Quick Setup: Building Ionic Mobile Apps with Nuxt.js
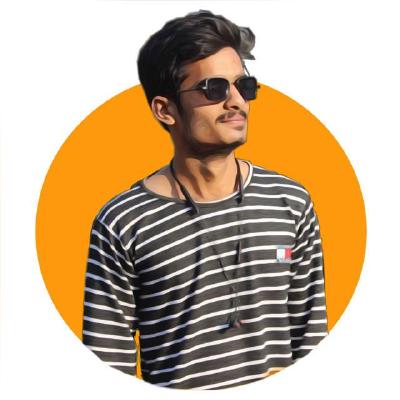
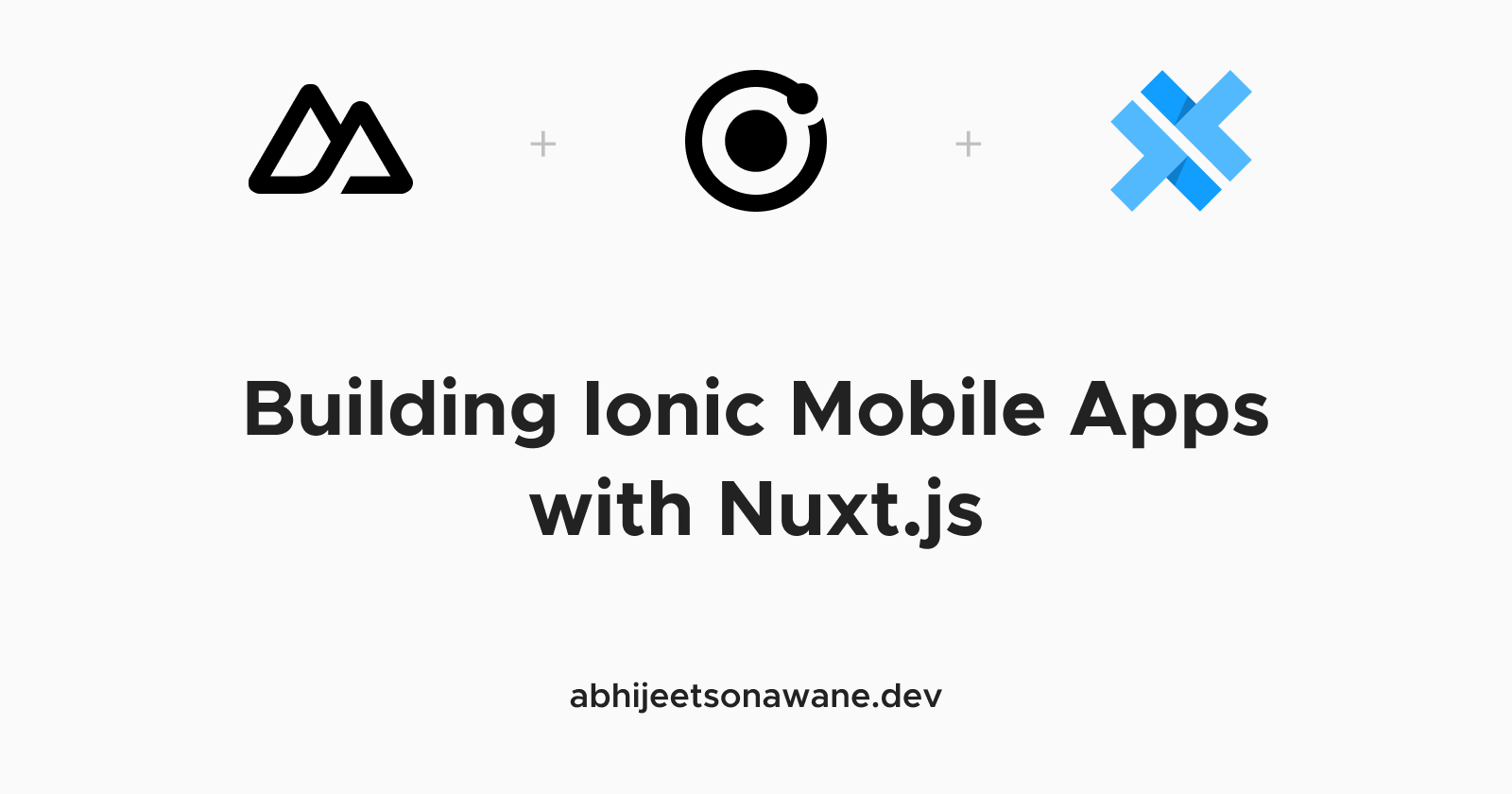
In this article, we will understand how to setup the Ionic Framework with Nuxt.js and build cross-platform apps using Capacitor.
Introduction
Nuxt.js is a free and open-source framework with an intuitive and extendable way to create type-safe, performant, and production-grade full-stack web applications and websites with Vue.js.
Ionic is an open source UI toolkit for building performant, high quality mobile apps using web technologies - HTML, CSS, JavaScript - with integrations for popular frameworks like Angular, React and Vue.
Capacitor is a cross-platform native runtime that makes it easy to build performant mobile applications that run natively on iOS, Android, and more using modern web tooling.
Create Nuxt.js Project
Prerequisites
- Node.js -
v18.0.0
or newer
Once you have Node.js installed, open a terminal and use the following command to create a new project:
npx nuxi@latest init <project-name>
Open your project folder in your favourite Code Editor.
If you are using VS Code, then use the following command:
code <project-name>
We have created a Nuxt.js project; now let's install the Ionic module.
Install Ionic
Now, let's add the @nuxtjs/ionic
module to our project's dev dependencies by using the following command:
npx nuxi@latest module add ionic
Once installed, next we have to add the module to our Nuxt configuration in nuxt.config.ts
.
export default defineNuxtConfig({
modules: ["@nuxtjs/ionic"]
})
If you are planning to deploy on iOS or Android, it's recommended to set ssr: false
in the nuxt config.
export default defineNuxtConfig({
modules: ['@nuxtjs/ionic'],
ssr: false,
})
Please checkout the Deploying to both Web and Device for more information.
Updating app.vue
We need to add the necessary <ion-app>
and <ion-router-outlet>
tags to our app.vue
to work with Ionic properly.
// app.vue
<template>
<ion-app>
<ion-router-outlet />
</ion-app>
</template>
<ion-app>
tag is the container element of Ionic, and there should be only one in the project. Please check out the Ionic ion-app for more information.
The <ion-router-outlet>
tag provides a mounting point for the route component as the Ionic router handles app routing.
Let's add a index.vue
page in our pages
directory
// ~/pages/index.vue
<template>
<ion-page>
<ion-header>
<ion-toolbar>
<ion-title>Home</ion-title>
</ion-toolbar>
</ion-header>
<ion-content class="ion-padding">Hello World</ion-content>
</ion-page>
</template>
<script setup>
</script>
Now let's run our app, use following command to run app
npm run dev
Open http://localhost:3000
in your browser, you will see it's showing our index.vue
page.
To understand more about how to use Ionic components with Nuxt.js or Vue.js, please checkout official Ionic Vue documentation.
Enable Capacitor
Prerequisites
- Please checkout official Capacitor Environment Setup
We don't need to install Capacitor in our app, as it is installed by default with @nuxtjs/ionic
. We just need to enable it in our Ionic app. Use the following command to enable capacitor in our app:
npx @ionic/cli integrations enable capacitor
Add support for platforms
To add build support for iOS:
npx @ionic/cli capacitor add ios
To add build support for Android:
npx @ionic/cli capacitor add android
Run app on iOS or Android
To run app on iOS or Android, first we have to create a web build. Use the following command to create a web build:
npx nuxi generate
Once we create a web build, we need to update our Capacitor project directories with our latest app build.
npx cap sync
It will update the capacitor project directories.
To run the app on iOS, use the following command:
npx cap run ios
To run the app on Android, use the following command:
npx cap run android
Make sure to run npx cap sync
after every new build to keep your Android and/or iOS capacitor project up-to-date.
Wrapping Up
Thanks for reading. I hope you found this article helpful. Any suggestions or corrections are appreciated.
References
Subscribe to my newsletter
Read articles from Abhijeet Sonawane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
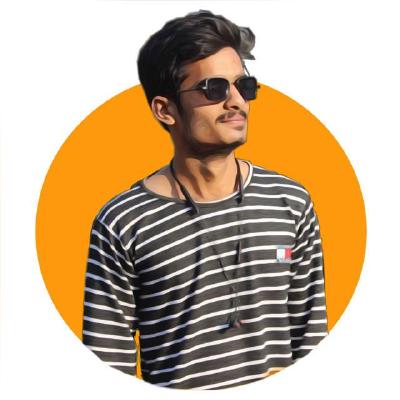
Abhijeet Sonawane
Abhijeet Sonawane
Hello, I'm Abhijeet Sonawane, a dedicated Software Engineer with a passion for Python, Django, Flask, PostgreSQL, HTML, CSS and JavaScript, coupled with a flair for Tailwind CSS. I'm a passionate self-taught enthusiast. Let's learn and grow together in the world of tech!