Interface Segregation Principle (ISP) - Javascript
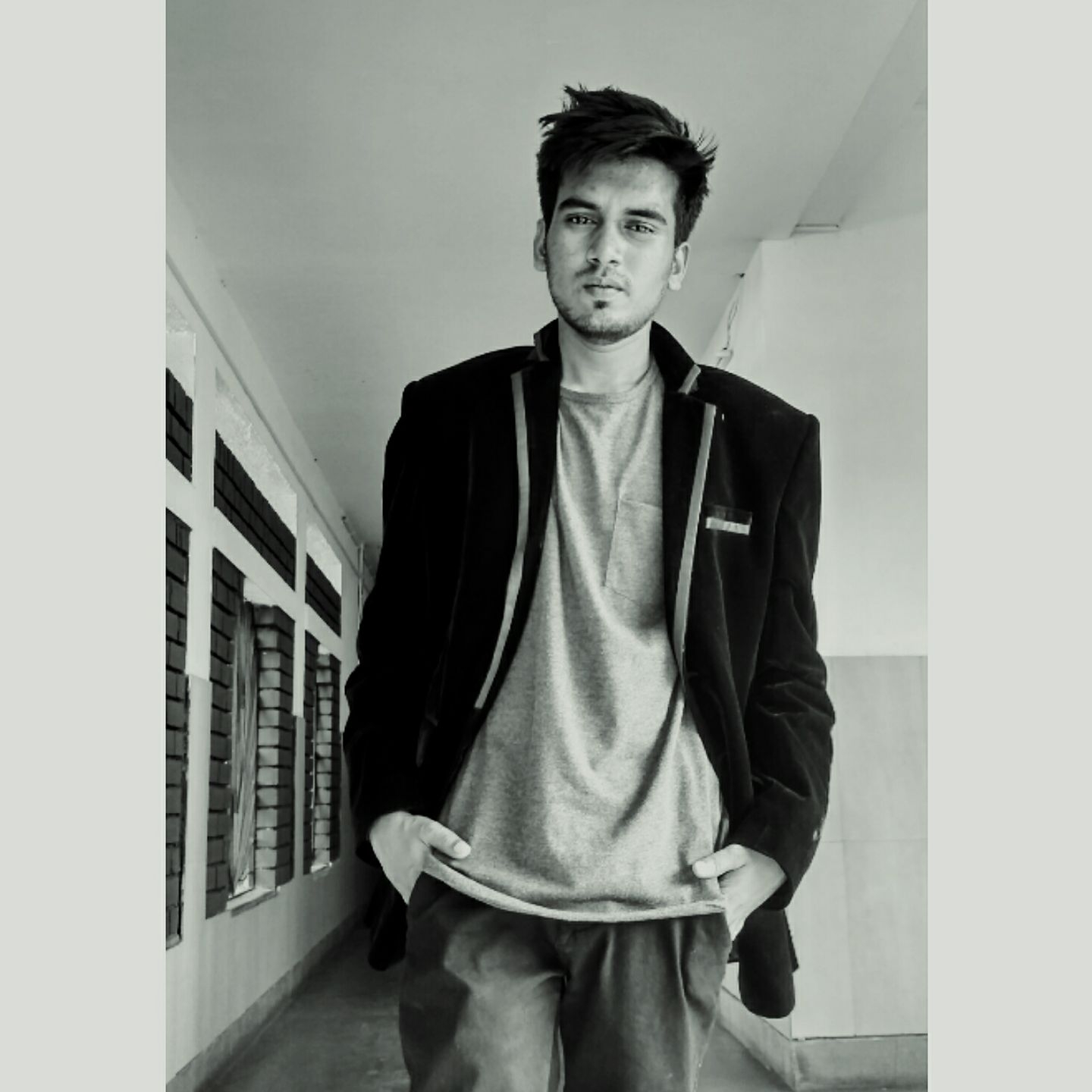
The Interface Segregation Principle (ISP) is one of the SOLID principles of object-oriented design. It suggests that no client should be forced to depend on methods it does not use. In other words, you should design your interfaces in a way that clients can use only the methods relevant to their needs.
Here's an example in JavaScript to illustrate the Interface Segregation Principle:
Suppose you are building a system for devices, and you have a generic interface called Device
class Device {
constructor(name) {
this.name = name;
}
turnOn() {
console.log(`${this.name} is turned on.`);
}
turnOff() {
console.log(`${this.name} is turned off.`);
}
changeVolume(volume) {
console.log(`${this.name} volume is adjusted to ${volume}.`);
}
play() {
console.log(`${this.name} is playing.`);
}
pause() {
console.log(`${this.name} is paused.`);
}
}
In the above code, we have the Device
class, which represents a generic device. However, not all devices support all the methods defined in this class.
Now, let's create specific device classes that implement the Device
interface, but only implement the methods relevant to their type of device
class Television extends Device {
changeVolume(volume) {
console.log(`${this.name} TV volume is adjusted to ${volume}.`);
}
play() {
console.log(`${this.name} TV is playing.`);
}
}
class Radio extends Device {
changeVolume(volume) {
console.log(`${this.name} radio volume is adjusted to ${volume}.`);
}
}
class DVDPlayer extends Device {
play() {
console.log(`${this.name} DVD player is playing.`);
}
pause() {
console.log(`${this.name} DVD player is paused.`);
}
}
// Usage
const livingRoomTV = new Television("Living Room");
const kitchenRadio = new Radio("Kitchen");
const dvdPlayer = new DVDPlayer("Home Theater DVD Player");
livingRoomTV.changeVolume(20);
kitchenRadio.play();
dvdPlayer.pause();
In this example, we have created three classes (Television
, Radio
, and DVDPlayer
) that implement the Device
interface. However, each class only implements the methods that are relevant to the specific type of device. This adheres to the Interface Segregation Principle because clients using these classes are not forced to depend on methods they don't use.
By designing the interfaces and classes this way, you ensure that clients only need to interact with the methods that make sense for their specific requirements, promoting a more modular and maintainable codebase.
Subscribe to my newsletter
Read articles from Harish Gautam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
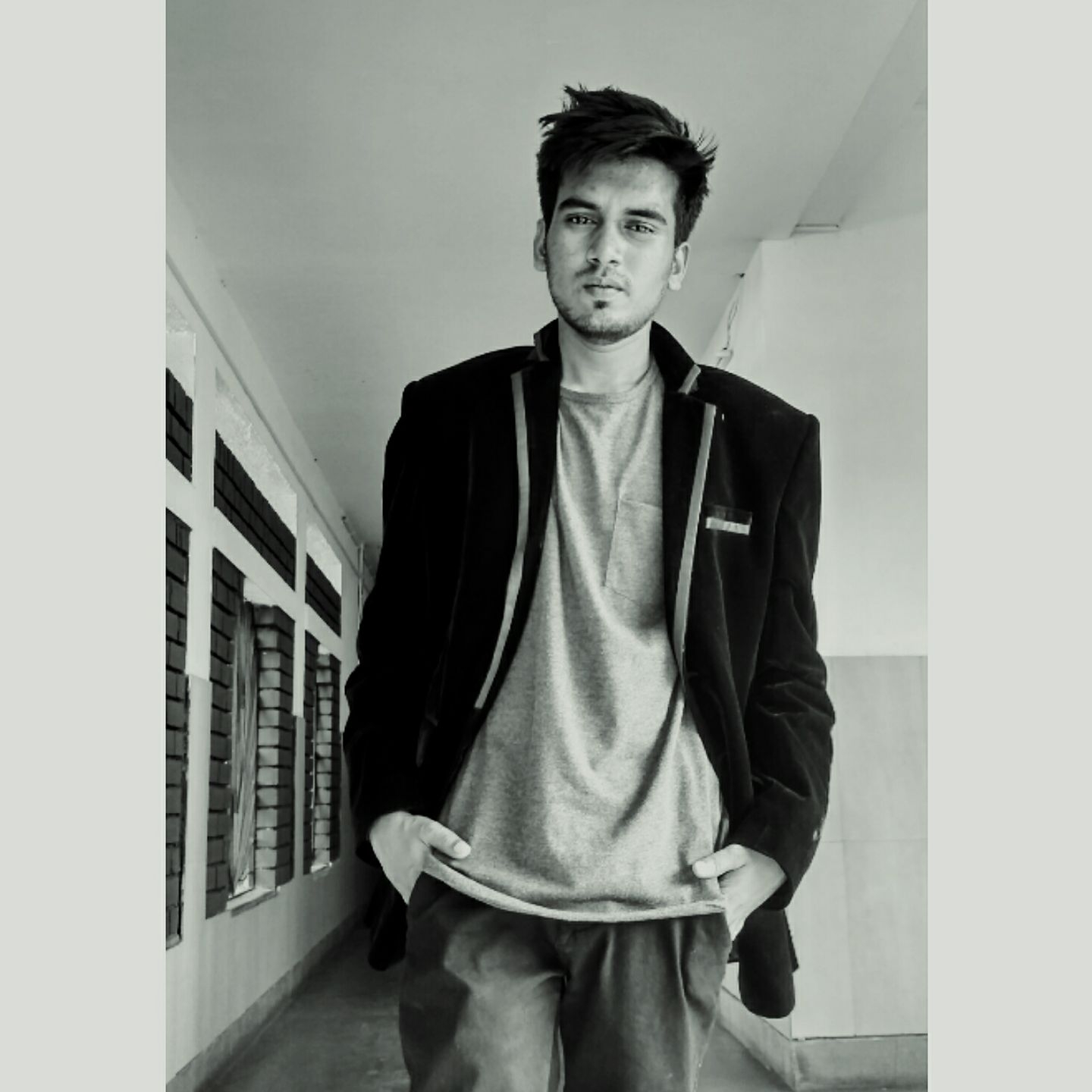