Understanding the Basics of Linear Search
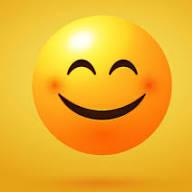
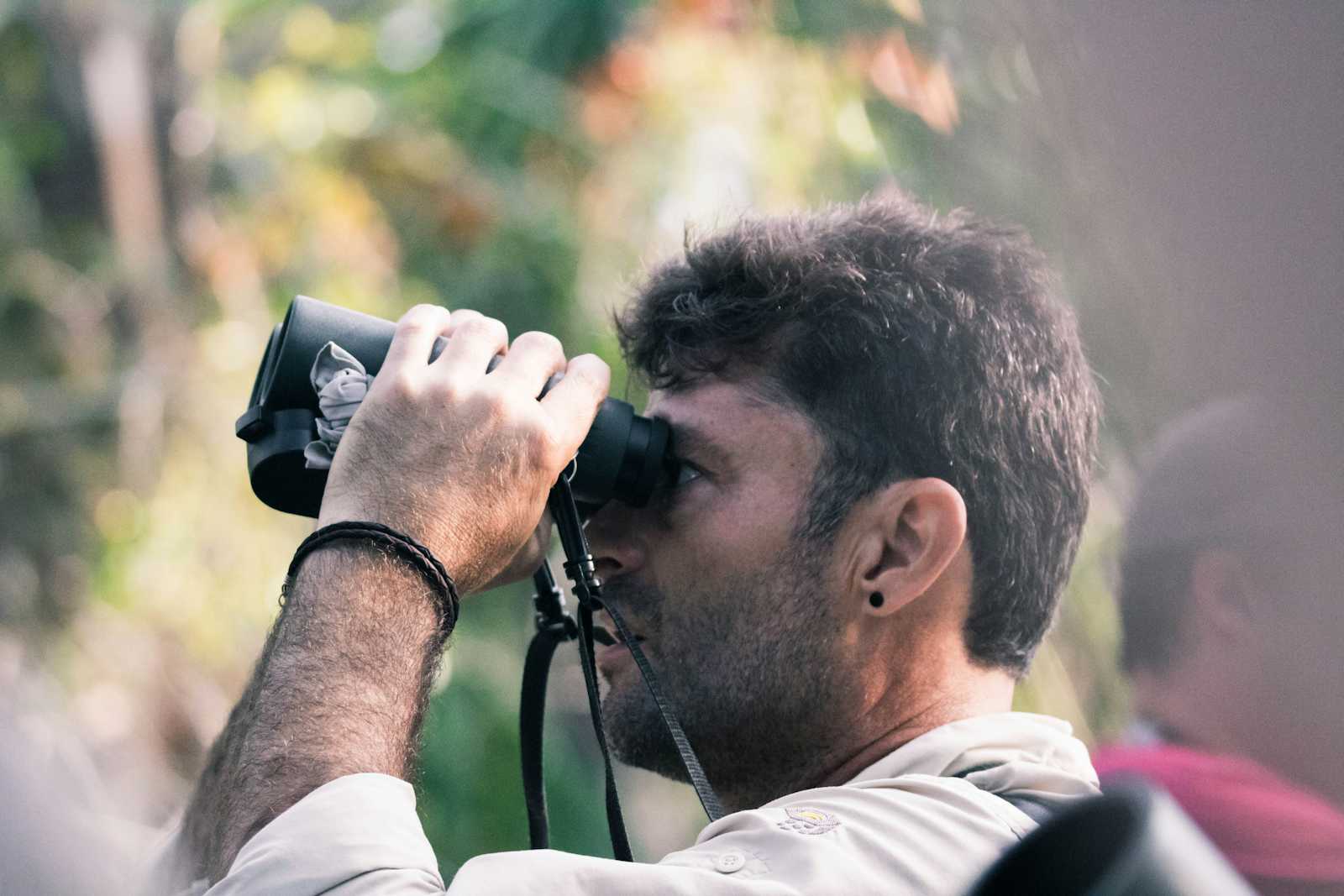
This algorithm searches for an element in an array sequentially, one by one, until either the element is found or the end of the array is reached
We start by taking the input array
arr[]
and the element to be searchedx
.We initialize a variable
n
to store the length of the array.We then traverse through each element of the array using a loop, starting from index 0 to
n-1
.At each iteration, we check if the current element of the array is equal to
x
. If it is, we return the index of that element.If we traverse the entire array without finding
x
, we return -1 to indicate thatx
is not present in the array.def linear_search(arr, x): n = len(arr) # Looping through all elements in the array for i from 0 to n-1: # If the current element is equal to x, return its index if arr[i] equals x: return i # If x is not present in the array, return -1 return -1
In short, the linear search algorithm has a time complexity of O(n) and a space complexity of O(1). It's a simple algorithm but not the best for big datasets because its time complexity increases in line with the array's size.
Subscribe to my newsletter
Read articles from YASH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
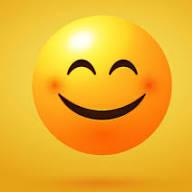
YASH
YASH
Hello there! I'm Yash, a passionate software developer with a knack for crafting engaging and user-friendly web experiences. I thrive on turning ideas into reality through code and design, always aiming to blend creativity with functionality in every project I undertake.