Unraveling the Mysteries of Numerical Computing with Numpy
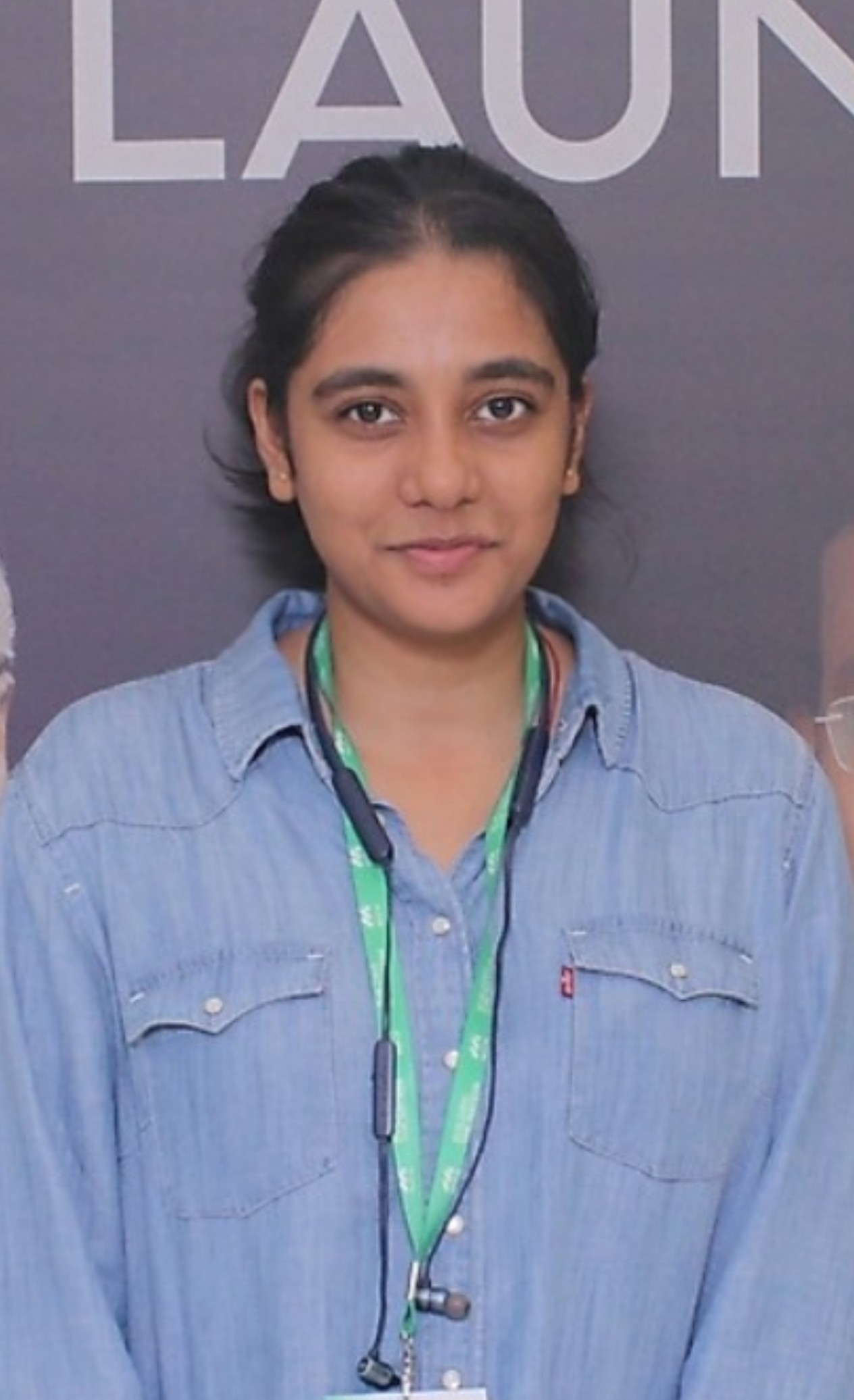
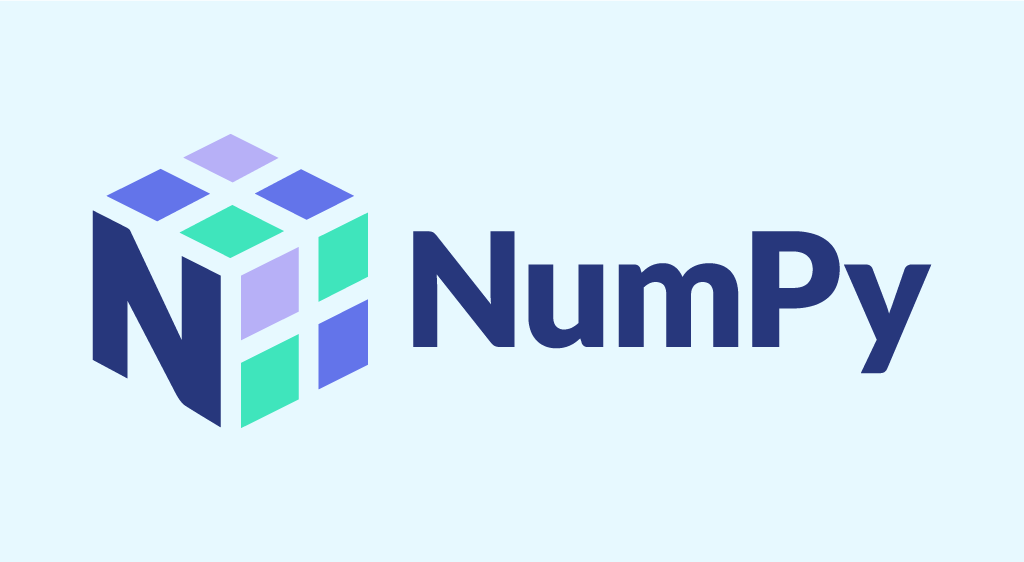
Introduction:
In the realm of numerical computing, efficiency and performance are paramount. Python, with its rich ecosystem of libraries, offers a powerful toolset for tackling complex mathematical computations. At the heart of this ecosystem lies Numpy, a fundamental library that revolutionizes numerical operations with its array-oriented computing capabilities. In this blog post, we'll embark on a journey to explore the depths of Numpy, uncovering its inner workings and showcasing its versatility through practical examples and code snippets.
Understanding the Array:
At the core of Numpy lies the ndarray, a powerful data structure that enables efficient storage and manipulation of arrays. Unlike traditional Python lists, ndarrays are homogeneous containers that facilitate vectorized operations, thereby enhancing performance and scalability. Let's delve into the anatomy of ndarrays and discover how they underpin Numpy's functionality.
import numpy as np
# Creating ndarrays
arr1d = np.array([1, 2, 3, 4, 5])
arr2d = np.array([[1, 2, 3], [4, 5, 6]])
# Array attributes
print("Shape of arr1d:", arr1d.shape)
print("Shape of arr2d:", arr2d.shape)
print("Data type of arr1d:", arr1d.dtype)
print("Data type of arr2d:", arr2d.dtype)
Output:
Shape of arr1d: (5,)
Shape of arr2d: (2, 3)
Data type of arr1d: int64
Data type of arr2d: int64
Efficient Array Operations:
One of Numpy's key strengths lies in its ability to perform element-wise operations efficiently through vectorization. By leveraging optimized C implementations under the hood, Numpy eliminates the need for explicit looping in Python, leading to significant performance gains.
Let's illustrate the concept of vectorization with a practical example.
# Element-wise addition
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
result = arr1 + arr2
print("Element-wise addition:", result)
Output:
Element-wise addition: [5 7 9]
Broadcasting:
Extending the Power of Arrays Numpy's broadcasting mechanism enables arrays of different shapes to be combined seamlessly in arithmetic operations. This powerful feature simplifies code and enhances readability by eliminating the need for manual array manipulation.
Let's explore broadcasting through a hands-on example.
# Broadcasting example
arr = np.array([[1, 2, 3], [4, 5, 6]])
scalar = 2
result = arr * scalar
print("Broadcasting result:")
print(result)
Output:
Broadcasting result:
[[ 2 4 6]
[ 8 10 12]]
Advanced Operations:
Exploring Beyond the Basics Beyond basic arithmetic operations, Numpy offers a plethora of advanced functions for linear algebra, random number generation, and more. From matrix multiplication to singular value decomposition, Numpy's comprehensive library of functions caters to diverse mathematical needs.
Let's dive into some advanced operations with Numpy.
# Advanced operations
# Matrix multiplication
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6], [7, 8]])
result = np.dot(matrix1, matrix2)
print("Matrix multiplication result:")
print(result)
Output:
Matrix multiplication result:
[[19 22]
[43 50]]
Conclusion:
Numpy stands as a testament to the power of open-source collaboration and innovation. By providing a robust foundation for numerical computing in Python, Numpy empowers scientists, engineers, and researchers to tackle complex problems with ease and efficiency.
Whether you're crunching numbers, analyzing data, or exploring mathematical concepts, Numpy serves as your steadfast companion on the journey of discovery. As we've witnessed, its array-oriented computing paradigm, combined with vectorization and broadcasting, revolutionizes the way we approach numerical computations. So, embrace the elegance of Numpy, and unlock new possibilities in the world of numerical computing.
Subscribe to my newsletter
Read articles from Ishika Ishani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
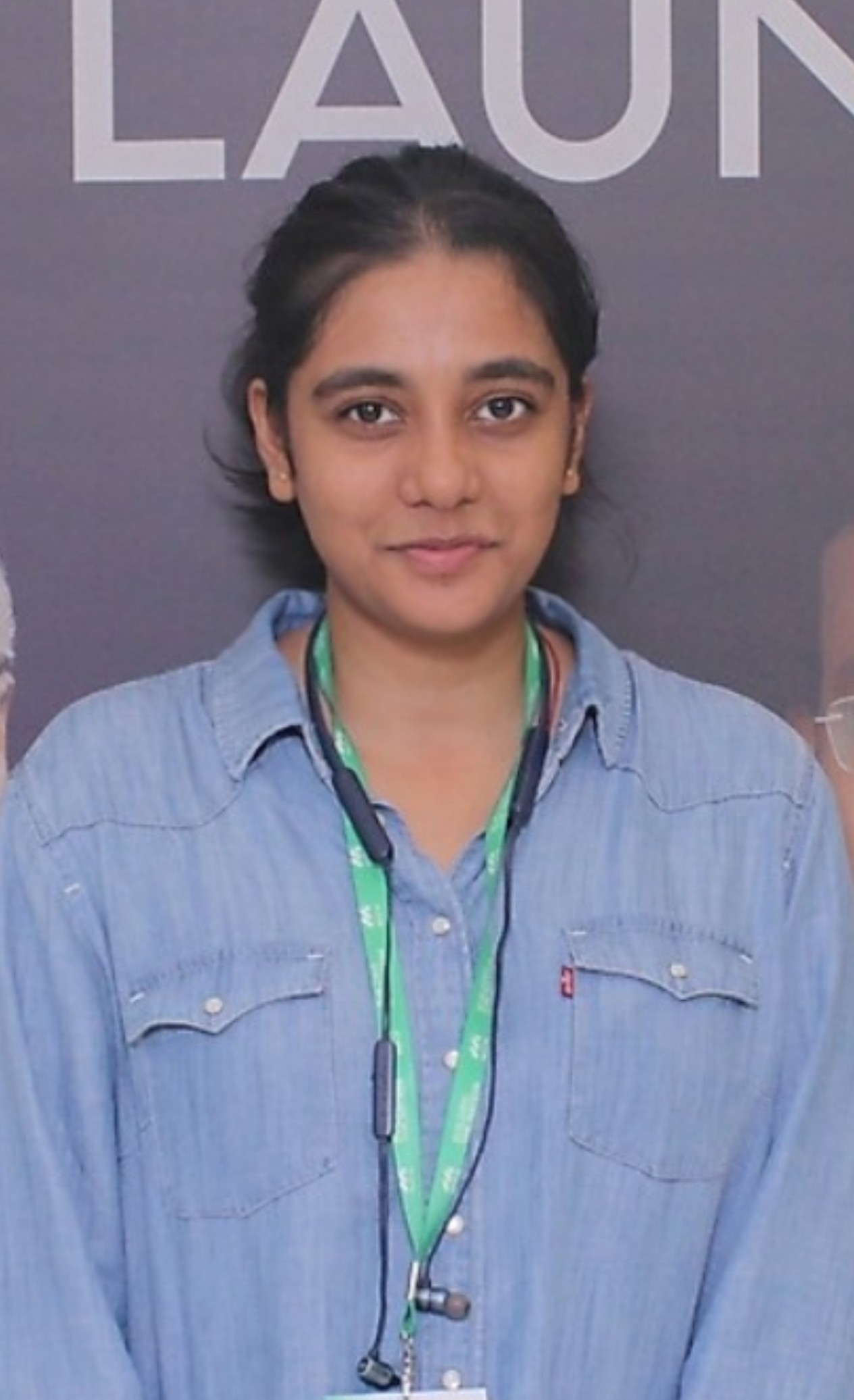
Ishika Ishani
Ishika Ishani
I am a versatile developer skilled in web development and data science. My expertise includes HTML, CSS, JavaScript, TypeScript, React, Python, Django, Machine Learning, and Data Analysis. I am currently expanding my knowledge in Java and its applications in software development and data science. My diverse skill set enables me to create dynamic web applications and extract meaningful insights from data, driving innovative solutions.