Topic: 8 Understanding Mixins in Dart

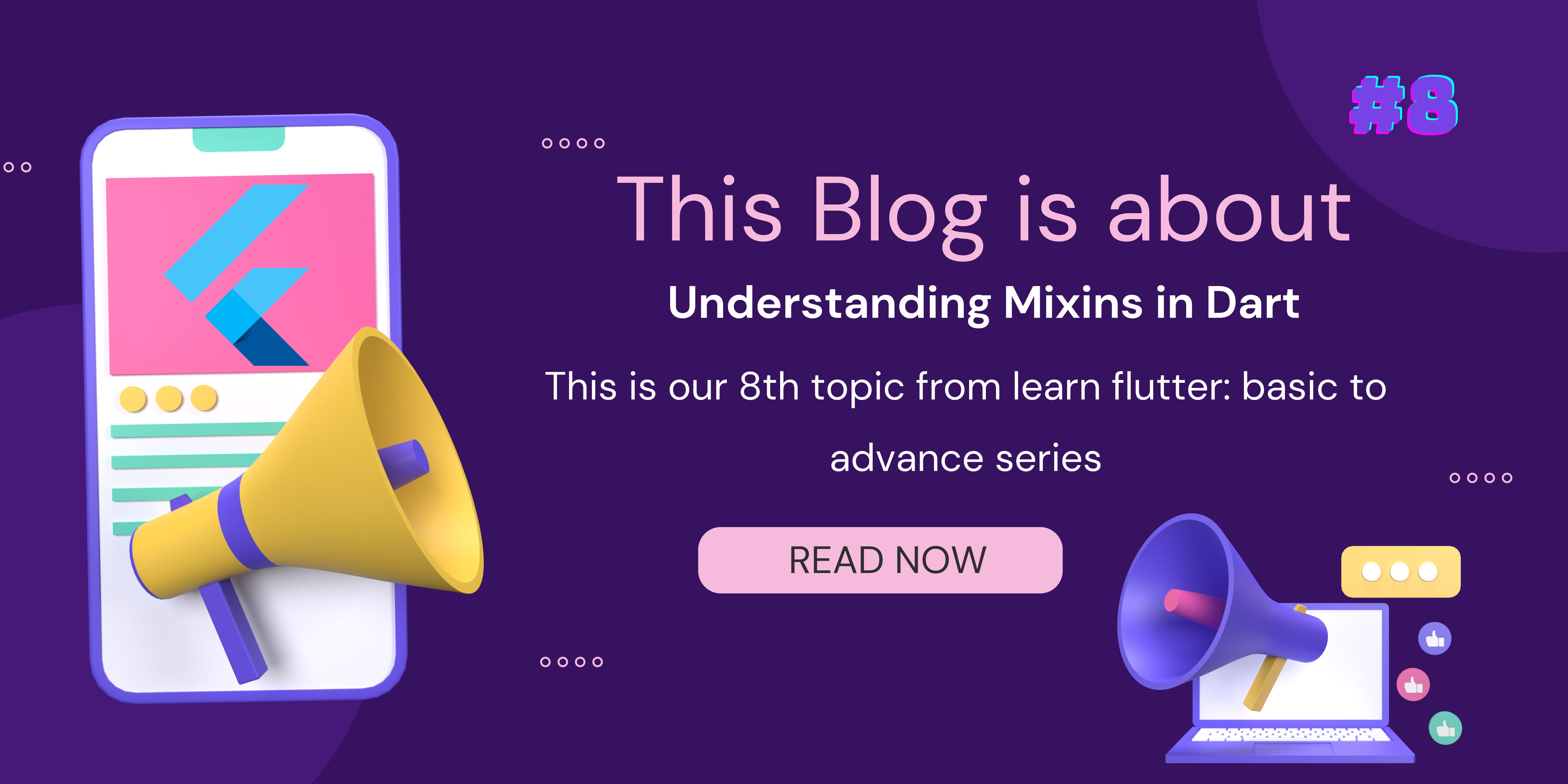
Hello developers! Today, we're going to talk about mixin classes. Mixin classes are super handy for blending different class hierarchies, giving you a more adaptable and modular way to organize your code. When you use mixin classes in your programming, you boost the reusability and manageability of your codebase. Let's dive deeper into this to get a full grasp of how mixin classes can improve your app development journey.
Mixin
In Flutter, just like in Dart, a mixin is a cool way to reuse a class's code in different class setups. It's like adding extra features to a class without fully inheriting from it. Mixins are handy for sharing functions among classes that aren't directly connected.
Imagine a mixin as a set of pre-made functions and properties that you can attach to your class. It's like expanding your toolkit without starting from scratch each time.
With mixins, you can keep your code neat and avoid repeating the same code in multiple spots. It's like having a set of tools ready to plug into your classes whenever you need them.
Basic Syntax of Mixins in Dart
In Dart, the language used by Flutter, mixins are created using the with
keyword. The syntax is as follows:
class MyClass with MyMixin {
// class implementation
}
mixin MyMixin {
// mixin implementation
}
Now Let's Understand Mixin class with one example.
import 'package:flutter/material.dart';
// Define a mixin for providing a text color
mixin TextColorMixin on StatelessWidget {
// Define a method to get text color
Color getTextColor(BuildContext context) {
// You can add any logic you want here to determine the text color
// For simplicity, let's just return a fixed color
return Colors.white;
}
}
// Create a widget that uses both mixins
class MyWidget extends StatelessWidget with BackgroundColorMixin, TextColorMixin {
@override
Widget build(BuildContext context) {
final backgroundColor = getBackgroundColor(context);
final textColor = getTextColor(context);
return Scaffold(
appBar: AppBar(
title: Text('Mixin Example'),
),
body: Container(
color: backgroundColor,
child: Center(
child: Text(
'Mixin Example',
style: TextStyle(color: textColor),
),
),
),
);
}
}
void main() {
runApp(MaterialApp(
home: MyWidget(),
));
}
Mixin Definition:
- We've created a special tool called
mixin
calledTextColorMixin
that helps you figure out the text color for a widget. This mixin is made to work with stateless widgets (StatelessWidget
) without needing any complex state management. By using this mixin, developers can quickly use the text color feature in their widgets without dealing with complicated state setups. This makes managing text color in widgets easier, speeds up development, and encourages reusing code.
- We've created a special tool called
Mixin Implementation:
- The
TextColorMixin
comes with a handy method namedgetTextColor(BuildContext context)
to help you get the color you need. When developers use this mixin, they can tweak this method in their classes to set up their own rules for deciding text colors. For example, in this case, we're simply using a fixed color (Colors.white
). This adaptability lets you manage text colors the way you want, making it easy to tailor the mixin to fit your project's needs perfectly.
- The
Widget Definition:
- We have a cool stateless widget called
MyWidget
that combines both theBackgroundColorMixin
andTextColorMixin
. By using thewith
keyword with the mixin names, our widget gets all the cool features and traits these mixins offer. This setup lets you tweak the methods in the mixins to create your own rules for choosing text colors. Right now, we're keeping it simple with a fixed color (Colors.white). This flexibility allows you to manage text colors just the way you like, making it super easy to adjust the mixin to suit your project perfectly.
- We have a cool stateless widget called
Widget Build Method:
In the
build
method ofMyWidget
, we use thegetBackgroundColor(context)
andgetTextColor(context)
methods from the mixins to get the background color and text color.These colors we get are crucial for styling different parts of the widget like the scaffold, container, and text components. This method guarantees that the widget shows the exact color scheme you want for your project. By using these methods, you can customize text colors to match your project's look, giving you more ways to personalize your project.
Main Function:
- In the
main
function, we create an instance ofMyWidget
and set it as the home widget for the Flutter application usingMaterialApp
.
- In the
Overall, this code shows how mixins are used to package and reuse common features in different widgets in a Flutter app, making the code more modular and reusable. In this example, the mixins help customize the background color and text color of the widget.
Mixins are like a helpful toolbox in Flutter development, allowing you to easily add reusable features to widgets. They cover various functionalities such as logging, animations, and state management. By using mixins, your code becomes more organized, simpler to maintain, and prepared to grow when needed.
In simple words, mixins assist you in merging common features into your widgets or classes, making your code more effective and simpler to handle overall.
Alright, devs, As we conclude this blog post, I trust that the information shared has illuminated Mixins classes in Dart for you. Stay tuned for our next discussion on the Expanded Widget and Flex Widget in the upcoming installment.
Connect with Me:
Hey there! If you enjoyed reading this blog and found it informative, why not connect with me on LinkedIn? ๐ You can also follow my Instagram page for more mobile development-related content. ๐ฒ๐จโ๐ป Letโs stay connected, share knowledge and have some fun in the exciting world of app development! ๐
Subscribe to my newsletter
Read articles from Mayursinh Parmar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mayursinh Parmar
Mayursinh Parmar
๐ฑMobile App Developer | Android & Flutter ๐๐ก Passionate about creating intuitive and engaging apps ๐ญโจ Letโs shape the future of mobile technology!