Elevating React State Management: Lifting State Up
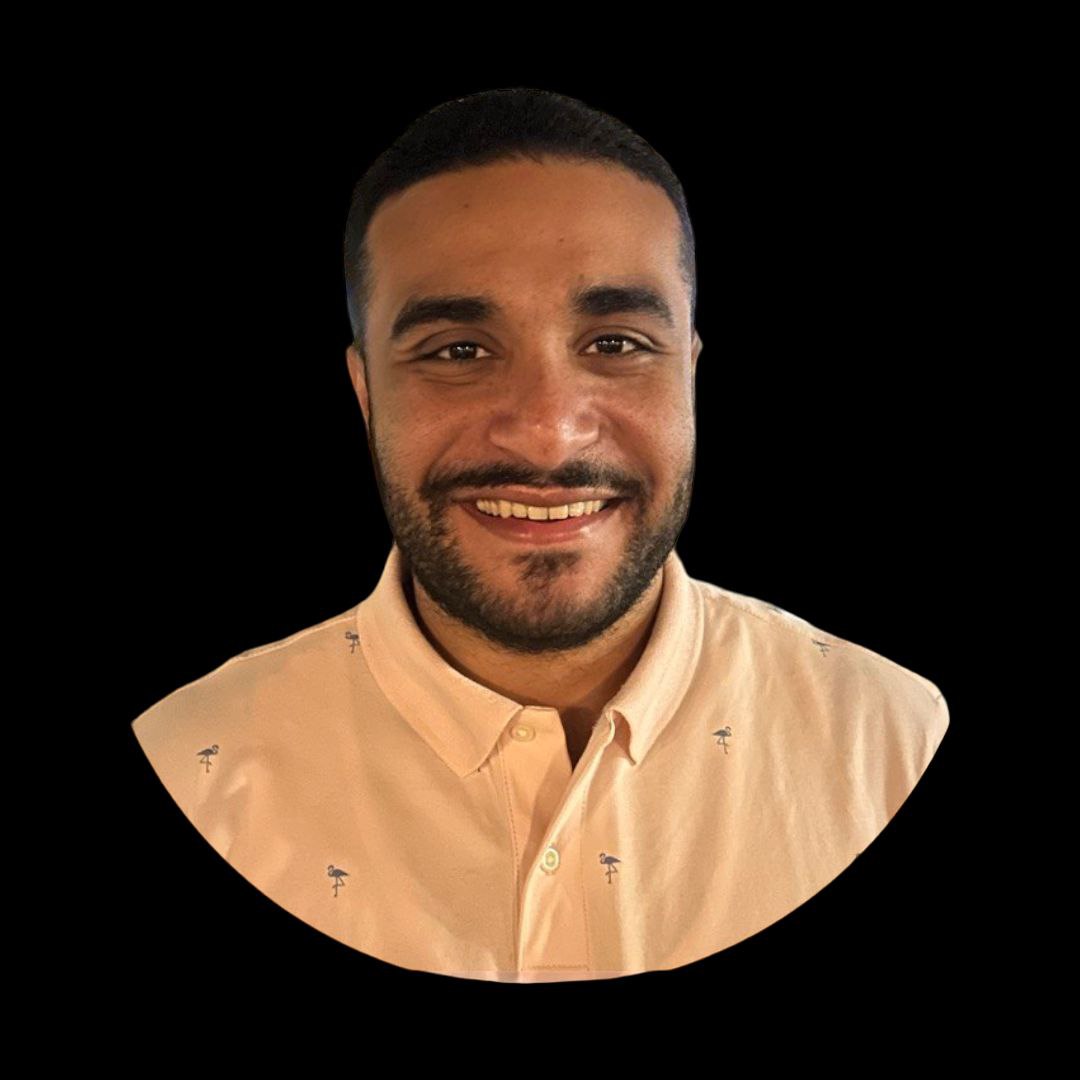
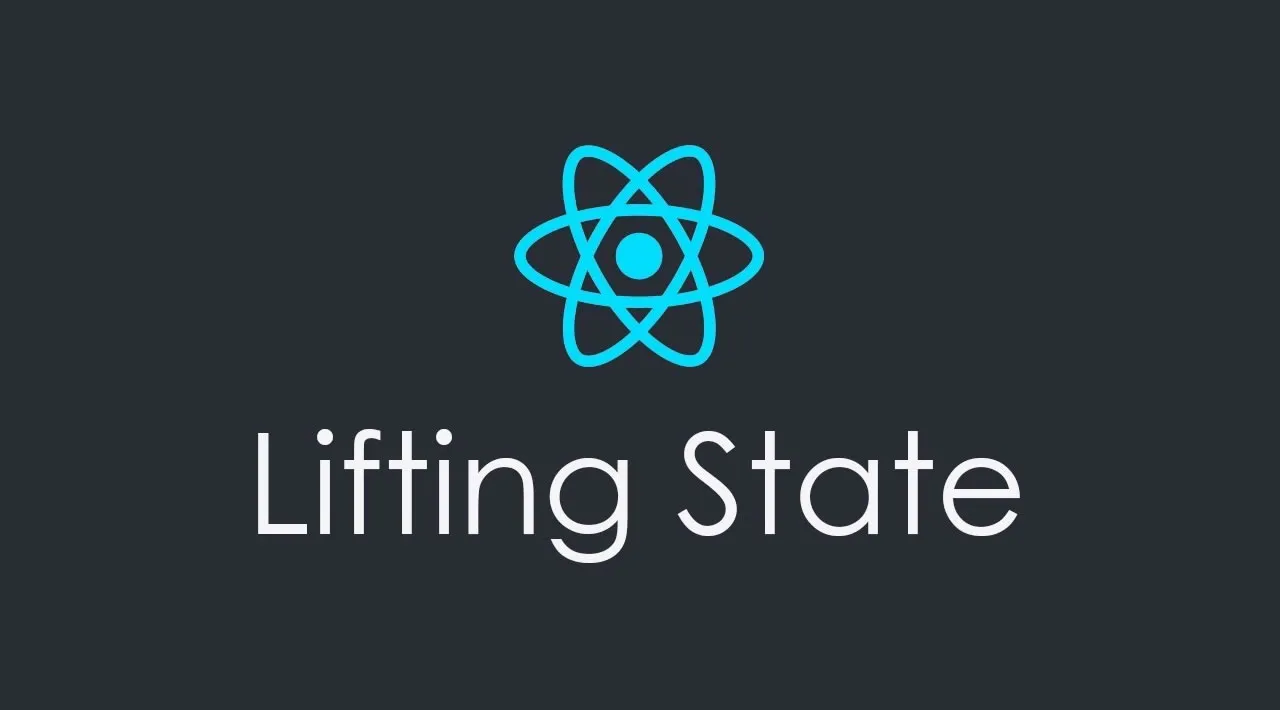
In the world of React development, managing how components communicate and share data is essential. This is especially true for forms, where data handling and state management become crucial. For new developers venturing into complex React applications, understanding when and why to "lift state up" can transform your approach to component interaction. Let's take a step-by-step journey through this concept, complete with code examples.
The Scenario: Prop Drilling in a Recipe App
Imagine we're building a recipe app. You have a search bar where users filter through recipes, and a list that displays the search results. Initially, you might pass the recipes down as props from App.js
to RecipeSearch.jsx
, and then to RecipeList.jsx
to display them.
// RecipeSearch.jsx - Before lifting state up
const RecipeSearch = ({ list, onSearch }) => {
// Initial code...
};
// RecipeList.jsx - Before lifting state up
const RecipeList = ({ recipes }) => {
// Initial code...
};
This approach is known as "prop drilling," while it might work fine for shallow component trees, it becomes less ideal as your application scales.
The Downsides of Prop Drilling
Prop drilling has a few drawbacks:
Maintenance Complexity: The more levels you pass props down, the harder your code becomes to maintain.
Component Coupling: It tightly couples components together, making them less reusable.
State Tracking: It can be challenging to track where the state should live or where it's being manipulated.
Introducing the Concept of Lifting State Up
Lifting the state up involves moving the state to a higher-level component in the hierarchy. This way, the state is managed centrally and shared only with the components that need it.
Let's see the before and after of applying this practice:
// App.js - Before lifting state up
function App() {
const [recipes, setRecipes] = useState([]);
// Imagine other functionalities related to fetching and handling recipes
return (
<div>
<RecipeSearch list={recipes} />
<RecipeList recipes={recipes} />
</div>
);
}
Now, we refactor to lift the state up:
// App.js - After lifting state up
function App() {
const [recipes, setRecipes] = useState([]);
const [filteredRecipes, setFilteredRecipes] = useState([]);
// Fetch recipes from an API when the component mounts
useEffect(() => {
// Fetching logic...
}, []);
const handleSearch = (query) => {
// Filter recipes based on the search query and update filteredRecipes
};
return (
<div>
<RecipeSearch onSearch={handleSearch} />
<RecipeList recipes={filteredRecipes} />
</div>
);
}
In the lifted version, we've added a filteredRecipes
state to App.js
that holds the filtered recipes array, reflecting the search results.
The Advantages of Lifting State Up
Here's why lifting the state up can be beneficial:
Centralized State Management: You can manage the state in one place, making it easier to modify or debug.
Improved Component Reusability: Components become less dependent on each other, enhancing their reusability.
Clear Data Flow: The data flow becomes more predictable and easier to understand.
When to Use Lifting State Up
Consider lifting the state up when:
Multiple components need access to and might modify the same data.
The data flow between components becomes too convoluted.
You foresee the need to use the same stateful logic in other parts of the application.
Implementing Search Functionality
When the user types in the search bar, we want to update the list of recipes that match the query. By lifting state up, we manage the search functionality at a higher level:
// Handling search in App.js after lifting state up
const handleSearch = (query) => {
const filtered = recipes.filter((recipe) =>
recipe.name.toLowerCase().includes(query.toLowerCase())
);
setFilteredRecipes(filtered);
};
// Updated RecipeSearch component with search handling logic
const RecipeSearch = ({ onSearch }) => {
const [searchQuery, setSearchQuery] = useState("");
const handleSearchChange = (e) => {
const query = e.target.value;
setSearchQuery(query);
onSearch(query);
};
// Search component JSX
};
This allows the RecipeSearch
component to remain focused on handling the user input while App.js
takes care of updating the list based on the current query.
Conclusion
Lifting state up in React offers a structured way to handle shared state among components, especially in complex applications. By centralizing state management, we can avoid the pitfalls of prop drilling and make our components more maintainable and reusable. As you progress in your React journey, keep this stra
Subscribe to my newsletter
Read articles from Mido Sayed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
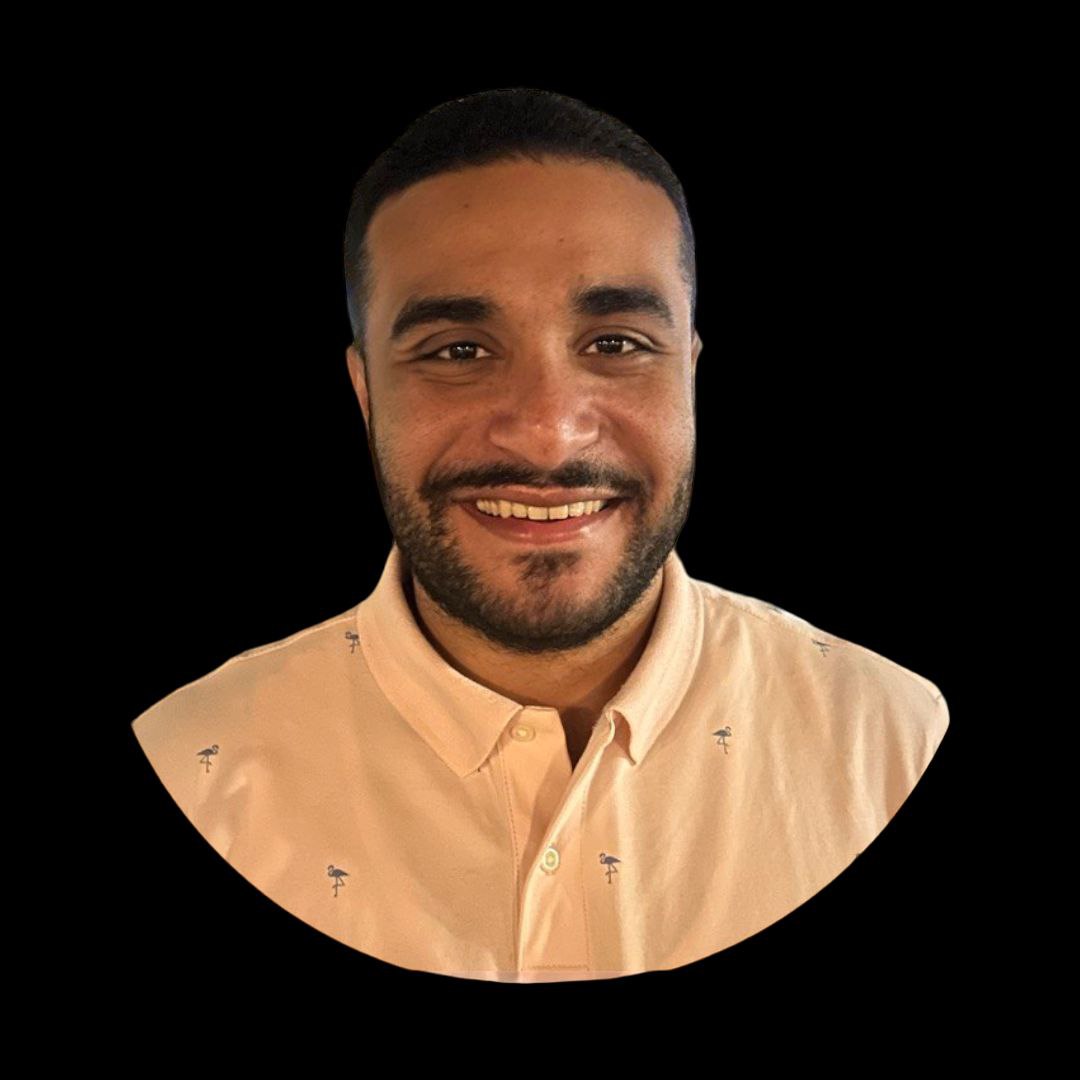
Mido Sayed
Mido Sayed
Certified AWS Solutions Architect, Software Developer, and Instructor with a passion for shaping the next-gen tech workforce. 10 years of dev experience, 6 years in curriculum development and teaching. MSc in Web Science, BSc in Computer Science. Experienced in web, data, and instructional design. Let's create tech wonders together!