Conquering Your Routes: A Guide to Laravel Routing
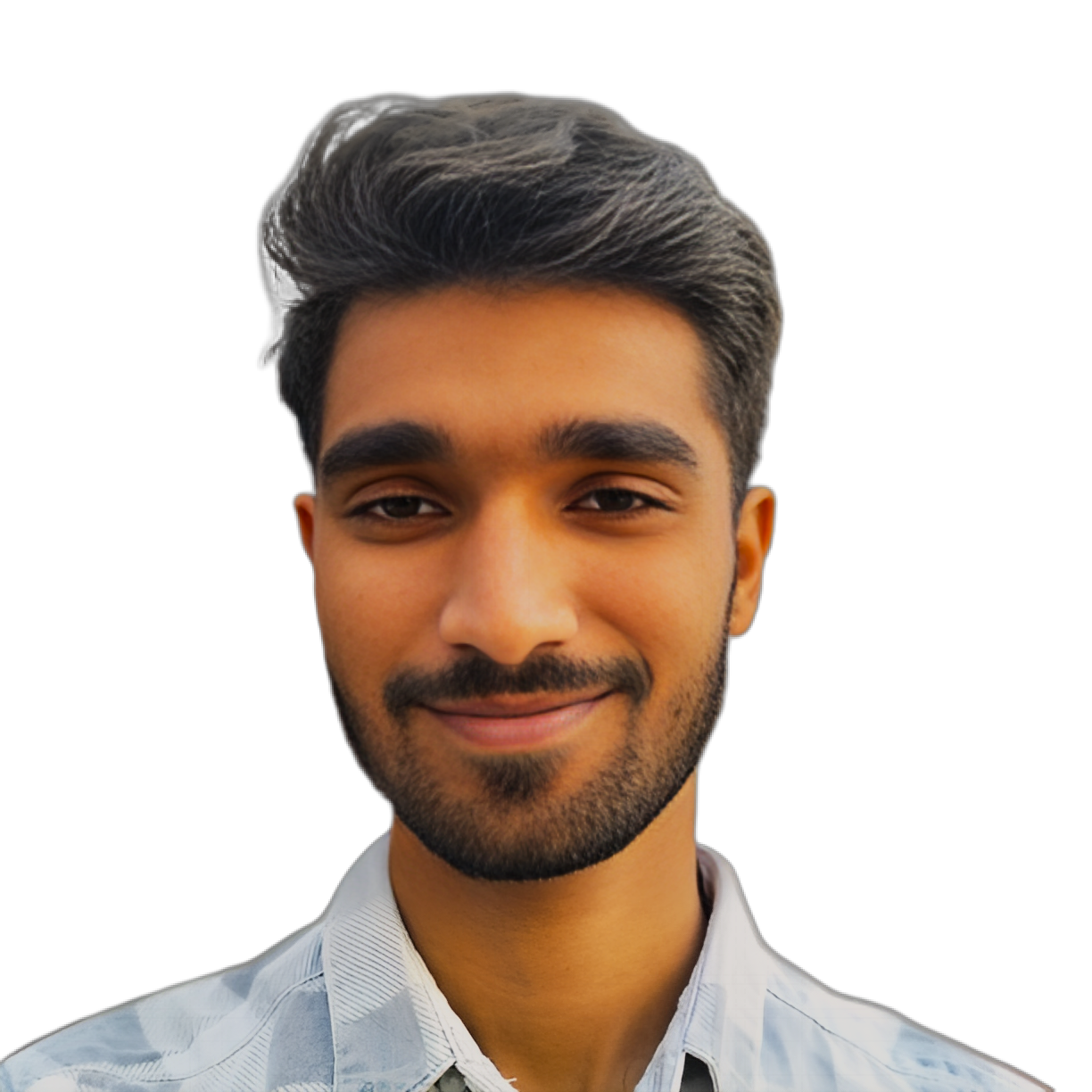
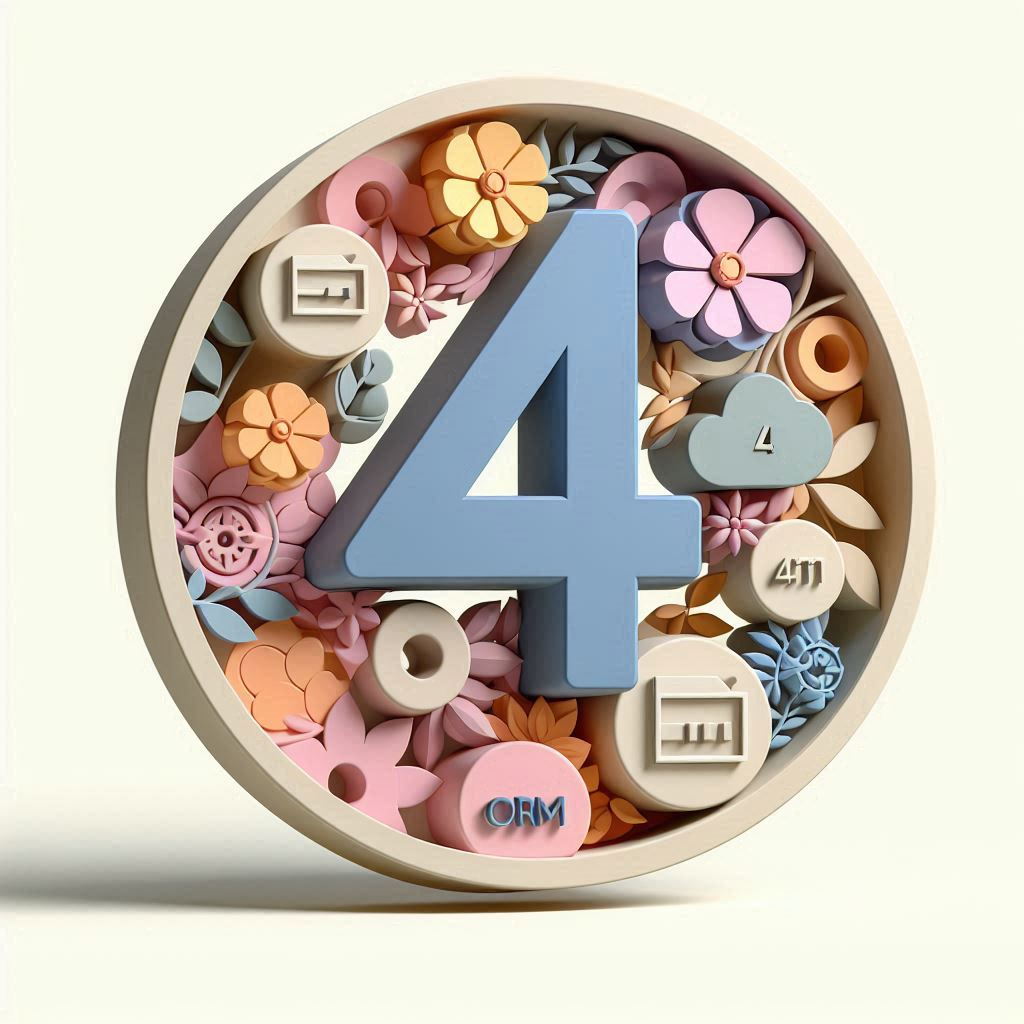
Hey there, web dev warriors! Today, we're embarking on a quest to master the highways and byways of your Laravel application – the realm of routing. Routing is the invisible mapkeeper behind the scenes, directing users to the exact functionalities they need based on the URL they enter. Let's break down how to define clear paths, handle user requests smoothly, and ultimately build a well-organized and maintainable codebase.
The Power of Routes: Your Navigation Compass
Imagine your Laravel application as a sprawling city. Users navigate through it using addresses (URLs), and routes act like clever signs that point them in the right direction. They connect incoming requests (like a user hitting a specific URL in their browser) to the corresponding controller actions that handle those requests.
Here's the beauty: routes aren't one-size-fits-all. We can specify different HTTP methods (like GET for grabbing data, POST for submitting forms, PUT for updating data, and more) to handle different user interactions. Additionally, routes can be like adaptable chameleons, capturing dynamic parts of the URL using route parameters. Let's see this in action with some code:
PHP
Route::get('/users/{id}', function($id) {
// Logic to retrieve user data based on $id
return view('user.profile', compact('user'));
});
In this example, the route defined with Route::get
listens for GET requests to the URL pattern /users/{id}
. The curly braces around {id}
indicate that this part of the URL is dynamic and can capture a specific value. When a user visits /users/123
, the $id
variable in the function will contain the value 123, allowing us to retrieve the user with that ID and display their profile information on the user.profile
view. Pretty cool, right?
Building a Route Empire: Organization is Key
As your Laravel application grows, keeping your routes organized becomes essential. Think of it like a bustling metropolis – if all the streets were unnamed and buildings scattered randomly, navigating it would be a nightmare! Here are some ways to maintain order in your route kingdom:
Grouping Routes with Prefixes: Imagine dividing your city into districts. We can group routes with a common purpose using prefixes. For example, all routes related to admin functionalities might be grouped under a
/admin
prefix. This makes your code more readable and easier to manage.Named Routes: Your Easy Navigation Shortcuts: Just like giving landmarks catchy names helps with directions, Laravel allows you to assign unique names to routes. This makes referring to them throughout your code much simpler. Imagine needing to redirect a user after a successful form submission – a named route lets you do that with just a few lines of code.
Resourceful Routing: The CRUD Crusader: This is where things get exciting for those who love efficiency. Laravel offers resourceful routing, a fantastic feature that automatically generates routes for common CRUD (Create, Read, Update, Delete) operations on your models. It's like having a pre-built highway system for your data – you just define your models, and Laravel takes care of the routing magic for basic CRUD actions, saving you tons of time and effort.
Why Developers Love Laravel Routing (and You Will Too!)
Let's be honest, developers are a picky bunch. But there's a reason Laravel's routing system has earned their loyalty. Here's what makes it stand out:
Clean and Maintainable Codebase: Expressive and well-defined routes contribute to a codebase that's easy to understand and navigate, even for developers joining the project later.
Expressive and Readable Routes: The syntax for defining routes in Laravel is clear and concise, making your code more self-documenting. Less time deciphering cryptic code, more time building awesome features!
Strong Community and Ecosystem: The Laravel community is a vibrant and supportive bunch. If you ever get stuck with a routing challenge, there's a wealth of resources available online, from official documentation to forums filled with helpful developers.
By mastering Laravel routing, you'll be well on your way to building well-structured and user-friendly web applications. Remember, the journey to becoming a Laravel routing expert starts with a single step. So, dive into the documentation, experiment with different route types, and don't hesitate to leverage the power of the Laravel community. Happy conquering!
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
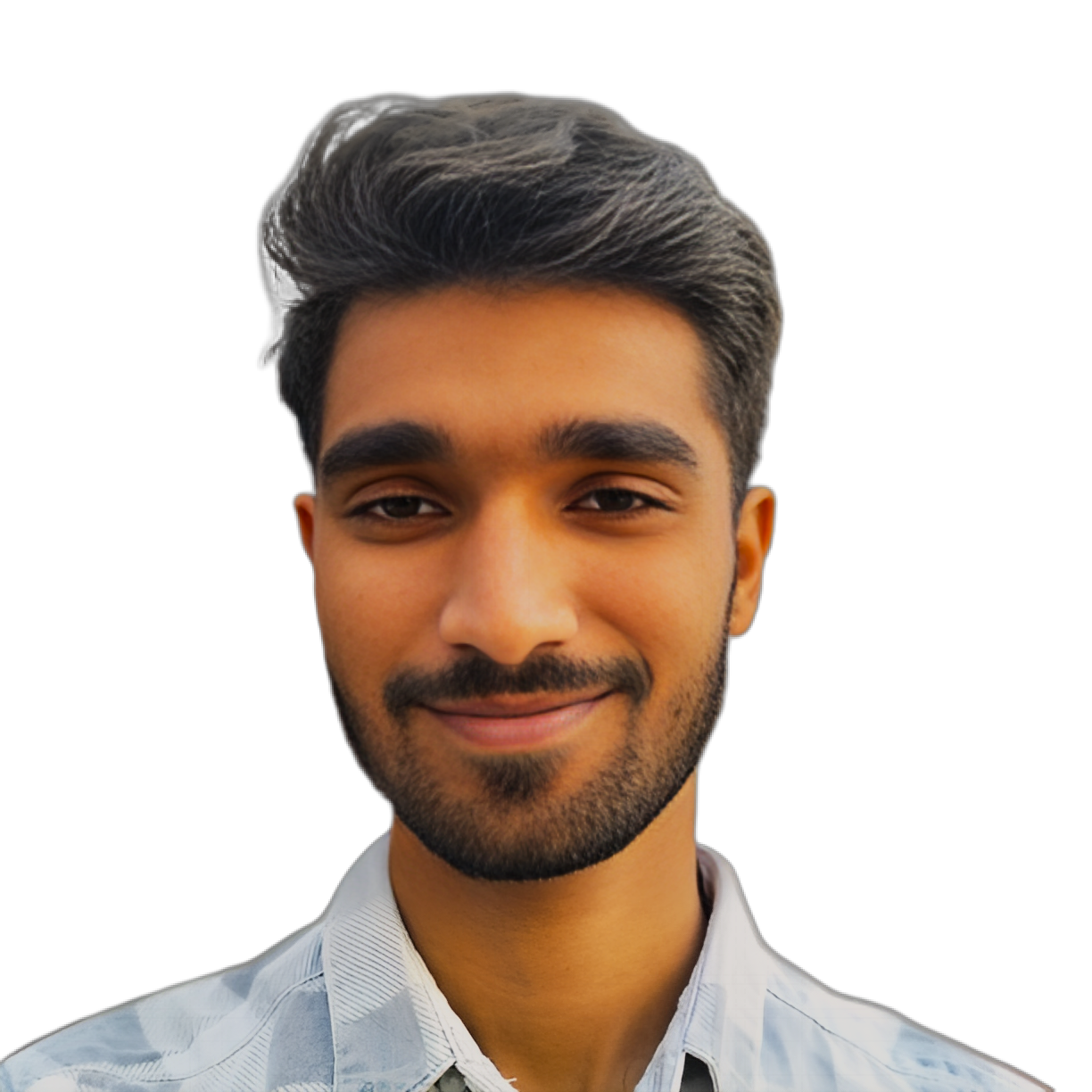
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.