Regular Expressions for Text Processing: Mastering Patterns and Manipulation

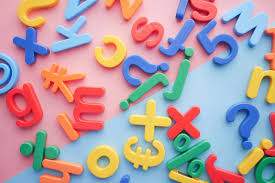
In the realm of text processing, regular expressions, often abbreviated as regex, stand as a powerful tool for manipulating and extracting specific patterns from textual data. This article delves into the world of regex, equipping intermediate Python programmers with the knowledge to unlock its potential.
Understanding the Basics:
Regular expressions are special sequences of characters that define a search pattern within a string. They act as concise yet expressive tools, enabling you to perform tasks like:
Matching: Identifying specific text segments based on predefined patterns.
Searching: Locating occurrences of a pattern within a string.
Extraction: Isolating and retrieving specific information from text.
Replacement: Substituting matched patterns with desired replacements.
Building Blocks of Regex:
The core elements of regular expressions consist of:
Literal Characters: These directly match their corresponding characters, including letters, numbers, and symbols.
Metacharacters: Special characters with specific meanings within a regex pattern. Common examples include:
.
(dot): Matches any single character.\d
: Matches any digit (0-9).\w
: Matches any alphanumeric character (a-z, A-Z, 0-9, and underscore).\s
: Matches any whitespace character (space, tab, newline).^
: Matches the beginning of a string.$
: Matches the end of a string.
Quantifiers: These specify the number of times a preceding element can be repeated.
*
: Matches zero or more repetitions.+
: Matches one or more repetitions.?
: Matches zero or one repetition.{n}
: Matches exactly n repetitions.
Putting Regex into Practice:
Let's explore some practical applications of regex in Python:
- Extracting Email Addresses:
import re
text = "Contact us at support@example.com or sales@company.org."
emails = re.findall(r"\w+@\w+\.\w+", text)
print(emails) # Output: ['support@example.com', 'sales@company.org']
This code uses the \w+
metacharacter to match alphanumeric characters, followed by @
and another pattern for the domain name, effectively extracting email addresses.
- Validating Phone Numbers:
phone_pattern = r"\d{3}-\d{3}-\d{4}"
phone_number = "My phone number is 123-456-7890."
if re.match(phone_pattern, phone_number):
print("Valid phone number format")
else:
print("Invalid phone number format")
Here, the pattern defines a specific format for phone numbers using digits and hyphens, allowing validation based on the match result.
- Cleaning Text Data:
text = "This text contains #hashtags and @mentions."
cleaned_text = re.sub(r"#\w+|@\w+", "", text)
print(cleaned_text) # Output: "This text contains and ."
This example uses the re.sub
function to replace hashtags and mentions with an empty string, effectively removing them from the text.
Beyond the Basics:
As you delve deeper into regex, you'll encounter more advanced concepts like:
Character Classes: Define groups of characters to match (e.g.,
[a-z]
for lowercase letters).Grouping and Capturing: Capture specific parts of matched patterns for further processing.
Lookarounds: Assert conditions before or after the main pattern match.
Mastering these concepts empowers you to tackle complex text manipulation tasks with greater precision and efficiency.
To sum up, regular expressions are a valuable asset for any Python programmer working with text data. By understanding their core principles and practicing with various applications, you can unlock their power for tasks ranging from data cleaning and validation to web scraping and information extraction. Remember, the more you experiment and explore, the more adept you'll become at wielding this versatile tool for your text processing needs.
Subscribe to my newsletter
Read articles from David Oshare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

David Oshare
David Oshare
I am a Python developer with 2 years of experience. I love building things with code and sharing that knowledge with others. Looking to collaborate and keep learning!