Best Practices for Pagination in ClickHouse Databases
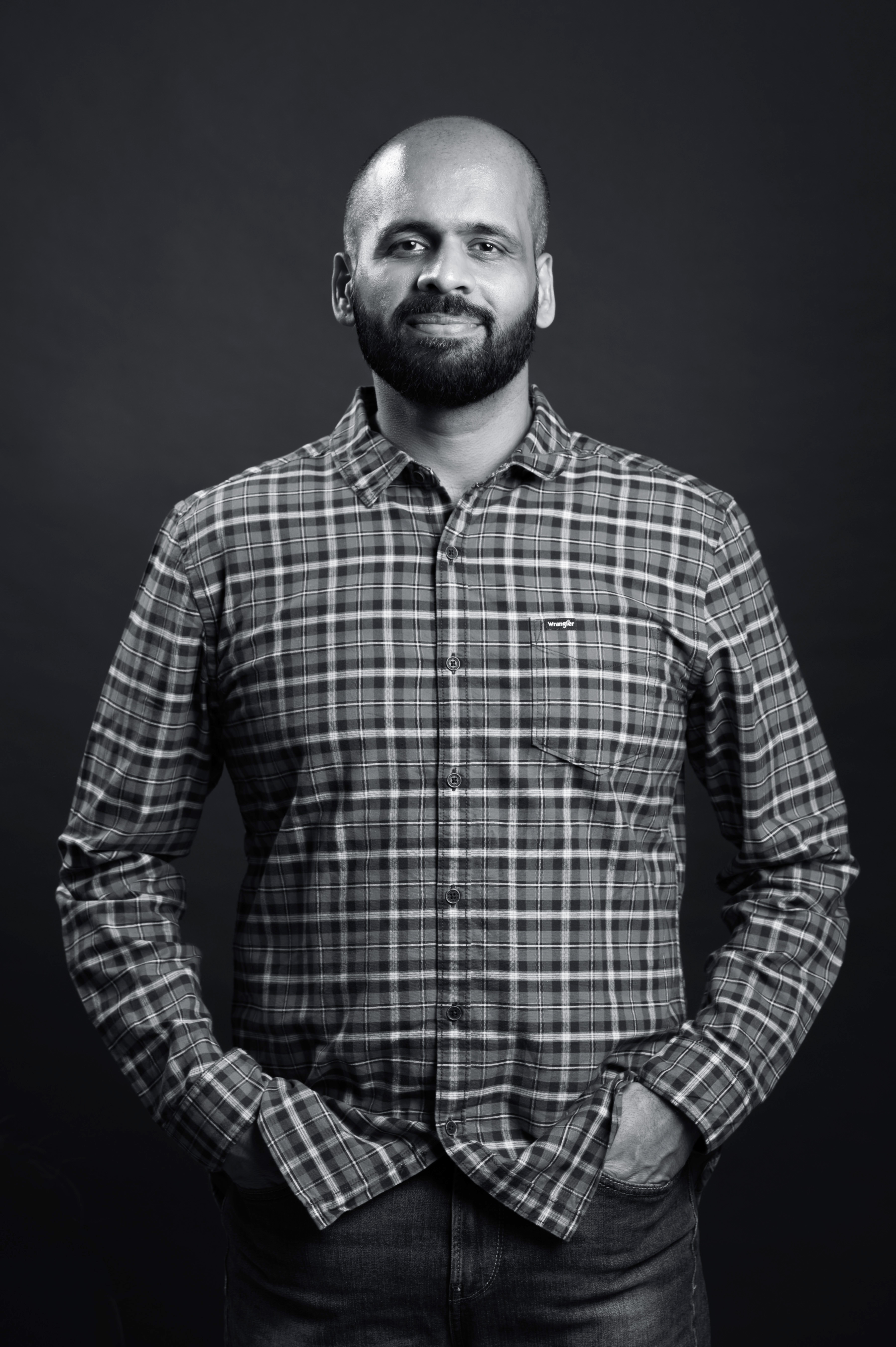
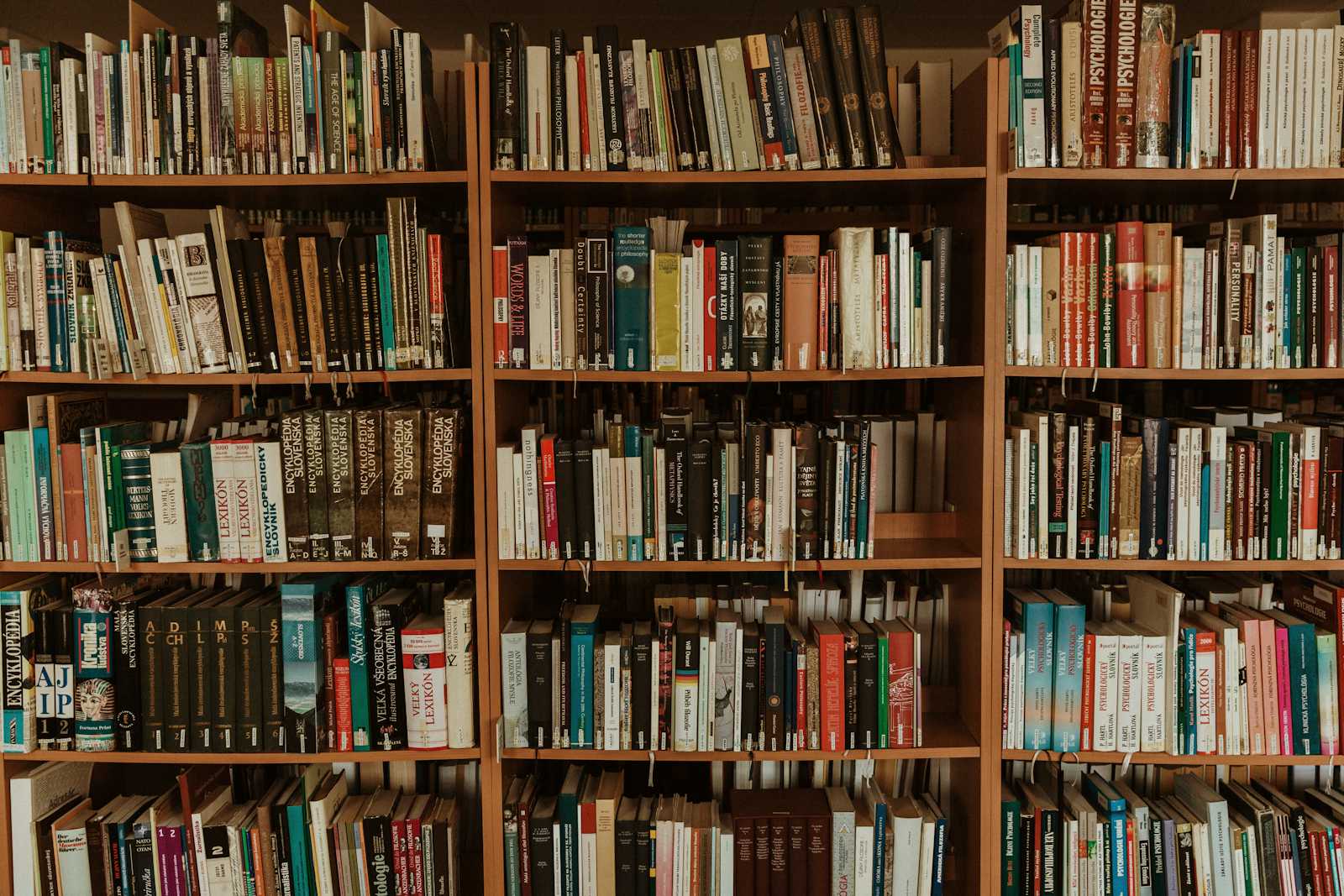
Implementing pagination in ClickHouse is crucial for efficiently handling large datasets, especially when building applications that require user interaction with substantial amounts of data. ClickHouse provides several approaches to achieve pagination, with considerations for performance and scalability. Here’s how you can implement pagination through a result set in ClickHouse:
Basic OFFSET and LIMIT Pagination
The simplest way to implement pagination is by using LIMIT
and OFFSET
clauses. This is straightforward but can become inefficient for very large datasets because OFFSET
skips the number of rows before starting to return the rows from LIMIT
.
Example Query:
SELECT * FROM my_table
ORDER BY id
LIMIT 100 OFFSET 200;
This query skips the first 200 rows and returns the next 100 rows. It's suitable for smaller or medium-sized datasets.
Keyset Pagination (Seek Method)
For larger datasets, keyset pagination (also known as the "seek method") is more efficient. This method uses a WHERE clause to filter rows beyond the last retrieved row's key, avoiding the performance cost of skipping rows.
Example Setup: Suppose you have a dataset ordered by a unique ID. To paginate through this dataset, you start by selecting the first page without an OFFSET
.
First Page Query:
SELECT * FROM my_table
ORDER BY id
LIMIT 100;
Subsequent Page Query: Assume you know the last ID retrieved from the previous query, say last_id
.
SELECT * FROM my_table
WHERE id > last_id
ORDER BY id
LIMIT 100;
This method is highly efficient because it uses the index on id
to quickly find the starting point of each new page.
Using Array Join for Pagination
You can use ClickHouse features like arrayJoin
to implement pagination. This involves transforming the rows into arrays, which can then be paginated.
Example Query:
WITH
groupArray(id) AS ids,
groupArray(name) AS names
SELECT
arrayJoin(ids) AS id,
arrayJoin(names) AS name
FROM my_table
LIMIT 100 OFFSET 200;
This method is particularly useful when combined with aggregation functions and where pagination over aggregated results is needed.
Considerations for Efficient Pagination
Use Indexing: Ensure that columns used in the
ORDER BY
for pagination are indexed. This is crucial for performance, especially with the seek method.Avoid OFFSET for Large Skips: As mentioned, using
OFFSET
can be inefficient for large datasets because it reads and discards rows. The seek method is usually better for high-offset scenarios.Stateless Pagination: In stateless pagination (typical in web applications), remember the last key seen by the user, and use it to fetch the next set of results.
Adjust Page Size: Consider the impact of the page size on performance and user experience. Smaller page sizes reduce the data transferred per query but may increase the total number of queries.
Caching: For datasets that do not change frequently, consider caching the results of paginated queries, especially the first few pages which are accessed more frequently.
By selecting the right method for pagination based on your specific dataset size and access patterns, you can significantly improve the performance of data retrieval operations in ClickHouse. For very large datasets with frequent access, using the seek method with proper indexing generally offers the best performance.
Subscribe to my newsletter
Read articles from Shiv Iyer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
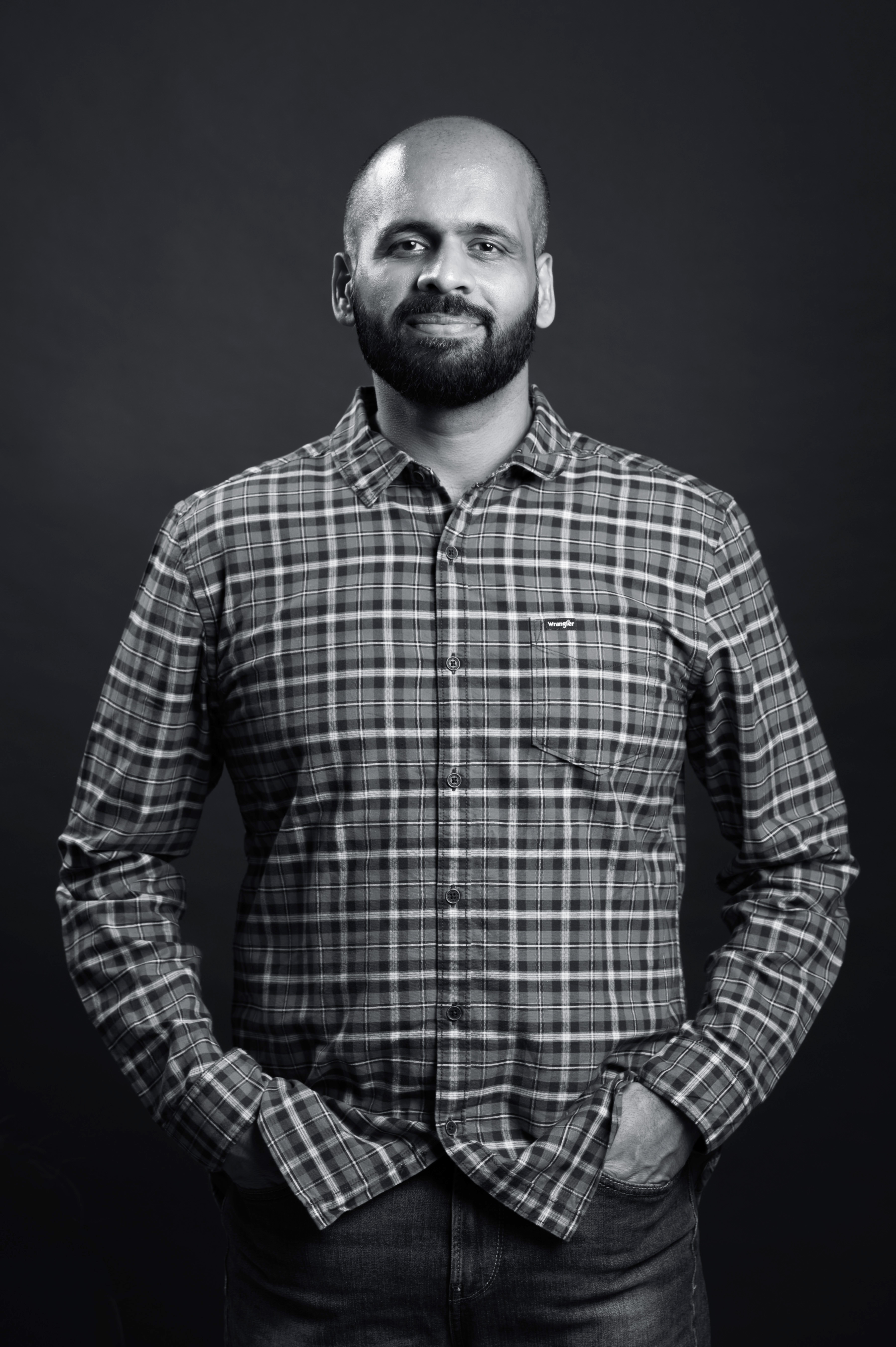
Shiv Iyer
Shiv Iyer
Over two decades of experience as a Database Architect and Database Engineer with core expertize in Database Systems Architecture/Internals, Performance Engineering, Scalability, Distributed Database Systems, SQL Tuning, Index Optimization, Cloud Database Infrastructure Optimization, Disk I/O Optimization, Data Migration and Database Security. I am the founder CEO of MinervaDB Inc. and ChistaDATA Inc.