Serverless APIs: A Deep Dive into the Future of API Development
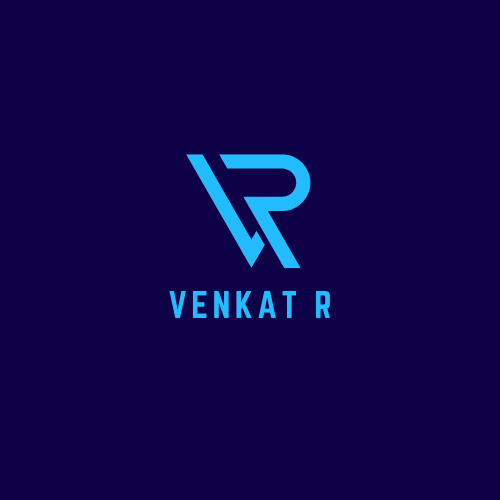
Serverless architecture is a paradigm shift in how software applications are designed, built, and - most importantly - scaled. It’s a model where the cloud provider dynamically manages the allocation and provisioning of servers. This model has a significant impact on API development, offering numerous benefits but also posing some challenges.
What is Serverless Architecture?
Serverless architecture refers to applications that significantly depend on third-party services (known as Backend as a Service or “BaaS”) or on custom code that’s run in ephemeral containers (Function as a Service or “FaaS”). The term “serverless” is somewhat misleading since servers are still involved. The difference is that developers no longer have to worry about server management tasks; instead, these duties are shifted to the cloud provider.
Benefits of Serverless APIs
Scalability: Serverless APIs can automatically scale based on the load. The cloud provider handles all the scaling configurations, allowing the system to handle more requests as demand increases.
Cost-Effective: With serverless architecture, you only pay for the execution time of your functions. This model can be more cost-effective than paying for idle server time, especially for APIs with variable loads.
Reduced Overhead: Server management, capacity planning, and system maintenance are handled by the cloud provider, reducing the operational overhead.
Faster Time to Market: Serverless architecture allows developers to focus on writing the code, speeding up the development process and reducing time to market.
Challenges of Serverless APIs
Cold Start: A cold start occurs when an API is invoked after being idle. The delay can impact performance, especially for APIs that require real-time response.
Monitoring and Debugging: Traditional monitoring and debugging tools may not work well in a serverless environment.
Vendor Lock-in: Applications are tightly coupled with the cloud provider’s technology, making it difficult to migrate to a different platform.
Real-World Use Cases
Use Case 1: Real-Time File Processing
A common use case for serverless APIs is real-time file processing. For example, a user uploads a photo to a cloud storage service (like AWS S3). This triggers a serverless function (like AWS Lambda) that processes the image in real-time, perhaps resizing it and then saving the thumbnail back to cloud storage.
import boto3
from PIL import Image
import os
s3_client = boto3.client('s3')
def resize_image(source_bucket, dest_bucket, source_key, dest_key, width, height):
s3_client.download_file(source_bucket, source_key, '/tmp/original.jpg')
with Image.open('/tmp/original.jpg') as img:
img.thumbnail((width, height))
img.save('/tmp/resized.jpg')
s3_client.upload_file('/tmp/resized.jpg', dest_bucket, dest_key)
def lambda_handler(event, context):
source_bucket = event['Records'][0]['s3']['bucket']['name']
source_key = event['Records'][0]['s3']['object']['key']
resize_image(source_bucket, source_bucket, source_key, 'resized/'+source_key, 128, 128)
Use Case 2: Data Transformation
Another use case is data transformation. A serverless API could be triggered whenever new data is added to a database. The function could clean, transform, and load the data into a data warehouse for further analysis.
const AWS = require('aws-sdk');
const docClient = new AWS.DynamoDB.DocumentClient({region: 'us-west-2'});
exports.handler = (event, context, callback) => {
event.Records.forEach((record) => {
const tableName = 'TransformedData';
const payload = AWS.DynamoDB.Converter.unmarshall(record.dynamodb.NewImage);
const params = {
Item: payload,
TableName: tableName
};
docClient.put(params, (err, data) => {
if (err) {
callback(err, null);
} else {
callback(null, data);
}
});
});
};
Serverless APIs represent a significant shift in the way we think about server-side logic. They offer many benefits, including automatic scaling, reduced operational costs, and faster time to market. However, they also present new challenges, such as cold starts and vendor lock-in. Despite these challenges, the serverless approach is an exciting development in the world of API development, and it’s worth exploring for many types of projects.
Subscribe to my newsletter
Read articles from Venkat R directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
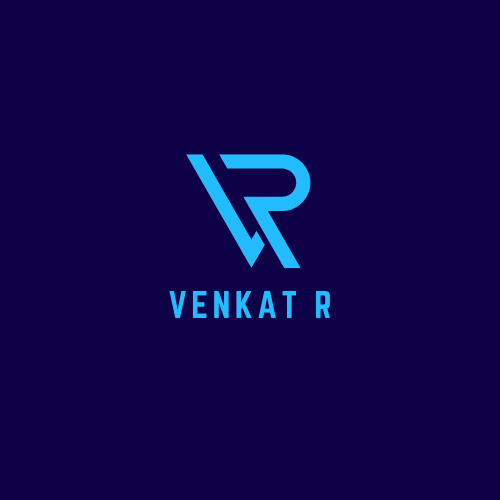
Venkat R
Venkat R
I am a marketer with the capacity to write and market a brand. I am good at LinkedIn. Your brand excellence on LinkedIn is always good with me.