Laravel Task Scheduling - Counts rows in Users table and writes a log
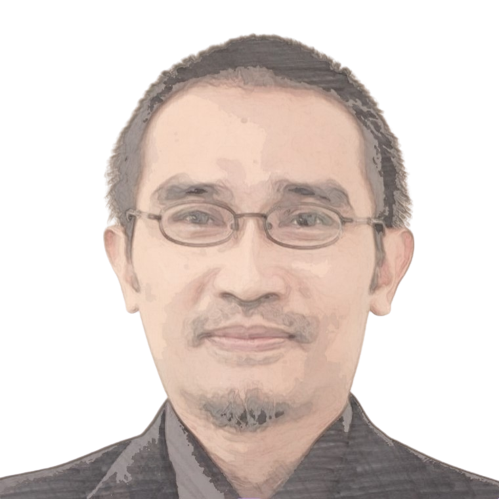
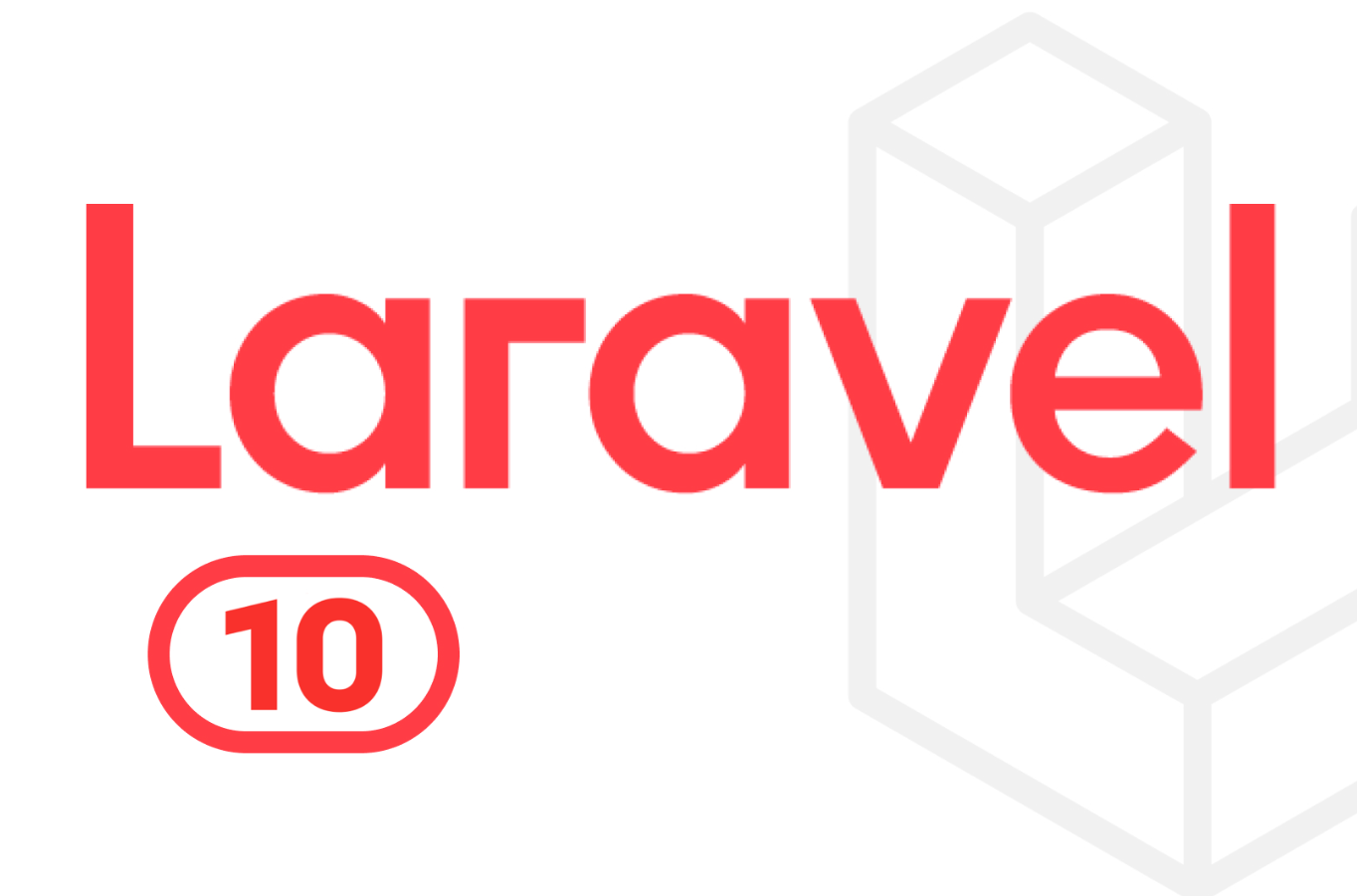
Laravel provides a powerful task scheduling feature that allows you to automate the execution of recurring tasks within your application. Task scheduling enables you to define tasks and specify when and how often they should run. This feature is useful for performing various activities, such as sending emails, cleaning up the database, or performing maintenance tasks.
To use Laravel's task scheduling feature, follow these steps:
Define the Task: In your Laravel application, define the tasks you want to schedule. Tasks are defined as methods on a class, typically located in the
app/Console/Commands
directory or any other directory of your choice. Each task should extend theIlluminate\Console\Command
class.Register the Task: After defining the task, you need to register it in the
app/Console/Kernel.php
file. This file contains the task scheduler configuration. In theschedule
method of theKernel
class, you can specify the tasks and their schedules using a fluent API.Task Execution: To make the task scheduling work, you need to set up a cron job that executes Laravel's
schedule:run
command at regular intervals. Theschedule:run
command checks the defined tasks and runs the ones that are due.Additional Configuration: Laravel's task scheduling also supports additional configuration options. For example, you can specify the user under which the scheduled tasks should run, define the output and error log locations, and more. Refer to the Laravel documentation for advanced configuration options.
[1] Create command
<?php
// App\Console\Commands\CheckUserCountCommand.php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Support\Facades\DB;
use Illuminate\Support\Facades\Log;
class CheckUserCountCommand extends Command
{
protected $signature = 'usercount:check';
protected $description = 'Check row count for users table';
public function handle()
{
$userCount = DB::table('users')->count();
Log::info("User count: $userCount");
}
}
?>
Code explanation:
Namespace and Class Declaration: The code starts by declaring the namespace and the class for the console command. The command is defined in the
App\Console\Commands
namespace, and the class is namedCheckUserCountCommand
.Signature and Description: The
$signature
property defines the command signature. In this case, the signature isusercount:check
, which means the command can be executed usingphp artisan usercount:check
in the console. The$description
property provides a brief description of what the command does.Handle Method: The
handle
method contains the logic that will be executed when the command is run. In this case, it retrieves the row count from theusers
table using theDB
facade'stable
method and thecount
method. The result is stored in the$userCount
variable.Logging: The
Log
facade is used to log the user count information. Theinfo
method is called with a string containing the user count value interpolated using the$userCount
variable. This logs the information to Laravel's default log file.
To use this command, you need to place the code in the appropriate file location, such as app/Console/Commands/CheckUserCountCommand.php
. After that, you can execute the command in the console using php artisan usercount:check
, and it will log the row count of the users
table.
[2] register command
<?php
//C:\laragon\www\razzi1\app\Console\Kernel.php
namespace App\Console;
use Illuminate\Console\Scheduling\Schedule;
use Illuminate\Foundation\Console\Kernel as ConsoleKernel;
class Kernel extends ConsoleKernel
{
/**
* Define the application's command schedule.
*/
protected function schedule(Schedule $schedule): void
{
// $schedule->command('inspire')->hourly();
}
/**
* Register the commands for the application.
*/
protected function commands(): void
{
$this->load(__DIR__.'/Commands');
require base_path('routes/console.php');
}
protected $commands = [
Commands\CheckUserCountCommand::class,
];
}
Code explanation:
Namespace and Class Declaration: The code starts by declaring the namespace and class for the
Kernel
class. The class extends theConsoleKernel
class, which is provided by Laravel.Schedule Method: The
schedule
method is used to define the application's command schedule. In the code you provided, theschedule
method is empty and commented out. You can uncomment this method and use it to define the schedule for your commands. For example, you can use the$schedule
object to schedule commands to run at specific intervals.Commands Method: The
commands
method is used to register the commands for the application. In the code you provided, it loads the commands from theapp\Console\Commands
directory using theload
method. It also includes theroutes/console.php
file.$commands
Property: The$commands
property is an array that lists the commands to be registered with the application. In the code you provided, it includes theCheckUserCountCommand
command by referencing its fully qualified class name.
To use this code, make sure you have the CheckUserCountCommand
class defined in the app\Console\Commands
directory. After that, you can uncomment the schedule
method and define the schedule for your commands. Finally, you can run the scheduled commands using Laravel's task scheduling feature, such as setting up a cron job.
[3] test
To run the usercount:check
command, open your terminal or command prompt, navigate to the root directory of your Laravel application, and execute the following command:
php artisan usercount:check
This will trigger the execution of the handle
method in the CheckUserCountCommand
class. The command will retrieve the row count from the users
table and log the result using Laravel's logging feature.
Subscribe to my newsletter
Read articles from Mohamad Mahmood directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
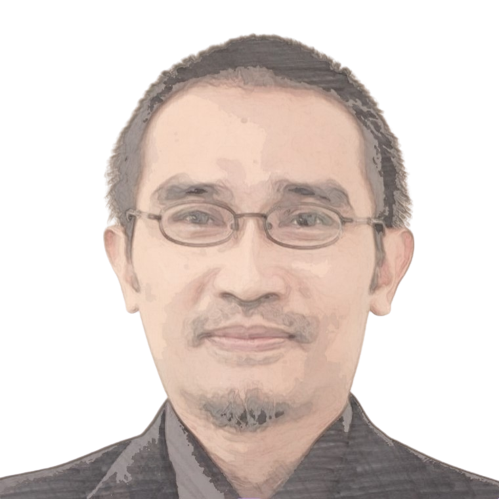
Mohamad Mahmood
Mohamad Mahmood
Mohamad's interest is in Programming (Mobile, Web, Database and Machine Learning). He studies at the Center For Artificial Intelligence Technology (CAIT), Universiti Kebangsaan Malaysia (UKM).