Leetcode 2441. Largest Positive Integer That Exists With It's Negative
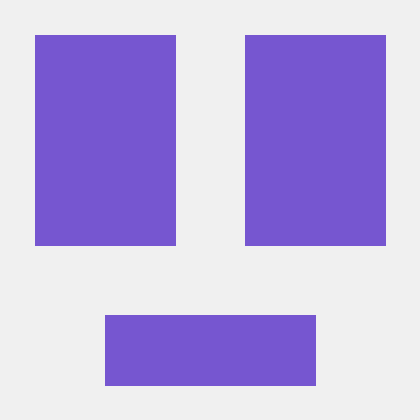
Intuition
Sort the array and check if the start and end of the array match. If not, increment or decrement accordingly.
Approach
First sort the array. Then use two pointers. One at the beginning, the other at the end. Check to see if the value at the beginning (the smallest integer) is the same as the value at the end (the highest integer) once converted from negative to positive.
If it's the same, return that value.
If the converted integer is smaller, decrement the right pointer.
If the converted integer is larger, increment the left pointer.
If the left and right pointers meet, there is no matching value so return -1.
Code
public class Solution
{
public int FindMaxK(int[] nums)
{
Array.Sort(nums);
int left = 0;
int right = nums.Length - 1;
while(left < right)
{
var comparer = nums[left] * -1;
if(comparer == nums[right])
{
return comparer;
}
else if(comparer < nums[right])
{
right--;
}
else
{
left++;
}
}
return -1;
}
}
Subscribe to my newsletter
Read articles from Kenny Carneal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
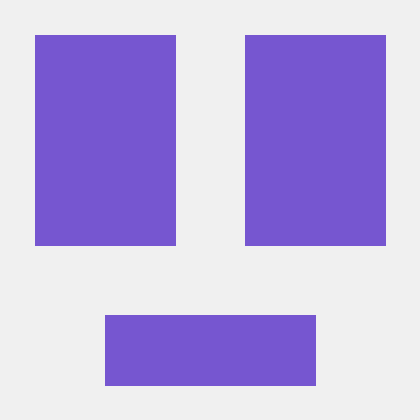
Kenny Carneal
Kenny Carneal
I am a developer from Nashville, TN. I specialize in the .NET tech stack. I have created many projects in Blazor WASM, Xamarin, MAUI, etc.