Move all negative elements to end
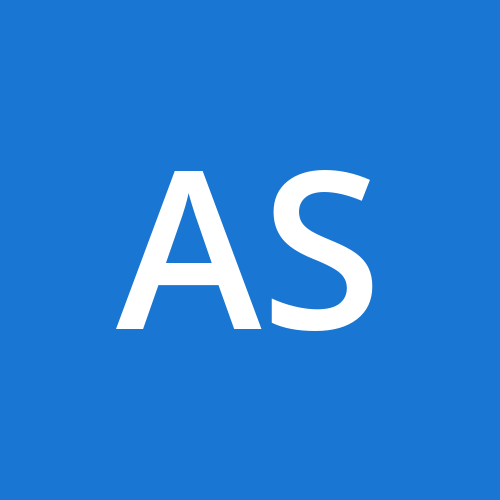
2 min read
Move all negative elements to end
Given an unsorted array arr[] of size n having both negative and positive integers. The task is place all negative element at the end of array without changing the order of positive element and negative element.
Example 1:
Input :
n = 8
arr[] = {1, -1, 3, 2, -7, -5, 11, 6 }
Output :
1 3 2 11 6 -1 -7 -5
Example 2:
Input :
n = 8
arr[] = {-5, 7, -3, -4, 9, 10, -1, 11}
Output :
7 9 10 11 -5 -3 -4 -1
Given an unsorted array arr[] of size n having both negative and positive integers. The task is place all negative element at the end of array without changing the order of positive element and negative element.
Example 1:
Input :
n = 8
arr[] = {1, -1, 3, 2, -7, -5, 11, 6 }
Output :
1 3 2 11 6 -1 -7 -5
Example 2:
Input :
n = 8
arr[] = {-5, 7, -3, -4, 9, 10, -1, 11}
Output :
7 9 10 11 -5 -3 -4 -1
Code - using Two Arrays
class Solution{
public:
void segregateElements(int arr[],int n)
{
int arr1[n]; // positive
int arr2[n]; // negative
int i, n1, n2;
for(i=0,n1=0,n2=0; i<n ; i++)
{
if(arr[i]>=0)
arr1[n1++] = arr[i];
else
arr2[n2++] = arr[i];
}
for(int i=0 ;i < n1;i++)
arr[i] = arr1[i];
// 1 3 2 1 6
// -1 -7 -5
for(int i=0,j=n1; i < n2, j<n ; i++,j++)
arr[j] = arr2[i];
}
};
Code - using One Array
class Solution{
public:
void segregateElements(int arr[],int n)
{int arr2[n], j=0;
for(int i=0; i<n;i++ )
if (arr[i] >=0)
arr2[j++]= arr[i];
for(int i=0; i<n;i++ )
if (arr[i] <0)
arr2[j++]= arr[i];
for(int i=0; i<n;i++ )
arr[i] = arr2[i];
}
};
Code - using Vector
class Solution{
public:
void segregateElements(int arr[],int n)
{
vector<int>neg;
vector<int>pos;
int i, j ;
for(int i=0;i<n;i++)
{
if(arr[i]>=0)
pos.push_back(arr[i]);
else
neg.push_back(arr[i]);
}
i=j=0;
while(i<pos.size())
arr[j++]=pos[i++];
i=0;
while(i<neg.size())
arr[j++]=neg[i++];
}
};
0
Subscribe to my newsletter
Read articles from Akshima Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
450dsa, reverse string C++ , DSA ,data structures450dsadata structuresProgramming BlogsC++DSAalgorithms
Written by
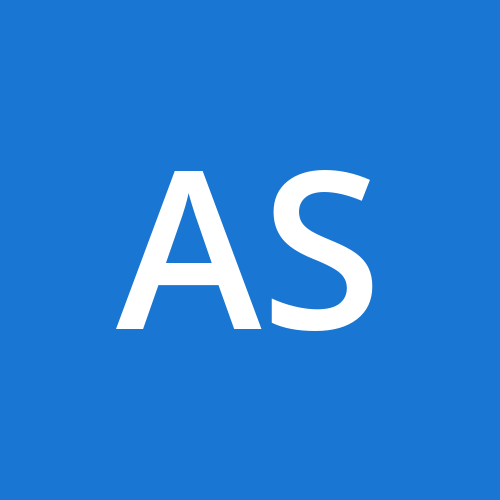