'Access-Control-Allow-Origin'
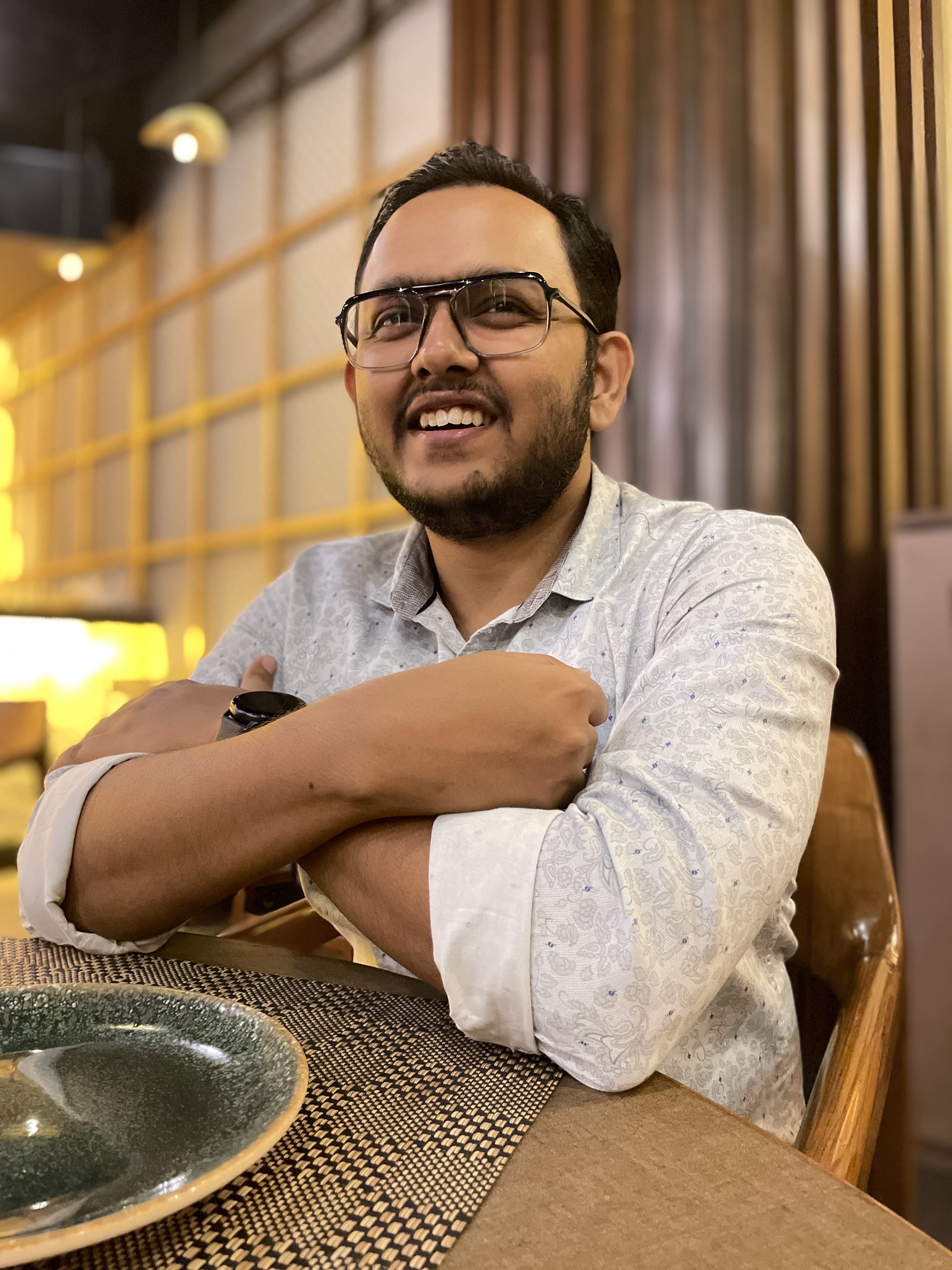
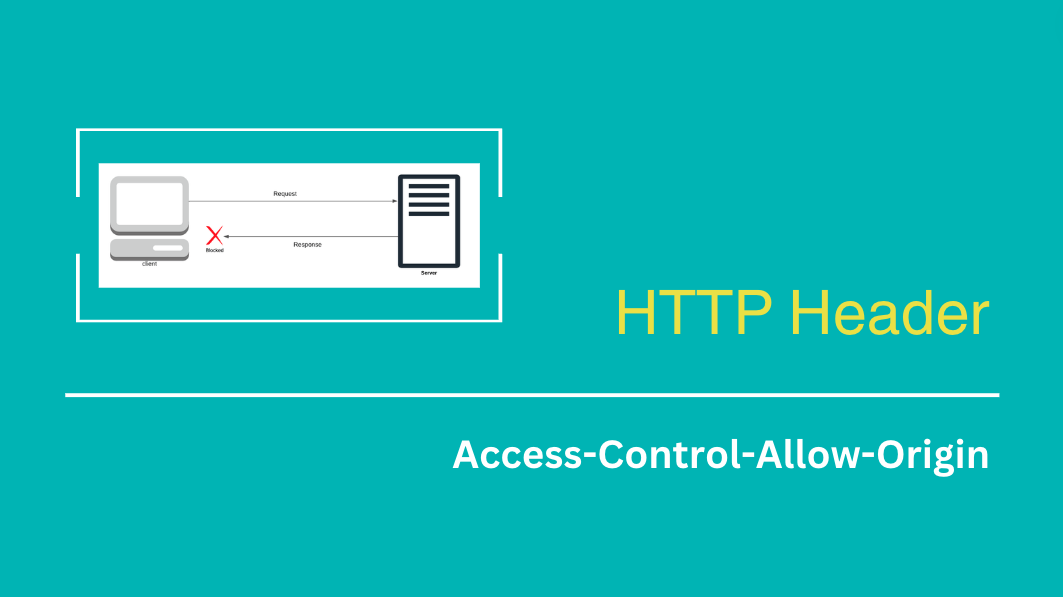
As per MDN "The Access-Control-Allow-Origin
response header indicates whether the response can be shared with requesting code from the given origin."
Basically, it is an HTTP header used to manage Cross-Origin Resource Sharing (CORS), which is a security feature that restricts how web resources are accessed across different origins (domains, ports, or protocols). CORS is designed to enhance security by preventing unauthorized access to resources from different origins.
Why is it important?
When a browser makes a cross-origin request, such as fetching data from a different domain, the server must explicitly allow that request for security reasons. The Access-Control-Allow-Origin
header indicates which origins are permitted to access a resource.
Use cases / Scenarios where it is important to use
If you're building a RESTful API, you might need to configure CORS to allow client-side applications from specific origins to interact with your API.
When integrating with third-party services or APIs, the service might need to configure CORS to permit your domain to access its resources.
How it works?
Single Origin:
You can specify a single origin that's allowed to access the resource. For example, if you want to allow requests from
https://test.com
, you would set the header to:Access-Control-Allow-Origin: https://test.com
Wildcard:
A wildcard (
*
) allows any origin to access the resource. This can be a security risk and is generally discouraged for production environments unless there's a specific reason.Access-Control-Allow-Origin: *
Multiple Origin:
To allow multiple specific origins, you need additional logic on the server side to determine if the requesting origin is allowed and dynamically set the header accordingly.
Let's look at an example with Express.js
where we are using cors middleware to manage CORS policies.
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors({
origin: "https://test.com", // for allowing a specific origin
}));
app.get("/data", (req, res) => {
res.json({ message: "Hello!" });
});
app.listen(3000, () => {
console.log("Running server on port 3000");
});
Also, if we want to set restrictions on the different method types, we can do so using Access-Control-Allow-Headers
.
For example, if we want to restrict test.com to GET and POST only as per the last example, we need to add another attribute methods
app.use(cors({
origin: "https://test.com",
methods: ["GET","POST"],
}));
Conclusion:
So with Access-Control-Allow-Origin
we can manage the CORS (Cross Origin Resource Sharing) policies by allowing cross-origin requests under specific conditions to ensure security for the application.
Subscribe to my newsletter
Read articles from Sudipta Saha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
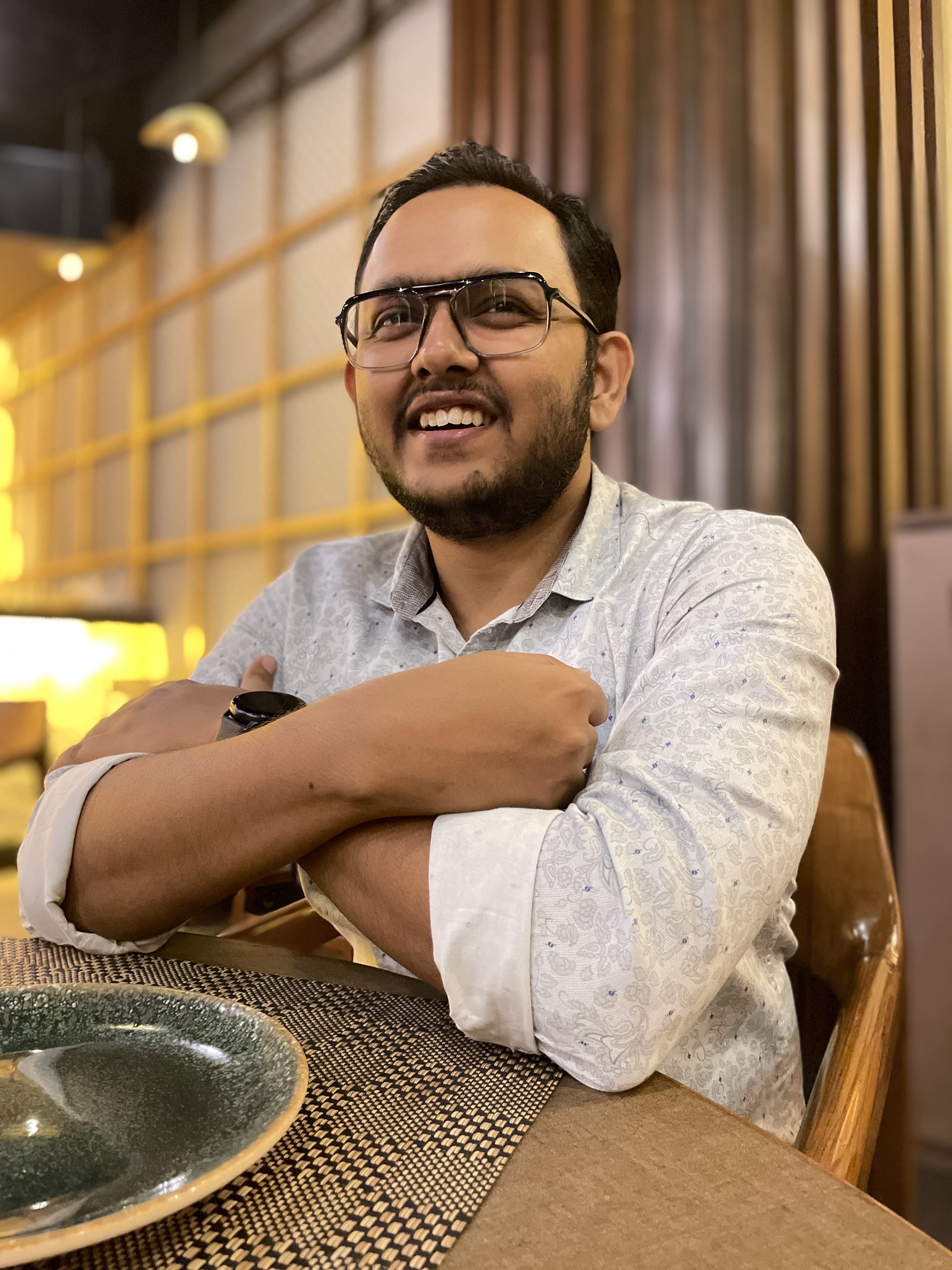