Mastering the fundamentals of C language
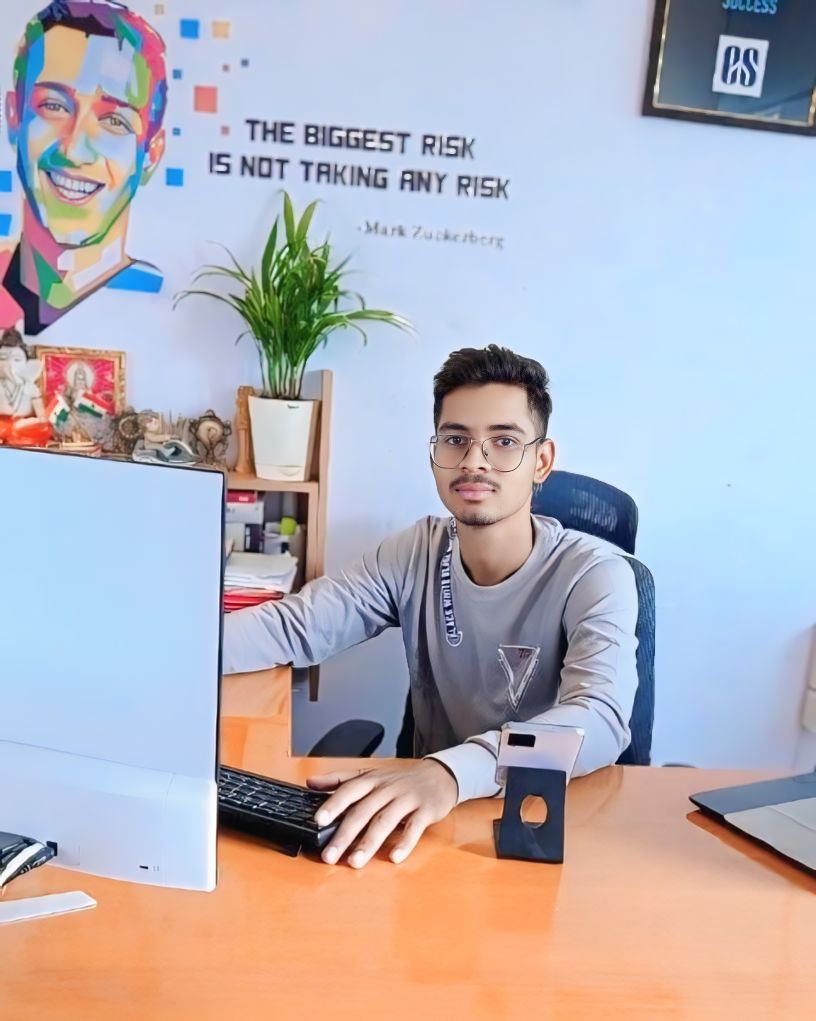
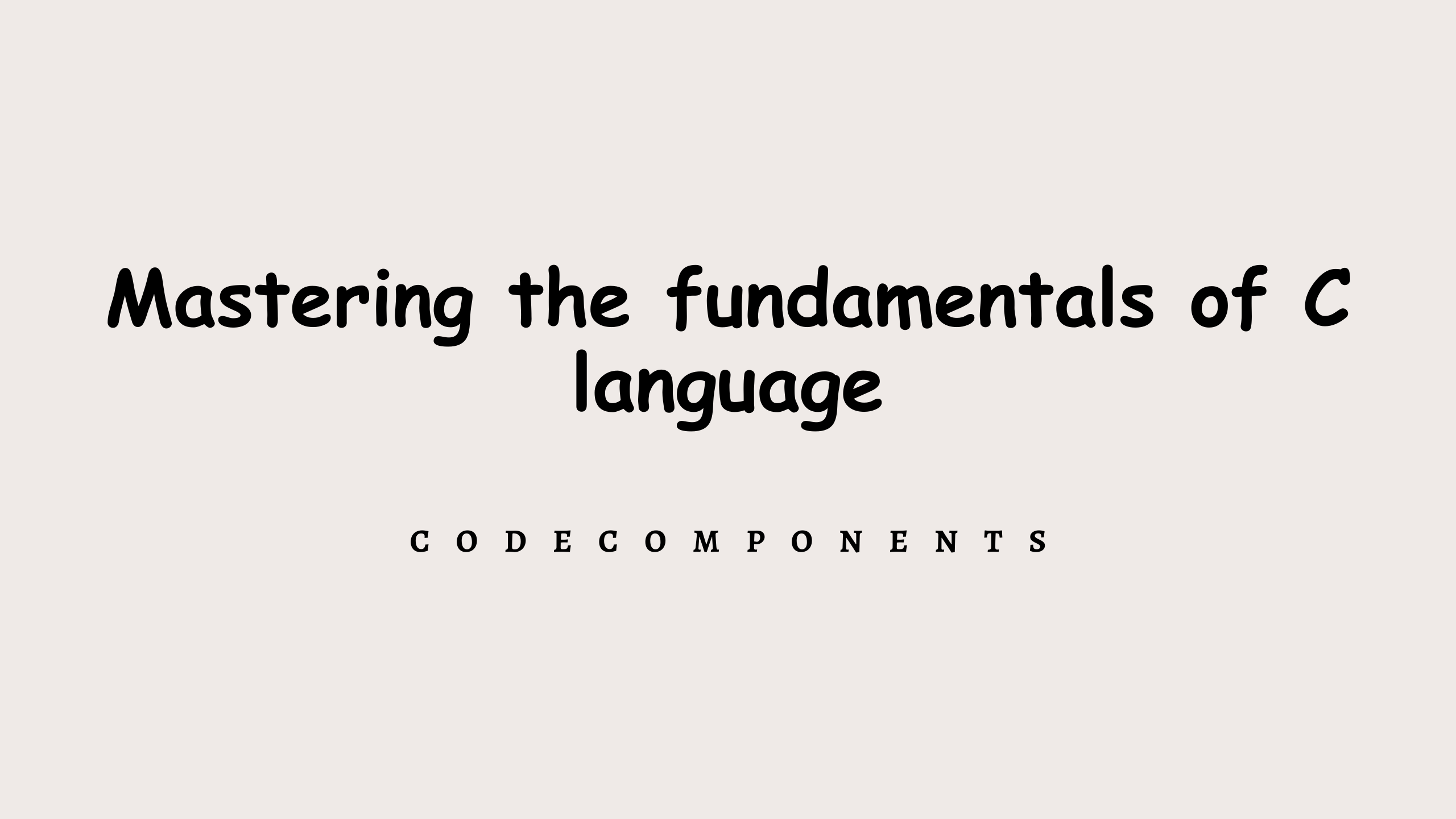
Topics Covered:
INTRODUCTION TO C
High level / middle level programming language.
Compiler based programming language.
Procedure oriented programming language [ POP’s]
General purpose language.
What is a program?
Set of instructions is called program.
What is a software?
Set of programs is called software. As per IT industry software is a digitalized and automated process.
Basically software divided into 2 types:
System software Eg: O.S, device drivers, Translators
Application software Eg: calculator, calendar, vlc media player,...
What is a language?
Generally the languages like Telugu, English, hindi, Marathi etc are used to communicate with the human beings. Hence they are called human languages. The languages like C / C++ / Java / .Net / Python / Go / R languages etc are used to develop the programs [ software ] to communicate with the machines.
Basically the languages divided into 3 types.
Machine language: Created with binary code. Eg: 11000111.
Low level / Assembly languages: Created with English like shortcuts called MNEMONICS. Eg: add, sub,...
#inclide<stdio.h>
void main(){
int a = 10, b = 20, c;
asm{
mov ax,a
mov bx,b
add ax,bx
mov c,ax
}
printf("c = %d",c);
}
c=30
- High level language: Created with simple English and easy to understand. Eg: addition, subtract,...
#include<stdio.h>
void main(){
int a=10, b=20, c=a+b;
printf("c=%d",c);
}
c=30
C is a high level language with low level features. Hence it is also called middle level language.
Clow level features used to design system software and high level features used to design application software.
What is a translator?
Basically the programmer given instructions are in English, which is called source code or source program.
But the machines are not able to understand this English code.
To convert this source code to binary code we are using the interface software, which is called translator like
Compiler
Interpreter
Assembler
Compiler and interpreter both are used to convert high level languages to binary code.
Compiler converts the total program into machine language at once, by leaving error lines.
After completion of total program show errors.
Interpreter converts line by line.
Assembler is used to convert low level programs to binary code.Assembler working style is similar to the compiler.
In C & C++ we are using compiler as a translator. Hence they are called compiler based programming languages.
In java / .net / python we are using compiler and interpreter. Hence they are called compiler based interpreted languages.
What is a programming paradigm?
Every programming language comes with particular structure with rules and regulations, which is technically called programming paradigm.
What is called monolithic programming paradigm?
Before C languages, the languages are using monolithic programming structure. In this the entire program they are designing with single program. Due to this it is very difficult to find the errors and there is no reusability.
#include<stdio.h>
main(){
printf("----------------------------------------");
printf("Good morning\n");
printf("----------------------------------------");
printf("Welcome to C\n");
printf("----------------------------------------");
printf("Thank You\n");
printf("----------------------------------------");
}
----------------------------------------
Good morning
----------------------------------------
Welcome to C
----------------------------------------
Thank You
----------------------------------------
To avoid the problems in monolithic programming they have introduced the***procedure oriented programming structure***.
In this a big program is divided into several small sub programs / sub routines / procedures / functions / modules / structures.
Hence c program is collection of procedures, it is called procedure oriented programming structure [ POP’s].
Advantages:
Modularity: Dividing big program into several small pieces according to the project requirement.
Simplicity: Easy to read / understand.
Reusability: write once, use many times.
Efficiency: Performance is high.
/*Procedur oriented programming */
#include<stdio.h>
void line(){
printf("-----------------------------------------------");
}
main(){
line();
printf("Good morning\n");
line();
printf("Welcome\n");
line();
printf("Thank You\n");
line();
}
-----------------------------------------------
Good morning
-----------------------------------------------
Welcome
-----------------------------------------------
Thank You
-----------------------------------------------
Function oriented programming structure:
C follows procedure oriented programming structure.
C++ & Python uses POP’s and OOP’s. hence they are called multi paradigm programming languages. Java / .Net follows only the OOP’s.
Disadvantages:
- In POP’s the data is public.
Object Oriented Programming Structure:
In POP’s the major problem is data is public i.e. any function can access the data from anywhere.
Data hiding
Encapsulation
Inheritance
Polymorphism – many + forms [ shapes ]
Abstraction
C++ / python having both pops and oops. Hence they are multi paradigm programming languages.
Example for pops:
#include<stdio.h>
void main(){
printf("Hi");
}
Hi
Example for oops:
#include <iostream>
class myclass {
private:
int a; // data
public:
myclass() : a(0) {} // constructor to initialize 'a'
void setA(int value) { // method to set the value of 'a'
a = value;
}
int getA() const { // method to get the value of 'a'
return a;
}
void show() { // method to display the value of 'a'
std::cout << "a=" << a << std::endl;
}
};
int main() {
myclass m1, m2, m3; // objects
m1.setA(100); // setting the value of 'a' for m1
m2.setA(200); // setting the value of 'a' for m2
m3.setA(300); // setting the value of 'a' for m3
m1.show(); // displaying the value of 'a' for m1
m2.show(); // displaying the value of 'a' for m2
m3.show(); // displaying the value of 'a' for m3
return 0;
}
Why C is a general purpose language?
Using C language we can develop the applications like
Operating system : Eg: windows, unix, linux, mac, ios, android,...
Translators : Eg: compiler, interpreter, assembler.
Device drivers : Eg: audio / video / usb drivers,....
Editors eg: notepad, wordpad, ms-word,...
Commercial applications Eg: super market / hotel / college programs
Media players Eg: vlc, mx-player, windows media player,...
Browsers Eg: google chrome, Mozilla firefox,.....
Antivirus Eg: avast, quick heal, nod,....
PC & Mobile games
Any type ofstandalone applications
Data base Eg: oracle, SQL Server, My SQL, mongodb, SQLite,...
Hence c is also called it is a multipurpose programming language.
HISTORY OF C
Basically the C language introduced in 1972 by “DENNIS RITCHIE”, A software engineer in AT & T Bell labs [ American Telephone and Telegraph ], located at Murray hills, New jersy, USA.
“DENNIS RITCHIE” adopted [ taken ] C language from B language or B compiler, developed by “KEN THOMSON”, software engineer in AT & T Bell labs.
“KEN THOMSON”, adopted B language from BCPL [ Basic Combined Programming Language ], developed by “MARTIEN RICHARDS”, one of the Assistant Professor in Cambridge University.
In 1989 ANSI [ American National Standards Institute ] released a new version of C language with the name “ANSI-C” ,which is familiar with the name “C-89”.
In 1999 ISO [ International Standard Organization ], formerly known as IOS [ International Organization for Standardization ] released a new version c language with the name “C-99”.
Basically C developed for UNIX Operating system. Nowadays we can create and execute a C program on any operating system with any processor. i.e. We can execute C program on 80386 / 80486 / 80586 / intel core i3 / i5 / i7 / i9 / amd rayzon etc. Hence C is called it is a machine independent programming language.
For example the languages like 8086 / 8088 are working only on 8086 & 8088 processors. Hence they are called machine dependent programming languages.
But C is a platform dependent programming language. i.e. the C application [ software ] designed for one operating system is not working in another operating system. For example the software designed with c language for UNIX is not working in windows operating system and this kind of languages are called platform dependent programming languages.
Because of machine independent and platform dependent c is called it is a partial portable language.due to this problem using C language only we can develop standalone applications and we can’t develop web applications.
The standalone applications installed in a single system and operated from that system only.
Eg: ms-office, calculato, antivirus, media player, oracle,....
The web application installed in a web server and used by the web client from various locations of the universe.
To develop the web applications we are using the machine independent and platform independent languages like java / .net / python. Hence they are portable languages.
FUNDAMENTALS OF C
C CHARACTER SET: Every programming language created with a particular character set and by using this character set only we can develop the programs [ software ]. C is using ASCII character set which comes with 256 characters. In this we are having 52 alphabets [ A-Z, a-z ], 10 digits [ 0-9 ], 44 operators [ +, -, *,... ], 14 separators [ , . ( ) ; : “ “ ,...] and remaining all are special characters.
ENGLISH | C LANGUAGE |
ALPHABET SET – 26 | ASCII CHAR SET - 256 |
English words | Key words - 32 |
English sentences | Instructions |
English paragraphs | Programs |
English documents | software |
ASCII - AMERICAN STANDARD CODE FOR INFORMATION INTERCHANGE
IBM CORPORATION – International business machines – American company
JAVA / .NET / PYTHON – UNICODE CHARACTER SET – 65536 CHARACTERS – UNIVERSAL code.
CHARACTERS | ASCII values |
a-z | 97-122 |
A-Z | 65-90 |
0-9 | 48-57 |
Space | 32 |
Back space | 8 |
Tab key | 9 |
Esc | 27 |
* | 42 |
+ | 43 |
C-TOKENS
The smallest individual words we are using in developing a c program are called c tokens. They are of different types.
Keywords: The system predefined / reserved words are called keywords. Each keyword is having certain meaning and a user we can’t change this meaning. C comes with 32 keywords.
Eg: auto, break, continue, const, char, case, do, double, default, else, enum, extern, float, for, goto, int, long, signed, unsigned, struct, while, union, typedef, register,.....
Constants: Fixed values are called constants. We can’t change a constant value during program execution. Constant value should be provided at the time of declaration only. i.e. further initializations not allowed.
Eg:
Numerical constants: const float pi = 3.14; const int rollno=1234;
character constants: const char name[ ]=”Ravi”; string constant const char gender=’M’; char constant.
DATA TYPES
Data type determines the type of value we are going to store in our computer. To store anything in our computer, we should have to allocate the memory. This memory allocation is depended on the data type we are using.
Data type determines the properties such as
No of bytes
Range
Type of value
In C language we are having 3 basic data types
Int – To store non-decimal numbers
Float – To store decimal numbers
Char – To stores alphabets, numbers and special char
Total data types are divided into 3 types.
Primitive data types
Derived data types
User defined data types
PRIMITIVE DATA TYPES:
Data type | Bytes | Conversion Character / format specifier | Storage Range |
int / signed int / short int | 2 | %d | -32768 to +32767 |
unsigned int | 2 | %u | 0 to 65535 |
long int | 4 | %ld | -2147483648 to 2147483647 |
unsigned long int | 4 | %lu | 0 to 4294967295 |
float | 4 | %f | 3.4 \ (10 to the power -38) to 3.4 \ (10 to the power +38) |
double | 8 | %lf | 1.7 \ (10 to the power -308) to 1.7 \ (10 to the power +308) |
long double | 10 | %lf | 3.4 * (10 to the power-4932) to 1.1 * (10 to the power +4932) |
char | 1 | %c | 1 character Signed char [-128 to +127] Unsigned char [ 0 to 255 ] |
char[10] (STRING) | 10 | %s | 9 char + 1 null char |
void [ empty data type ] | nothing |
These are the regular data types we are using in our c programs.
DERIVED DATA TYPES:
They are derived from primitive data types.
Array [ non-primitive ]
Pointer
Function
USER DEFINED DATA TYPES:
These are the data types created by the user.
structure
union
enum
Identifiers:
Identifiers are nothing but names of variables / functions / files / array / pointer etc.
Example for variables:
int a=10; // a is used to identify 10. Hence a is an identifier for 10.
Example for functions:
void sum(){
...,
}
void sub(){
...,
}
Both sum and sub are a identifier.
Example for files:
a.c, b.cpp, c.java, d.py, e.txt,....
Example for array:
Int a[100]; int array variable
Example for pointer:
int * p; int pointer var
Identifier naming Rules:
Name should have to start with alphabet or underscore.
Numbers allowed but not at first position.
No special character except underscore.
Spaces not allowed.
Names are case sensitive i.e. lower and upper are different.
Duplicate identifiers in same function not allowed.
Keywords not allowed in names.
Name may contain up to 32 characters and excess characters ignored by the compiler.
- Hence C is a case sensitive language.
Example:-
//1th points
int abc; //Allowed
int 1abc; //Not Allowed
//2nd points
int a1b3c; //Allowed
int 0a1b3c; //Not Allowed
//3rd points
int a$; //Not Allowed
int _a_; //Allowed
//4th points
int a b; //Not Allowed
//5th points
int a = 10; //Allowed
int A = 20; //Allowed
//6th points
int a = 10; //Not Allowed
int a = 20; //Not Allowed
int a = 30; //Not Allowed
//7th points
int while;
//8th points
int abcdefghijklmnopqrstuvwxyz123456; //Allowed
Installation:
We can use turbo c / c++ / dev c++ / vs code / code blocks / online compilers for c programs.
Turbo C++ installation:
Install the software like winrar or unzip or any zip software.Open any browser [ chrome ]. Type download winrar.
String:
A group of characters is called string.
Eg: char s[10]=”Hyd-1”;
It is a character array.
It is implicit [ internal ] pointer.
It is alpha-numeric. i.e. in a string we can store both alphabets, numbers and special char’s.
Note:
One byte should be left for null char [\0]. Otherwise we are getting garbage values.
String variable size never smaller than the string. Otherwise we are getting error.
We can’t copy a string using = operator. We have to use strcpy().
We can’t compare two strings using == operator. We have to use strcmp().
OPERATORS
Operator is a special symbol designed for a particular task [work]. C comes with 44 operators and 14 separators. Operator works on operands.
Based on no of operands participating in operation, the operators divided into 3 types.
Unary operator: Require one operand. Eg: a++, a--, ++a, --a, sizeof(a), +a, -a, ~a, !a,..
Binary operators: Require two operands. Eg: a+b, a>b, a!=b, a<<b,....
Ternary / conditional operator [ ?: ]: It need three operands and going to start with a condition.
Eg: conditional part ? true part : false part;
5>3 ? printf(“5 is big”) : printf(“3 is big”);
Based on operation, the operators divided into several types.
Assignment operator [ = ]: It copies [ assign ] the value on its right side into the variable on its left side. In assignment always the left side operand should be a variable i.e. constants or expressions not allowed on left side.
Eg:
a=10;
b=1.2;
c=’x’;
d=”abc”;Error because of string copy not allowed.
a=b=c=100;
10=20;Error because of 10 is a constant
c=a+b;
a+b=c;Error because of a+b return constant valule
10+20=30; Error because of 10+20 is 30, which is a constant valule
//Variable declaration and initialization. //Example: 01 : int a; //declaration float b; char c; a = 10; //initialization b = 1.2; c = 'X'; //Output : a=10, b=1.200000, c=X //----------------------------------------------------- //Example: 02 char c[10]; //declaration c = "Kishore"; // Wrong initialization //Output : Lvalue error. strcpy(c, "Kishore"); // initialization //----------------------------------------------------- //Example: 03 int a,b,c; //declaration a=b=c=100; //declaration //----------------------------------------------------- //Example: 04 100 = 100; //Output : Lvalue error. //----------------------------------------------------- //Example: 05 int a=10, b=20, c; c = a+b; //----------------------------------------------------- //Example: 06 int a=10, b=20, c=30; c = a+b; //Output : Lvalue error. a+b = c; //Output : Lvalue error.
Note: Lvalue error means left side value not changeable.
Arithmetic operators [ +, -, , %, / ]:
They are used to perform mathematical calculations.
Eg: a+b, a-b, a*b,...
% - modules: It returns the remainder.
5%2=1
2 ) 5 ( 2 is a quotient (/)
4
1 is a remainder (%)
2%5=2
5 ) 2 ( 0 is a quotient (/)
2 is a remainder (%)
Note: If the divisor is bigger than dividend then the dividend is the answer.
5%2 => 1 2%5 => 2 9%25 => 9 5.0%2.0 => Error Note: In C & C++ we can’t do floating modulus with % operator. For this we have to use fmod() available in <math.h> 5.0%2.0 => Error 5%2.0 => Error 5.0%2 => Error fmod(5.0,2.0) => 1.000000 fmod(5.0,2) => 1.000000 fmod(5,2.0) => 1.000000 fmod(5,2) => 1.000000 101%10 => 1 94%10 => 4 3%10 => 3 Note: Any no%10 gives last digit. 5%-2 => 1 -5%2 => -1 -5%-2 => -1 Note: In modules if the numerator is negative then result also negative.
Division [ / ]: It always returns the quotient.
5/2=2 [ int/int=int ]
5.0/2=2.500000
Note: int/int gives always int. Any one float or both are floats then result is float.
//------------------------------------------------ 5/2.0=2.500000 5.0/2.0=2.500000 (float)5/2=2.500000 /* explicit type casting */ 5/(float)2=2.50000 int a=1.2; /* implicit type casting */ printf(a); => 1 float b=10; printf(b); => 10.000000 (float)(5/2)=2.000000 //------------------------------------------------ int a = 1.2; //Implicit type casting float b = 10; printf("%d, %d", a, b); //Output : 1, 10.000000 //------------------------------------------------ printf("%d",5/2); //Output : 2 printf("%f",5.0/2); //Output : 2.500000 printf("%f",5/2.0); //Output : 2.500000 printf("%f",5.0/2.0); //Output : 2.500000 printf("%f",(float)5/2); //Output : 2.500000 printf("%f",(float)5/2); //Output : 2.000000 //------------------------------------------------ printf("%d",123/10); //Output : 12 printf("%d",12/10); //Output : 1 printf("%d",1/10); //Output : 0 Note: Any no/10 removes the last digit. Any no%10 gives the last digit.
#include<stdio.h> int main(){ int n = 123; printf("%d reverse id %d",n,n%10); //Out:- 3 n = n/10 printf("%d%d",n%10,n/10); //Out:- 21 }
Note: In division any one operand is negative then result also negative. If both are negative then result is positive.
Relational operators [ == ( comparison), <, >, <=,=, != (not equal) ]:
They are used to check the given expression or condition is true or false. If condition true always the answer is 1. If condition false always answer is 0.
printf("%d", 9==9) //Out:- 1 printf("%d", 9==8) //Out:- 0 printf("%d", 'a' == 'a') //Out:- 1 printf("a addr=%u, a addr=%u", "a","a") //Out:- a addr=438, a addr=440 printf("%d", "a"=="a") //Out:-0 printf("%d","a"=='a') //Out:- 0 printf("%d",0.90 == .9) //Out:- 1 printf("%d",9 == 9.0) //Out:- 1 printf("%d",'9'==9) //Out:- 0 printf("%d",'a'>'A') //Out:- 1 printf("%d",'a'-32 == 'A') //Out:- 1 printf("%d",'A'<='A') //Out:- 1 printf("%d",1!=1.0) //Out:- 0 printf("%d",5+3/2==4) //Out:- 0 printf("%d",(5+3)/2==4) //Out:- 1 printf("%d",5-3+2==4) //Out:- 1 printf("%d",5/2*2==4) //Out:- 1 printf("%d",5/2*2==4) //Out:- 1 printf("%d",5*2/2==5) //Out:- 1 printf("%d",2+3*4+5==45) //Out:- 0 printf("%d",2+3*4+5==25) //Out:- 0 printf("%d",2+3*4+5==19) //Out:- 1 printf("%d",(2+3)*(4+5)==45) //Out:- 1 printf("%d",'X' > 'x') //Out:- 0 printf("%d","X">'x') //Out:- 1
int main(){ int a,b,c; a = printf("I"); b = printf("Dont"); c = printf("know"); printf("%d%d%d",a,b,c); } //Out:- IdontKnow144 int main(){ int a; a = printf("I Dont know") == printf("I Dont care"); printf("%d",a); } //Out:- I Dont knowI Dont care1 int main(){ int a; a = printf("I Dont know") != printf("I Dont care about you"); printf("%d",a); } //Out:- I Dont knowI Dont care about you1 int main(){ int a; a = printf("I Dont know\n") != printf("I Dont care about you"); printf("%d",a); } /*Out:- I Dont know I Dont care about you0 */ int main(){ int a,b,c; a = printf("I Dont know Rahul\n"); b = a+printf("I Dont care Rahul\n"); c = a+b+printf("Bye Bye Rahul\n"); printf("%d%d%d",a,b,c); } /*Out:- I Dont know Rahul I Dont care Rahul Bye Bye Rahul 183668 */
Logical operators: [ &&, ||, != ] :
&& => logical and
|| => logical
! => not
&&, || are used to combine two or more expressions into a single expression.
! used for negation i.e. true becomes false and false become true.
Note: In c other than 0 anything is 1 i.e. true.
Truth tables: (1 - true, 0 - false)
!true = false
!false = true
printf("%d",3>=3,8!=1); //Out:- 1
printf("%d, %d",3>=3,8!=1); //Out:- 1, 0
printf("%d",3>=3 && 8!=8); //Out:- 0
printf("%d",3>=3 || 8!=8); //Out:- 1
printf("%d",!(3>3)); //Out:- 1
printf("%d",1==1 && 2>=2 && 3!=3); //Out:- 0
printf("%d",1==1 || 2>=2 && 3!=3); //Out:- 1
printf("%d",(1 == 1 || 2>=2) && 3!=3); //Out:- 0
printf("%d",3>2>1); //Out:- 0
printf("%d",3>2>0); //Out:- 1
printf("%d",5 && -5); //Out:- 1
printf("%d",1.1 && 2.2); //Out:- 1
printf("%d",0 || -1); //Out:- 1
printf("%d",0 && 'a'); //Out:- 0
printf("%d",!0 && !2); //Out:- 0
Note: && have more priority than || operator.
#include<stdio.h>
#include<consio.h>
void main(){
clrscr();
a = printf("I Like ") && printf("You\n");
b = printf("") || printf("I like You dood\n");
c = printf("") && printf("I Love You\n");
d = printf("I Like Mritunjay ") || printf("I like Mritunjay Kumar\n");
printf("%d%d%d%d",a,b,c,d);
getch();
}
Note:
In && operation when left one false, right one not checked
In || operation when left one true, right one not checked
Out:-
I Like You
I like You dood
I Love You
#include<stdio.h>
#include<consio.h>
void main(){
int a,b,c,d;
clrscr();
a = "" || printf("hi");
b = printf("Hello");
printf("a=%d, b=%d",a,b);
gatch();
}
Out:-
Helloa=1, b=5;
Increment / decrement / modify operators / [ ++ / -- ]:
They always used to increment or decrement a variable value by 1.
Eg:
Int a=4;
Int b=3;
a++; //i.e.:- a=a+1 => a=5
b--; //ie.:- b=b-1; => b=2
#include<stdio.h>
#include<conio.h>
void main(){
int a = 4, b =3;
clrscr();
a++;
b--;
printf("a=%d, b=%d",a,b);
getch();
}
//Out:- a=5, b=2
#include<stdio.h>
#include<conio.h>
void main(){
int a = 4, b =3;
clrscr();
a+++;
b----;
printf("a=%d, b=%d",a,b);
getch();
}
//Out:- Error
#include<stdio.h>
#include<conio.h>
void main(){
int a = 4, b;
clrscr();
b=a++;
printf("a=%d, b=%d\n",a,b);
a= 4;
b=++a;
printf("a=%d, b=%d",a,b);
getch();
}
/*Out:-
a=5, b=4
a=5, b=5
*/
Note: Until assigning to any other variable pre and post operations are same.
Operator precedence
(PRIORITY OF OPERATORS)
()
+, - , ! (sign operators, unary operators)
++, -- (pre increment & decrement)
*, / , %
+, - (Binary)
\= =, !=
&&
||
? : (ternary operator)
\=
++, -- ( Post increment & decrement )
, (comma)
In Devc++:
#include<stdio.h>
#include<conio.h>
main()
{
int a=2, b;
b = a++ + a++ + a++;
printf("a=%d, b=%d\n",a,b);
a=2;
b = ++a + ++a + ++a;
printf("a=%d, b=%d", a, b);
getch();
}
/*Out:-
a=5, b=9
a=5, b=13
*/
Compound assignment operator/shorthand operators:
Here we are combining assignment operator with other operators as follows.
+=, -=, *=, %=, /=, <<=, >>=,~=,..
Example.:-
//1 th
Int a=10, b=25;
Float c=5;
a+=2; //i.e. a=a+2 10+2 12
b*=4; //i.e. b=b*4; 25*4 = 100
c/=2; //i.e. c=c/2 5/2 = 2.500000
/*Out:-
a=12, b=100, c=500000
*/
//2nd
int a = 4;
a+=2+3; //a=a+2+3
printf("%d",a);//a=9
() and, separators:
Find the next / previous multiple of given no without using if or ternary operator.
Address operators:
& -> Address of current variable.
* -> pointer – address of another variable.
1tb hd
4 bits = 1 nibble
8 bits = 2 nibbles / 1 byte
1024 bytes = 1kb
1024kb = 1 mb
1024mb = 1 gb
1024gb = 1tb
sizeof( ) operator: It return the no of bytes taken by a variable / data type / value.
Bitwise operators:
They work on bits [ 0,1 ]
It is one of the low level feature of c.
They work only on int data.
C & C++ working under 16 bit compiler. Hence bitwise operators are taken from 2 the power 0 to 2 the power 15 .
When starting bit is 0 the given no is +Ve. If the starting bit is 1 given no is –Ve. Hence the starting bit is also called sign bit.
Eg:
00011111 => +ve
11111000 => -ve
We have to take only the 1’s positions, not 0’s. because of any no * 0 is 0.
C comes with 6 type of bitwise operators.
Bitwise and - &
Bitwise or - |
XOR – Exclusive or - ^
Compliment op - ~
Left shift op - <<
Right shift op - >>
Bitwise and - &
25 && 15 = 1
25 & 15 = 9
BITWISE OPERATORS
Bitwise operator’s works on bits. Turbo-c is a 16 bit compiler. Due to this bitwise operations are limited to 16 bits only [20
to 215]. Bitwise operators operate integer type values only. We have to calculate only the on bits [ 1 ].
When the first bit[Sign bit] is 1 then the number is Negative and it is 0 then the number is positive. They are very much used in system software development.
Note: Bitwise operator is low level feature.
C-Language supports following bitwise operators.
& -Bitwise and
| - Bitwise or
^ - XOR ==> Exclusive OR
~ - Compliment operator
<< - Left shift operator
>> -Right shift operator
& - Bitwise and: In this both bits are 1’s then result bit is
Otherwise result bit is 0.
Subscribe to my newsletter
Read articles from Mritunjay Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
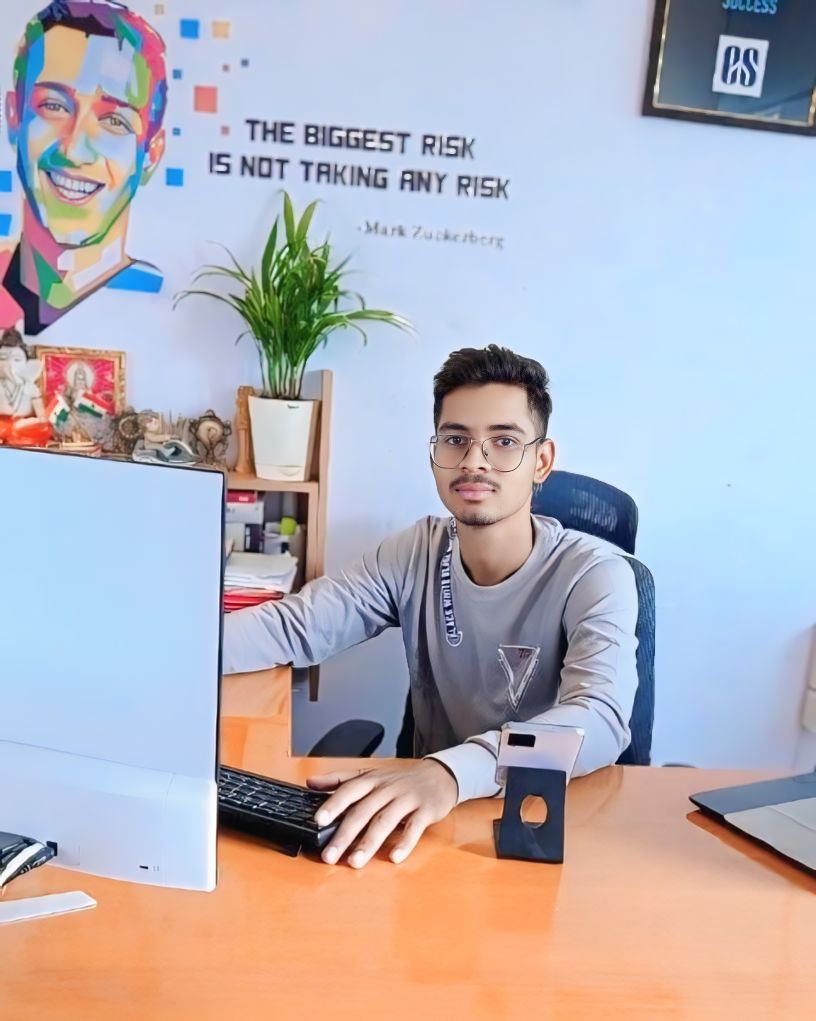