Improving Geospatial Data Performance: Exploring Douglas-Peucker, Radial Distance Algorithms, and Simplify-JS

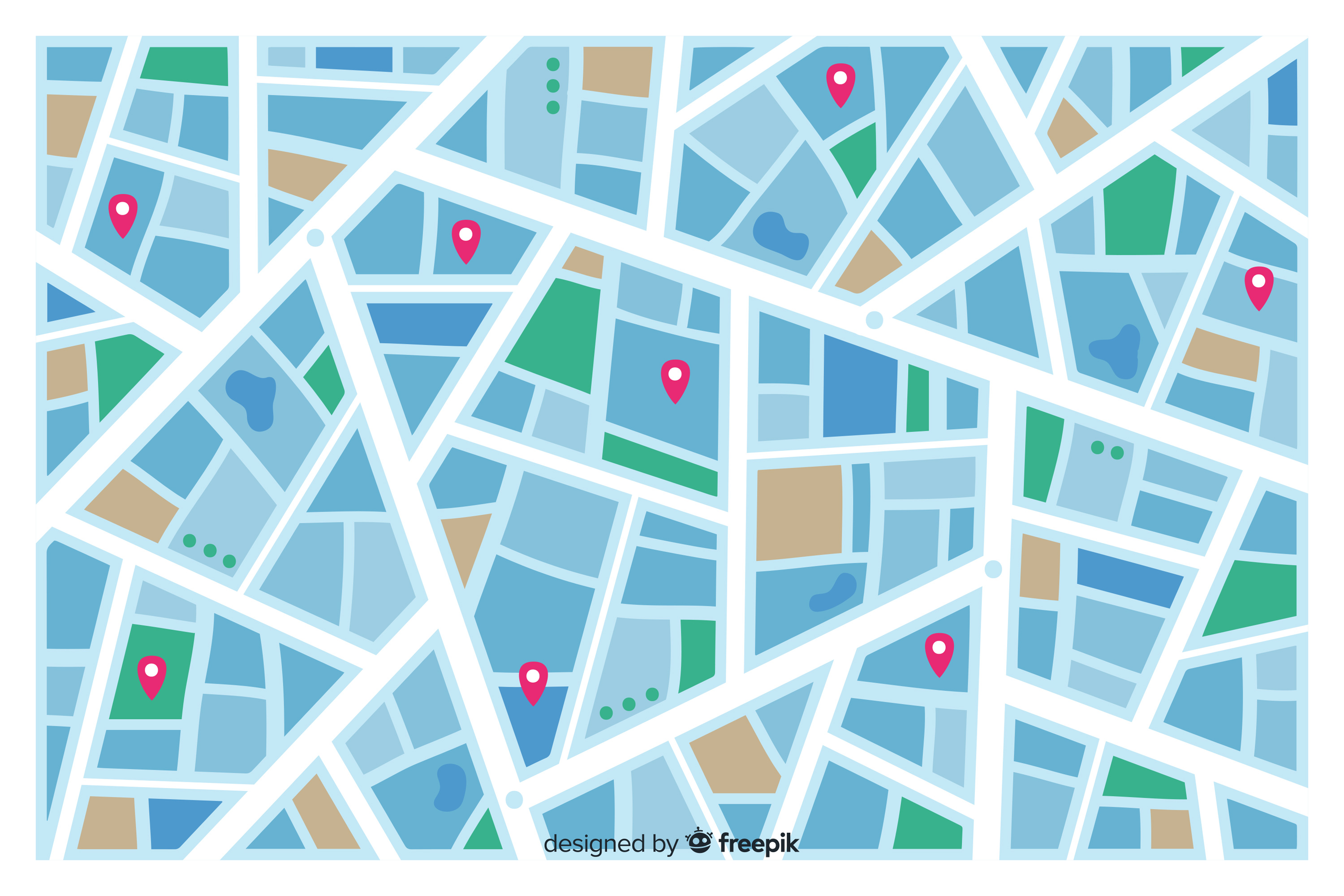
The integration of mapping functionality, particularly with services like Google Maps, has become ubiquitous. However, with great functionality comes the challenge of performance optimization, especially when dealing with large datasets of geographic coordinates. In this article, we'll explore how the combination of two powerful algorithms, Douglas-Peucker and Radial Distance, along with the Simplify-JS library, can drastically enhance the performance of frontend applications, resulting in smoother user experiences.
Understanding the Challenge
Imagine you're building a frontend application that tracks and displays the route taken by a user on a Google Map. As the user traverses various locations, the application continuously logs their coordinates, resulting in a growing array of latitude and longitude values. While this approach accurately captures the user's journey, it can quickly lead to performance issues when rendering the entire route on the map. With thousands of coordinate points to display, the frontend may become sluggish, negatively impacting user experience.
Enter Douglas-Peucker and Radial Distance Algorithms
To address the challenge of rendering complex polyline data efficiently, developers often turn to algorithms that can intelligently reduce the number of points while preserving the essential shape of the route. Two such algorithms are Douglas-Peucker and Radial Distance.
Douglas-Peucker Algorithm: This algorithm simplifies a polyline by recursively dividing it into smaller segments and retaining only those that deviate significantly from the original shape. By specifying a tolerance parameter, developers can control the level of simplification, balancing between accuracy and performance.
Radial Distance Algorithm: In contrast to Douglas-Peucker, which focuses on preserving the overall shape of the polyline, the Radial Distance algorithm simplifies by removing points that are within a certain distance threshold of each other. This approach is particularly effective for streamlining curved segments of a route while maintaining its general trajectory.
Introducing Simplify-JS
While the Douglas-Peucker and Radial Distance algorithms offer powerful simplification techniques, implementing them from scratch can be complex and time-consuming. This is where the Simplify-JS library comes into play. Simplify-JS provides a user-friendly interface for applying these algorithms to polyline data, streamlining the integration process and enabling developers to focus on building robust applications.
Official Doc: https://mourner.github.io/simplify-js/
NPM Package: https://www.npmjs.com/package/simplify-js
Practical Implementation
Let's examine how these concepts are applied in a real-world scenario using Angular and Google Maps:
First run: npm i simplify-js
import * as simplify from 'simplify-js';
drivingPath = []
pushOnMap(lat, lng) {
// Push new coordinates to the array
this.drivingPath.push({ lat: parseFloat(latitude), lng: parseFloat(longitude) });
// Simplify the polyline using Simplify-JS
const tolerance = 0.0001;
const simplifiedPath = simplify(this.changeKeyNames(this.drivingPath), tolerance, false);
// Convert simplified path to Google Maps-compatible format
const miniMapPath = simplifiedPath.map(point => ({ lat: point.y, lng: point.x }));
this.drivingPath= miniMapPath;
}
// Convert keys from lat/lng to x/y for Simplify-JS compatibility
changeKeyNames(array) {
return array.map(obj => ({
y: obj.lat,
x: obj.lng
}));
}
In this Angular component method, pushOnMap()
, new coordinates are added to the drivingPath
array and then simplified using the Simplify-JS library with a specified tolerance. The resulting simplified polyline is then converted to the format expected by Google Maps for rendering.
Conclusion
By leveraging the power of algorithms like Douglas-Peucker and Radial Distance, combined with the ease of use provided by libraries like Simplify-JS, you can significantly enhance the performance of frontend applications that utilize Google Maps. By intelligently reducing the complexity of polyline data while preserving its essential shape, these techniques enable smoother rendering and improved user experiences.
Thanks for reading ❤
Subscribe to my newsletter
Read articles from Bablu Roy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
