Building Great APIs: A Foundation in Design Principles
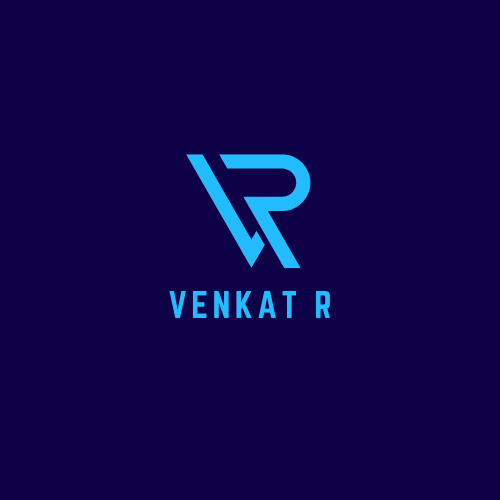
APIs, or Application Programming Interfaces, are the workhorses of the modern software world. They power communication between different applications, services, and devices, enabling seamless data exchange and functionality sharing. But just like any good tool, a well-designed API is essential for smooth operation and widespread adoption. Here, we delve into four fundamental principles of API design: simplicity, consistency, flexibility, and discoverability, providing practical examples and tips to guide you in crafting exceptional APIs.
1. Simplicity: Keep it Clean and Clear
Imagine an API with convoluted endpoints, cryptic error messages, and an inconsistent data format. Not a pretty picture, right? Simplicity is paramount. Users should be able to grasp the core functionality of the API quickly. Here's how to achieve simplicity:
Resource-oriented design: Structure your API around clear resources, like users, products, or orders. Each resource should have well-defined endpoints for CRUD (Create, Read, Update, Delete) operations.
Intuitive URLs: Use descriptive and human-readable URLs that map to the resources they represent. For instance, /users to get a list of users or /products/{id} to retrieve a specific product by its ID.
Meaningful HTTP verbs: Leverage standard HTTP verbs like GET, POST, PUT, and DELETE to indicate the desired action on a resource. This aligns with developer expectations and reduces confusion.
Example (Python using Flask):
from flask import Flask, jsonify, request
app = Flask(__name__)
User resource with CRUD operations
users = []
@app.route('/users', methods=['GET'])
def get_users():
return jsonify(users)
@app.route('/users', methods=['POST'])
def create_user():
... process user creation logic
return jsonify({'message': 'User created successfully!'})
... similar routes for user update and deletion
2. Consistency: Predictability is Key
Imagine an API that throws random error codes or uses different data formats for similar responses. Consistency builds trust and reduces development time. Here's how to ensure consistency:
Uniform naming conventions: Maintain consistent naming for resources, parameters, and response structures throughout the API. This improves readability and reduces the learning curve for developers.
Standardized error handling: Use a defined set of error codes and response formats to communicate errors. This allows developers to handle errors gracefully and provide meaningful feedback to users.
Versioning: If your API evolves over time, implement versioning to allow developers to continue using older versions while you introduce new features.
Example (Error Response Format):
JSON
{
"error": {
"code": 400,
"message": "Invalid request parameter: name"
}
}
3. Flexibility: Cater to Diverse Needs
A one-size-fits-all approach rarely works in the API world. Your API should be adaptable to accommodate various use cases and future growth. Here's how to design for flexibility:
Optional parameters: Allow developers to specify optional parameters for filtering or sorting results. This caters to specific needs without cluttering the core functionality.
Pagination and filtering: Enable developers to retrieve data in manageable chunks and filter based on specific criteria. This is especially important for handling large datasets.
Support for multiple data formats: Consider offering data in JSON, XML, or other popular formats to cater to different developer preferences and tooling.
Example (Optional Parameters with Flask):
Python
@app.route('/users', methods=['GET'])
def get_users():
limit = request.args.get('limit', 10) # Default limit of 10 users
return jsonify(users[:limit])
4. Discoverability: Make Your API Findable
A well-designed API is useless if no one knows it exists! Discoverability is crucial for attracting users and fostering a developer community. Here's how to make your API discoverable:
Comprehensive documentation: Provide clear and up-to-date API documentation that explains functionalities, endpoints, parameters, and response formats. Utilize tools like Swagger or OpenAPI for interactive documentation.
Code samples and tutorials: Offer code samples in popular programming languages to demonstrate API usage. Tutorials with step-by-step guides can further lower the barrier to entry for new developers.
Interactive sandboxes: Consider providing an interactive sandbox environment where developers can experiment with the API and test their code without worrying about production data.
By adhering to these fundamental principles, you can create well-designed APIs that are simple to understand, consistent in behavior, adaptable to diverse needs, and easily discoverable by developers.
Subscribe to my newsletter
Read articles from Venkat R directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
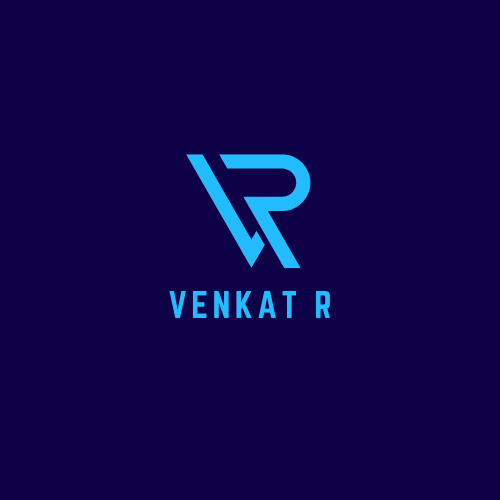
Venkat R
Venkat R
I am a marketer with the capacity to write and market a brand. I am good at LinkedIn. Your brand excellence on LinkedIn is always good with me.