Purging Viewport Types

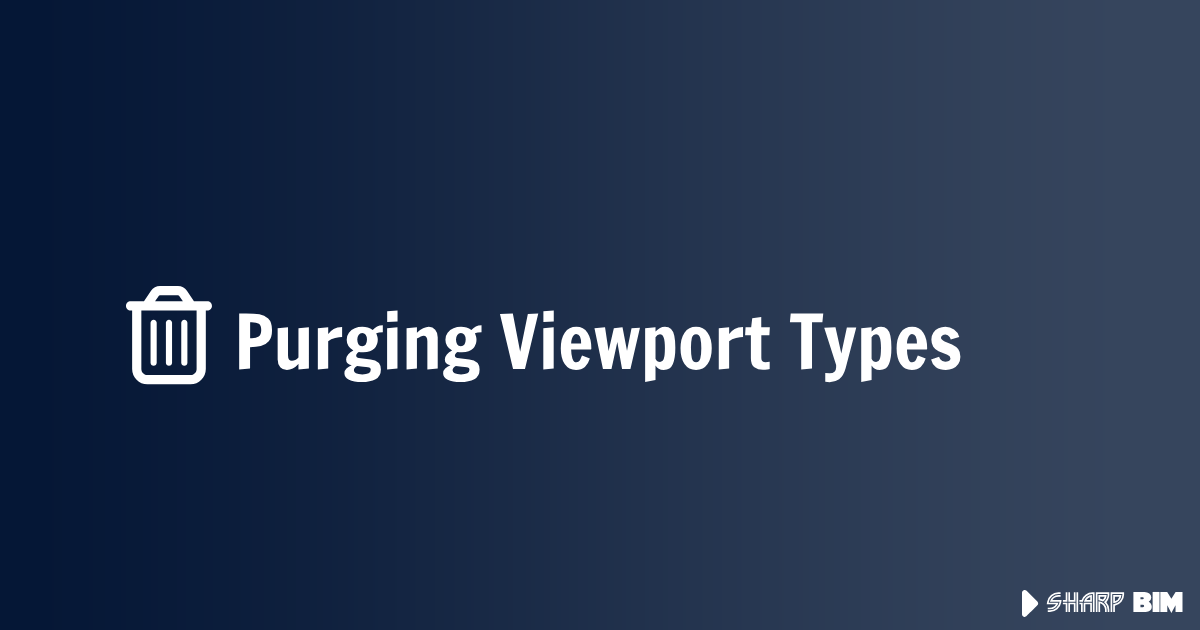
In RevitAPI, filtering and collecting elements are present in almost every developed code for writing add-ins. Usually, we locate elements by their Category
or class
type using statements like this, for example when we want to retrieve all walls from the current document.
var elements = new FilteredElementCollector(doc).OfClass(typeof(Wall));
var elements = new FilteredElementCollector(doc).OfCategory(BuiltInCategory.OST_Walls);
The process becomes trickier when trying to find an element without a defined class, like Viewport Types. When we inspect a viewport using Revitlookup, we discover that the ViewportType is categorized as ElementType.
ElementType serves as a fundamental class for all Revit elements; hence, it is not helpful. Considering alternative approaches, one could explore the possibility of identifying purgable viewports indirectly by targeting existing parameters. For example, since ViewportType is a system family all must possess the "Show Extension Line" parameter BuiltInParameter.VIEWPORT_ATTR_SHOW_EXTENSION_LINE
, we can use this parameter as a filter rule to identify all types that possess it. Fortunately, this parameter is a bool parameter, whose value is either 0 or 1, then we can use ParameterFilterRuleFactory
.
CreateGreaterOrEqualRule
and supply a value of 0 for checking.
With a list of viewport types established, the next step involves iterating through each type to retrieve the associated viewports using the GetDependentElements(ElementFilter) method.
Here's an example to illustrate the process:
// https://forums.autodesk.com/t5/revit-api-forum/deleting-unpurgeable-viewport-types-through-api/td-p/8790238
[Description("Purge Viewports")]
public void PurgeViewPorts()
{
try
{
var doc = RvtApi.Doc;
// create filterRule to be used in collection
var rule = ParameterFilterRuleFactory.CreateGreaterOrEqualRule(
new ElementId(BuiltInParameter.VIEWPORT_ATTR_SHOW_EXTENSION_LINE),
0
);
// get all elements that comply to this rule
var viewPortTypes = new FilteredElementCollector(doc)
.WhereElementIsElementType()
.OfCategory(BuiltInCategory.INVALID)
.WherePasses(new ElementParameterFilter(rule));
// now for each viewportType find a viewport instance dependent
List<ElementId> purgeableIds = new List<ElementId>();
foreach (var viewportType in viewPortTypes)
{
var viewports = viewportType.GetDependentElements(
new ElementCategoryFilter(BuiltInCategory.OST_Viewports)
);
if (!viewports.Any())
{
purgeableIds.Add(viewportType.Id);
}
}
// show a list of all purgeable Ids
MessageBox.Show(
string.Join("\r\n", purgeableIds.Select(o => o.Value().ToString()))
);
// ... handle these ids
}
catch (Exception ex)
{
// something bad happened
}
return;
}
Update: 30.12.24
I recently read of another cleaner way to fetch these viewport Types, I think i would be more inclined to the below code, less code, simpler, and easier to maintain.
FilteredElementCollector collector = new FilteredElementCollector(doc)
.OfClass(typeof(FamilySymbol))
.OfCategory(BuiltInCategory.OST_ViewportLabel);
Subscribe to my newsletter
Read articles from Moustafa Khalil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Moustafa Khalil
Moustafa Khalil
I'm Moustafa Khalil El-Sayed, an Egyptian native with a passion for architecture and technology. After completing high school in 1999, I embarked on my journey into architecture, earning my Bachelor's degree in Architecture in 2004. My academic pursuits continued, and I later acquired my Master's degree in Architecture from Wings University. However, my thirst for knowledge extended beyond architectural design, leading me to pursue a degree in Computer Science as well. Driven by a desire to innovate, I began self-teaching computer science, which later empowered me to achieve my Associate degree in Computer Science from the University of the People more easily. Armed with dual expertise in architecture and computer science, I've cultivated a unique skill set that blends creativity with technical acumen. My career has taken me across borders, from Egypt to Oman and the UAE, where I've honed my skills as an architect. As a graduate architect and a proficient .NET BIM Solution Developer, I specialize in translating unique visions into tangible realities. My role extends beyond conventional architectural practices; I leverage technology to streamline processes and enhance project outcomes. My journey into programming began with developing script-based Excel tools to augment project management efforts, notably during the Sharm El Sheikh development. Over time, I expanded my programming prowess to address practical challenges encountered in bridging Revit and AutoCAD within my professional sphere. Several of my applications, born from this evolution, have gained recognition, with some even being featured on the Autodesk website. I am committed to pushing boundaries and continually enriching the intersection of architecture and technology, ensuring that my clients benefit from cutting-edge solutions tailored to their needs.