Optimizing Performance in JavaScript: Best Practices
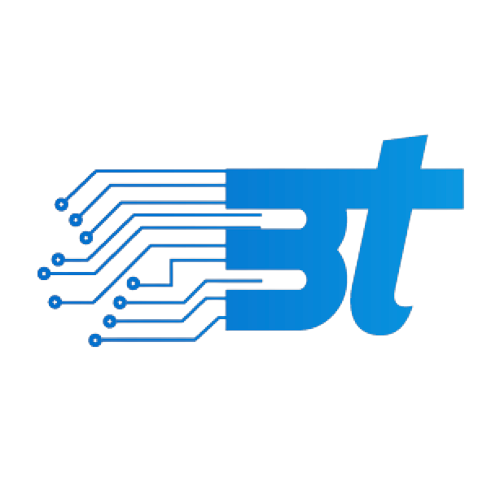
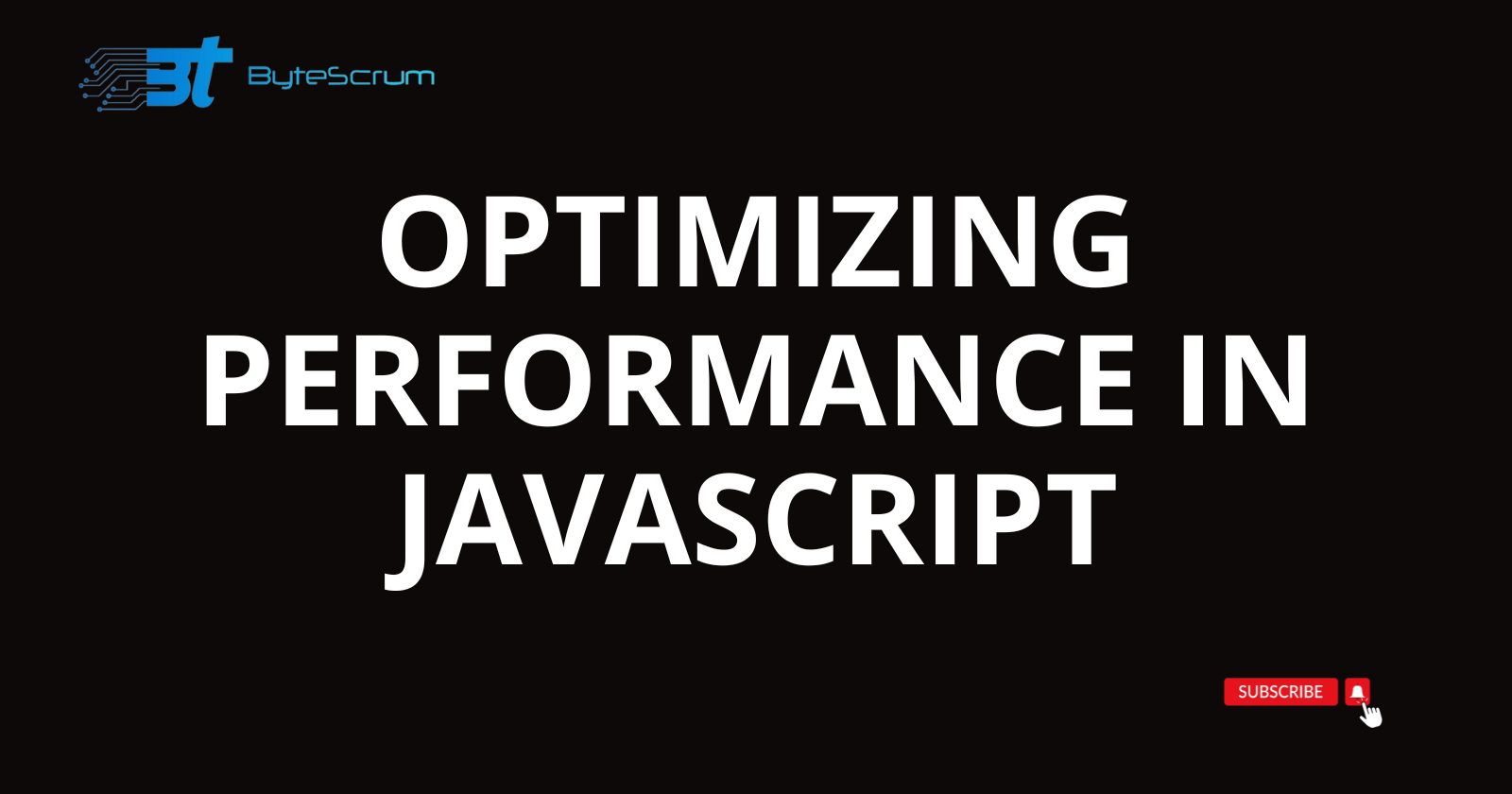
Introduction
JavaScript is a versatile and powerful language used for building interactive web applications. However, as applications grow in complexity, it's important to optimize the performance of your JavaScript code to ensure a smooth and responsive user experience. In this blog post, we will discuss some best practices for optimizing performance in JavaScript.
Minimize DOM Manipulation
DOM manipulation can be expensive, especially when performed repeatedly. Minimize the number of DOM manipulations by batching operations together and using techniques like document fragment to make changes offscreen before appending them to the DOM.
Example:
// Bad practice: Manipulating the DOM in a loop
for (let i = 0; i < 1000; i++) {
document.getElementById('container').innerHTML += '<div>' + i + '</div>';
}
// Better practice: Batch DOM manipulations
let content = '';
for (let i = 0; i < 1000; i++) {
content += '<div>' + i + '</div>';
}
document.getElementById('container').innerHTML = content;
Use Efficient Selectors
When selecting elements in the DOM, use efficient selectors like getElementById
and querySelector
instead of complex CSS selectors. Also, cache references to frequently accessed elements to avoid repeated queries.
Example:
// Bad practice: Using complex CSS selectors
let elements = document.querySelectorAll('.container > ul > li');
// Better practice: Using efficient selectors
let element = document.getElementById('container');
let items = element.getElementsByTagName('li');
Avoid Synchronous AJAX Requests
Synchronous AJAX requests can block the browser's UI thread, causing the application to become unresponsive. Use asynchronous AJAX requests instead to keep the UI responsive while data is being fetched.
Example:
// Bad practice: Synchronous AJAX request
let xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', false); // synchronous
xhr.send();
console.log(xhr.responseText);
// Better practice: Asynchronous AJAX request
let xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true); // asynchronous
xhr.onload = function() {
if (xhr.status >= 200 && xhr.status < 300) {
console.log(xhr.responseText);
}
};
xhr.send();
Optimize Loops
Avoid unnecessary work inside loops by moving calculations outside the loop whenever possible. Use techniques like loop unrolling and caching array lengths to optimize loop performance.
Example:
// Bad practice: Performing calculations inside a loop
let sum = 0;
for (let i = 0; i < 1000; i++) {
sum += i * i;
}
// Better practice: Moving calculations outside the loop
let sum = 0;
let square;
for (let i = 0; i < 1000; i++) {
square = i * i;
sum += square;
}
Debounce and Throttle Events
When handling events that can trigger frequent updates, debounce or throttle the event handlers to reduce the number of times they are executed. This can prevent performance bottlenecks, especially in scenarios like scrolling and resizing.
Example:
// Debounce example
function debounce(func, delay) {
let timeout;
return function() {
clearTimeout(timeout);
timeout = setTimeout(() => {
func.apply(this, arguments);
}, delay);
};
}
window.addEventListener('scroll', debounce(function() {
console.log('Scrolling...');
}, 250));
// Throttle example
function throttle(func, limit) {
let inThrottle;
return function() {
if (!inThrottle) {
func.apply(this, arguments);
inThrottle = true;
setTimeout(() => {
inThrottle = false;
}, limit);
}
};
}
window.addEventListener('scroll', throttle(function() {
console.log('Scrolling...');
}, 250));
Use RequestAnimationFrame for Animations
For smooth and efficient animations, use the requestAnimationFrame
method instead of setTimeout
or setInterval
. This method schedules a repaint of the window before the next repaint, ensuring smoother animations and better performance.
Example:
function animate() {
// Animation logic
requestAnimationFrame(animate);
}
// Start the animation
animate();
Optimize Memory Usage
Avoid memory leaks by properly managing object references and event listeners. Use tools like the Chrome DevTools Heap Snapshot tool to identify and fix memory leaks in your code.
Example:
// Bad practice: Adding event listeners without removing them
function onClick() {
console.log('Clicked');
}
document.getElementById('button').addEventListener('click', onClick);
// Better practice: Removing event listeners when they are no longer needed
document.getElementById('button').removeEventListener('click', onClick);
Lazy Load Resources
Load resources like images, scripts, and stylesheets only when they are needed, especially for content below the fold. Lazy loading can significantly reduce initial page load times and improve overall performance.
Example:
// Lazy loading images
let lazyImages = document.querySelectorAll('img.lazy');
lazyImages.forEach(function(img) {
img.src = img.dataset.src;
});
// Lazy loading scripts
function lazyLoadScript(url) {
let script = document.createElement('script');
script.src = url;
document.body.appendChild(script);
}
// Usage
lazyLoadScript('https://example.com/script.js');
Use Web Workers for CPU-Intensive Tasks
Offload CPU-intensive tasks to Web Workers to avoid blocking the main UI thread. Web Workers allow you to run scripts in the background, enabling parallel execution and improved performance for tasks like image processing and data computation.
Example:
// Create a new Web Worker
let worker = new Worker('worker.js');
// Send a message to the Web Worker
worker.postMessage({ data: 'some data' });
// Receive messages from the Web Worker
worker.onmessage = function(event) {
console.log('Received message:', event.data);
};
// Web Worker script (worker.js)
self.onmessage = function(event) {
console.log('Received message in Web Worker:', event.data);
// Perform CPU-intensive tasks
// Send result back to main thread
self.postMessage({ result: 'some result' });
};
Profile and Optimize
Use browser developer tools to profile your JavaScript code and identify performance bottlenecks. Once identified, optimize the critical parts of your code to improve overall performance.
Example:
// Use Chrome DevTools to profile JavaScript code
function fibonacci(n) {
if (n <= 1) {
return n;
} else {
return fibonacci(n - 1) + fibonacci(n - 2);
}
}
console.time('fibonacci');
console.log(fibonacci(30));
console.timeEnd('fibonacci');
Conclusion
Thank you for reading the blog, please subscribe for more!!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
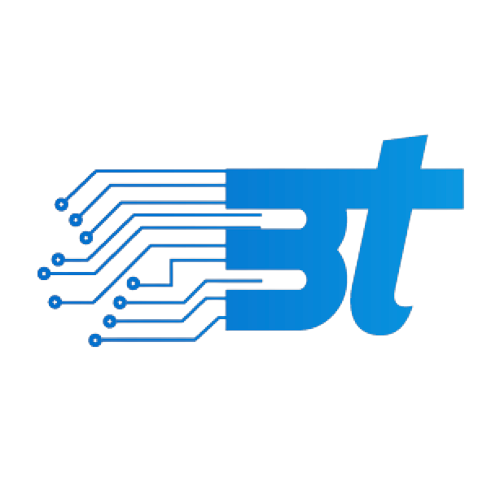
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.