Understanding Objects in JavaScript
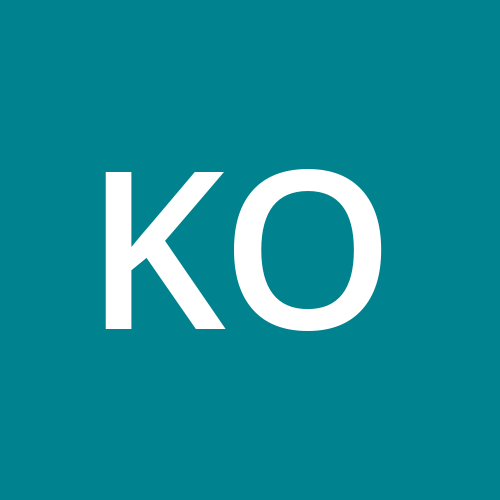
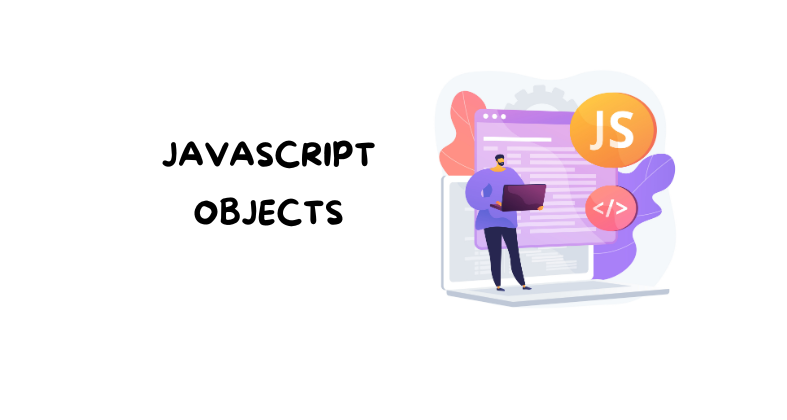
Welcome to the world of JavaScript! If you're just starting your journey into web development or programming in general, understanding objects is a crucial step. Objects in JavaScript are fundamental building blocks that allow you to organize and manipulate data in a meaningful way. In this beginner-friendly blog post, we'll explore what objects are, how to create them, and some basic operations you can perform with them.
What are Objects in JavaScript? ๐งฉ
In JavaScript, an object is a collection of key-value pairs, where each key is a string (also called a property name), and each value can be of any data type: a string, a number, a boolean, another object, or even a function. This flexibility is what makes objects so powerful and versatile.
Creating Objects ๐ ๏ธ
There are a several methods to create objects in JavaScript. Here are some of the most common methods:
Object Literals
Constructor Function
Let's start with the most common method using object literals:
// Creating an object using object literal syntax
let person = {
name: "John Doe",
age: 30,
isStudent: true,
hobbies: ["reading", "coding", "playing guitar"],
greet: function() {
console.log("Hello!");
}
};
In this example, person
is an object with four properties (name
, age
, isStudent
, hobbies
) and one method (greet
). Notice how we define properties and methods inside curly braces {}
.
Accessing Object Properties ๐๏ธ
You can access object properties using two methods.
Dot notation ( . )
Bracket Notation [ ]
Here's how you can access object properties using both methods:
1.Dot Notation :- (objectName.propertyName
)
const person = {
name: 'John',
age: 30,
city: 'New York'
};
console.log(person.name); // Output: John
console.log(person.age); // Output: 30
console.log(person.city); // Output: New York
2. Bracket Notation :- (objectName[propertyName]
)
const person = {
name: 'John',
age: 30,
city: 'New York'
};
console.log(person['name']); // Output: John
console.log(person['age']); // Output: 30
console.log(person['city']); // Output: New York
Adding and Modifying Properties ๐
Objects in JavaScript are mutable, which means you can add new properties or modify existing ones easily using assignment operator ( =
):
// Adding a new property
person.city = "New York";
// Modifying an existing property
person.age = 32;
console.log(person.city); // Output: New York
console.log(person.age); // Output: 32
Object Methods ๐ฏ
As mentioned earlier, objects can also have methods, which are simply functions stored as object properties. You can define and use methods like this:
// Defining a method
person.sayHello = function() {
console.log(`Hello, my name is ${this.name}.`);
};
// Calling the method
person.sayHello(); // Output: Hello, my name is John Doe.
Inside the method, this
refers to the object itself (person
in this case), allowing you to access its properties within the method.
Object Iteration ๐
You can loop through an object's properties using for...in
loop:
// Iterating over object properties
for (let key in person) {
console.log(`${key}: ${person[key]}`);
}
This loop will log each property name and its corresponding value in the person
object.
Conclusion ๐
Objects are a fundamental part of JavaScript and are used extensively in web development for organizing and managing data. By understanding how to create, access, modify, and iterate over objects, you've taken a significant step toward becoming proficient in JavaScript programming.
Keep practicing and exploring different aspects of JavaScript to deepen your understanding and enhance your skills. Happy coding! ๐
Subscribe to my newsletter
Read articles from Kashish Oza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
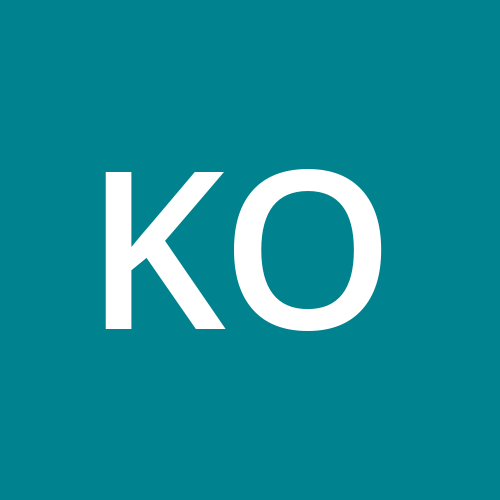