Topic: 9 Understanding Expanded Widget and Flex Widget in Flutter

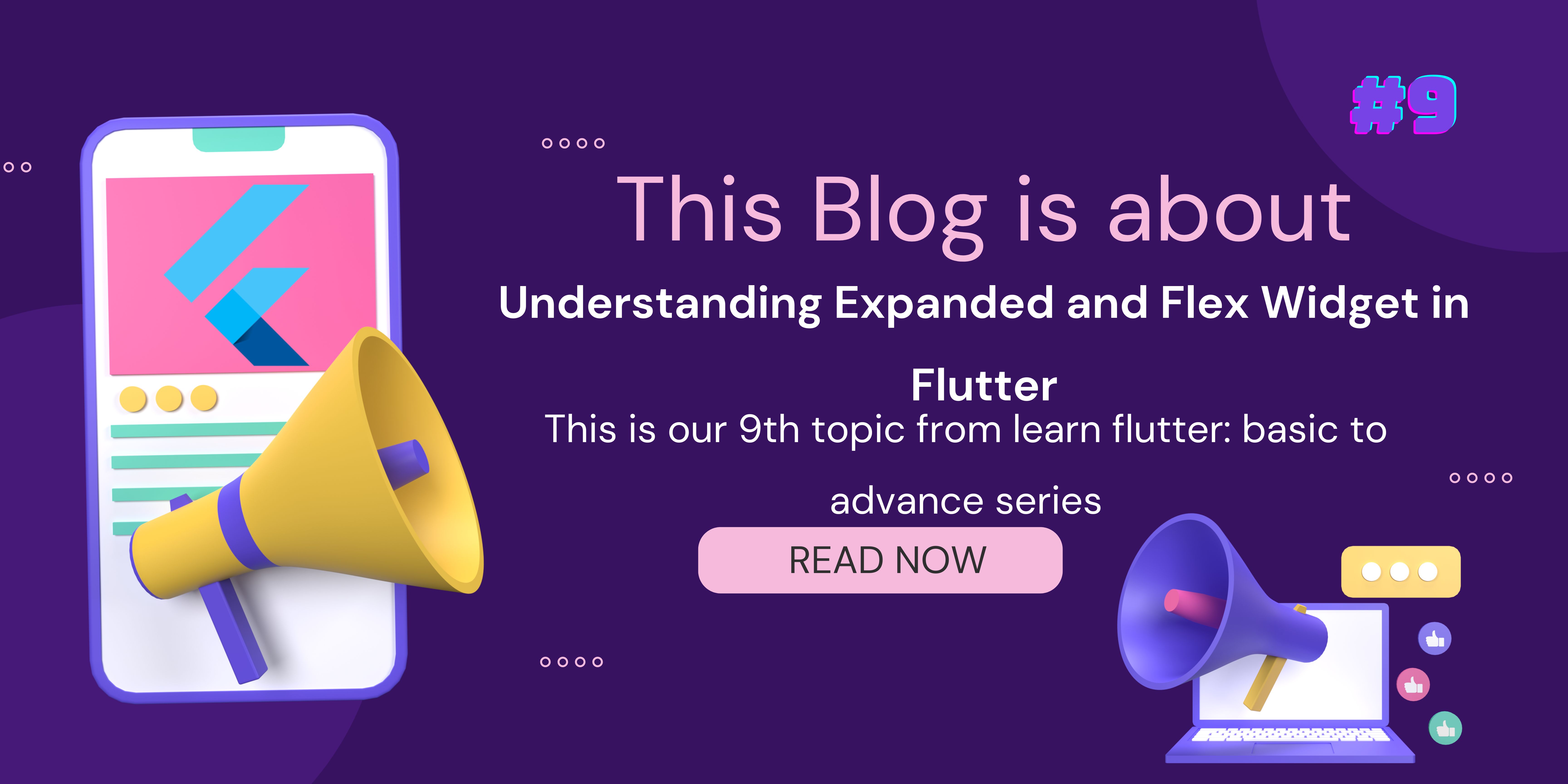
Hello devs, Today, let's dive into two important widgets in Flutter that help adjust widget sizes according to screen size. Knowing how these widgets work can really boost your Flutter development journey. We'll take a closer look at each widget to understand their importance and how they can make your user interface look great on different screen sizes.
Expanded Widget
The Expanded
widget in Flutter is like a stretchy band, making its child widget expand to fill available space. It works well with Flex
widgets, allowing for dynamic layouts where widgets can grow or shrink based on their flex factor.
For example, if you have a row of boxes and want one box to be twice the size of the others, you can use the Expanded
widget with a flex factor of 2
for the larger box and 1
for the rest. This helps Flutter distribute space proportionally among the boxes.
This widget is great for creating layouts that adapt to different screen sizes, ensuring a visually appealing user interface on various devices and orientations. It helps developers design responsive layouts that improve the user experience in their Flutter apps.
Row(
children: [
Expanded(
flex: 2,
child: Container(color: Colors.blue),
),
Expanded(
flex: 1,
child: Container(color: Colors.green),
),
Expanded(
flex: 1,
child: Container(color: Colors.red),
),
],
)
In this code, we see a Row
widget with three Expanded
widgets. The first widget, in blue, has a flex of 2, meaning it will take up twice as much space as the green and red widgets, which have a flex of 1 each.
Understanding the usage of the Expanded
widget is crucial for creating flexible and responsive layouts in Flutter applications. Let's dive into some common scenarios where the Expanded Widget proves to be beneficial.
Use Cases:
Creating responsive layouts where widgets adjust their sizes based on available screen space.
Distributing space evenly among child widgets in a
Row
orColumn
.Designing UIs where certain widgets need to take up more space than others based on importance or priority.
While using Expanded
, it's important to ensure that the parent widget has enough space to accommodate the expanded children. Otherwise, you may encounter overflow errors or unintended layout behaviors.
Now it's time to explore Flex Widget.
Flex Widget
The Flex
widget in Flutter is a useful tool for making layouts that can change based on different screen sizes. It lets you decide how space should be divided among child widgets along the main axis, giving you a lot of control and flexibility.
By using the Flex
widget, you can create layouts that adjust well to various devices, making sure your app looks good on screens of all sizes, from small phones to large tablets.
By using the Flex
widget, you can arrange widgets in either a row or a column, giving you control over how space is distributed for each widget. This control is applied along the main axis of the Flex
widget, which is determined by its direction
property. Whether you choose Axis.horizontal
for a row layout or Axis.vertical
for a column layout, the Flex
widget helps organize and size child widgets along this main axis.
Moreover, the Flex
widget's flexibility allows child widgets to have different flex factors, influenced by Flex
or Expanded
widgets. This capability lets you determine how much space each child widget takes up relative to others along the main axis, allowing you to adjust the layout to meet your design needs.
Additionally, the fit
property of the Flex
widget is essential in deciding how child widgets resize when they don't use all the available space along the main axis. Choosing FlexFit.tight
makes child widgets use all available space tightly, ensuring a snug fit within the layout. On the other hand, selecting FlexFit.loose
allows child widgets to size themselves based on their inherent dimensions, offering a more relaxed approach to space allocation within the layout.
Let's Understand Flex Widget with Example.
Flex(
direction: Axis.horizontal,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Flexible(
flex: 2,
child: Container(color: Colors.blue),
),
Flexible(
flex: 1,
child: Container(color: Colors.green),
),
Flexible(
flex: 1,
child: Container(color: Colors.red),
),
],
)
In this example, we use a Flex
widget with a row (Axis.horizontal
) direction. We specify three Flexible
widgets as children, each with a different flex factor. This controls how space is distributed among the colored containers.
Okay devs, Let's see some of the use cases for the Flex Widget.
Creating flexible layouts where widgets adjust their sizes based on available space.
Designing UIs with dynamic content where the arrangement and sizing of widgets need to adapt to different screen sizes and orientations.
In Short, the Flex
widget helps you arrange widgets in a row or column, and it lets you control how much space they take up, making your app look good on any device.
Difference Between Expanded Widget and Flex Widget
Aspect | Expanded Widget | Flex Widget |
Purpose | Used to make a single child widget of a Row , Column , or Flex expand to fill the available space along the main axis. | Used to create flexible layouts where the arrangement and sizing of child widgets can adapt to available space along the main axis of a Row , Column , or Flex . |
Child Widgets | Can only be used as the direct child of a Row , Column , or Flex widget. | Can contain multiple child widgets, each of which can have its own flex factor. |
Sizing Behavior | Expands to fill the available space along the main axis of its parent widget. | Determines how space should be distributed among its child widgets along the main axis. |
Child Flex | Can only have one child widget with the Expanded widget, which expands to fill the available space. | Can have multiple child widgets, each of which can have a flex factor to control its relative size. |
Usage | Typically used when you want a single widget to expand to fill available space. | Typically used when you want to create a flexible layout with multiple widgets, controlling their relative sizes along the main axis. |
Alright devs, This is the end of our blog. I hope this post helps you understand the Expanded Widget and Flex Widget in Flutter more easily. Alright, let's catch up on our next topic - Some of the Flutter Widgets.
Connect with Me:
Hey there! If you enjoyed reading this blog and found it informative, why not connect with me on LinkedIn? ๐ You can also follow my Instagram page for more mobile development-related content. ๐ฒ๐จโ๐ป Letโs stay connected, share knowledge and have some fun in the exciting world of app development! ๐
Subscribe to my newsletter
Read articles from Mayursinh Parmar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mayursinh Parmar
Mayursinh Parmar
๐ฑMobile App Developer | Android & Flutter ๐๐ก Passionate about creating intuitive and engaging apps ๐ญโจ Letโs shape the future of mobile technology!