Mastering Data Analysis with Pandas:
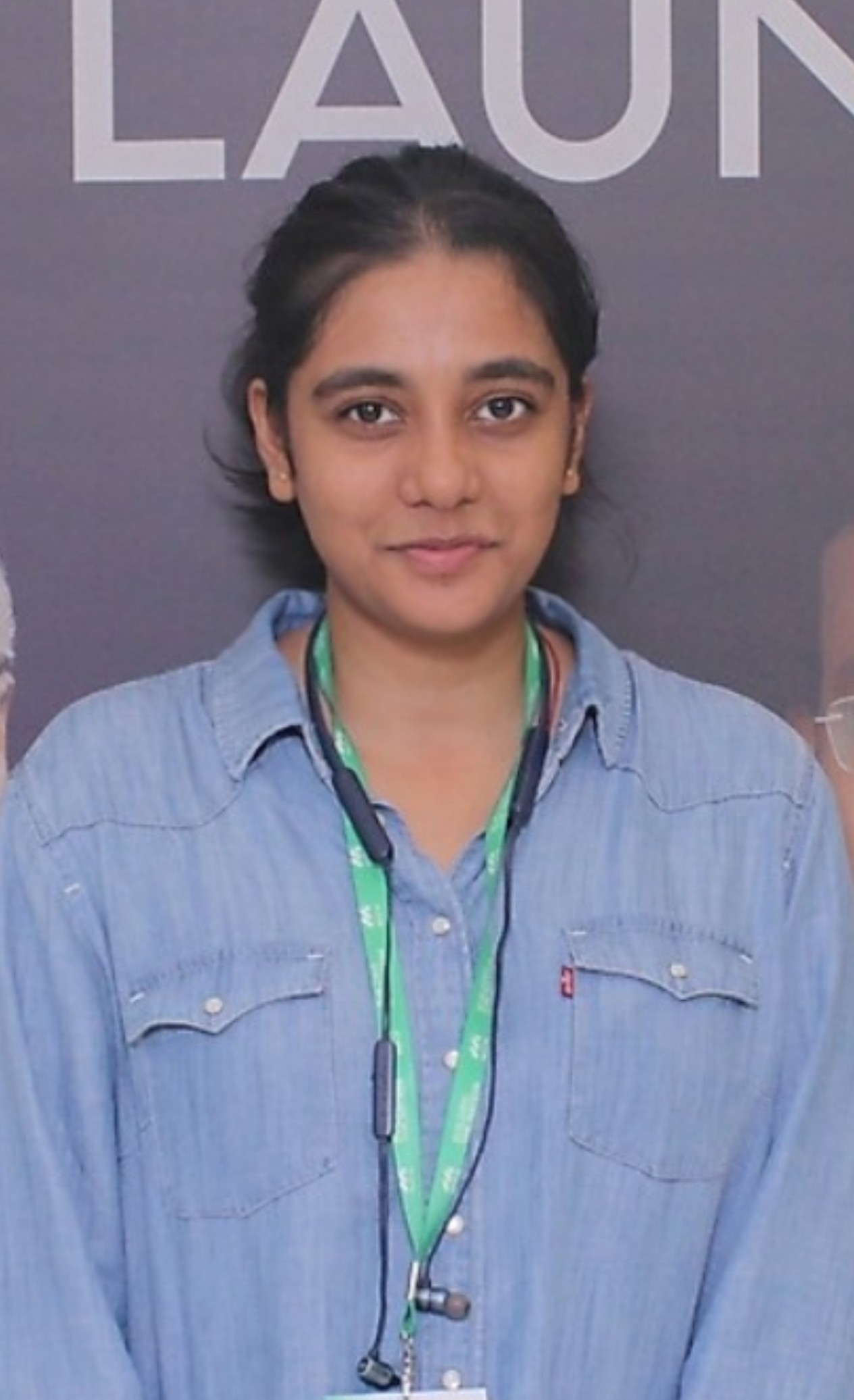

Introduction:
In the vast landscape of data analysis, the ability to efficiently manipulate and analyze data is paramount. Enter Pandas, a powerful Python library that simplifies the entire data analysis workflow, from data loading to transformation and visualization. In this blog post, we'll embark on a journey to unravel the capabilities of Pandas, exploring its versatile data structures and powerful functionalities through practical examples and code snippets.
Understanding the DataFrame:
The Backbone of Pandas At the heart of Pandas lies the DataFrame, a tabular data structure that seamlessly handles heterogeneous data with ease. With rows and columns akin to a spreadsheet, DataFrames empower users to perform a myriad of data manipulation tasks efficiently.
Let's dive into the anatomy of DataFrames and explore their functionalities.
import pandas as pd
# Creating a DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'Salary': [50000, 60000, 70000]}
df = pd.DataFrame(data)
print("DataFrame:")
print(df)
Output:
Name Age Salary
0 Alice 25 50000
1 Bob 30 60000
2 Charlie 35 70000
Efficient Data Manipulation:
Harnessing the Power of Pandas Pandas provides a rich set of functions for data manipulation, ranging from basic operations like filtering and sorting to advanced techniques like grouping and aggregation. Leveraging Pandas' intuitive API, users can streamline their data analysis tasks with ease.
Let's demonstrate some common data manipulation operations using Pandas.
# Filtering data
filtered_df = df[df['Age'] > 25]
print("Filtered DataFrame:")
print(filtered_df)
# Grouping and aggregation
grouped_df = df.groupby('Age').mean()
print("Grouped DataFrame:")
print(grouped_df)
Output:
Filtered DataFrame:
Name Age Salary
1 Bob 30 60000
2 Charlie 35 70000
Grouped DataFrame:
Age Salary
25 50000
30 60000
35 70000
Data Visualization:
Unleashing Insights with Pandas Visualization plays a crucial role in data analysis, enabling users to gain insights and communicate findings effectively. Pandas seamlessly integrates with Matplotlib, allowing users to create insightful visualizations directly from DataFrames.
Let's explore how Pandas facilitates data visualization through a practical example.
import matplotlib.pyplot as plt
# Creating a bar plot
df.plot(x='Name', y='Salary', kind='bar', legend=False)
plt.title('Salary Distribution')
plt.xlabel('Name')
plt.ylabel('Salary')
plt.show()
Conclusion:
Pandas stands as a cornerstone in the realm of data analysis, empowering users to wrangle, manipulate, and analyze data effortlessly. Whether you're a data scientist exploring complex datasets or a business analyst extracting insights from spreadsheets, Pandas offers a robust toolkit for your data analysis needs.
By mastering Pandas' versatile data structures and powerful functionalities, you unlock new possibilities in the world of data analysis. So, embrace the power of Pandas, and embark on a journey of discovery in the realm of data analysis.
Subscribe to my newsletter
Read articles from Ishika Ishani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
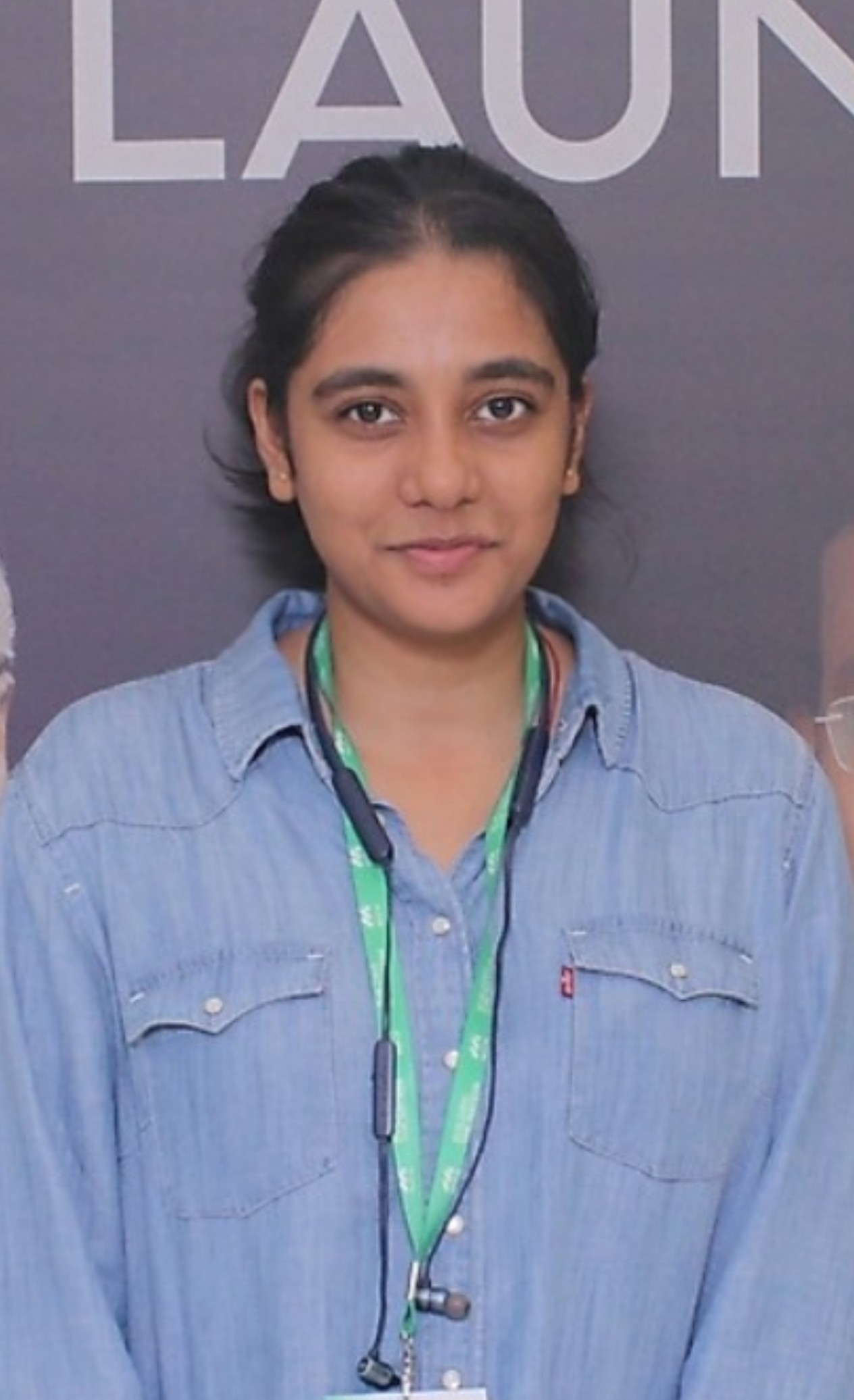
Ishika Ishani
Ishika Ishani
I am a versatile developer skilled in web development and data science. My expertise includes HTML, CSS, JavaScript, TypeScript, React, Python, Django, Machine Learning, and Data Analysis. I am currently expanding my knowledge in Java and its applications in software development and data science. My diverse skill set enables me to create dynamic web applications and extract meaningful insights from data, driving innovative solutions.