Mastering MERN: A Beginner's Guide
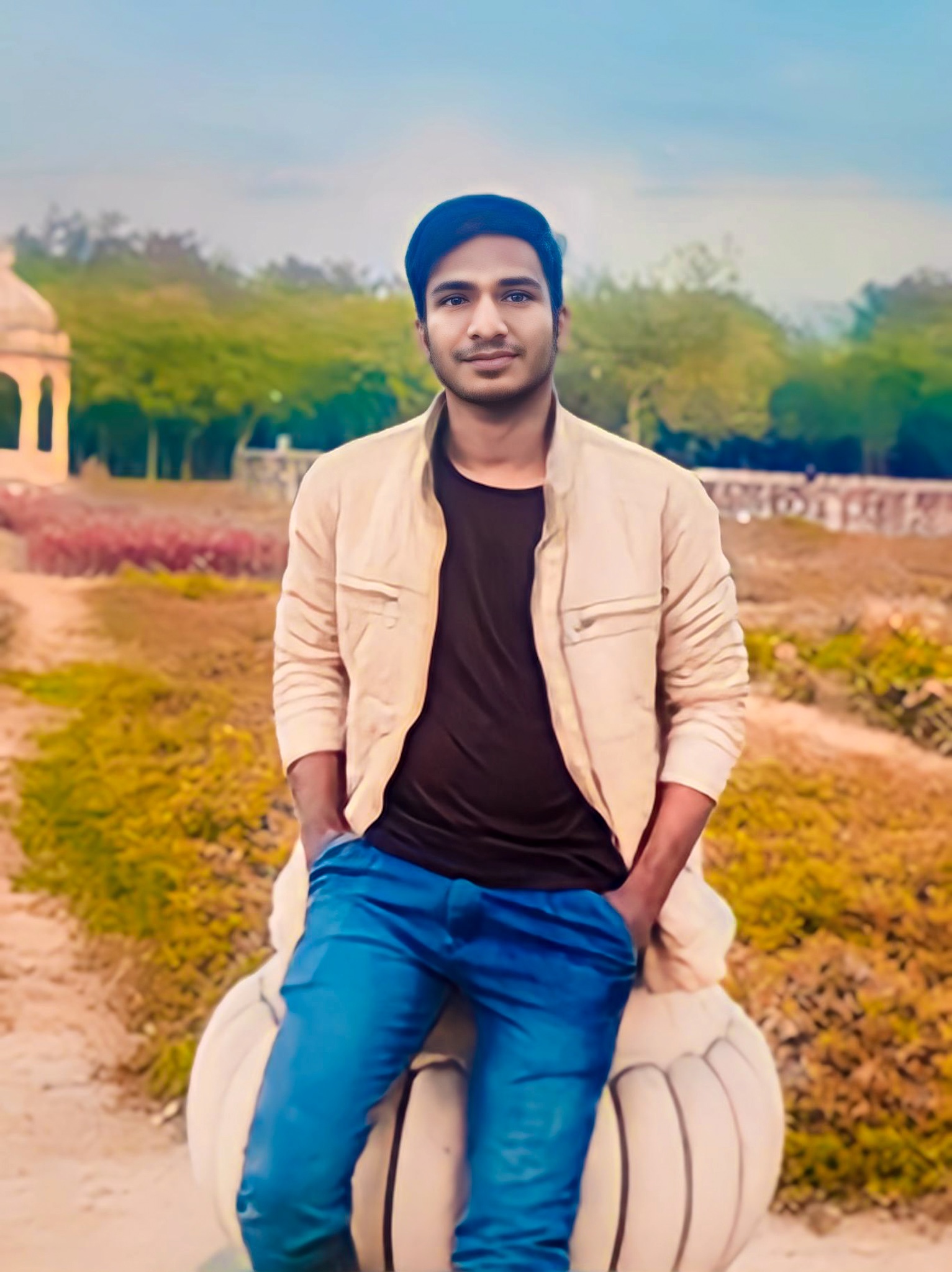
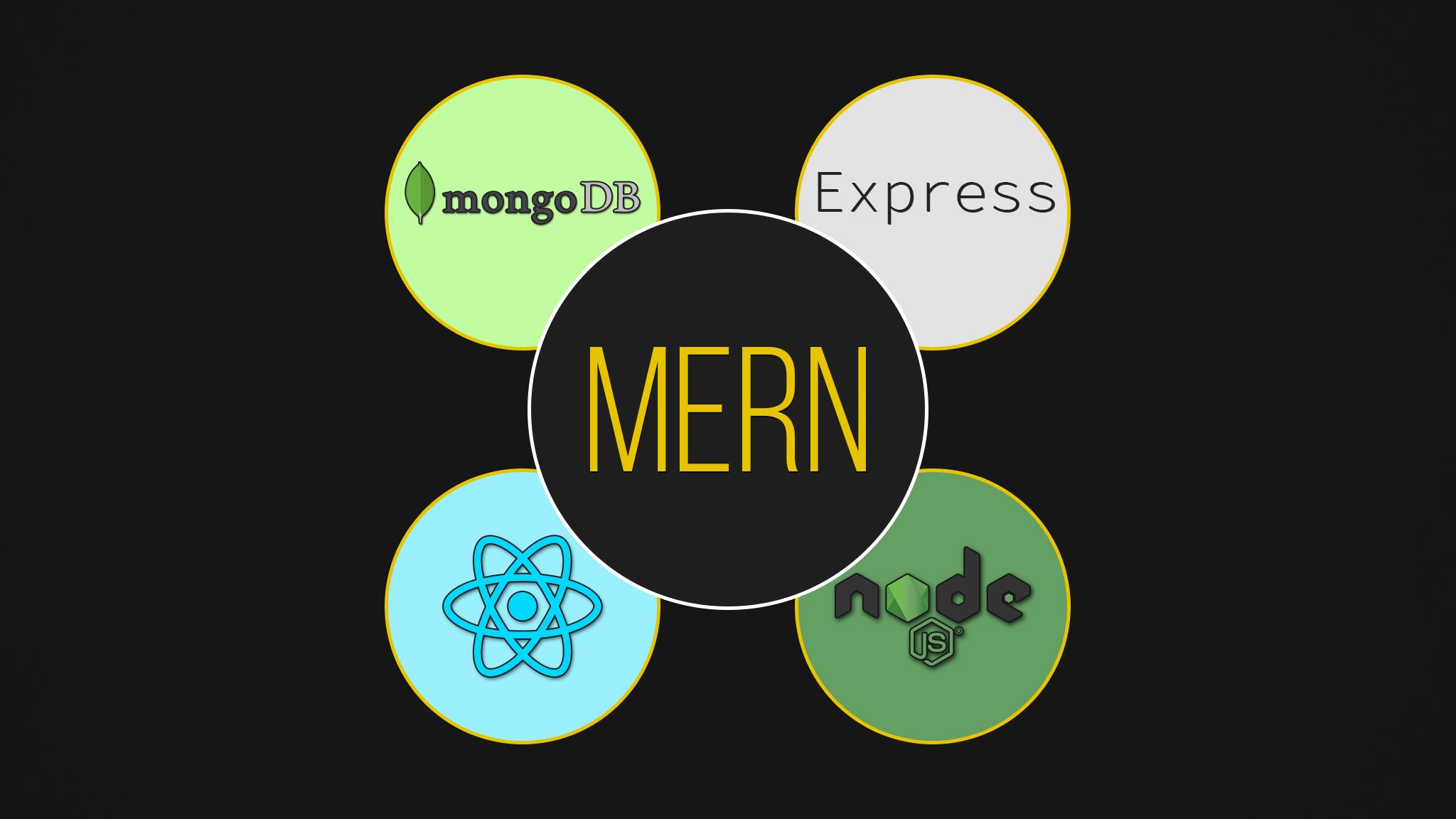
Introduction to MERN Development
Overview of the MERN Stack
The MERN stack is a powerful combination of four technologies which is used for building modern web applications. It's consist of four main components:
MongoDB : These is a NoSQL database used for storing data in a JSON-like document. It's store data in a flexible way.
Express : It's is a web application framework that runs on node.js. and provide a set of tools for building server-side of web application.
React : A JavaScript library for building user-interface, it's known for component based architecture and declarative syntax.
Node.js: A JavaScript runtime environment that allows for server-side execution of JavaScript code.
Why Choose MERN for Web Development
There are several reasons why MERN is a popular choice for web development.
Full-Stack-JavaScript : MERN allows developer to build full-stack web application using a single language, JavaScript. This make it easier to develop and maintain code , as well as share code between between the front-end and back-end.
Scalability : MERN stack is highly scalable and can handle large amounts of data and traffic.
Rich User Interfaces : React.js allows for the creation of dynamic and interactive user interfaces, leading to a better user experience.
Community : MERN stack is open-source and has a large community of developers, which means that there are many resources and tools available for developers to use.
Prerequisites for learning MERN development
Before diving into MERN development, it's essential to have a basic understanding of:
HTML and CSS : Familiarity with HTML markup and CSS styling is crucial for building web interfaces.
JavaScript : A solid foundation in JavaScript programming is necessary, including concepts like variables, functions, arrays, and objects.
Node.js and npm : Understanding the basics of Node.js and npm (Node Package Manager) will be helpful for server-side development.
Databases concepts : Some knowledge of databases and querying languages (e.g., SQL) will aid in understanding MongoDB and database interactions.
Finally, you should have a strong desire to learn and be willing to put in the time and effort to become proficient in MERN development.
Getting Started with HTML, CSS and JavaScript :
Getting Started with HTML basics
HTML (Hypertext Markup Language) is the foundation of any website or web application. It defines the structure and content of your webpages by using tags and elements. Here are some key points about getting started with HTML :
Understand basic HTML syntax : Tags come in pairs – opening tag
<tagname>
and closing tag</tagname>
. Elements consist of both an opening and closing tag enclosing text or other elements.Use semantic HTML : Choose appropriate HTML5 elements like
<header>
,<nav>
,<main>
,<section>
, etc., instead of generic containers like<div>
and<span>
.Learn how to create headings, paragraphs, lists, images, links, forms, tables, and more.
Learning CSS for Styling Web Pages
CSS (Cascading Style Sheets) adds style and visual appeal to your web pages. Here are some tips for starting out with CSS :
Familiarize yourself with selectors : Selectors allow you to target specific HTML elements based on their ID, class, type, or location within the document tree.
Get comfortable with properties and values : Properties define what aspect of an element you want to change, such as color, font size, background image, etc..
Practice creating stylesheets : Apply CSS rules either inline, embedded in the HTML file, or linked from an external file.
Explore advanced techniques like flexbox, grid layout, animations, transitions, and media queries.
Introduction to JavaScript Programming
JavaScript is a powerful scripting language used to add interactivity and dynamic behavior to websites. To get started with JavaScript :
Install a modern browser : Most browsers support JavaScript natively, but Chrome and Firefox offer developer tools that make debugging and testing easier.
Set up a local environment : A simple way to start coding is to create a new HTML file and include a
<script></script>
block where you will place your JavaScript code.Write your first program : Create variables, perform arithmetic operations, display output, and respond to events like button clicks.
Study JavaScript fundamentals : Variables, functions, conditionals, loops, arrays, objects, and event handling are all essential concepts to master.
Dive into JavaScript libraries and frameworks : Popular options include jQuery, React, Angular, Vue, and Svelte, each offering different features and benefits.
Remember that practice is crucial when learning any programming language. Try applying your newly acquired skills to small projects and experiment with various examples to solidify your understanding. Happy coding!
Building a Strong Foundation
Exploring MongoDB for Data Storage
MongoDB is a popular NoSQL database that uses JSON-like documents for storing data. Key aspects of working with MongoDB include :
Document-Oriented Data Model : Understanding MongoDB's document-based data model, which allows for storing data in JSON-like documents.
CRUD Operations : Learning how to perform Create, Read, Update, and Delete operations using MongoDB's query language and methods.
Indexes and Aggregation : Exploring advanced features like indexing for optimizing queries and aggregation pipelines for data analysis.
Getting Started with Express.js for Server-Side Development
Express.js is a minimalist web application framework built on top of Node.js. Some important things to know about Express.js :
Routing : Define routes to handle incoming requests and send responses.
Middleware : Add functionality before or after routing.
Template engines : Render templates dynamically using Handlebars, EJS, or another engine.
Error handling : Catch errors and provide custom error messages.
Understanding React.js for Building User Interfaces
React is a popular JavaScript library for building user interfaces. When diving into React, consider the following :
Components : Break down UI into reusable components.
JSX : Combine JavaScript and HTML to describe component markup.
State management : Manage state within components using hooks or Redux.
Lifecycle methods : Perform actions at specific stages during a component's lifecycle.
Introduction to Node.js for Server-Side JavaScript
Node.js is a JavaScript runtime environment that enables running JavaScript outside of the browser. Important aspects of Node.js include :
Asynchronous I/O : Process input and output efficiently without blocking the event loop.
Event-driven architecture : Respond to events triggered by users, filesystem interactions, network connections, etc.
Modules : Organize code into separate modules and manage dependencies via npm.
By exploring these technologies and practicing their usage, you will establish a strong foundation for developing web applications using the MERN stack.
Practical Exercises and Projects
Building CRUD Applications with MERN Stack
CRUD (Create, Read, Update, Delete) applications are a common type of web application that allow users to interact with data. To build a CRUD application with the MERN stack, you can follow these steps :
Set up a MongoDB database and create a schema for your data.
Create an Express.js server to handle HTTP requests and connect to the MongoDB database.
Build a React front-end to display and manipulate data.
Implement CRUD functionality by creating routes in Express.js to handle create, read, update, and delete operations.
Creating RESTful APIs with Express.js and MongoDB
RESTful APIs are a popular way to expose data and functionality to other applications. To create a RESTful API with Express.js and MongoDB, you can follow these steps :
Set up a MongoDB database and create a schema for your data.
Create an Express.js server to handle HTTP requests and connect to the MongoDB database.
Define routes in Express.js to handle CRUD operations.
Use middleware to handle authentication, validation, and error handling.
Test your API using tools like Postman or Insomnia.
Implementing User Authentication and Authorization
User authentication and authorization are important aspects of many web applications. To implement user authentication and authorization with the MERN stack, you can follow these steps :
Authentication:
Choose an authentication method: Decide whether you'll implement traditional session-based authentication or token-based authentication (JWT).
Session-based authentication: Use libraries like Passport.js with Express.js to handle user login, session management, and authentication strategies (e.g., local, OAuth).
Token-based authentication: Implement JSON Web Tokens (JWT) for stateless authentication. Upon successful login, generate a token containing user information and send it to the client to include in subsequent requests.
Secure sensitive information: Store passwords securely using techniques like salting and hashing to protect user credentials.
Authorization:
Define user roles and permissions: Determine the access levels (e.g., admin, user) and the actions each role can perform (e.g., create, read, update, delete).
Middleware for authorization: Create middleware functions in Express.js to check whether a user is authorized to access certain routes or perform specific actions. For example, verify the user's role or check if the user owns the resource they're trying to modify.
Protect routes: Apply authorization middleware to routes that require authentication and specific permissions. Redirect unauthorized users or return appropriate error responses.
Conclusion
Mastering MERN development requires a solid understanding of MongoDB, Express.js, React, and Node.js, along with proficiency in HTML, CSS, and JavaScript. By building projects, practicing coding exercises, and exploring real-world examples, you can strengthen your skills and become proficient in developing modern web applications.
Remember to stay updated with the latest trends, best practices, and tools in the MERN ecosystem. Leverage online resources, documentation, and developer communities to continuously learn and improve your skills.
Whether you're a beginner or an experienced developer, the MERN stack offers a versatile and powerful platform for building scalable, dynamic, and feature-rich web applications. Embrace the journey of learning and mastering MERN development, and enjoy creating innovative solutions to solve real-world problems. Happy coding!
Subscribe to my newsletter
Read articles from Prince Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
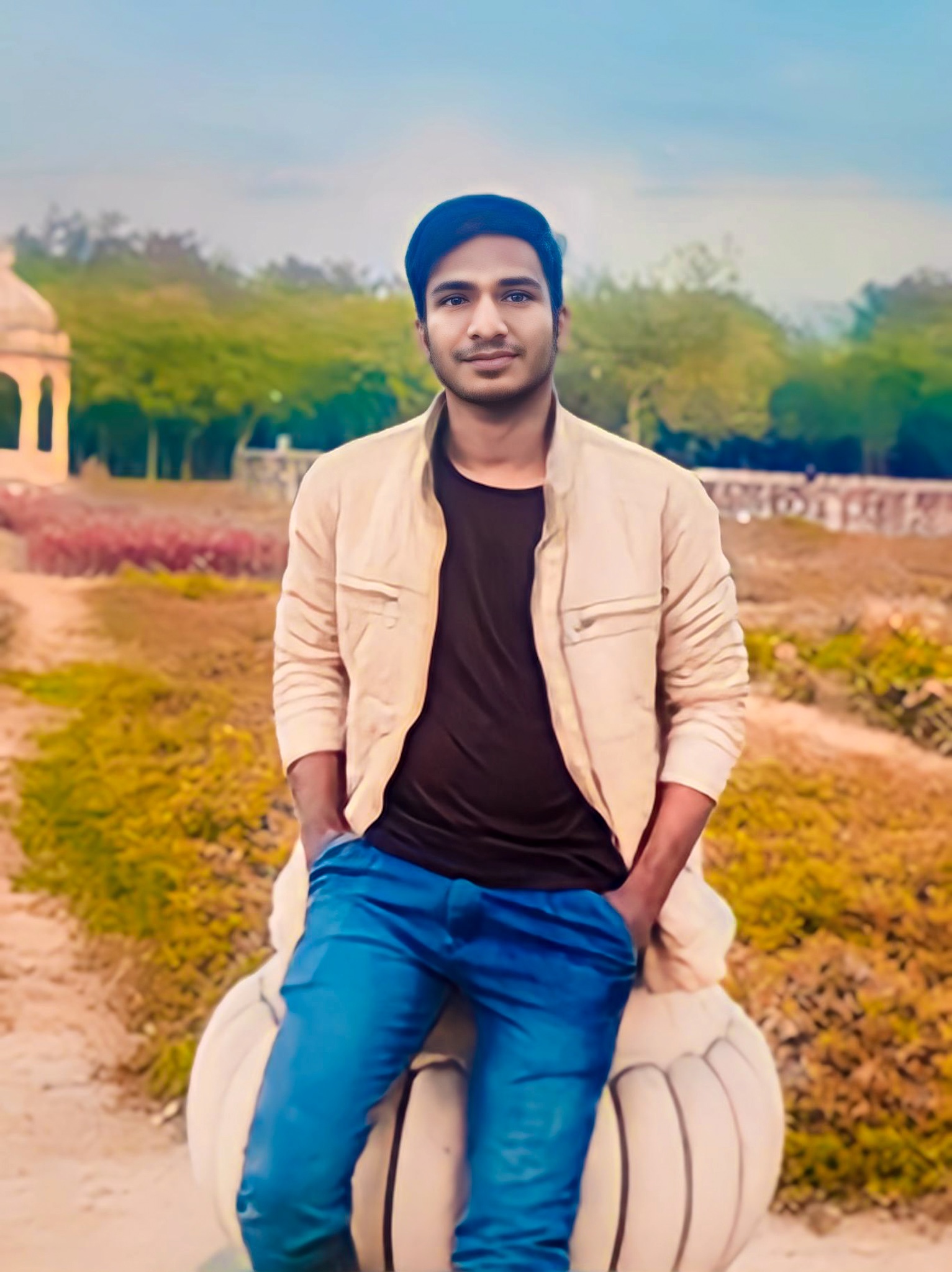
Prince Kumar
Prince Kumar
Hello, I'm Prince, a passionate MERN (MongoDB, Express.js, React.js, Node.js) developer with a keen interest in crafting scalable and efficient web applications. With experience in both front-end and back-end development, I enjoy bringing ideas to life through clean, maintainable code. Let's build something amazing together!