration.Loop and Scope Mechanics: For each pass through the loop, the
let
statement creates a fresh binding (or instance) ofi
. Thus, eachsetTimeout
callback is closed over its respective loop iteration's scope, which contains its uniquei
.Closure in Action: Since each iteration of the loop has its own
i
, the closures formed by thesetTimeout
callbacks capture differenti
values (from0
to4
). This is fundamentally different from usingvar
, where all iterations share the samei
.Execution Timing: The
setTimeout
callbacks are still placed in the queue during the loop execution but are called only after the loop completes. Thanks to block scoping, each callback references the correcti
from its respective iteration, hence printing0
,1
,2
,3
, and4
after the one-second delay.
This showcases the importance of understanding variable scoping in JavaScript, especially when dealing with loops and asynchronous callbacks. The use of let
in a loop with asynchronous code is a crucial pattern for avoiding common pitfalls with closures and shared variable access.
Q3. Using an IIFE with var
:
for (var i = 0; i < 5; i++) {
(function(i) {
setTimeout(() => console.log(i), 1000);
})(i);
}
Output: 0 1 2 3 4
Why This Works:
The IIFE creates a new scope for each iteration, capturing the current value of
i
in each loop.This mimics how
let
works but with an explicit function to create scope.
Q4. Passing Arguments to setTimeout
:
for (var i = 0; i < 5; i++) {
setTimeout((i) => {
console.log(i);
}, 1000, i);
}
Output: 0 1 2 3 4
Key Points:
Modern JavaScript environments allow passing additional arguments to
setTimeout
, which are used in the callback.This method avoids closure-related issues by directly providing the loop index as an argument to the callback.
Q5. Clearing Timers in React:
useEffect(() => {
const timerId = setTimeout(() => {
console.log("Timer fired");
}, 1000);
return () => {
clearTimeout(timerId);
console.log("Timer cleared");
};
}, []);
React Lifecycle Consideration:
- In React, the cleanup code for
setTimeout
, such asclearTimeout
, is typically placed in theuseEffect
cleanup function, which acts as thecomponentWillUnmount
lifecycle method.
Conclusion: Understanding how setTimeout
interacts with JavaScript’s scoping rules is essential for writing bug-free asynchronous code. By exploring these examples, developers can better handle timing functions in their applications, avoiding common pitfalls related to closures and scoping.
Thank you for reading! 🧑💻 Keep experimenting, keep learning, and always test your assumptions through code!
Subscribe to my newsletter
Read articles from gunjan agarwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
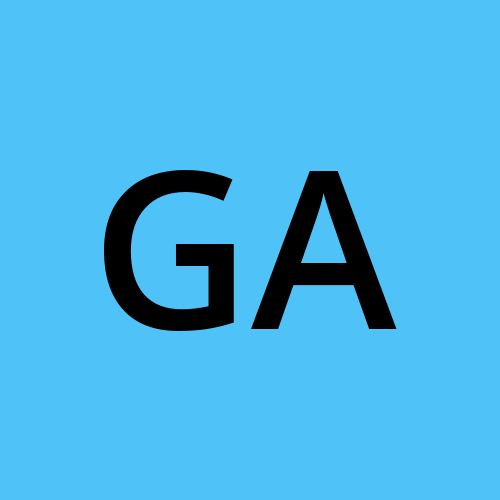