Sui Move Language - PTBs

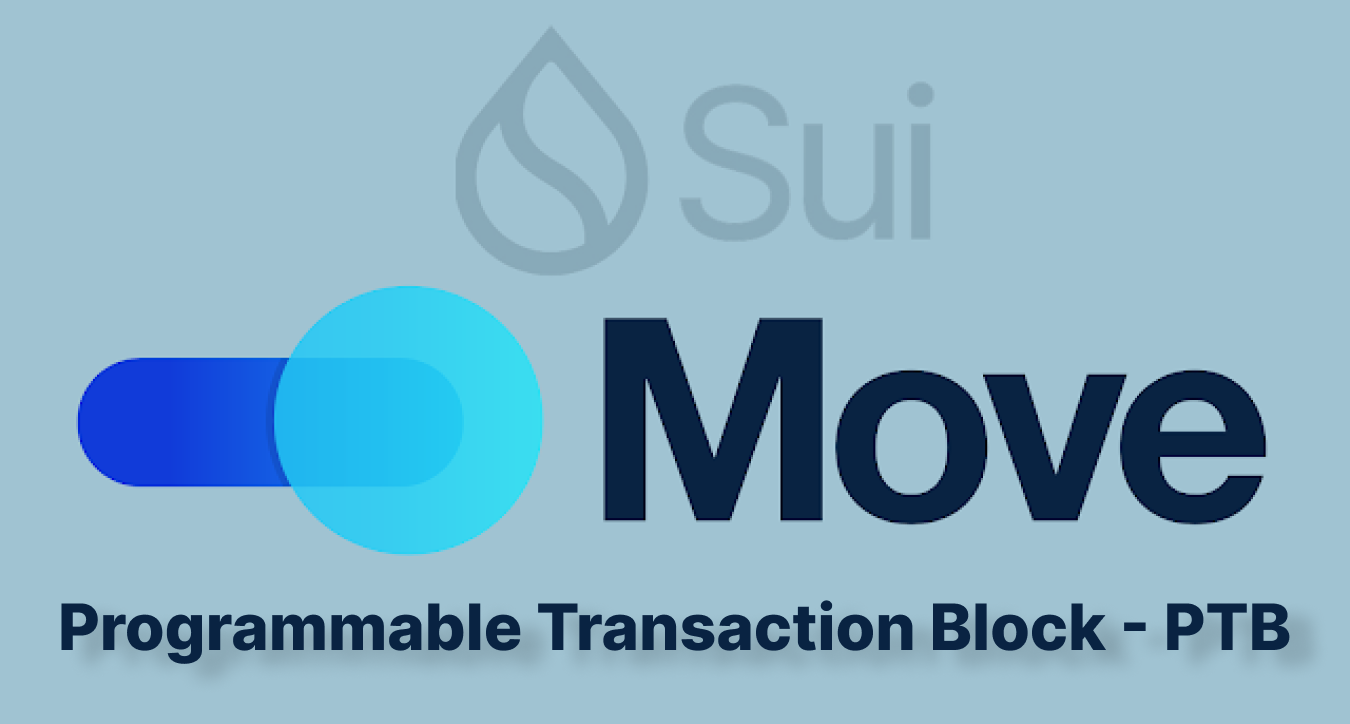
Hello Everyone.
Welcome to another day of exploring Web3 Engineering. Today we are going to look into the Sui's Programmable Transaction Blocks (PTB) and how to make use of them. So, without any further ado, let's get started.
Programmable Transaction Blocks
Programmable Transaction Blocks (PTB) in Sui blockchain are used to interact with the network. They allow users to batch multiple transactions into a single executable transaction without using any external Multi-call contracts (in EVM blockchains). By combining transactions, we can pay less gas fee and also achieve faster execution.
As mentioned, each PTB is comprised of individual transaction commands (sometimes referred to simply as transactions or commands). Each transaction command executes in order, and you can use the results from one transaction command in any subsequent transaction command. The effects, specifically object modifications or transfers, of all transaction commands in a block are applied atomically at the end of the transaction. If one transaction command fails, the entire block fails and no effects from the commands are applied.
There are two parts of a PTB that are relevant to execution semantics. Other transaction information, such as the transaction sender or the gas limit, might be referenced but are out of scope. The structure of a PTB is:
{
inputs: [Input],
commands: [Command],
}
The possible commands we can include in a PTB are as follows
TransferObjects
sends multiple (one or more) objects to a specified address.SplitCoins
splits off multiple (one or more) coins from a single coin. It can be anysui::coin::Coin<_>
object.MergeCoins
merges multiple (one or more) coins into a single coin. Anysui::coin::Coin<_>
objects can be merged, as long as they are all of the same type.MakeMoveVec
creates a vector (potentially empty) of Move values. This is used primarily to construct vectors of Move values to be used as arguments toMoveCall
.MoveCall
invokes either anentry
or apublic
Move function in a published package.Publish
creates a new package and calls theinit
function of each module in the package.Upgrade
upgrades an existing package. The upgrade is gated by thesui::package::UpgradeCap
for that package.
Comparison between EVM Txs and Sui PTBs
A typical scenario in the Ethereum ecosystem is when we are swapping tokens on any DEX. For that, the user is required to make at least two transactions. One for approving the incoming tokens to the DEX contract and then the other is make the swap transaction call. The disadvantages here are:
We are making 2 transactions i.e., we are paying base gas fee for two transactions.
If the network is busy, the approve tx may take longer for execution and in the mean time, the values we set for the swap call for example, the slippage value may not be efficient.
But in Sui, we batch the both approve (coin split, transfer) and swap transactions into one. Which means, we are only paying the base gas fee once and also since they are both executed at onetime, the efficiency of the slippage value is high.
Complexity and Efficiency
PTBs support up to 1,024 individual transactions, allowing for the creation of complex and sophisticated applications. This capability is particularly useful for applications that require multiple actions to be performed in a single transaction, such as minting multiple NFTs at once, which can be done with a single transaction at a cost comparable to minting a single NFT.
Code
Here is a typescript example on using PTBs with sui.js
package
import { TransactionBlock } from '@mysten/sui.js/transactions';
const txb = new TransactionBlock();
// create a new coin with balance 100, based on the coins used as gas payment
// you can define any balance here
const [coin] = txb.splitCoins(txb.gas, [100]);
// transfer the split coin to a specific address
txb.transferObjects([coin], '0xSomeSuiAddress');
// execute the transaction
client.signAndExecuteTransactionBlock({ signer: keypair, transactionBlock: txb });
With this, we have covered all the basic concepts required to start working with the Sui blockchain network and move language smart contracts.
Subscribe to my newsletter
Read articles from Jay Nalam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Jay Nalam
Jay Nalam
Hi, I'm Jay Nalam, a seasoned Web3 Engineer committed to advancing decentralized technologies. Specializing in EVM-based blockchains, smart contracts, and web3 protocols, I've developed NFTs, DeFi protocols, and more, pushing boundaries in the crypto realm.