Day63: Terraform Variables
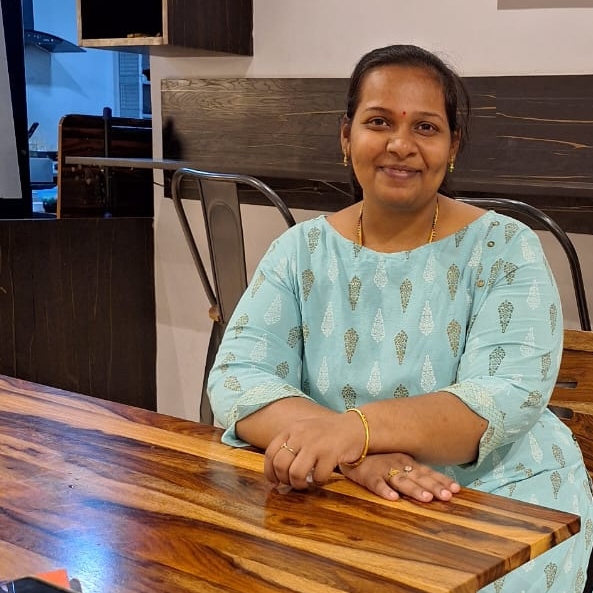
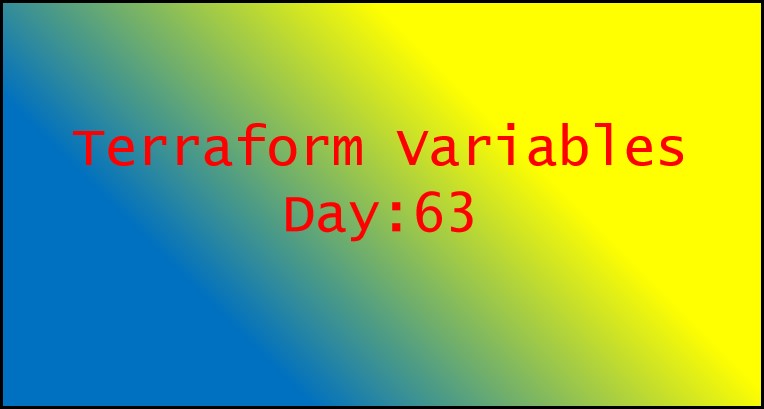
Variables in Terraform are quite important, as you need to hold values for names of instance, configurations, etc. We can create avariables.tffile that will hold all the variables.
What are terraform variables?
Terraform variables allow you to define values that can be reused throughout your Terraform setup. They increase the dynamic and flexibility of your configuration while also improving the parametrization of your code. Variables allow you to quickly adapt multiple setups without changing your code, as you can just adjust the values of these variables to achieve different use cases.
variable "filename" {
default = "/home/ubuntu/terraform/var-demo.txt"
}
variable "content" {
default = "This is terraform variable demo file"
}
These variables can be accessed by var object in main.tf
Using Variables: You can use the declared variables in your resource blocks, data blocks, or other parts of your configuration by referencing them with the var
prefix. For example:
resource "aws_instance" "example_instance" {
ami = var.example_var
instance_type = "t2.micro"
}
In this case, we’re using the example_var
variable as the ami
attribute for an AWS EC2 instance.
Variable Values: When you execute your Terraform configuration, you can assign values to the variables using a various ways, including command-line arguments, variable files, and environment variables.
Inline Value: You can set a variable value directly in your Terraform configuration file using an inline value assignment.
Example:
variable "region" {
type = string
default = "us-west-2"
}
Environment Variables: You can set variable values using environment variables. Terraform automatically reads environment variables with a
TF_VAR_
prefix and matches them to the corresponding variable names.Example:
export TF_VAR_region="us-west-2"
Command-Line Flags: You can pass variable values directly through command-line flags when running Terraform commands.
Example:
terraform apply -var="region=us-west-2"
Variable Files: You can define a separate file to store variable values and reference that file in your Terraform configuration.
Example:
variable "region" {
type = string
default = "us-east-1"
}
variable "instance_type" {
type = string
default = "t2.micro"
}
Create a terraform.tfvars
or variables.tfvars
file and set the variable values:
region = "us-west-2"
instance_type = "t3.small"
These are the common methods to set variable values in Terraform. You can choose the method that best suits your requirements and workflow.
Variable defaults: Variable defaults in Terraform allow you to provide a default value for a variable in case no other value is explicitly set. This ensures that the variable has a value even if it is not explicitly assigned. Here's how you can define variable defaults in Terraform
variable "region" {
type = string
default = "us-west-2"
}
In the example above, the region
variable has a default value of "us-west-2"
. If no value is explicitly assigned to the region
variable, Terraform will use the default value.
Variable defaults are useful when you want to provide a sensible default value for a variable but still allow users to override it if necessary. They help ensure that your configuration is valid even if certain variables are not explicitly provided.
Variable Types: Terraform supports various variable types, including string, number, boolean, list, map, and more. You specify the variable type in the type
attribute when declaring the variable.
Here is the example for some of the variable types:
variable "name" {
type = string
default = "example"
}
variable "port" {
type = number
default = 8080
}
variable "ip_addresses" {
type = list(string)
default = ["192.168.1.1", "192.168.1.2", "192.168.1.3"]
}
variable "metadata" {
type = map(string)
default = {
owner = "John Doe"
region = "us-west-2"
}
}
variable "config" {
type = object({
timeout = number
replicas = number
tags = map(string)
})
default = {
timeout = 60
replicas = 3
tags = {
Name = "example"
Environment = "production"
}
}
}
You can customize the input values for these variables when running terraform apply
by using a .tfvars
file, environment variables, or command-line arguments.
Using variable types in Terraform allows you to define flexible and reusable configurations that can be parameterized and adjusted based on your specific requirements.
Task1:
Create a local file using Terraform
Step 1: Create a variable.tf, and add details regarding file creation by providing the new file complete path.
sudo vim var.tf
# var.tf
variable "filename" {
default = "/home/ubuntu/terraform-variables/demo-var.txt"
}
variable "content" {
default = "This is coming from a variable which was updated"
}
Step 2: Create a main.tf file where we will access the variables that have defined before.
resource "local_file" "devops" {
filename = var.filename
content = var.content
}
Step 3: Initializes a new terraform working directory.
terraform init
Step 4:
terraform plan command generates an execution plan that shows Terraform's operations to reach the target state given in the configuration file.
terraform plan
Step 5:
Once terraform apply
command runs, Terraform will create a file called demo-var.txt in the local directory with the content specified in the content variable that we had mentioned before.
terraform apply
Step 6:
Check whether the file is created in a specified folder using ls
command.
terraform refresh:
The Terraform refresh command compares the infrastructure's current state to the state defined in the Terraform configuration files. It updates the Terraform state file with any changes performed outside of Terraform, ensuring that resource status and characteristics are tracked accurately.
Data Types in Terraform
In Terraform, data types are used to define the structure and format of values that are used within the configuration. Terraform supports various data types that can be used to define inputs, outputs, variables, and other configuration elements. Here are some commonly used data types in Terraform :
- String: A string is a sequence of characters. It is enclosed within double quotes ("") or single quotes ('').
variable "region" {
type = string
default = "us-west-2"
}
- Number: A number represents a numeric value, either integer or floating-point.
variable "instance_count" {
type = number
default = 2
}
- Boolean: A boolean represents either true or false.
variable "enable_logging" {
type = bool
default = true
}
- List: A list is an ordered collection of values of the same type.
variable "security_groups" {
type = list(string)
default = ["sg-12345678", "sg-87654321"]
}
- Map : A map is an unordered collection of key-value pairs.
variable "tags" {
type = map(string)
default = {
Name = "my-instance"
Environment = "production"
}
}
- Object : An object is a complex data type that combines multiple attributes into a single value.
variable "instance" {
type = object({
instance_type = string
ami_id = string
subnet_id = string
})
default = {
instance_type = "t2.micro"
ami_id = "ami-12345678"
subnet_id = "subnet-87654321"
}
}
These are some of the commonly used data types in Terraform. You can use them to define variables, input parameters, and outputs in your Terraform configurations.
Task2:
Use terraform to demonstrate usage of List, Map, Set and Object datatypes:
Step 1: Inside variable.tf, Create a variable type map
and put content inside it.
variable "filename" {
default = "/home/ubuntu/terraform/terraform-variables/terra-dt/demo-map1.txt"
}
variable "content" {
default = "This is coming from a variable which was updated"
}
variable "file_contents" {
type = map
default = {
"statement1" = "this is cool stmt1"
"statement2" = "this is cooler stmt2"
}
}
Step 2: Now from the main.tf access the variables that have been created before inside variable.tf file.
resource "local_file" "devops" {
filename = var.filename
content = var.file_contents["statement1"]
}
resource "local_file" "devops_var" {
filename = "/home/ubuntu/terraform/terraform-variables/terra-dt/demo-map2.txt"
content = var.file_contents["statement2"]
}
Step 3: Initializes a new terraform working directory.
terraform init
step4: Use terraform plan
command
step5: Execute the command terraform apply.
step6: Now check the file is created in specified folder using ls
command.
List:
In Terraform, a list
is a data structure that allows you to store and manage a collection of values. Lists
are often used to define input variables, output values, and resource configurations.
Step 1: Inside variables.tf, Create a variable of type list
to pass the list of files.
variable "file_list"{
type = list
default = ["/home/ubuntu/terraform/terraform-variables/terra-dt/file1.txt","/home/ubuntu/terraform/terraform-variables/terra-dt/file2.txt"]
}
Step 2: Now from the main.tf access the variables that have been created before inside variable.tf file.
resource "local_file" "devops" {
filename = var.file_list[0]
content = var.file_contents["statement1"]
}
resource "local_file" "devops_var" {
filename = var.file_list[1]
content = var.file_contents["statement2"]
}
Step 3: Run the command terraform init
Step 3: Run the command terraform plan
step4: Execute terraform apply
command
Step 5: Now check the contents of file1 and file2 using following commands
Object
Unlike a map and list, an object
is a structural type that is used to store structured data with named attributes and each has its type.
Objects
are commonly used to group relevant attributes and give an organized approach to organizing and managing data inside a setup. Terraform represents object data types as maps, which are key-value pairs.
Step 1: Inside variables.tf, Create a variable of type object
to pass the list of files.
variable "aws_ec2_object"{
type = object({
name = string
instances = number
keys = list(string)
ami = string
})
default = {
name = "test_ec2-instance"
instances = 4
keys = ["key1.pem", "key2.pem"]
ami = "ami-0c7217cdde317cfec"
}
}
Step 2: Now, create an output file to view the output of a specific value as per our wish. And add it to main.tffile.
resource "local_file" "devops" {
filename = var.file_list[0]
content = var.file_contents["statement1"]
}
resource "local_file" "devops_var" {
filename = var.file_list[1]
content = var.file_contents["statement2"]
}
output "aws-ec2-instance" {
value = var.aws_ec2_object.ami
}
step3: Run the commands terraform init
and terraform plan
step4: Execute the command terraform apply.
and we can see the output value of aws ec2-instance.
Set
In Terraform, a set is a data structure that let you to store and manage a group of distinct values. Sets are frequently used to establish input variables and manage resource structures that require a unique set of values.
Step 1: Inside variables.tf, Create a variable for the type set
to pass the string of security groups.
variable "security_groups"{
type = set(string)
default = ["sg-131284", "sg-123184"]
}
Step 2: Create a output file to check the security group, add it in main.tf file.
output "security_group"{
value = var.security_group
}
Step 3: Run the commands terraform init
, terraform plan
.
Step 4: Now execute command terraform apply
and you can see the output of security_group value.
By accomplishing above steps, you have successfully demonstrated the use of several data types in Terraform and updated the state to reflect changes to your configuration.
In conclusion, Terraform variables and data types offer significant flexibility for customizing and structuring your infrastructure installations. Using variables allows you to construct flexible and repeatable settings. Understanding the various data types in Terraform enables you to design and manage your infrastructure more efficiently.
Thank you for 📖reading my blog, 👍Like it and share it 🔄 with your friends .Hope you find it helpful🤞
Happy learning😊😊
Subscribe to my newsletter
Read articles from Siri Chandana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
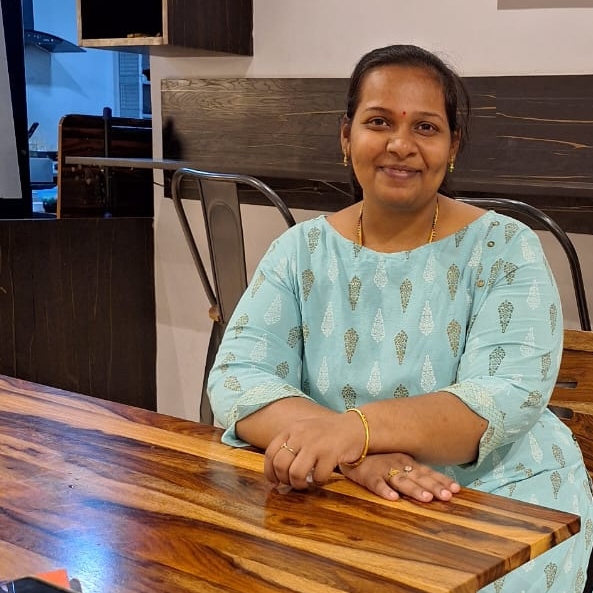