Introduction to Angular CLI
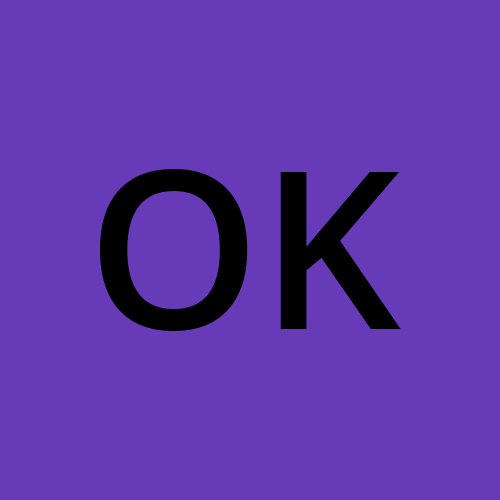
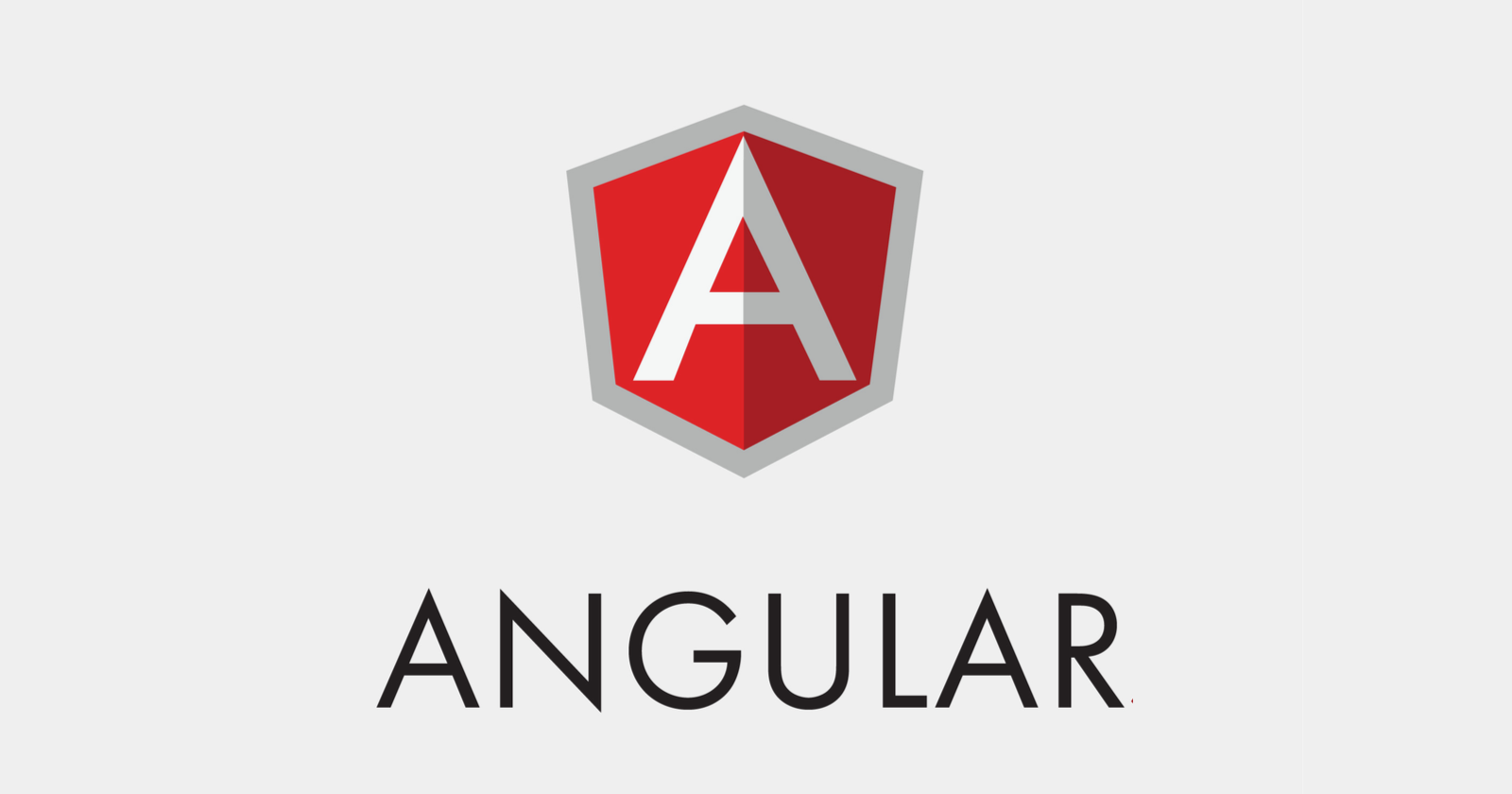
Angular stands out in the web development realm as a top framework for crafting dynamic, interactive applications. Its popularity stems from robust features, extensive tooling, and robust community support. At the core of Angular's ecosystem lies the Angular CLI, a potent command-line interface that simplifies and accelerates application development. Whether you're a seasoned pro or just starting, mastering the Angular CLI is crucial for boosting productivity. This article offers a beginner-friendly overview of the Angular CLI, covering its features, installation, basic usage, and more. By the end, you'll be equipped to leverage the Angular CLI to enhance your development workflow.
What is the Angular CLI?
The Angular CLI, or Command Line Interface, is a robust tool created by the Angular team to simplify the development of Angular applications. It's a command-line utility designed to automate tasks like setting up projects, generating code, testing, and deploying Angular applications.
Role in Angular Development
The Angular CLI greatly simplifies Angular development by streamlining complex tasks and providing developers with a standardized workflow. Here's a breakdown of its key functions:
Project Setup: Angular CLI swiftly sets up new Angular projects by creating a predefined directory structure and configuration. It generates essential files like
app.module.ts
,main.ts
, andangular.json
, allowing developers to start building features immediately without the hassle of manual project setup.Code Generation: Developers can easily generate Angular components, services, modules, directives, and other elements using simple commands with Angular CLI. This eliminates the need for manual file creation and configuration, saving time and reducing potential errors.
Development Server: Angular CLI features a built-in development server that enables developers to run their Angular applications locally during development. By using the
ng serve
command, developers can quickly preview changes, benefit from automatic live reloading, and debug their applications in real time.Build and Optimization: Angular CLI automates the process of preparing Angular applications for deployment. By executing the
ng build
command, developers can generate optimized bundles of their application code, assets, and dependencies, ready to be deployed to a web server or hosting platform.Testing and Quality Assurance: Angular CLI seamlessly integrates with popular testing frameworks such as Jasmine and Karma, facilitating the writing and execution of unit tests and end-to-end tests. Additionally, it offers built-in support for code linting and formatting, ensuring consistent code quality across the project.
Importance for Streamlining Development Workflows
The Angular CLI plays a crucial role in streamlining development workflows by providing a standardized and efficient approach to building Angular applications. Here's why it is indispensable for developers:
Consistency: By enforcing a consistent project structure and coding conventions, the Angular CLI promotes best practices and ensures uniformity across projects, making it easier for team members to collaborate and maintain codebases.
Productivity: With its intuitive commands and automation capabilities, the Angular CLI significantly reduces repetitive tasks and boilerplate code, allowing developers to focus on implementing features and delivering value to end-users faster.
Ease of Adoption: The Angular CLI abstracts away the complexities of configuring build tools and setting up development environments, making Angular more accessible to developers of all skill levels. This lowers the barrier to entry and accelerates the onboarding process for new team members.
Getting Started with the Angular CLI
Starting with the Angular CLI is straightforward due to its user-friendly commands and efficient project setup. Here, we'll guide you through creating a new Angular project using the ng new
command, clarify the project structure generated by the Angular CLI, and give an overview of the main files and directories created.
To initiate a new Angular project, open your terminal or command prompt and execute the following command:
ng new <file name>
Replace <file name>
with your desired name for the Angular application. This command will establish a new Angular project within a directory bearing the specified name, and configure essential files and dependencies.
Angular Project Structure
Upon running the ng new command, the Angular CLI generates a standard project structure for your Angular application. Here's an overview of the main directories and files created:
e2e/
: This directory contains end-to-end tests for your application. It is used for testing the application's behavior from the user's perspective. The tests are written using tools like Protractor and Jasmine.node_modules/
: This directory contains all the dependencies of your Angular project. It is created and managed by npm (Node Package Manager) and should not be modified manually.src/
: This directory is the heart of your Angular application and contains all the source code, assets, and configuration files. Here's a breakdown of the main files and directories withinsrc/
:app/
: This directory contains the components, services, modules, and other Angular artifacts that comprise your application. The main component (app.component.ts
), along with its HTML template (app.component.html
) and styles (app.component.css
), are located here.assets/
: This directory is used to store static assets such as images, fonts, and other files that are referenced in your application.environments/
: This directory contains environment-specific configuration files, such asenvironment.ts
andenvironment.prod.ts
, which define settings for development and production environments, respectively.index.html
: This file is the main HTML file of your Angular application and serves as the entry point for the browser. It contains the<app-root>
tag, which is the root component of your application.styles.css
: This file contains global CSS styles that are applied to your entire application.
angular.json
: This file is the Angular CLI configuration file and contains project-specific configuration settings such as build options, file paths, and dependencies.package.json
andpackage-lock.json
: These files contain metadata and dependencies for your Angular project. They are used by npm to manage project dependencies and scripts.
Serving the Angular Application
Once you've set up your Angular project using the Angular CLI, the next step is to serve your application locally for development and testing purposes. In this section, we'll explore how to accomplish this using the ng serve
command, along with options for configuring the development server and accessing the locally served application in the web browser.
To start the development server and serve your Angular application locally, navigate to your project directory in the terminal or command prompt and run the following command:
ng serve
This command will compile your Angular application and launch a development server, making your application accessible at http://localhost:4200 by default.
Options for Configuring the Development Server
The ng serve
command offers various options to customize the development server according to your requirements. Some commonly used options include:
Port Number (
--port
or-p
): You can set a specific port for the development server using the--port
option followed by the desired port number. For example:ng serve --port 3000
Live Reload (
--live-reload
or-lr
): By default, the development server enables live reloading, which automatically refreshes the browser when code changes are made. You can turn off live reloading with the--no-live-reload
option. For example:ng serve --no-live-reload
Proxy Configuration (
--proxy-config
or-pc
): If your Angular application needs to communicate with a backend server hosted on a different domain or port, you can set up a proxy to handle these requests during development. Specify a proxy configuration file using the--proxy-config
option. For example:ng serve --proxy-config proxy.conf.json
Note: You must create a
proxy.conf.json
file with the required proxy configuration settings.Once the development server is operational, you can view your Angular application in a web browser by entering http://localhost:4200 (or a custom port if specified) into the address bar.
Additional Features and Commands
In addition to its core functionalities for project setup, code generation, and serving applications, the Angular CLI offers a range of useful features and commands to enhance the development experience and maintain code quality. In this section, we'll explore some of these features and commands, including testing, linting, internationalization, and project management.
Testing
The Angular CLI provides built-in support for testing Angular applications using popular testing frameworks like Jasmine and Karma. Developers can generate unit tests and end-to-end tests for their components and services, and run them using simple commands.
Unit Tests: Generate unit tests for components or services with
ng generate component
orng generate service
followed by the--spec
flag. For example:ng generate component my-component --spec
End-to-end Tests: End-to-end tests are located in the
e2e
/ directory of your Angular project. You can run them using theng e2e
command, which launches the Protractor test runner.
Linting
Linting helps maintain code quality and enforce coding standards across projects. The Angular CLI integrates with popular linting tools like ESLint and TSLint to analyze and report code issues.
Running Linting Checks: To run linting checks on your Angular project, use the
ng lint
command. By default, it uses TSLint to analyze TypeScript files and provides feedback on potential issues.Customizing Linting Rules: You can customize linting rules by modifying the
tslint.json
file in your project. This allows you to tailor linting rules to match your team's coding standards and preferences.
Internationalization (i18n)
Internationalization support enables developers to create multilingual applications with ease. The Angular CLI provides tools for extracting and managing translation messages in your application.
Extracting Translation Messages: To extract translation messages from your Angular application, use the
ng extract-i18n
command. This command generates a translation file (.xlf
or.xliff
) containing the extracted messages.Managing Translations: Once translation messages are extracted, developers can use tools like
@angular/localize
to manage translations and localize their applications for different languages and locales.
Project Management
The Angular CLI offers additional commands for managing Angular projects, updating dependencies, and performing various maintenance tasks.
Updating Dependencies: The
ng update
command allows you to update dependencies and migrate your project to newer versions of Angular and related packages. It automatically analyzes your project and suggests updates based on compatibility and best practices.Running Custom Schematics: Schematics are code generators that can be used to scaffold and modify code in Angular projects. You can create and run custom schematics using the
ng generate
command with theschematic
option.
Conclusion
The Angular CLI is a vital tool for Angular developers, streamlining workflows and boosting productivity and code quality. It automates tasks like project setup, code generation, testing, and deployment, freeing up developers to focus on creating innovative features. With standardized processes and intuitive commands, it promotes consistency and empowers developers of all skill levels. The Angular CLI isn't just convenient; it's essential for modern Angular development, helping developers unlock the framework's full potential and build outstanding web applications efficiently.
Subscribe to my newsletter
Read articles from Okere Kelvin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
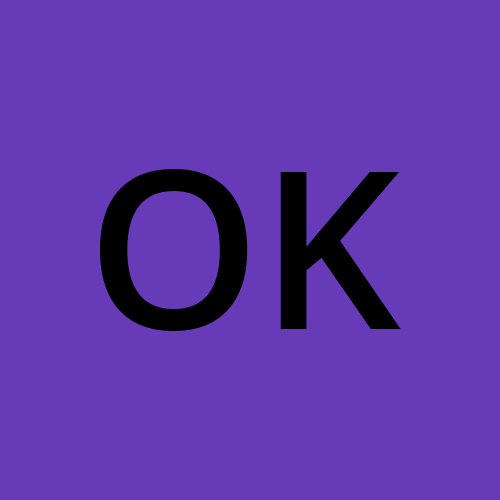