Understanding the Option Type in OCaml
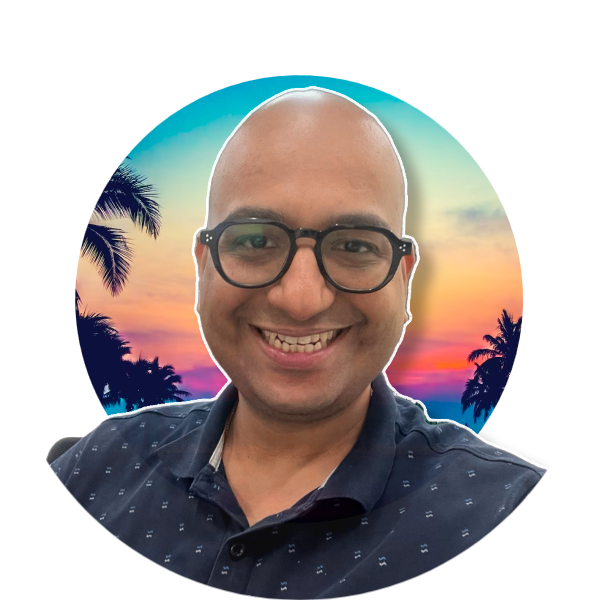
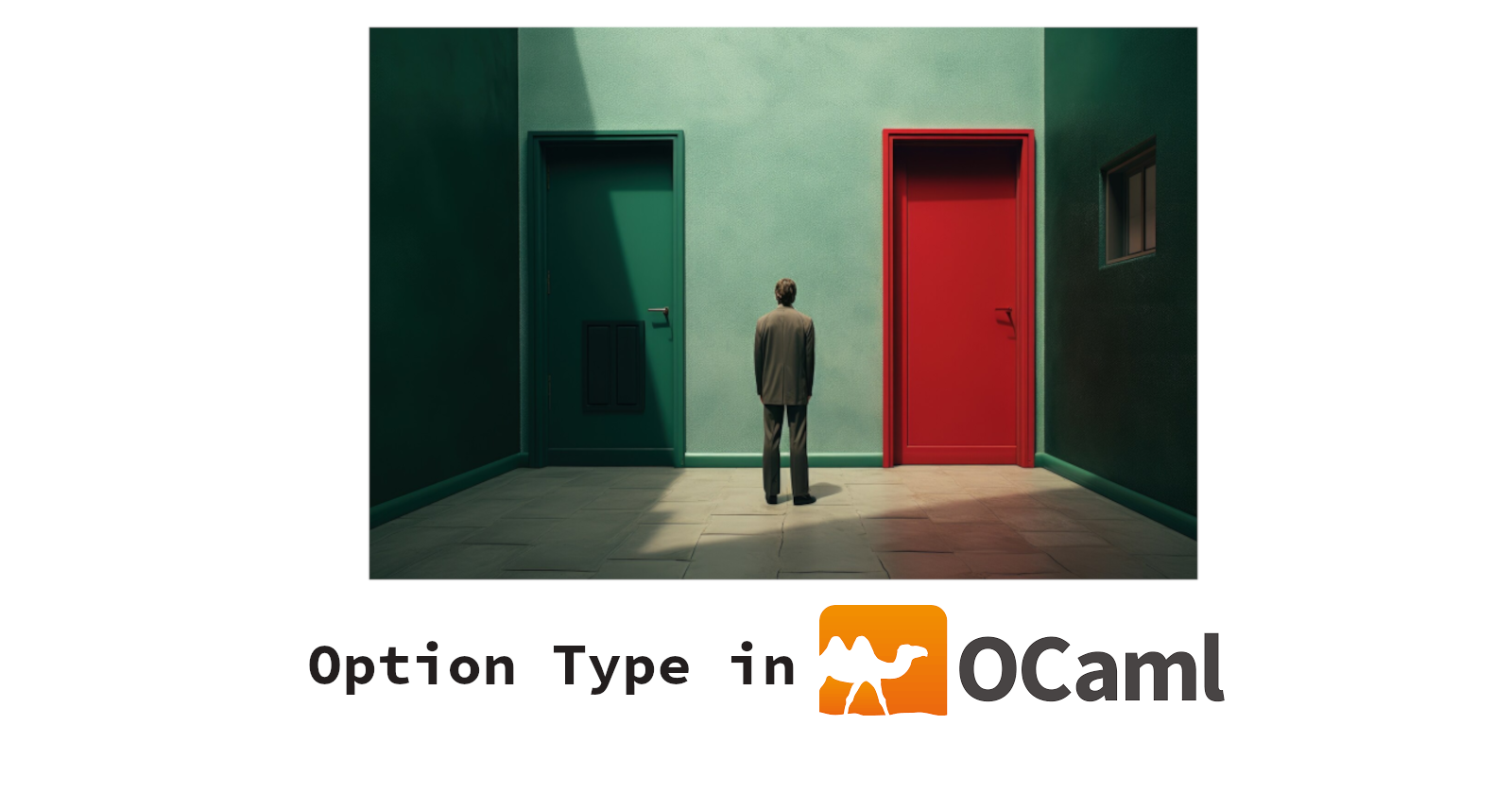
Introduction
In the vast world of programming languages, OCaml stands out for its robust type system and functional programming paradigm, offering developers a unique toolbox for writing concise, efficient, and safe code. Among its many features, the Option type is particularly noteworthy, representing a fundamental departure from the more traditional error-handling and data-representation methods found in languages like C, Java, or Python. The Option type encapsulates the possibility that a value might be absent, addressing a common problem in programming: how to safely handle data that may or may not be present.
Traditionally, languages have relied on null references, special values, or exception handling to denote or manage the absence of data. However, these approaches come with their own set of problems, such as null-pointer exceptions and the overhead of exception handling. The Option type in OCaml offers a more elegant and safer alternative, making the presence or absence of a value explicit in the type system itself. This not only aids in catching potential errors at compile-time but also forces developers to explicitly deal with the absence case, leading to more robust code.
Understanding the Option type and how to effectively use it is crucial for anyone looking to dive into OCaml or functional programming in general. It epitomizes the functional approach of handling errors and data variability without resorting to exceptions or nulls, paving the way for writing clear, maintainable, and error-resistant software. Let's explore the Option type in more detail, starting with a simplified explanation suitable for beginners.
Simpler explanation
Imagine you have a treasure chest. This chest can either hold a precious gem or be empty. In OCaml, the Option type is like this chest. It's a special box that can either hold a value (Some value
) or hold nothing (None
). This is very helpful in programming because it allows us to safely handle situations where a value might be missing without causing errors.
Examples
Handling potential absence of environment variables:
You want to read an environment variable, but it might not be set.
Normally, trying to read an unset variable could cause an error.
With Option, you can check if the value is
Some
(exists) orNone
(doesn't exist) and decide what to do next.
let get_env_variable name =
match Sys.getenv_opt name with
| Some value -> Printf.sprintf "Found: %s" value
| None -> "Not found"
Preventing division by zero errors:
Division by zero is a common source of errors in programming.
Before dividing, you can ensure the denominator is not zero and return an option type.
This makes your function safer by explicitly handling the zero case.
let safe_divide x y =
if y = 0 then None else Some (x / y)
Use cases
Error handling without exceptions: Instead of using try-catch blocks or letting exceptions propagate, you can use the Option type to represent the presence or absence of an error.
Optional function parameters: Functions can take optional parameters wrapped in an Option type, allowing callers to provide a value or leave it out.
Safe resource access: When accessing resources that mighsimilar language features int not be available (like files or network services), the Option type can signal whether the operation succeeded without resorting to error codes or exceptions.
Database queries: When querying a database, the result might be an empty set. Wrapping the result in an Option type can cleanly represent this possibility.
Comparison with other popular languages
Go: Go handles absence using pointers and a special
nil
value. However, it lacks a built-in option type, making null-pointer errors more common.Python: Python often uses
None
to indicate the absence of a value. This is similar to theOption
type but is less type-safe, asNone
can be assigned to any variable regardless of its expected type.TypeScript: TypeScript uses the concept of "optional" types and values. A variable can be marked as optional with
?
, allowing it to hold either a value orundefined
. This is similar to OCaml's Option type but integrated into the type system at a language level.
Conclusion
The Option type in OCaml offers a powerful and type-safe way to handle the absence of values in a program. By explicitly requiring the handling of Some
and None
cases, it ensures that programs are more robust and less prone to errors caused by unhandled null or undefined values. This contrasts with and improves upon the approaches taken by languages like Go and Python, where the absence of a built-in option or maybe type can lead to null-pointer exceptions or the misuse of nil
or None
. Compared to TypeScript's optional types, OCaml's Option type provides a more explicit way to deal with optional values, requiring explicit handling at runtime. As modern software development moves towards more reliable and error-resistant designs, understanding and using the Option type effectively can significantly improve the quality and safety of OCaml programs.
References
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
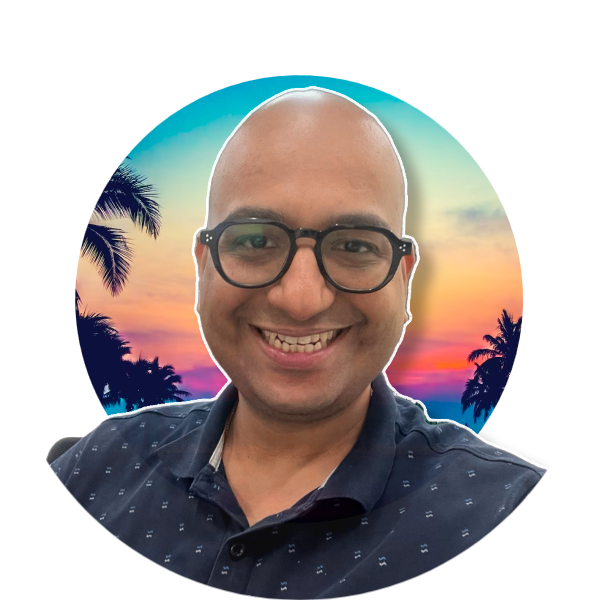
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.