Exploring OOP Fundamentals: Part 4 Breakdown
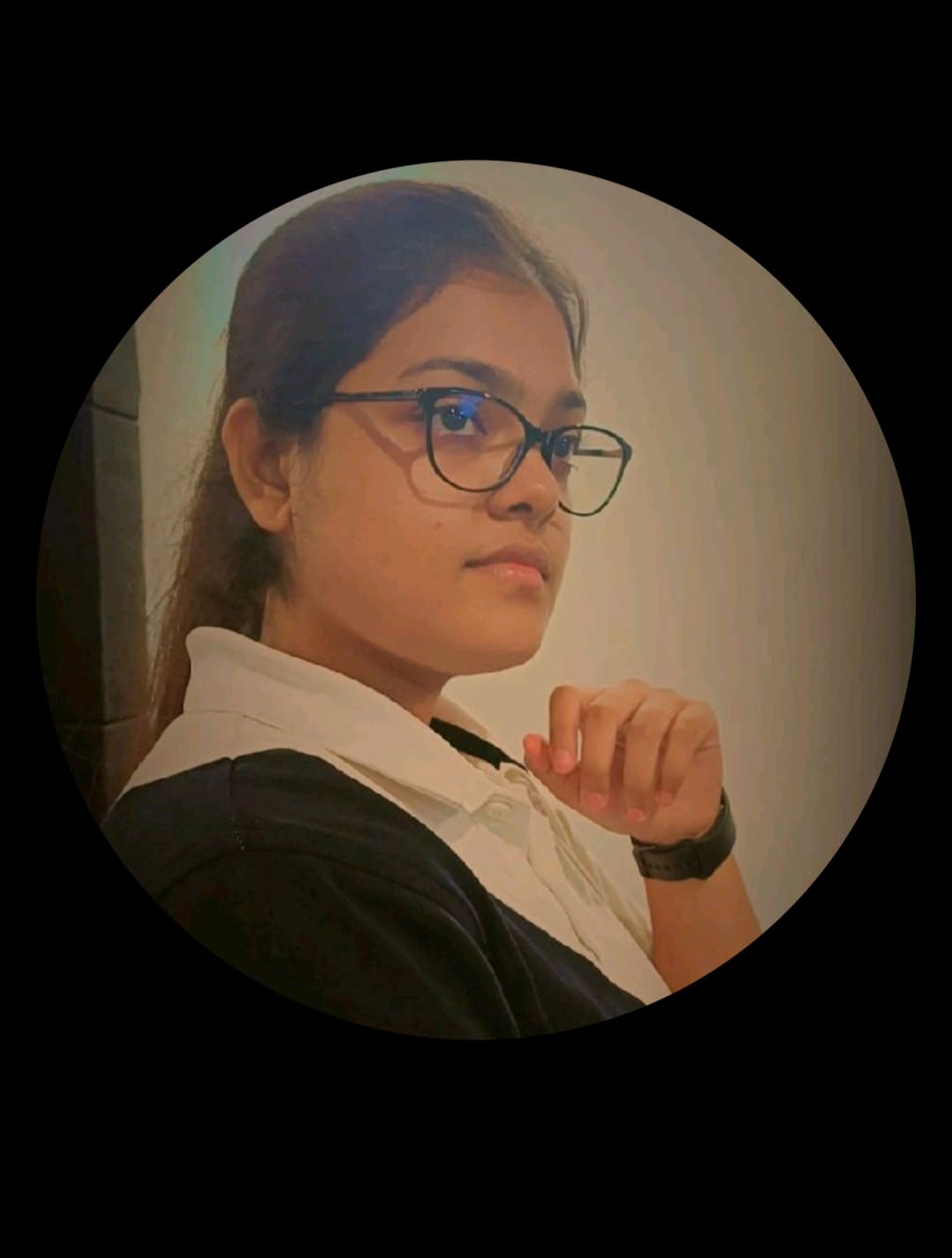
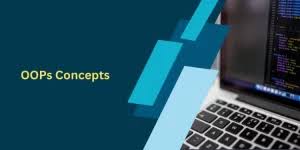
THIS POINTER
Its is a keyword which points to the objects which invokes the member function.
It is used for return object.
CODE:
class A{
int a;
public:
void setdata(int a){
this->a=a;
}
void getdata(){
cout<<"the value of a is"<<a<<endl;
}
};
int main(){
A a;
a.setdata(9);
a.getdata();
return 0;
}
STATIC KEYWORD
It is a keyword which belongs to not instance which means for accessing data member , it is not necessary to create object.
class fruit{
public:
static int count;
fruit(){
count++;
}
~fruit(){
count--;
}
};
class apple:public fruit{
public:
static int a count;
apple():fruit(){
a count++;
}
~apple(){
a count--;
}
};
class mango:public fruit{
public:
static int m count;
mango():fruit(){
m count++;
}
~mango(){
m count--;
}
};
int fruit::count=0;
int apple::a count=0;
int mango::m count=0;
int main(){
apple a1,a2,a3;
mango m1,m2,m3;
cout<<"fruits"<<fruit::count<<endl;
cout<<"apples"<<apple:: a count<<endl;
cout<<"mango"<<mango:: m count<<endl;
return 0;
}
STRUCTURE
It is a collection of different data types as similar to the class.
If access specifier is not declared explicitly then by default access specifier will be public.
SYNTAX:
struct structure_name{
}
CODE:
#include <iostream>
using namespace std;
struct Rectangle
{
int width, height;
};
int main() {
struct Rectangle rec;
rec.width=8;
rec.height=5;
cout<<"Area of Rectangle= "<<(rec.width * rec.height)<<endl;
return 0;
}
ENUMERATION
In short we can say this as "enum" keyword which consist of named constants and also improves type safety.
CODE:
enum week{mon,tue,wed,thur,fri,sat,sun};
int main(){
enum week day;
day=tue;
cout<<day;
return 0;
}
FRIEND FUNCTION
(IN HINDI we can say that compiler kisi bhi class ke private data ko, kisi doosre function ko access nahi karne deta hain)
It is not in the scope of class.
It can be invoked without the help of object.
It usually contains the objects as arguments.
It can be declared inside public or private section of class.
SYNTAX:
friend return_type function_name(arguments);
CODE:
class A{
int a;
int b;
public:
void input(){
a=s;
b=6;
}
friend float mean(A s);
};
float mean(A s);
{
return (s.a+s.b)/2;
}
int main(){
A obj;
obj.input();
cout<<mean(obj);
return 0;
}
FRIEND CLASS
It can be access both private and protected members of the class in which it has been declared as a class.
SYNTAX:
friend class class_name;
CODE:
class A{
int a=5;
friend class B;
};
class B{
public:
void display(A &x){
cout<<x.a;
}
};
int main(){
A obj1;
B obj2;
cout<<obj2.display(obj1);
return 0;
}
Subscribe to my newsletter
Read articles from Kali Baranwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
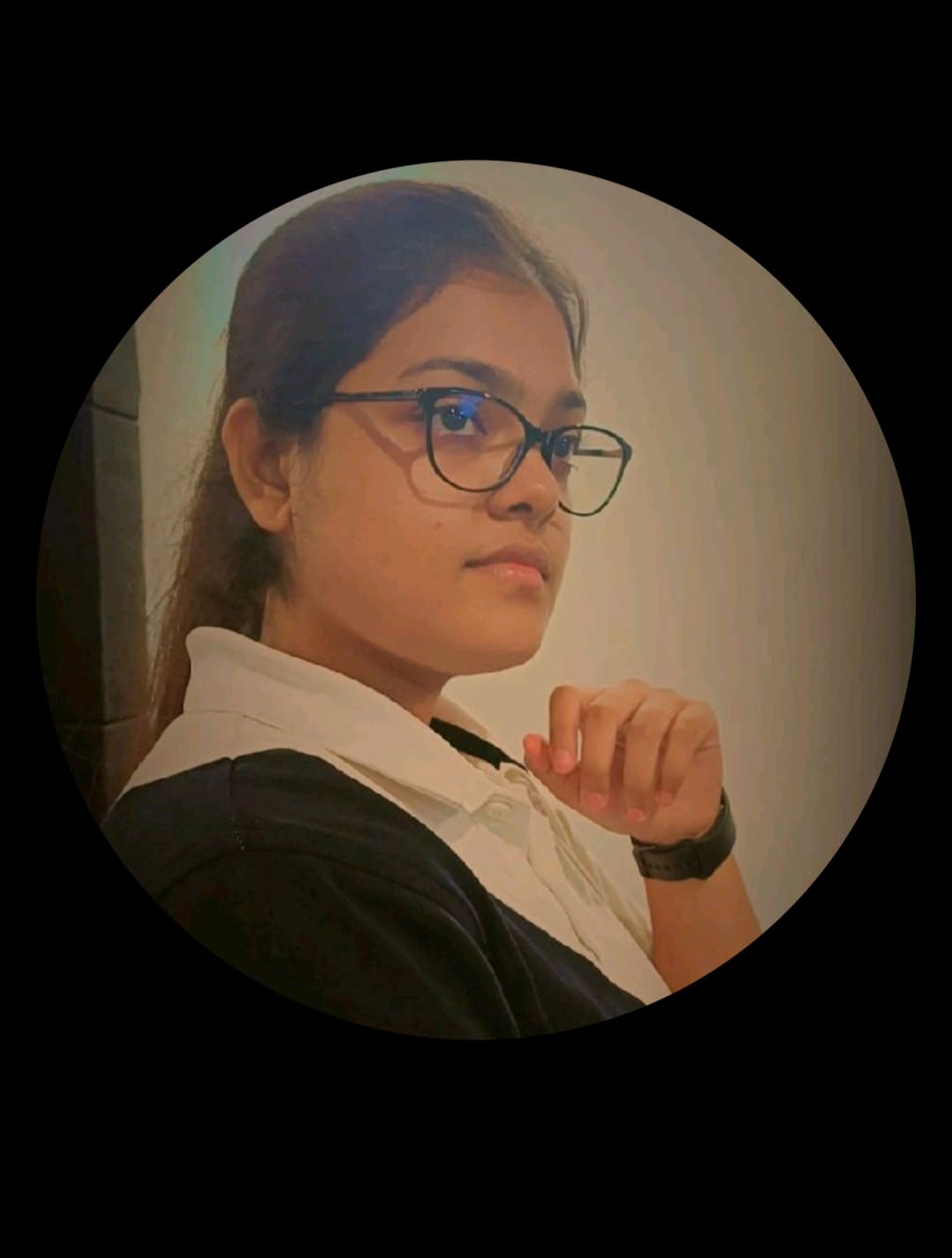
Kali Baranwal
Kali Baranwal
"๐ Hello, fellow developers! I'm Kali Baranwal , a 2nd year student . I've , exploring in another blog post also. Skilled in frontend developer, UI/UX design , C++ Join me as I share insights, of notes here on Hashnode .๐ Beyond technical skills, I'm also passionate about continuous learning and personal development. Let's connect, learn, and grow together!"