Day 77/100 100 Days of Code
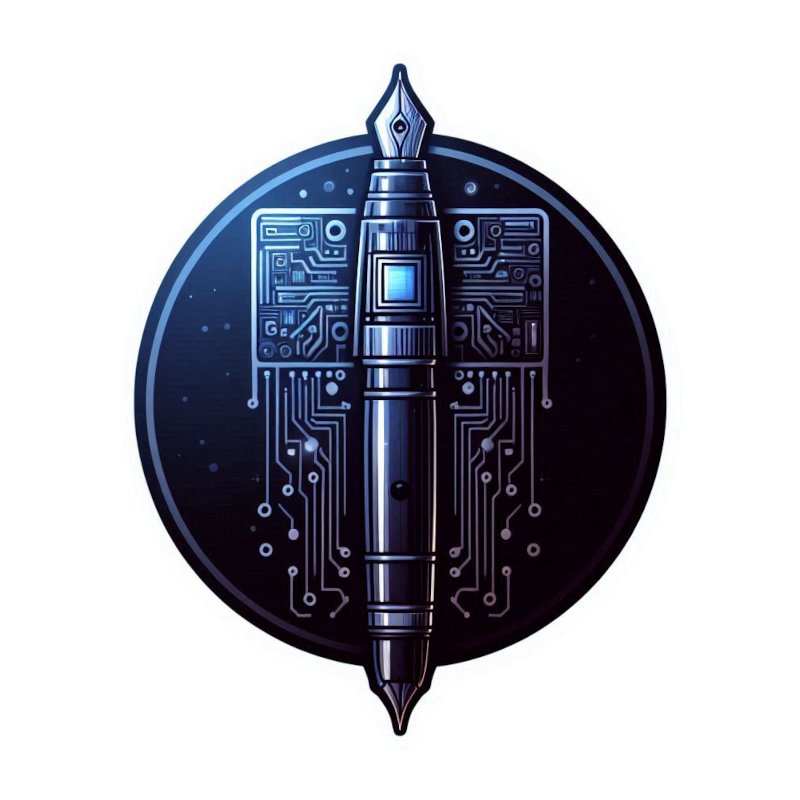
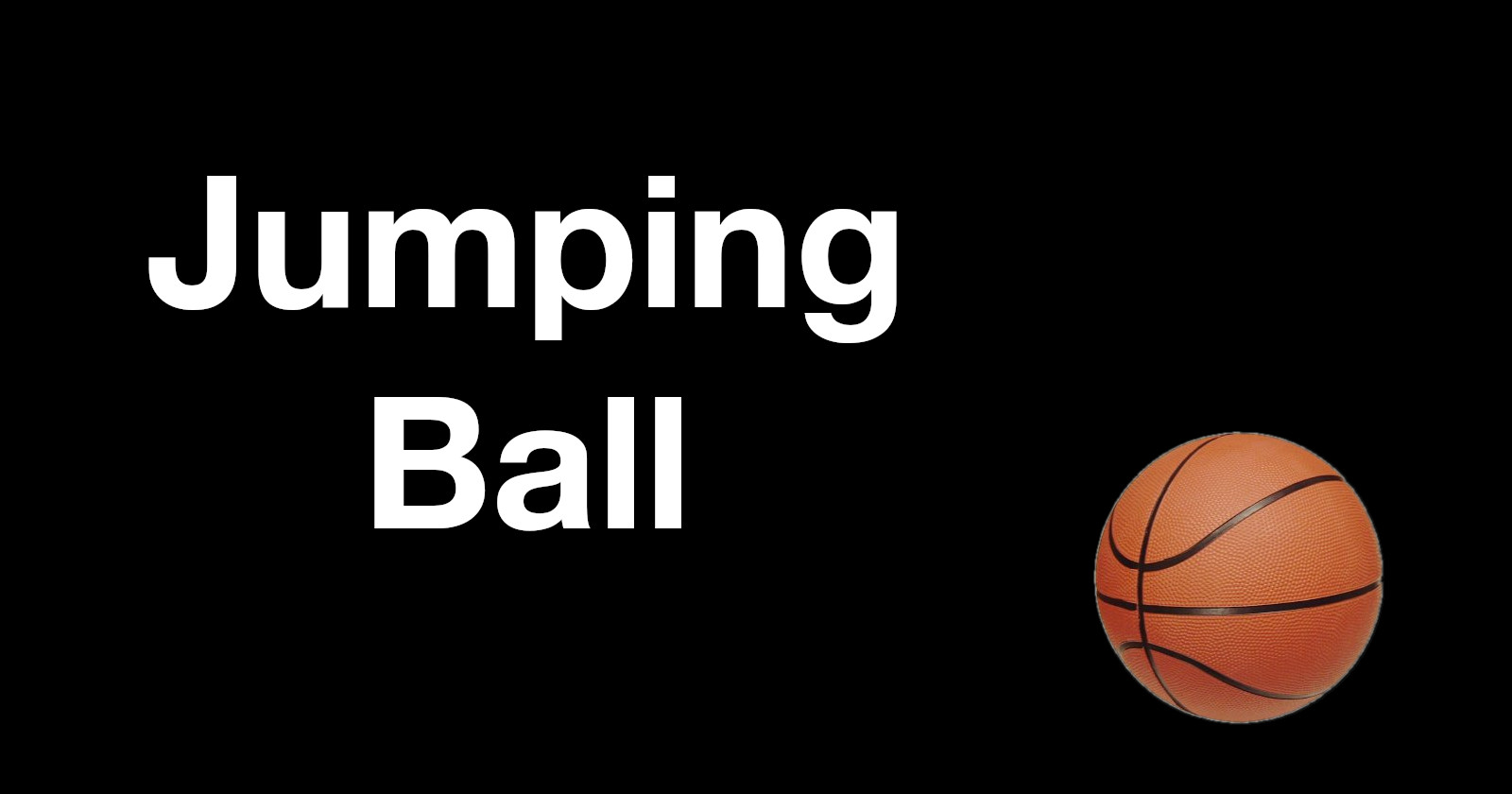
Hey! I figured out what I should name the project. The original name was "jumping_physics" but I didn't really like it. Jumping Ball is much better.
I made a lot of changes to the project and removed every line of code that was relative to C++. Now, the Project is C only.
I started working on the main components of the application and started building the Initialise
, FindResource
, and ExitApplication
functions and created a Resource
struct.
The initialise function will be used to handle the initialisation of every component the application needs.
bool InitApp()
{
if (SDL_Init(SDL_INIT_VIDEO) != 0)
{
printf("%s", SDL_GetError());
return false;
}
appWindow = SDL_CreateWindow( "Jumping Ball", screenwidth,
screenheight, SDL_WINDOW_MAXIMIZED);
if (appWindow == NULL)
{
printF("Failed to create window %s", SDL_GetError());
return false;
}
appRenderer = SDL_CreateRenderer( appWindow, NULL, 0);
if (appRenderer == NULL)
{
printf("Failed to create rendenrer %s", SDL_GetError());
return false;
}
return true;
}
The ExitApplication
function will be used to remove and deactivate every component.
void ExitApplication()
{
SDL_DestroyRenderer(appRenderer);
SDL_DestroyWindow(appWindow);
mainWindow = NULL;
appRenderer = NULL;
SDL_Quit();
}
The Resource
struct will be used to handle resource loading with the help of FindResource()
function.
typedef struct Resource
{
char *location;
};
char* FindResource(char *fileLocation)
{
char *execPath;
getBundlePath(execPath);
strcat(execPath, fileLocation);
return execPath;
}
And last but not least, I updated the CMakeLists.txt file to include some missing components.
# this is a cmake list
cmake_minimum_required(VERSION 3.27)
project("JumpingBall" LANGUAGES C VERSION 0.1 DESCRIPTION "Jumping Ball Physics Simulator")
set(SDL3_DIR "${PROJECT_SOURCE_DIR}/modules/SDL/build")
set(SDL3_ttf_DIR "${PROJECT_SOURCE_DIR}/modules/SDL_ttf/build")
set(liblocate_DIR "${PROJECT_SOURCE_DIR}/modules/cpplocate")
# Handle Submodules: https://cliutils.gitlab.io/modern-cmake/chapters/projects/submodule.html
find_package(Git QUIET)
if(GIT_FOUND AND EXISTS "${PROJECT_SOURCE_DIR}/.git")
# Update submodules as needed
option(GIT_SUBMODULE "Check submodules during build" ON)
if(GIT_SUBMODULE)
message(STATUS "Submodule update")
execute_process(COMMAND ${GIT_EXECUTABLE} submodule update --init --recursive
WORKING_DIRECTORY "./modules/"
RESULT_VARIABLE GIT_SUBMOD_RESULT)
if(NOT GIT_SUBMOD_RESULT EQUAL "0")
message(FATAL_ERROR "git submodule update --init --recursive failed with ${GIT_SUBMOD_RESULT}, please checkout submodules")
endif()
endif()
endif()
# Has SDL been downloaded?
if(NOT EXISTS "${PROJECT_SOURCE_DIR}/modules/SDL/CMakeLists.txt")
message(FATAL_ERROR "The submodules were not downloaded! GIT_SUBMODULE was turned off or failed. Please update submodules and try again.")
endif()
# Has SDL_ttf been downloaded?
if(NOT EXISTS "${PROJECT_SOURCE_DIR}/modules/SDL_ttf/CMakeLists.txt")
message(FATAL_ERROR "The submodules were not downloaded! GIT_SUBMODULE was turned off or failed. Please update submodules and try again.")
endif()
# Has cpplocate been downloaded?
if(NOT EXISTS "${PROJECT_SOURCE_DIR}/modules/cpplocate/CMakeLists.txt")
message(FATAL_ERROR "The submodules were not downloaded! GIT_SUBMODULE was turned off or failed. Please update submodules and try again.")
endif()
add_subdirectory("${PROJECT_SOURCE_DIR}/modules/SDL")
add_subdirectory("${PROJECT_SOURCE_DIR}/modules/SDL_ttf")
add_subdirectory("${PROJECT_SOURCE_DIR}/modules/cpplocate")
find_package(SDL3_ttf REQUIRED CONFIG REQUIRED COMPONENTS SDL3_ttf)
find_package(SDL3 REQUIRED CONFIG REQUIRED COMPONENTS SDL3)
find_package(liblocate REQUIRED CONFIG REQUIRED COMPONENTS liblocate)
add_executable( ${PROJECT_NAME} ./src/main.c
./src/app.h ./src/app.c ./src/resourcelocator.h
./src/resourcelocator.c ./src/text.h ./src/text.c)
target_link_libraries(${PROJECT_NAME} PRIVATE cpplocate::liblocate)
target_link_libraries(${PROJECT_NAME} PRIVATE SDL3::SDL3)
target_link_libraries(${PROJECT_NAME} PRIVATE SDL3_ttf::SDL3_ttf)
Subscribe to my newsletter
Read articles from Chris Douris directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
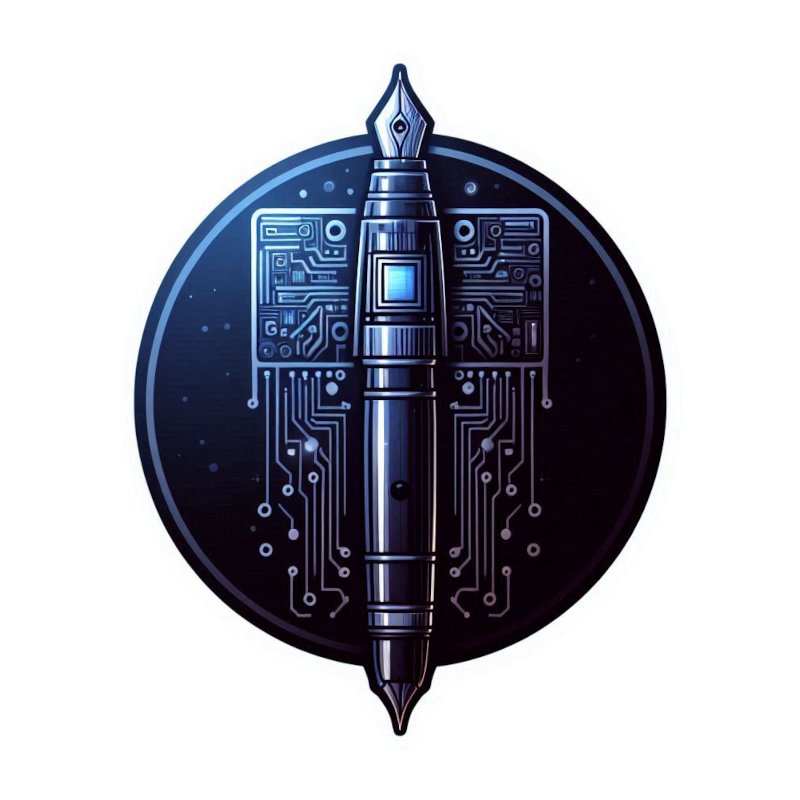
Chris Douris
Chris Douris
AKA Chris, is a software developer from Athens, Greece. He started programming with basic when he was very young. He lost interest in programming during school years but after an unsuccessful career in audio, he decided focus on what he really loves which is technology. He loves working with older languages like C and wants to start programming electronics and microcontrollers because he wants to get into embedded systems programming.