Leveraging Test-Driven Development (TDD) in an API-First Approach
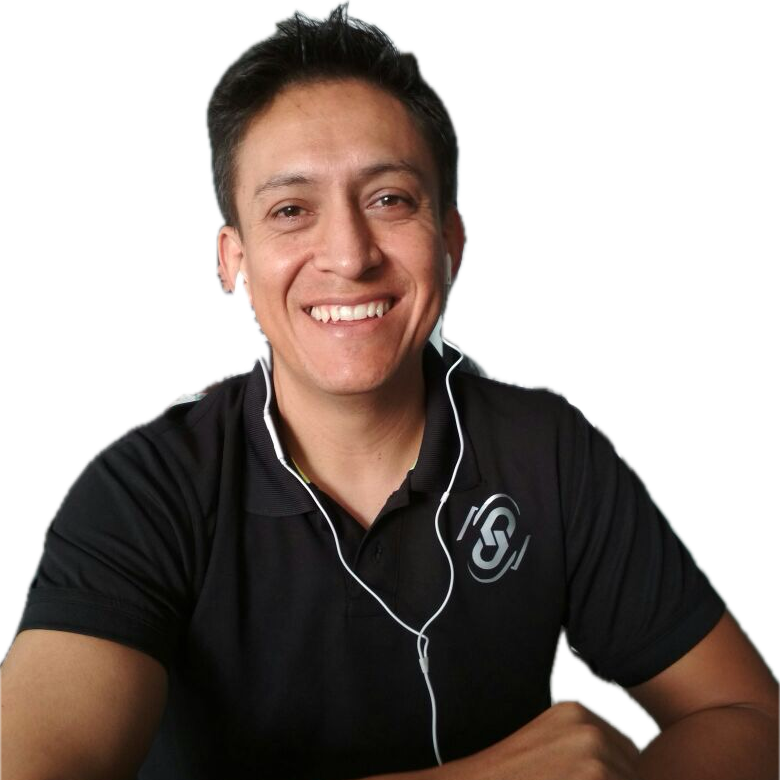
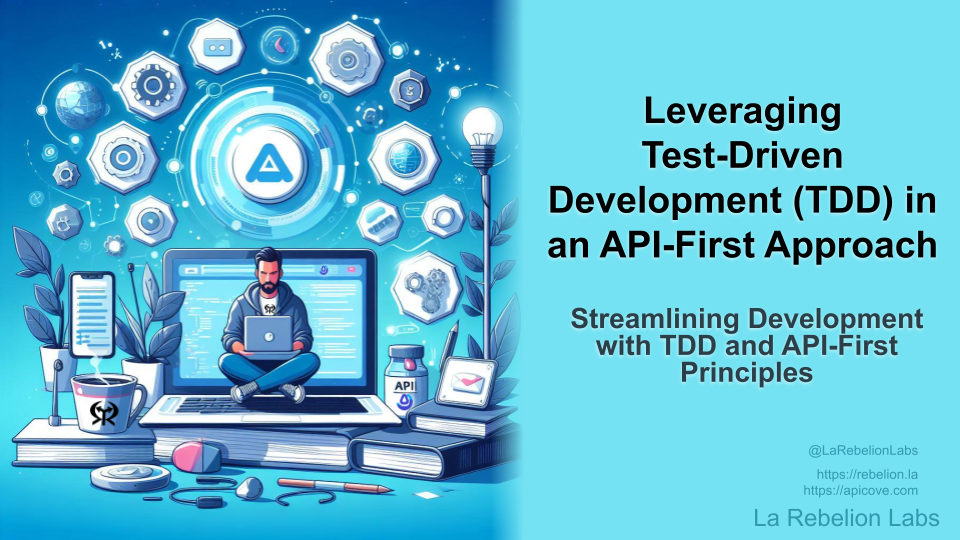
Adopting the right methodologies in today's fast-paced development landscape can make all the difference in delivering robust and scalable software solutions. One such powerful combination is Test-Driven Development (TDD) alongside the API-first approach. In this blog post, we'll explore how integrating TDD principles with an API-first mindset can streamline the development process, enhance code quality, and ensure the success of your projects.
The tools and concepts we'll cover include:
Understanding the API-First Approach
At the core of the API-first approach is the idea of designing and building the API before anything else. This involves meticulously crafting the API specifications, defining endpoints, request/response schemas, and error-handling mechanisms upfront. With the API serving as the contract between different components of the system, this approach fosters clarity, consistency, and interoperability.
Swagger Specifications
Swagger is a popular framework for designing, building, and documenting APIs. It provides a set of tools for creating API specifications in the OpenAPI format, which defines a standard way to describe RESTful APIs. Swagger specifications can be written in YAML or JSON and include details such as endpoints, methods, parameters, responses, and security requirements.
There are two versions of the OpenAPI specification: OpenAPI 2.0 (formerly known as Swagger - 2014-09-08) and OpenAPI 3.0. OpenAPI 3.0 introduces several enhancements, including improved support for request/response validation, enhanced security definitions, and better support for complex data structures.
Swagger Editor
The Swagger Editor is a web-based tool that allows developers to create and edit Swagger specifications interactively. It provides a user-friendly interface for defining API endpoints, parameters, responses, and security requirements. The Swagger Editor also offers real-time validation and syntax highlighting to help developers write valid and well-structured API specifications.
Contract-First Development
In the API-first approach, developers follow a contract-first development model, where the API contract serves as the foundation for building the system. This contract defines the expected behavior of the API, including input/output formats, error handling, and authentication mechanisms. By establishing a clear contract upfront, developers can ensure that different components of the system interact seamlessly and consistently.
Contract-First vs. Code-First
Don't confuse contract-first with code-first development. In the code-first approach, developers write the implementation code first and then generate API specifications from the code. While code-first development can be faster in the short term, it often leads to inconsistencies, undocumented features, and tight coupling between components. In contrast, the contract-first (or design-first) approach promotes loose coupling, better separation of concerns, and improved maintainability.
The long-term benefits of contract-first development include:
Improved Interoperability: By defining a clear contract, developers can ensure that different components of the system can communicate effectively with each other.
Enhanced Documentation: API specifications serve as living documentation that describes the API's functionality, usage, and constraints.
Better Testability: With well-defined contracts, developers can write comprehensive test suites that cover all possible scenarios and edge cases.
Easier Maintenance: Changes to the API can be made more confidently and efficiently, as developers can refer back to the contract to understand the impact of modifications.
Faster Onboarding: New team members can quickly understand the API's design and behavior by referring to the contract.
Increased Collaboration: API specifications provide a common language for developers, testers, and stakeholders to discuss requirements and expectations.
Reduced Risks: By validating the API contract early in the development process, teams can catch potential issues before they escalate into costly problems.
Scalability and Flexibility: Well-designed API contracts can adapt to changing business requirements and evolving technologies.
Compliance and Security: API specifications can help ensure that the API complies with industry standards, regulations, and security best practices.
Code Generation from API Specifications
One of the key benefits of the contract-first approach is the ability to generate code from API specifications automatically. Tools like APICode Converter and Swagger Codegen can transform OpenAPI specifications into client libraries, server stubs, and API documentation in various programming languages. This code generation process accelerates development, reduces manual errors, and ensures consistency between the API contract and the implementation code.
Integrating TDD into the API-First Workflow
TDD is a development practice where tests are written before the actual implementation code. The process typically follows the Arrange-Act-Assert (AAA) pattern, where tests are structured into setup, action, and verification phases, some call this the "Red-Green-Refactor" cycle. By applying TDD principles within the API-first workflow, developers can ensure that the API meets the specified requirements and behaves as expected.
Arrange-Act-Assert (AAA) Pattern
The AAA pattern is a common structure for organizing test cases in TDD. It consists of three main sections:
Arrange: Set up the test environment by initializing objects, configuring dependencies, and preparing the system under test.
Act: Perform the action or operation that you want to test, such as calling a method, sending a request, or triggering an event.
Assert: Verify the outcome of the action by checking the expected results, state changes, or side effects. Ensure that the API contract is accurately reflected in the implementation code.
Red-Green-Refactor cycle
The Red-Green-Refactor cycle is a fundamental principle of TDD that guides developers through the process of writing tests and implementing code iteratively. The cycle consists of three main steps:
Red: Write a failing test that captures the desired behavior or functionality.
Green: Write the minimum amount of code needed to make the test pass.
Refactor: Improve the codebase by removing duplication, enhancing readability, optimizing performance, and enhancing maintainability.
Combining the AAA pattern with the Red-Green-Refactor cycle helps developers write clean, maintainable, and well-tested code that meets the specified requirements.
Finally, in this integration, the cycle is extended to include the following steps:
Teardown: Clean up any resources, reset the system under test, and prepare for the next test case.
Repeat: Iterate through the cycle for each test case, focusing on a single behavior or scenario at a time until all requirements are met and the codebase is clean and well-tested.
Step-by-Step Approach
Let's break down the steps to follow when combining TDD with the API-first approach:
Review API Documentation: Start by thoroughly reviewing the API documentation, including Swagger specifications or any other relevant documentation.
Generate Test Cases: Utilize tools or frameworks to automatically generate test cases based on the API documentation. These tests should cover all endpoints, request/response scenarios, and error conditions. You can use tools like Postman, Rest-Assured, Karate, Jest, or Mocha for API testing. APICove Tools also offers a Test Case Generator that can create test cases from OpenAPI specifications. ๐
By using test automation frameworks, developers can automate the testing process, validate the API's behavior, and catch regressions early in the development cycle.
Set Up Initial Test Suite: Create an initial test suite based on the generated test cases. Write tests for basic functionality and edge cases, adhering to the AAA pattern and the Red-Green-Refactor cycle.
Implement API Endpoints: Begin implementing the API endpoints based on the API specifications. Use the TDD cycle to write just enough code to pass the existing tests. APICove Tools also offers a Code Generator that generates the endpoint code from OpenAPI specifications. ๐ช
Continuous Testing and Refinement: Continuously run the test suite to validate the implemented functionality and catch any regressions. Refactor code as needed to improve testability and maintainability.
Continuous Integration/Continuous Deployment (CI/CD) Pipelines: Integrate the API tests into your CI/CD pipelines to automate testing, deployment, and monitoring. Tools like Jenkins, Travis CI, CircleCI, or GitHub Actions can help automate the testing and deployment process.
API Documentation and Versioning: Keep the API documentation up to date with the latest changes and versions. Use tools like Swagger UI, ReDoc, Stoplight Elements, Redocusaurus, or Slate to generate interactive API documentation from OpenAPI specifications.
Benefits of TDD in API-First Development
Early Validation: TDD ensures that the API meets the specified requirements and behaves as expected from the outset of development.
Improved Code Quality: By writing tests first, developers are forced to think about the design and behavior of the API upfront, leading to cleaner, more modular, and better-tested code.
Reduced Bug Incidence: TDD helps catch bugs early in the development process, minimizing the likelihood of introducing defects into the API.
Enhanced Collaboration: With clear API specifications and comprehensive test suites, developers, testers, and stakeholders can collaborate more effectively, leading to better communication and alignment.
Increased Developer Productivity: TDD encourages developers to focus on writing code that fulfills the requirements, leading to faster development cycles and higher productivity.
Better Code Maintainability: Well-tested code is easier to maintain and refactor, as developers can make changes confidently without breaking existing functionality.
Faster Time-to-Market: By automating testing and ensuring the API's correctness early on, teams can accelerate the development process and deliver features to users more quickly.
Improved User Experience: A well-tested API results in a more reliable and consistent user experience, enhancing user satisfaction and loyalty.
Scalability and Interoperability: TDD promotes loose coupling and modular design, making it easier to scale the API and integrate it with other systems.
Cost Savings and Risk Mitigation: By catching bugs early and ensuring the API's correctness, teams can reduce development costs and mitigate risks associated with defects and failures.
Regulatory Compliance and Security: TDD helps ensure that the API complies with industry standards, regulations, and security best practices, reducing the risk of security vulnerabilities and compliance issues.
Continuous Improvement and Innovation: TDD fosters a culture of continuous improvement and innovation, as developers can iterate on the API design and functionality based on feedback and changing requirements. As explained above, the CI/CD pipelines can help automate the testing and deployment process, enabling teams to iterate quickly and deliver value to users.
Business Agility and Adaptability: TDD enables teams to respond to changing business requirements and market conditions more effectively, as they can quickly test and validate new features and enhancements. By embracing TDD, teams can adapt to evolving needs and stay ahead of the competition.
Competitive Advantage and Market Differentiation: A well-tested API that meets user needs and expectations can set your product apart from competitors, attracting more users and driving business growth. By delivering high-quality software solutions, you can establish a reputation for reliability and excellence in the market.
Customer Satisfaction and Loyalty: Ultimately, TDD helps ensure that the API delivers value to users, enhances their experience, and meets their needs. By prioritizing user satisfaction and loyalty, you can build a strong customer base and drive long-term success for your product.
Conclusion
By integrating Test-Driven Development into the API-first approach, developers can build robust, scalable, and well-tested APIs that form the foundation of successful software projects. By following the outlined steps and leveraging the power of TDD, teams can streamline their development process, mitigate risks, and deliver high-quality software solutions that meet the needs of their users.
Embrace the synergy between TDD and the API-first approach, and unlock the full potential of your development endeavors. Happy clean, reliable, and well-tested coding! ๐
Don't forget to give it a try to the APICove Tools to generate test cases and code from OpenAPI specifications. Let me know your thoughts and experiences in the comments below. I'd love to hear your feedback and insights on leveraging TDD in an API-first approach.
Subscribe to my newsletter
Read articles from La Rebelion Labs directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
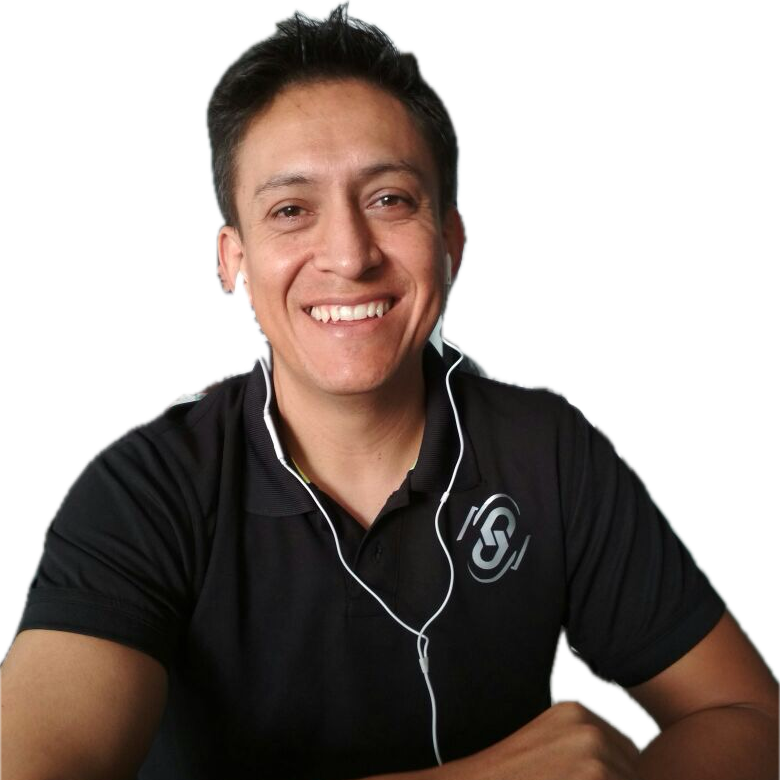
La Rebelion Labs
La Rebelion Labs
I was thinking about it, I feel that the concept of entrepreneurship is quite similar to the idea of being a Rebel. As a 'rebel,' I am passionate about experimenting and creating novel solutions to large problems.