(2024) REST Calls using Web Client in Spring 5 and Springboot 3
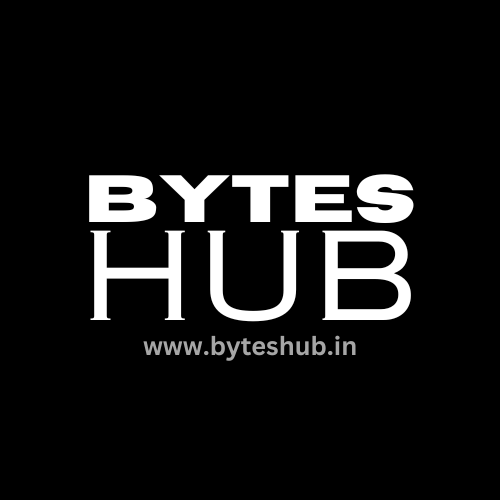
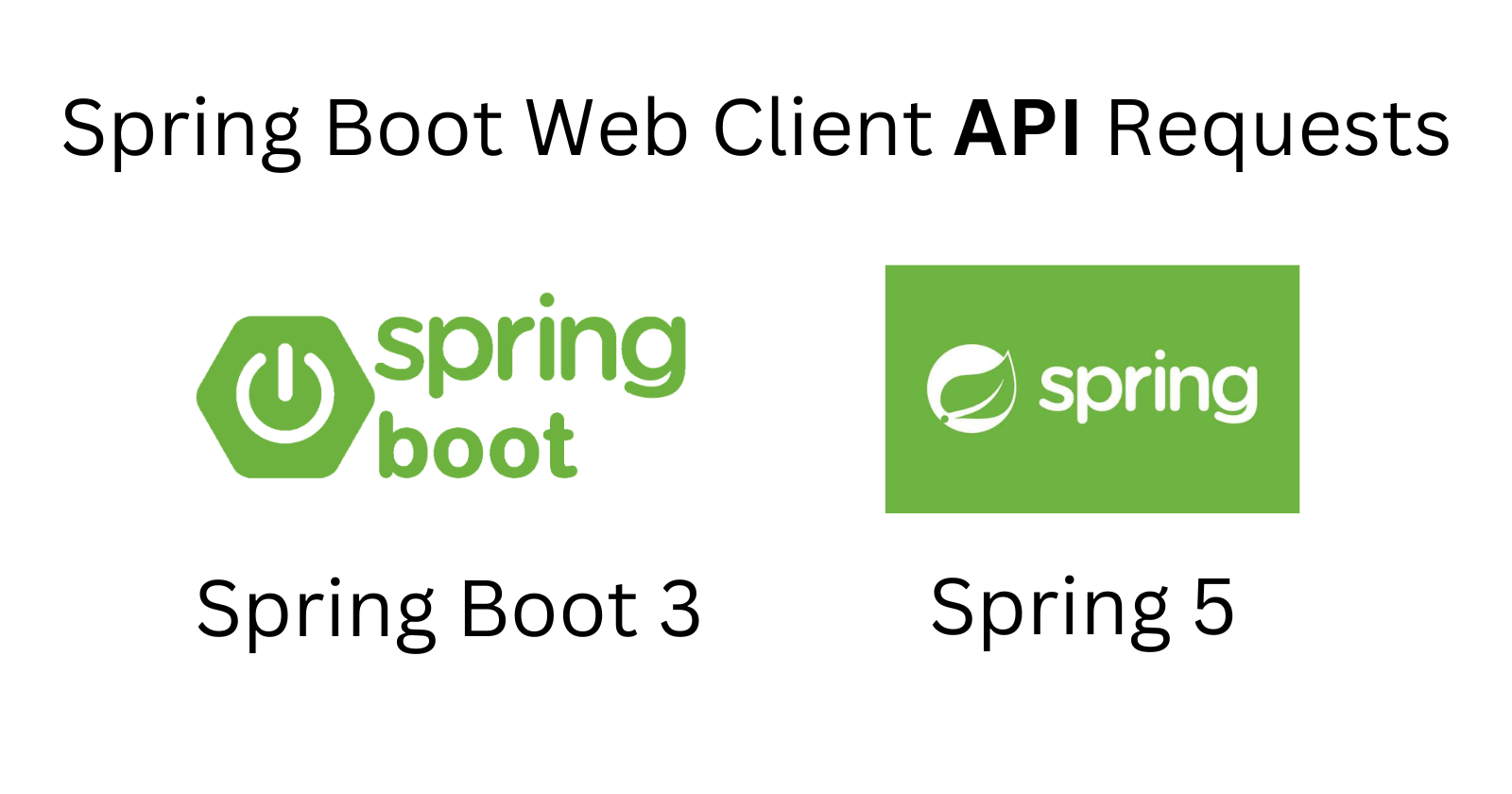
In last article, we discussed about how you can configure Spring WebClient in your springboot project. You can check out that article by clicking here. This article is continuation of the previous article in which we will be discussing about how you can REST calls using WebClient.
As discussed in previous article, we'll be using Gradle project management tool in this article as well. You can use Maven as well; both will work same.
Synchronous & Asynchronous requests
Synchronous API calls or requests are those in which the code execution blocks the code flow (normally called as blocking state) and will wait for the API to return the data to proceed further. This method of API calls uses more resources and time to perform the operation.
For example, let's say you have a signup screen in your application where user enters all his personal information required to create his account. As soon as, the user hits the submit button then a backend API request will fire which sends the data from frontend to backend and your signup page will show a loading screen. This phenomenon is synchronous API request as we have to wait until the backend code performs all its operation such as saving data in DB, issuing a authentication token etc to move forward to home screen.
Asynchronous API calls or requests are those in which the code execution doesn't block the code flow and executes in parallel. We don't have to wait for API to return the data and we can focus on executing other functionalities. The main operation will execute once we got the data back from API after processing in parallel.
For example, let's say we have a contact us page on application where user will submit the form details in case of any query or feedback. As soon as user submits the form, a backend API request will be fired but this time there will be no loading screen or blocking screen and we can simply navigate to other screens and resume our work. Meanwhile, the API request will store all the data in DB and will trigger a mail to user to notify about success of query submission.
GET and DELETE API Request
Use the code below to make a GET/DELETE request using spring webclient.
URI uri = URI.create("https://jsonplaceholder.typicode.com/posts");
String response = webClient
.get() // .delete() for DELETE requests
.uri(uri)
.accept(MediaType.APPLICATION_JSON)
.attribute("attribute_name","attribute_value")
.cookie("cookie_name","cookie_value")
.header("header_name","header_value")
.retrieve()
.bodyToMono(String.class)
.block();
Use
URI.create()
(Mandatory) method to create a URI (Uniform Resource Identifier) which needs to be pass in.uri()
method ofwebclient.get()
Use
.accept()
(Optional) to provide the acceptance type of request data..attribute("attribute_name","attribute_value")
(Optional) can be used to provide the query parameters (if needed) for given URI..cookie("cookie_name","cookie_value")
(Optional) can be used to provide the cookie which is required to make the API request..header("header_name","header_value")
(Optional) can be used to provide the header value (if needed) to make the API request..retrieve()
(Mandatory) needs to be used to make the request and fetch the returned data.We can use
.bodyToMono(classType)
(Optional) if you would like to get data directly to your specified class type and not as Mono data..block()
method is Optional but important. If you want to make synchronous request, then use this method otherwise don't use it.
POST and PATCH API Request
Use the code below to make a POST/PATCH request. The code is similar to what we used in GET request. The only difference is that we need to use.body(bodyValue, classType)
method to pass request body to the request. Rest of the methods will be same, and we will not be discussing those again. Please refer to GET request section for details.
String response2 = webClient
.post() // .patch() for PATCH requests
.uri(uri)
.body("body_value", String.class)
.accept(MediaType.APPLICATION_JSON)
.attribute("attribute_name","attribute_value")
.cookie("cookie_name","cookie_value")
.header("header_name","header_value")
.retrieve()
.bodyToMono(String.class)
.block();
Conclusion
It was that simple to configure Spring web client and to perform CRUD operations. in our next article, we will be discussing on how you can make extra configuration in webclient to configure timeouts (readTimeout, requestTimeout etc).
If you face any issue or have any feel free to drop an email on official@byteshub.in to request article on any choice of yours. Connect with me on my LinkedIn Rohan Sanger for collaboration/feedback.
Don't forget to join our FREE telegram channel for latest updates on articles. Join here.
Subscribe to my newsletter
Read articles from BytesHub directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
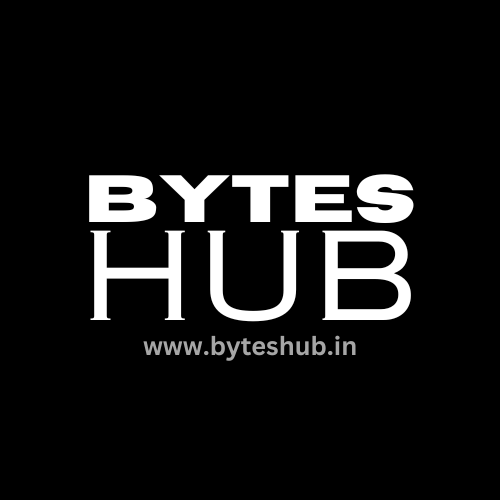
BytesHub
BytesHub
Byteshub provides you latest coding content and articles.