React Data Fetching: Exploring Diverse Methods
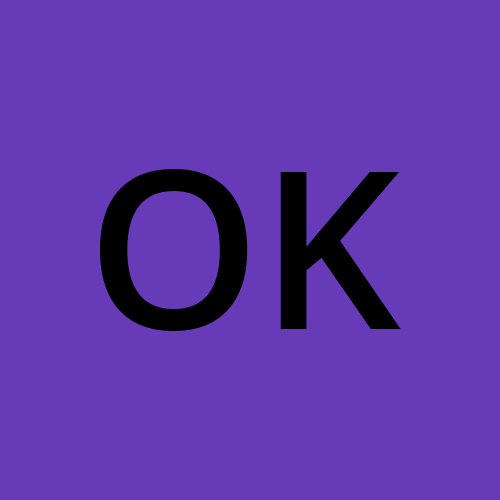
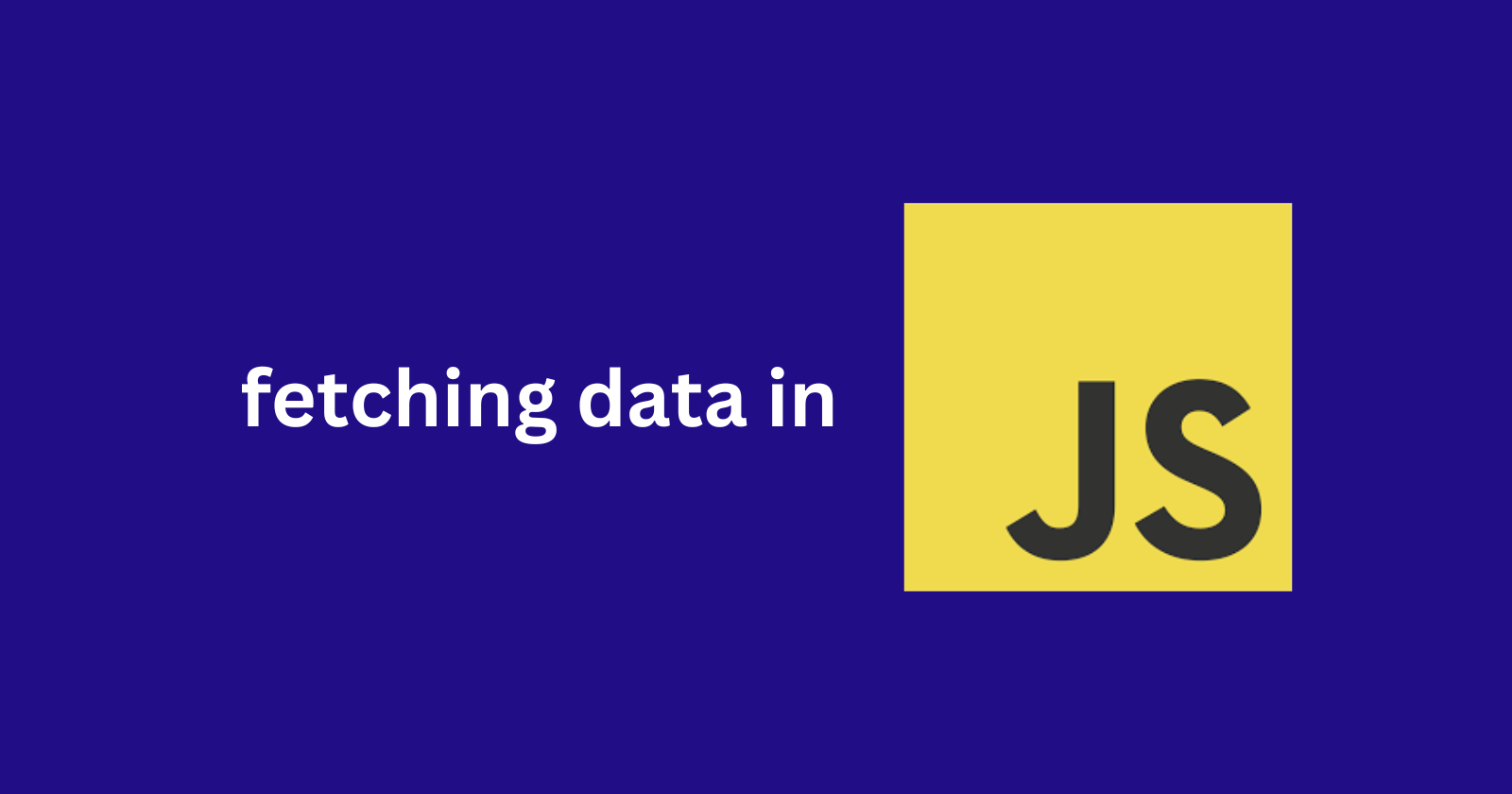
Data fetching is essential in modern web development for creating dynamic and interactive applications. In the React ecosystem, developers have various methods for efficiently fetching data, ranging from the traditional Fetch API to modern solutions like React Query. This article explores these methods to help developers understand how to fetch data effectively in React applications.
Overview of Data Fetching in React
In React, data fetching involves obtaining information from external sources like APIs or databases and displaying it within React components. React offers various methods for data fetching, such as the built-in Fetch API, libraries like Axios, and modern solutions like React Query and SWR. Each method has its strengths and specific use cases, empowering developers to select the most suitable approach for their application's needs.
Efficient data fetching is paramount in React applications to ensure optimal performance and user experience. Ineffective data fetching can result in slow load times, increased network traffic, and unresponsive interfaces, ultimately leading to a diminished user experience.
We can categorize data fetching techniques into two main groups: Built-in Fetching Methods, which encompass tools like the Fetch API and Axios, and Third-party Libraries for Advanced Data Fetching, which include solutions like React Query and SWR.
Setting the Development environment
To get started, let's create a new React application. You can do this by using the following command in your terminal and replacing <file name>
with your preferred name:
npx create-react-app <file name>
Once the app is created, navigate to the newly generated directory:
cd <file name>
Now, open the project in Visual Studio Code:
code .
Next, within the src
directory, create a new folder named FetchData
. Inside this folder, create a file named FetchData.js
.
In your index.js
file, import the FetchData
component:
import FetchData from "./FetchData";
To render the FetchData
component to the DOM, replace <App />
with <FetchData />
:
import React from 'react';
import ReactDOM from 'react-dom';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<FetchData />
</React.StrictMode>
);
Using the Fetch API
The Fetch API is a built-in web API provided by modern browsers for making HTTP requests. It provides a native way to fetch resources from servers and handle responses in JavaScript. In React applications, the Fetch API is often used to retrieve data from external APIs or backend servers.
The advantage over other methods
Native Browser Support: The Fetch API is supported natively by modern browsers, eliminating the need for external libraries or dependencies.
Simplicity and Consistency: The Fetch API provides a simple and consistent interface for making HTTP requests, making it easy to understand and use in React applications.
Promise-Based: Fetch requests return Promises, allowing developers to use modern JavaScript syntax like async/await for asynchronous operations.
Modern Standard: As a part of the web platform standard, the Fetch API is actively maintained and updated, ensuring compatibility with future browser versions and standards.
Implementing Fetch API to get data
Start by creating a React function component named FetchData
and exporting it. Import React and define the component as follows:
import React from 'react';
function FetchData() {
return (
<div></div>
);
}
export default FetchData;
Afterward, utilize the useState
hook to define a state variable named records
in the FetchData
method. This variable will store the data fetched from the API.
const [records, setRecords] = useState([]);
In our React component, we utilize the useEffect
hook to handle side effects. Specifically, we aim to retrieve data when the component is mounted. To achieve this, we employ the useEffect
hook with an empty dependency array ([]
) as its second argument.
import React, { useEffect, useState } from 'react';
function FetchData() {
const [records, setRecords] = useState([]);
useEffect(() => {
// Fetch data here
}, []);
return (
<div></div>
);
}
export default FetchData;
In the useEffect
hook, we utilize the fetch
API to perform a GET
request to retrieve data from a specific URL. In this case, we're fetching data from the /users
endpoint of the JSONPlaceholder API. Upon receiving a response from the API, we employ the .json()
method to parse the response into JSON format. Subsequently, we update the state variable records
with the fetched data by utilizing the setRecords
function. Additionally, we include a .catch()
block to handle any potential errors that might occur during the fetch operation.
useEffect(() => {
fetch("https://jsonplaceholder.typicode.com/users")
.then((response) => response.json())
.then((data) => setRecords(data))
.catch((err) => console.log(err));
}, []);
In the final step of our process, we display the retrieved data within the user interface (UI) of our application. This is achieved by iterating over the records
array and generating a list item for each record.
return (
<div>
<ul>
{records.map((record, index) => (
<li key={index}>
{record.id} | {record.name} | {record.email}
</li>
))}
</ul>
</div>
);
By executing this code, we effectively render the data fetched from an external API directly into our React component. Below, you can see the data being presented within our application.
Axios library
Axios is a popular JavaScript library for making HTTP requests in both the browser and Node.js environments. It provides a simple and intuitive API for performing asynchronous operations, including data fetching, with built-in support for features like request and response interception, request cancellation, and more. In React applications, Axios is commonly used as an alternative to the Fetch API for handling data fetching tasks.
The advantage over other methods
Simplified API: Axios offers a simpler and more intuitive API compared to the Fetch API, making it easier for developers to perform common HTTP operations.
Request Interceptors: Axios allows developers to intercept and modify HTTP requests and responses, enabling features like adding headers, logging, or global error handling.
Response Interceptors: Similarly, Axios provides response interceptors for modifying responses before they are passed to the
.then()
or.catch()
handlers.Request Cancellation: Axios supports request cancellation, allowing developers to cancel pending requests when they are no longer needed. This can help improve performance and reduce unnecessary network traffic.
Cross-Browser Compatibility: Axios abstracts away differences in browser implementations, providing a consistent interface for making HTTP requests across different environments.
While Axios offers several advantages over the Fetch API, it's important to note that it introduces an additional dependency on the project.
Fetching data with Axios
To integrate Axios into a React project, begin by installing it through npm:
To do this, execute the following command:
npm install axios
Upon successful installation, import Axios into React components as you would with any other module:
import axios from 'axios';
Utilize the useEffect
hook to execute code after the component renders. Within this hook, we use Axios, to perform a GET request to the designated URL: "
https://jsonplaceholder.typicode.com/users
"
.
The axios.get
function returns a Promise, enabling us to handle the response or any potential errors with .then()
and .catch()
methods respectively.
Inside the .then()
method, we access the response data via response.data
, representing an array of user records retrieved from the API. Subsequently, we employ the setRecords
function to update the component's state with the fetched data, prompting a re-render with the updated information.
Should an error occur during the request, the .catch()
method is triggered, logging the error object to the console along with an informative message.
import React, { useState, useEffect } from 'react';
import axios from 'axios';
function FetchData() {
const [records, setRecords] = useState([]);
useEffect(() => {
axios
.get("https://jsonplaceholder.typicode.com/users")
.then((response) => {
setRecords(response.data);
})
.catch((error) => {
console.log("Error fetching data:", error);
});
}, []);
return (
<div>{/* Render the fetched data here */}</div>
);
}
export default FetchData;
Lastly, we display the fetched data in the UI, similar to our previous approach using the fetch method.
React Query
React Query is a library for handling and caching asynchronous data within React applications. It streamlines the processes of data fetching, caching, synchronization, and updating through its declarative API and robust caching system. Unlike other libraries, React Query is designed to be compatible with various frameworks, making it adaptable for integration into any JavaScript project, including those built with React.
The advantage over other methods
Simplified Data Fetching: React Query simplifies data fetching in React applications by abstracting away complex logic and boilerplate code, resulting in cleaner and more maintainable codebases.
Automatic Caching and Invalidation: React Query's built-in caching and invalidation mechanisms eliminate the need for manual data management, reducing the risk of stale data and improving performance.
Data Synchronization Across Components: React Query facilitates seamless data synchronization across components, ensuring consistency and coherence in the UI.
Comprehensive Features: React Query offers a comprehensive set of features, including pagination, prefetching, optimistic updates, and more, making it a versatile solution for various data fetching scenarios.
Framework-Agnostic: React Query is framework-agnostic, meaning it can be used in any JavaScript project, not just React applications. This flexibility makes it suitable for a wide range of use cases and development environments.
Fetching data with React Query
To integrate React Query into your React project, start by installing it using npm.
npm install react-query
Once React Query is installed, you can incorporate it into your FetchData.js
file by importing the useQuery
hook.
import { useQuery } from 'react-query';
In the FetchData
function, use the useQuery
hook from React Query, and pass it a unique key "users"
to identify the query. Additionally, include a second argument, which should be a function responsible for fetching the data using the Fetch API. This function should return a Promise resolving to the fetched data.
// Utilizing the useQuery hook from React Query to retrieve data
const { data: records, isLoading, isError } = useQuery("users", () =>
// Performing a GET request to the JSONPlaceholder API's '/users' endpoint
fetch('https://jsonplaceholder.typicode.com/users').then((res) =>
res.json() // Parsing the response as JSON
)
);
When the data is being fetched, indicated by the isLoading
state being true, a "Loading..." message is displayed to inform the user that a process is ongoing in the background. In case an error occurs during the fetching process, denoted by the isError
state being true, an "Error fetching data" message is displayed to notify the user of the issue.
if (isLoading) {
return <div>Loading...</div>;
}
if (isError) {
return <div>Error fetching data</div>;
}
Render the fetched data in the UI as done previously. Here’s the FetchData.js
file for reference.
function FetchData() {
// Using the useQuery hook from React Query to fetch data
const { data: records, isLoading, isError } = useQuery("users", () =>
// Making a GET request to the JSONPlaceholder API's '/users' endpoint
fetch('https://jsonplaceholder.typicode.com/users').then((res) =>
res.json() // Parsing the response as JSON
)
);
// Displaying loading message while data is being fetched
if (isLoading) return <div>Loading...</div>;
// Displaying error message if an error occurs during fetching
if (isError) return <div>Error fetching data</div>;
// Once data is fetched successfully, rendering the data in the UI
return (
<div>
{/* Render the fetched data here as we did previously */}
</div>
);
}
Navigate to the index.js
file and import QueryClient
and QueryClientProvider
.
import { QueryClient, QueryClientProvider } from "react-query"
Create an instance of QueryClient
.
const queryClient = new QueryClient();
To ensure the fetch data component functions correctly, it needs to be enclosed within a QueryClientProvider
component. This ensures that the necessary data querying functionalities are available to it. The QueryClientProvider
component requires the client
prop to be passed, which should be an instance of QueryClient
that you created earlier.
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<QueryClientProvider client={queryClient}>
<FetchData />
</QueryClientProvider>
</React.StrictMode>
);
Once implemented, you'll be able to view the fetched data in your browser.
SWR (Stale-While-Revalidate)
SWR (stale-while-revalidate) is a React hook library for data fetching developed by Vercel. It provides a simple and efficient way to fetch data, manage caching, and synchronize data across components in React applications. SWR is designed to improve the user experience by displaying stale data immediately while fetching the latest data in the background.
The advantage over other methods
Simple and Intuitive API: SWR offers a simple and intuitive API for data fetching and caching, reducing boilerplate code and making data management easier.
Automatic Caching and Stale-While-Revalidate Strategy: SWR's automatic caching and stale-while-revalidate strategy improve performance and reduce network traffic, resulting in faster and more responsive applications.
Data Synchronization Across Components: SWR ensures consistent and coherent data across components, simplifying state management and reducing the risk of inconsistency bugs.
Built-in Error Handling: SWR provides built-in error handling capabilities, allowing developers to handle errors gracefully and provide informative error messages to users.
Framework Agnostic: SWR is framework-agnostic, meaning it can be used in any JavaScript project, not just React applications. This flexibility makes it suitable for a wide range of use cases and development environments.
Fetching data with SWR
To integrate swr
into your React project, begin by installing using the command below.
npm install swr
After installation, import SWR
into the FetchData.js
file of your project:
import useSWR from 'swr';
In the FetchData
method, use the useSWR
hook provided by the SWR library to fetch data from the specified URL: "https://jsonplaceholder.typicode.com/users". The useSWR
hook takes two arguments:
The first argument is the key, which uniquely identifies the data being fetched. In this case, it's the URL of the API endpoint.
The second argument is a function that fetches the data. Inside this function, we use the fetch API to make a
GET
request to the URL and parse the response as JSON.
The useSWR
hook returns an object containing the fetched data (records
) and an error (error
). We check if an error occurred during the fetch operation. If an error is present, we render an error message. Additionally, we check if the data is not yet available (!records
), indicating that the fetch operation is still in progress. In this case, we render a loading indicator.
function FetchData() {
// Utilizing SWR to fetch data
const { data: records, error } = useSWR('https://jsonplaceholder.typicode.com/users',
url => fetch(url).then(response => response.json())
);
if (error) return <div>Error fetching data</div>;
if (!records) return <div>Loading...</div>;
return (
<div>
{/* Render the fetched data here as we did previously */}
</div>
);
}
export default FetchData;
We can see the data it returned is the same as the one for the fetch method.
Conclusion
Mastering data fetching in React is crucial for delivering efficient and engaging web applications. By exploring various methods such as the Fetch API, Axios, React Query, and SWR, developers can leverage a diverse toolkit to fetch, manage, and display data effectively.
Subscribe to my newsletter
Read articles from Okere Kelvin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
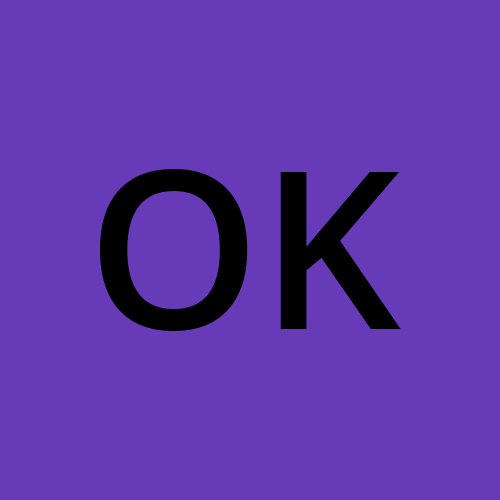