Exploring the useEffect Hook in React

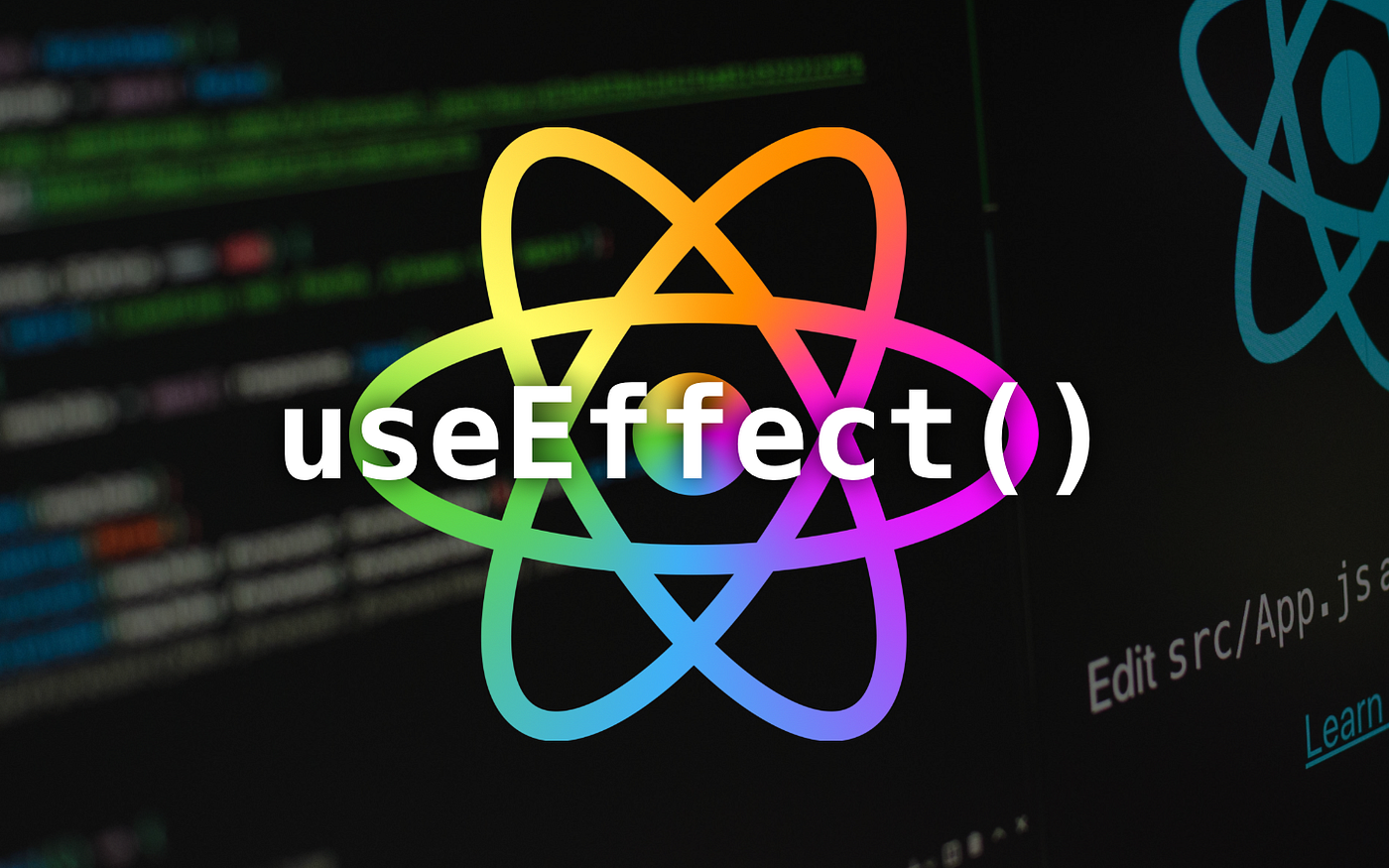
Today, let's delve into the useEffect hook in React, a powerful tool for managing side effects within functional components. I'll walk you through its functionality, the significance of the dependencies array, and the vital role cleanup plays.
Understanding the useEffect Hook:
At its core, the useEffect hook enables you to perform side effects within your React components. These side effects encompass tasks such as fetching data, interacting with the DOM, or establishing subscriptions—actions that go beyond mere rendering.
How It Operates:
When incorporated into a component, useEffect notifies React that after rendering, certain actions need to be executed. React then memorizes the provided function and triggers it subsequent to DOM updates. Effectively, useEffect amalgamates the functionalities of the old lifecycle methods—componentDidMount, componentDidUpdate, and componentWillUnmount.
import React, { useState, useEffect } from 'react';
function App() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `Clicks: ${count}`;
});
return (
<div>
<p>Clicks: {count}</p>
<button onClick={() => setCount(count + 1)}>
Click me!
</button>
</div>
);
}
The Dependencies Array:
Additionally, useEffect allows you to manage when your effects occur by specifying a dependencies array. This array comprises values that, upon alteration, trigger the effect to reoccur.
useEffect(() => {
console.log('Effect executed!');
}, [count]); // Runs whenever 'count' changes
Cleanup in useEffect:
What does cleanup entail? Cleanup within useEffect pertains to halting or eliminating actions initiated by the hook to prevent potential repercussions on other components or system functionalities. It involves tasks like terminating data subscriptions, clearing timers, or reverting DOM modifications.
useEffect(() => {
const timer = setTimeout(() => console.log('Timer done!'), 1000);
return () => {
clearTimeout(timer);
console.log('Cleanup executed!');
};
}, []);
The Importance of Cleanup:
Why is cleanup indispensable? It's crucial for optimizing memory usage and maintaining app performance. Analogous to turning off devices in a room to conserve energy, cleanup ensures that unnecessary tasks are ceased, preventing memory leaks and runtime issues.
When to Implement Cleanup:
Cleanup should be executed when components are unmounted or before re-executing an effect to prevent conflicts and ensure smooth functionality.
In Summary:
The useEffect hook empowers React developers to manage side effects efficiently while maintaining code cleanliness and performance. By leveraging dependencies arrays and cleanup functions, you can ensure that your app runs smoothly, free from memory leaks and performance bottlenecks.
Subscribe to my newsletter
Read articles from Yassine Cherkaoui directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
