Session 1 - Intro to 🧑💻programming with Dart
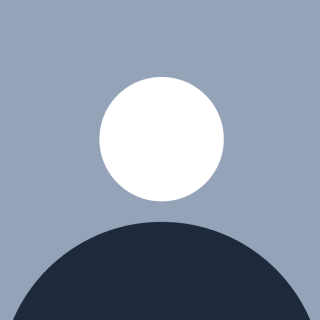
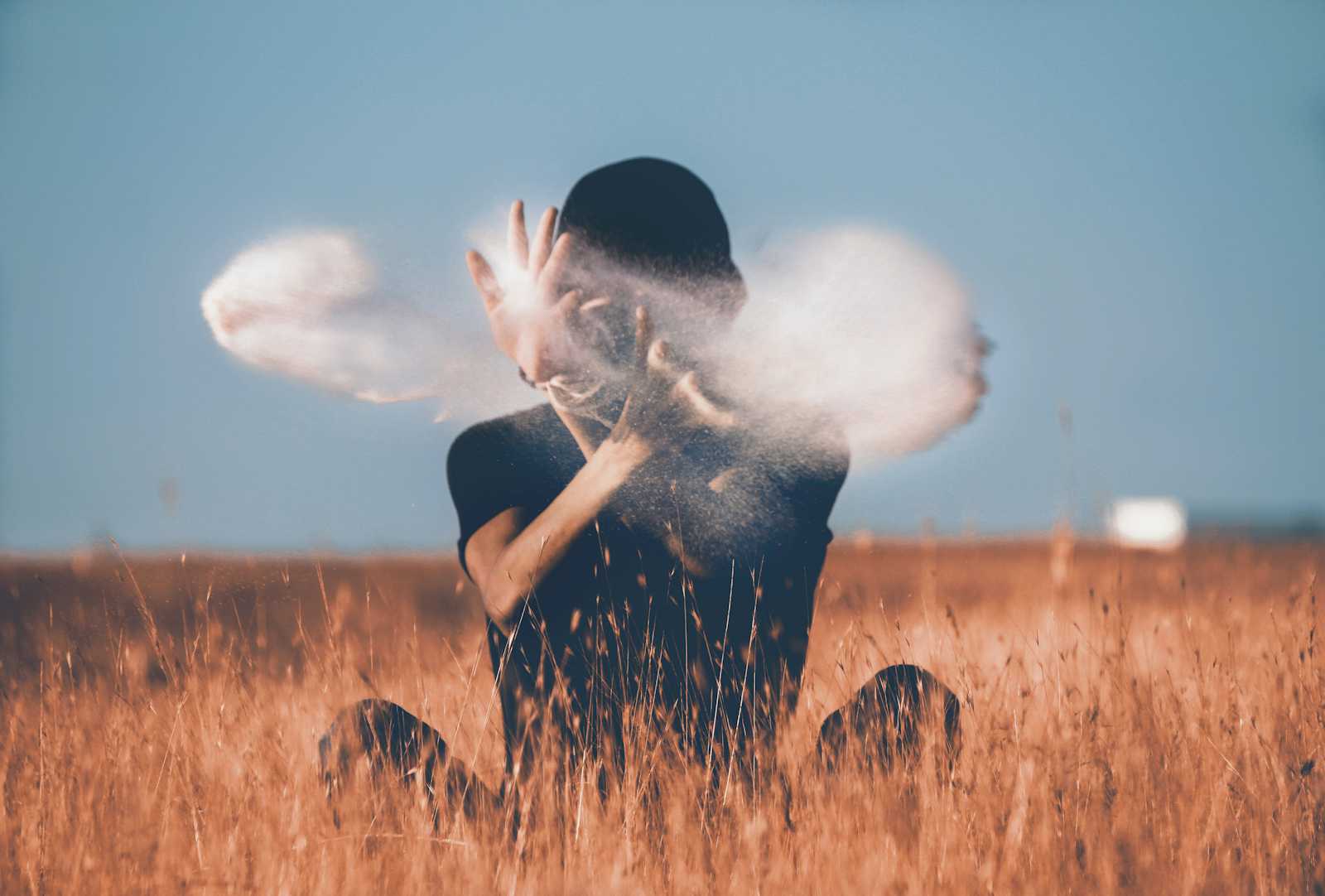
Welcome to a long journey through flutter app development, if you are reading this, I'd assume 2 things, you are a totally new to programming, and want to get into flutter or you are a pro but just want to follow along 🤓; either ways you are welcome🤗
So this session will cover the basic of programming, touching the few basic 🥜 nuts and 🔩 we use in building software📱.
By the end of the journey you will have gone from novice to publishing an app on Playstore; not just for publishing sake, but an app you can tell your friends to download because it will be worth it.
To follow along with the code in the story: head to https://dartpad.dev to test your code as you progress; make sure you type in the code into the editor instead of copying and pasting, this code of conduct is important to weather you will learn or you will run; no shortcuts. Happy learning.
Your Journey into CodeIgba
here you are entering another land. going forward, your name is now Ikem. (My Effort)
Curtain opens
Once upon a time, in the mystical land of CodeIgba, there lived a young apprentice named Ikem. Ikem dreamed of becoming a legendary coder, known far and wide for his mastery of the ancient arts of programming.
One fateful day, Ikem received a summons from the wise old sage, Ikenga, who resided atop the towering Mount Algorithm. With a sense of anticipation and excitement, Ikem set forth on a journey to seek the sage's guidance.
Chapter 1: Variables and Data Types
As Ikem journeyed through the enchanted forest, He encountered a mysterious chest guarded by a fearsome dragon. With a steady hand, Ikem approached the chest and unlocked its secrets, revealing a set of magical crystals of various colors.
"These crystals represent the essence of data," declared Ikenga, appearing before Ikem in a shimmering haze. "Each crystal holds a different type of information - numbers, words, truths, and falsehoods. With the power of variables, you can harness the magic within."
// Ikeme's Adventure: Harnessing the Power of Variables
void main() {
// Ikem discovers the magic of variables and data types.
int age = 25; // A crystal of numerical wisdom
String name = 'Ikem'; // A crystal of textual identity
bool isBrave = true; // A crystal of courage
double height = 5.7; // A crystal of precision
// Ikem's journey continues...
}
Chapter 2: Conditionals and Decision Making
As Ikem ventured deeper into the realm of CodeIgba, she encountered a fork in the road, with two paths stretching out before his - one bathed in sunlight, and the other shrouded in darkness.
"Choose wisely, young apprentice," warned Ikenga. "For your decisions shall shape your destiny."
With a furrowed brow, Ikem pondered his options, weighing the risks and rewards of each path before making his choice.
// Ikem's Adventure: Making Decisions with Conditionals
void main() {
// Ikem faces a decision point on his journey.
bool isSunshine = true; // Is the sun shining?
// Ikem must choose his path wisely.
if (isSunshine) {
print('Ikem follows the path bathed in sunlight.');
} else {
print('Ikem braves the darkness and forges ahead.');
}
// Ikem's destiny unfolds...
}
Chapter 3: Loops and Iteration
As Ikem traversed the rolling hills of CodeIgba, he encountered a mystical portal guarded by a gatekeeper with a stern gaze.
"To pass through this portal, you must prove your worth through perseverance and determination," declared the gatekeeper.
Undaunted, Ikem embarked on a quest to demonstrate his mastery of loops and iteration, proving his mettle through the power of repetition.
// Ikem's Adventure: Mastering Loops and Iteration
void main() {
// Ikem faces the challenge of the mystical portal.
int tasksCompleted = 0; // Number of tasks completed
// Ikem must prove his worth through perseverance.
while (tasksCompleted < 3) {
print('Ikem completes a task and moves closer to his goal.');
tasksCompleted++;
}
// Ikem triumphantly passes through the portal to continue his journey.
}
And so, with each step of his journey, Ikem grew in skill and knowledge, drawing ever closer to his goal of becoming a legendary coder. Armed with the wisdom of variables, the power of conditionals, and the mastery of loops, Ikem's adventure was just beginning...
Chapter 4: Switch Statement
In his journey, Ikem stumbled upon a village where the locals were troubled by a mischievous spirit that changed the weather unpredictably. Ikem took it upon himself to create a program to help the villagers predict the weather.
// Ikem's Adventure: Taming the Unpredictable with Switch Statement
void main() {
// Ikem encounters a challenge in predicting the weather.
String weather = 'sunny'; // Current weather condition
// Ikem analyzes the weather and provides a forecast.
switch (weather) {
case 'sunny':
print('The sun is shining bright. It\'s a beautiful day!');
break;
case 'cloudy':
print('The sky is covered with clouds. Expect some rain.');
break;
case 'rainy':
print('Raindrops are falling gently from the sky. It\'s a rainy day.');
break;
default:
print('The weather is unpredictable. Be prepared for anything!');
}
// Ikem's quest to predict the weather continues...
}
And so, with each step of his journey, Ikem grew in skill and knowledge, drawing ever closer to his goal of becoming a legendary coder. Armed with the wisdom of variables, the power of conditionals, the mastery of loops, and the versatility of switch statements, Ikem's adventure was just beginning...
Chapter 5: For Loop and Iteration
In his travels through the vast expanse of CodeIgba, Ikem stumbled upon a bustling marketplace where merchants traded goods from distant lands. Intrigued by the array of exotic wares on display, Ikem decided to explore the market and learn more about its inhabitants.
// Ikem's Adventure: Exploring the Marketplace with For Loop
void main() {
// Ikem arrives at the bustling marketplace.
List<String> items = ['apples', 'bananas', 'oranges', 'grapes', 'kiwis']; // Array of items for sale
// Ikem explores the market and interacts with the merchants.
for (String item in items) {
print('Ikem examines a basket of $item.');
print('Merchant: "Fresh $item for sale! Get your $item here!"');
}
// Ikem's curiosity leads his to discover new treasures in the marketplace.
}
As Ikem wandered through the market stalls, she marveled at the diversity of goods on offer and engaged in lively conversations with the merchants. With each step, she gained valuable insights into the power of the for loop, which allowed his to iterate over the array of items and interact with each merchant in turn. And thus, his journey of discovery continued, fueled by the endless possibilities that awaited his in the world of coding.
Curtain closes
Assignment
Write a dart program that list 10 of your friends nickname and tells them, hi {friend name} I am learning programming; using a loop.
Thats the end of this session
Subscribe to my newsletter
Read articles from Ucheolisah Philips Nge directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by