Rotate BoundingBox

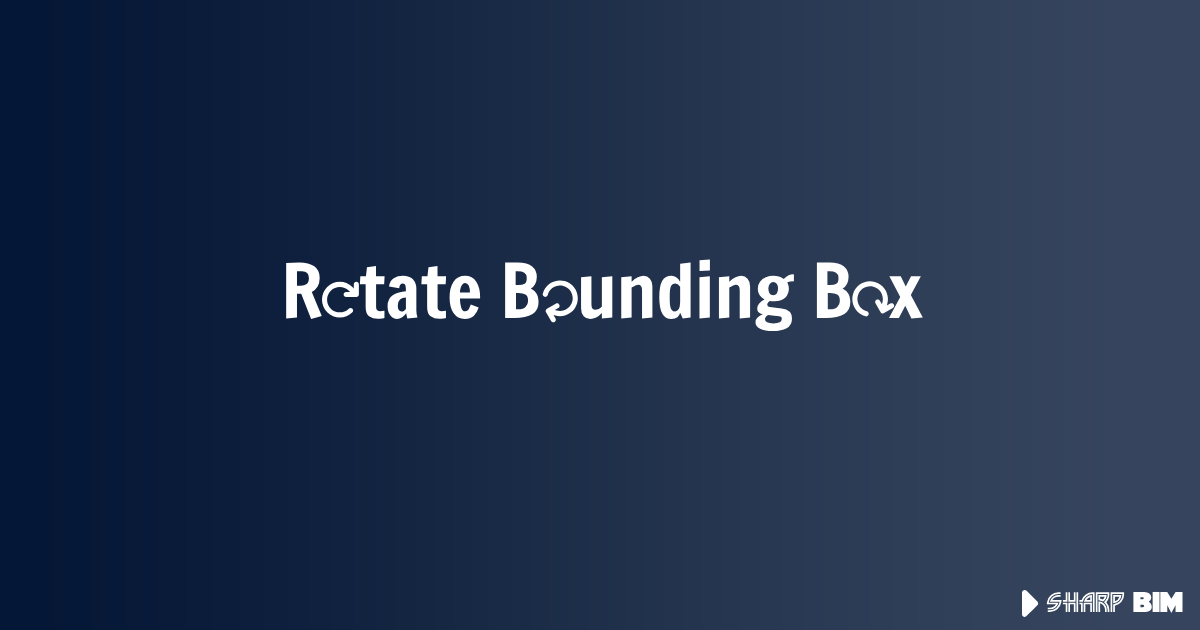
BoundingBox, as the name suggests, is a box that contains elements inside it. The RevitAPI allows developers to access this box for various purposes like filtering, determining its position, dimensions, and more. It's important to note that the bounding box is always aligned with the cardinal axis. This means it doesn't change based on how the elements inside are rotated or scaled.
In the illustration below, the red dashed rectangle defines the bounding box around elements.
This might be a bit confusing for new developers because one might initially think the box would also rotate. However, we can virtually rotate the bounding box using a Transformation. For instance, if we want a rotated transform, we need to define an angle and position. Think of it like drawing a circle with a compass where the needle is the position and the angle is how we rotate the head.
We can then obtain any point using Transform.OfPoint(XYZ)
. So, rotating is figured out. The issue now is that if we rotate the bounding box, it may end up larger than it should be. For example, in the illustration below, the bounding box that originally fit a wall may no longer match the wall.
To solve this problem, we need to determine the angle of the wall that best aligns horizontally or vertically. Then, after temporarily rotating the wall with the negative of the angle, getting the bounding box, rolling back the transaction, and finally rotating the bounding box, you'll achieve the desired result.
I have created a pseudocode below to illustrate how this process works. This pseudocode generates detail lines of the bottom face of the rotated bounding box.
// get the angle by which defines the element rotation
var angle = element.Direction.AngleTo(XYZ.BasisX);
// start a transaction
Trans.Start();
// rotate element
ElementTransformUtils.RotateElement(
element.Document,
element.Id,
Line.CreateBound(bound.GetCG(), bound.GetCG() + XYZ.BasisZ),
-angle
);
// regenerate the document to refresh the changes
RvtApi.Doc.Regenerate();
// extract the bounding box
bound = element.GetBoundingBox();
// roll back the transaction since we don't need to rotate the actual element
Trans.RollBack();
// create a rotation transform
var transform = Transform.CreateRotationAtPoint(XYZ.BasisZ, angle, bound.GetCG());
// extract the points needed
var points = bound
.Midpoints()
.BottomCorners.Select(o => transform.OfPoint(o))
.ToList();
// draw lines on Revit to illustrate the result
Trans.Start();
for (int i = 1; i < points.Count; i++)
{
Doc.Create.NewDetailCurve(
UiDoc.ActiveGraphicalView,
Line.CreateBound(points[i - 1], points[i])
);
}
Doc.Create.NewDetailCurve(
UiDoc.ActiveGraphicalView,
Line.CreateBound(points.Last(), points.First())
);
Trans.Commit();
Subscribe to my newsletter
Read articles from Moustafa Khalil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Moustafa Khalil
Moustafa Khalil
I'm Moustafa Khalil El-Sayed, an Egyptian native with a passion for architecture and technology. After completing high school in 1999, I embarked on my journey into architecture, earning my Bachelor's degree in Architecture in 2004. My academic pursuits continued, and I later acquired my Master's degree in Architecture from Wings University. However, my thirst for knowledge extended beyond architectural design, leading me to pursue a degree in Computer Science as well. Driven by a desire to innovate, I began self-teaching computer science, which later empowered me to achieve my Associate degree in Computer Science from the University of the People more easily. Armed with dual expertise in architecture and computer science, I've cultivated a unique skill set that blends creativity with technical acumen. My career has taken me across borders, from Egypt to Oman and the UAE, where I've honed my skills as an architect. As a graduate architect and a proficient .NET BIM Solution Developer, I specialize in translating unique visions into tangible realities. My role extends beyond conventional architectural practices; I leverage technology to streamline processes and enhance project outcomes. My journey into programming began with developing script-based Excel tools to augment project management efforts, notably during the Sharm El Sheikh development. Over time, I expanded my programming prowess to address practical challenges encountered in bridging Revit and AutoCAD within my professional sphere. Several of my applications, born from this evolution, have gained recognition, with some even being featured on the Autodesk website. I am committed to pushing boundaries and continually enriching the intersection of architecture and technology, ensuring that my clients benefit from cutting-edge solutions tailored to their needs.