Next.js Rendering Wars: Decoding CSR, SSR, and SSG for Maximum Impact
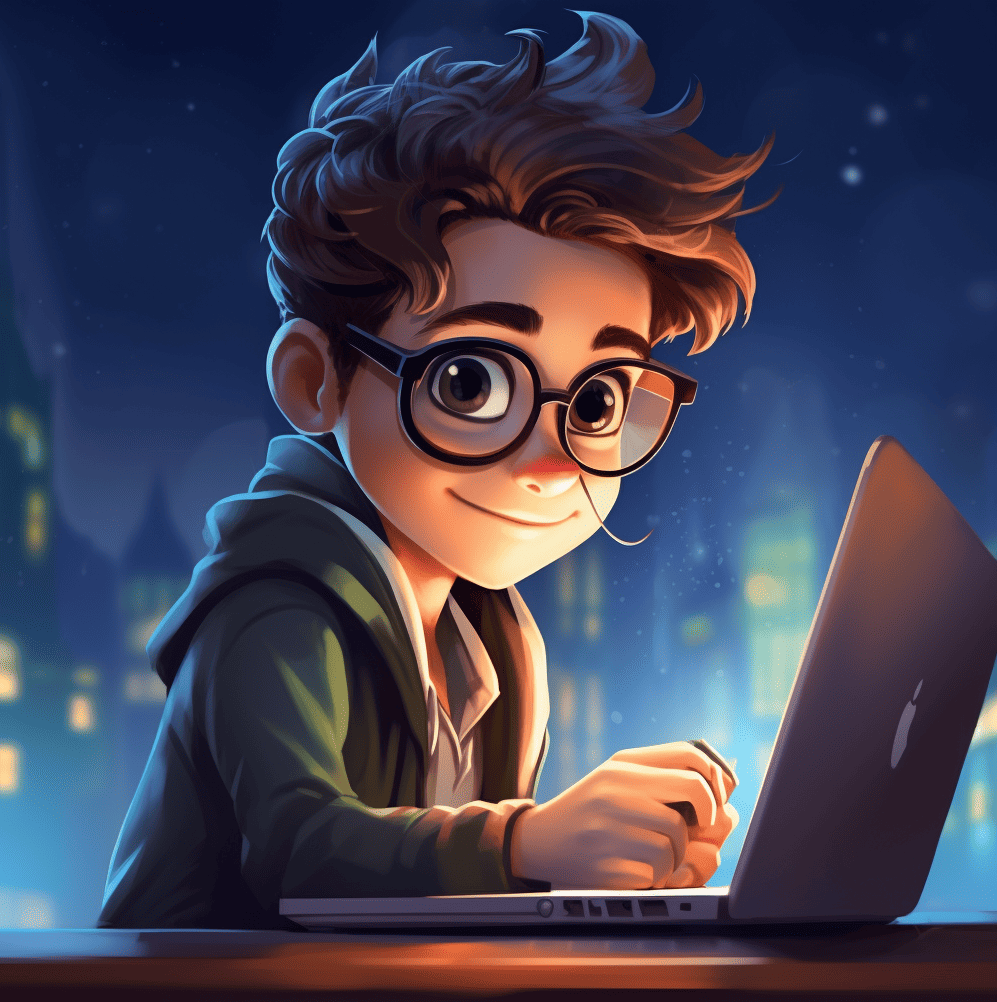
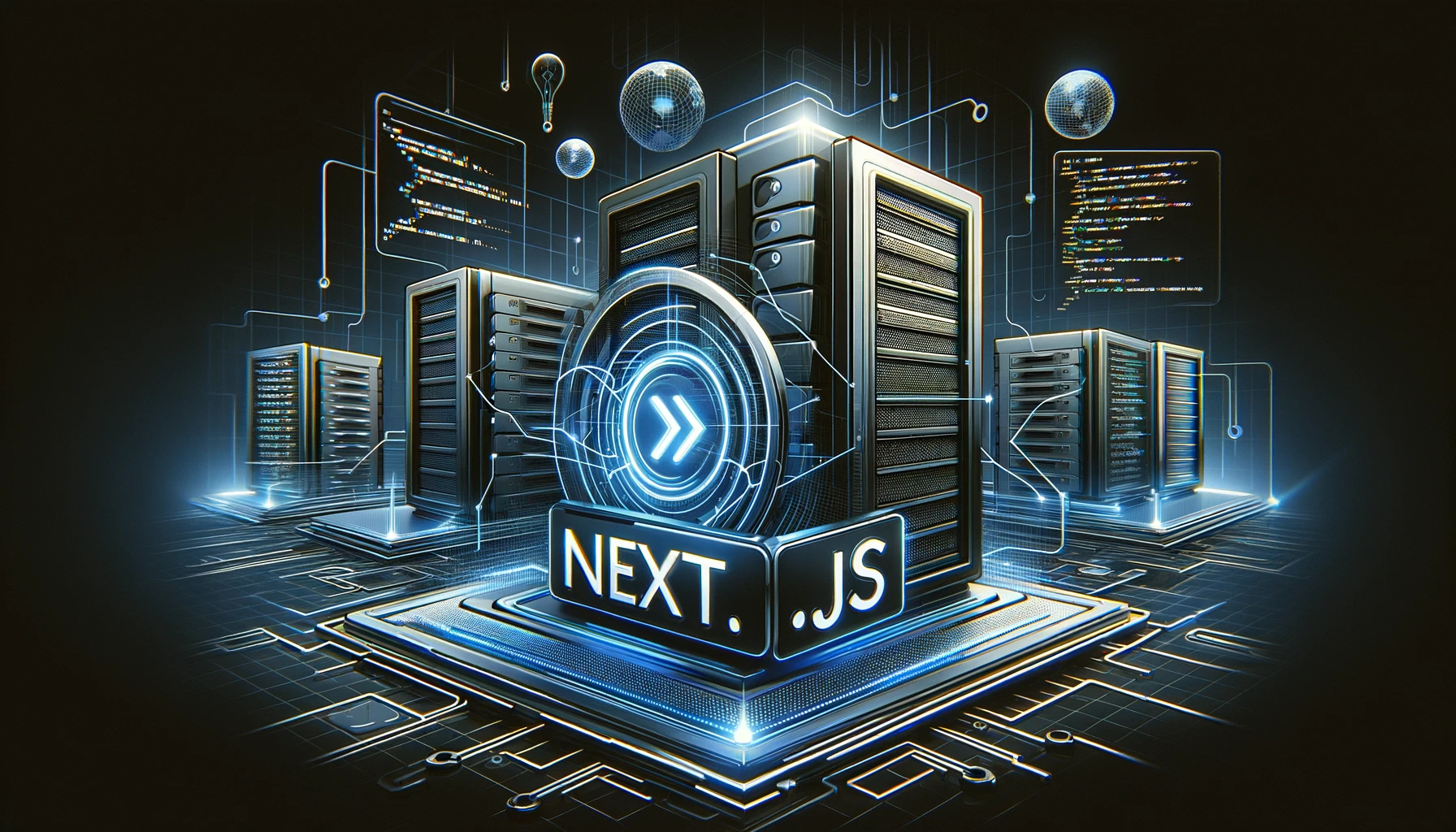
Exploring Next.js: Client Side vs Server Side Rendering vs Static Site Generation
Introduction
In the realm of modern web development, choosing the right rendering method is crucial for optimal performance and user experience. Next.js, a popular React framework, offers three main rendering methods: Client Side Rendering (CSR), Server Side Rendering (SSR), and Static Site Generation (SSG). Each method has its strengths and weaknesses, making it essential to understand their differences and use cases. Let's delve into each method, providing detailed examples and covering all scenarios.
Client Side Rendering (CSR)
Client Side Rendering (CSR) is the traditional approach where the browser fetches a minimal HTML page containing JavaScript. Subsequently, the JavaScript code fetches data from an API and renders the page dynamically on the client side.
Advantages of CSR:
Fast Initial Page Load: The initial HTML page is lightweight, leading to faster initial page loads.
Rich Interactivity: Since rendering happens on the client side, the user experiences seamless interactions without full-page reloads.
Dynamic Content: CSR is ideal for applications requiring real-time data updates or personalized content.
Disadvantages of CSR:
SEO Challenges: Search engine crawlers may face difficulties in indexing dynamically rendered content, affecting SEO performance.
Performance Issues on Low-end Devices: CSR may lead to slower page rendering on low-end devices due to the client-side processing overhead.
Poor First Contentful Paint (FCP): Users may experience a delay in seeing meaningful content as JavaScript executes to render the page.
Example:
// pages/index.js
import React, { useState, useEffect } from 'react';
const Home = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
return (
<div>
<h1>Client Side Rendering Example</h1>
{data.map(item => (
<div key={item.id}>{item.name}</div>
))}
</div>
);
};
export default Home;
Server Side Rendering (SSR)
Server Side Rendering (SSR) involves rendering the HTML content on the server and sending a fully formed HTML page to the client.
Advantages of SSR:
Improved SEO: SSR ensures that search engines receive fully rendered HTML content, enhancing SEO performance.
Better Performance on Low-end Devices: Since the server handles rendering, users on low-end devices experience faster initial page loads.
Graceful Degradation: SSR ensures that users receive content even if JavaScript fails to load or execute on the client side.
Disadvantages of SSR:
Increased Server Load: Rendering pages on the server can lead to increased server load, especially for high-traffic websites.
Complexity in Caching: Caching SSR pages requires careful consideration to ensure cache coherence and efficiency.
Limited Client-side Interactivity: SSR limits client-side interactivity compared to CSR, as certain functionalities require client-side JavaScript execution.
Example:
// pages/index.js
import React from 'react';
const Home = ({ data }) => (
<div>
<h1>Server Side Rendering Example</h1>
{data.map(item => (
<div key={item.id}>{item.name}</div>
))}
</div>
);
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return {
props: {
data,
},
};
}
export default Home;
Static Site Generation (SSG)
Static Site Generation (SSG) involves pre-rendering pages at build time, generating static HTML files that are served to clients.
Advantages of SSG:
Superior Performance: SSG delivers blazing-fast page loads since content is pre-rendered and served as static files.
Low Server Load: With no need for server-side rendering on each request, SSG reduces server load significantly.
Excellent SEO: SSG ensures that search engines receive fully rendered HTML content, maximizing SEO benefits.
Disadvantages of SSG:
Limited Dynamic Content: SSG is not suitable for highly dynamic content that requires real-time updates.
Build Time Overhead: Large websites with complex content structures may experience longer build times due to SSG.
Cache Invalidation Challenges: Updating content frequently may require careful cache invalidation strategies to ensure timely updates.
Example:
// pages/index.js
import React from 'react';
const Home = ({ data }) => (
<div>
<h1>Static Site Generation Example</h1>
{data.map(item => (
<div key={item.id}>{item.name}</div>
))}
</div>
);
export async function getStaticProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return {
props: {
data,
},
};
}
export default Home;
FAQs
1. When should I use Client Side Rendering (CSR)?
CSR is suitable for applications requiring dynamic content updates and rich client-side interactivity. It's ideal for single-page applications (SPAs) and web apps with real-time data requirements.
2. When is Server Side Rendering (SSR) a better choice?
SSR shines in scenarios where SEO is crucial, and initial page load performance is a priority. Content-heavy websites, blogs, and e-commerce platforms can benefit significantly from SSR.
3. Why choose Static Site Generation (SSG)?
SSG is perfect for content-focused websites, blogs, and documentation sites where content changes infrequently. It offers unmatched performance, SEO benefits, and scalability, making it an excellent choice for various projects.
4. Can I combine these rendering methods in a Next.js application?
Yes, Next.js allows hybrid rendering, enabling developers to combine CSR, SSR, and SSG based on specific page requirements. This flexibility empowers developers to optimize performance and user experience effectively.
Conclusion
Choosing the right rendering method in Next.js depends on various factors such as SEO requirements, performance goals, and content dynamics. By understanding the differences between Client Side Rendering, Server Side Rendering, and Static Site Generation, developers can make informed decisions to create efficient and scalable web applications.
Subscribe to my newsletter
Read articles from chintanonweb directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
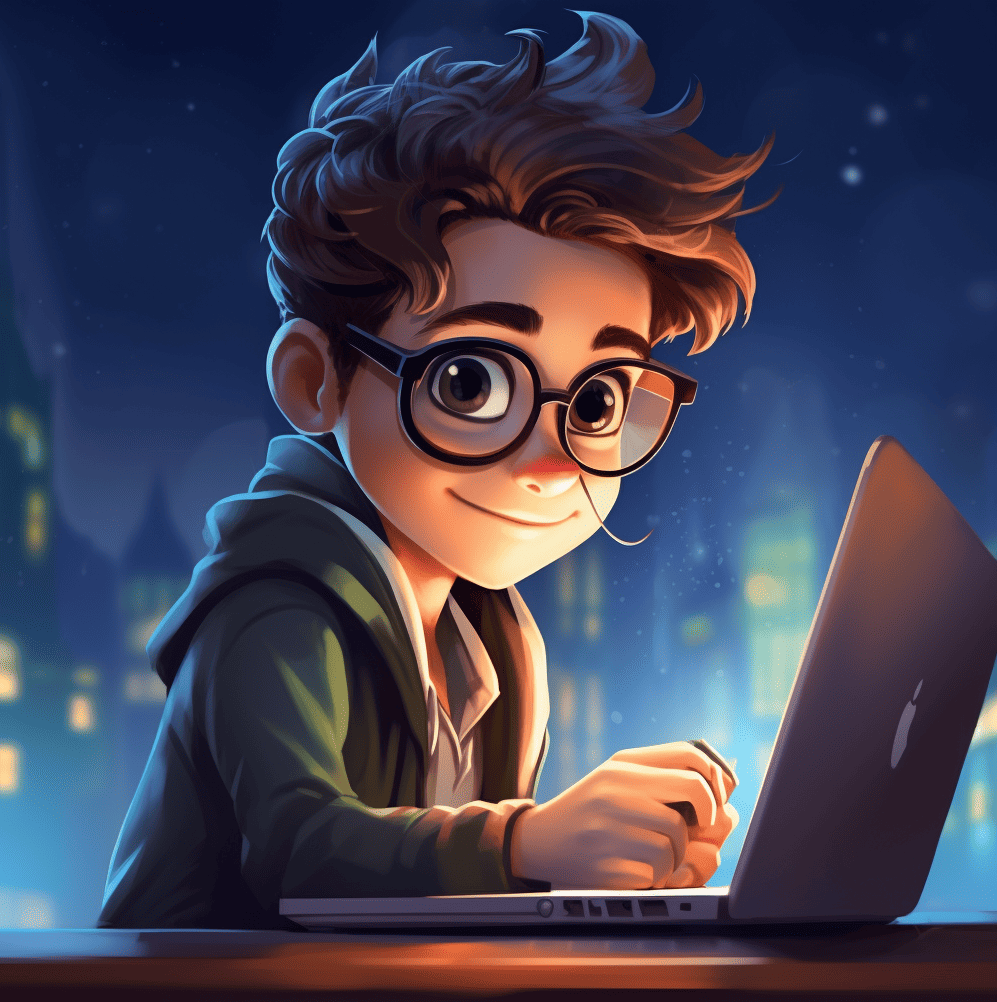
chintanonweb
chintanonweb
As a software engineer, I find joy in turning imaginative ideas into tangible digital experiences. Creating a better world through code is my passion, and I love sharing my vision with others.