Looping Through Data and Handling Controls

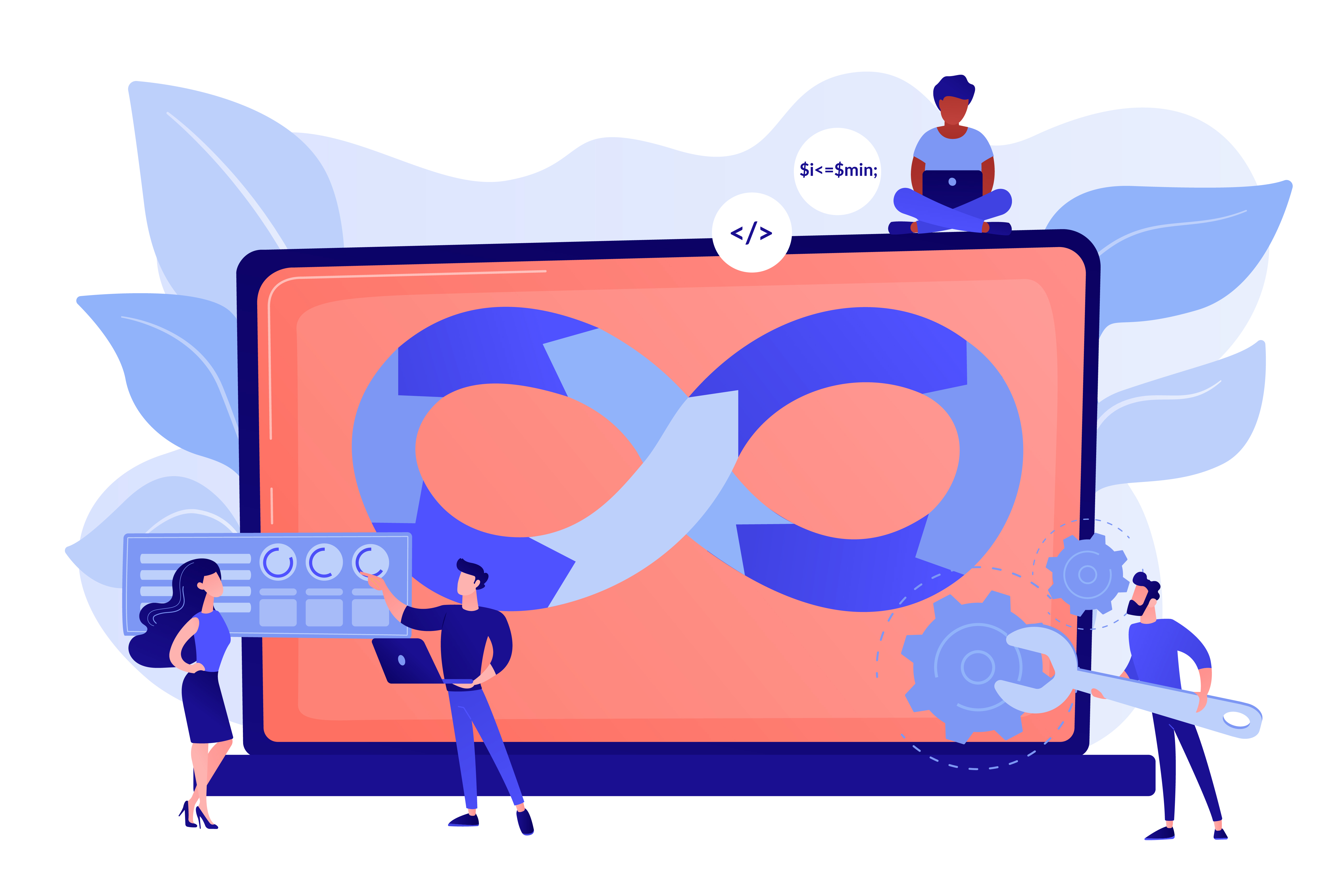
Imagine you've just got a new job as a Developer and your first task is having to take all the items in one list consisting of all the languages in the world and outputting them somewhere. Being the brilliant and hardworking Developer that you are you decide to list them all out one by one ( as in print(languageList[0], print(languageList[1]...) and you're happy as you see the Senior Developer stand above your shoulder smiling at your brilliance...wait that's a mocking smile isn't it?
That was a bad dream I had. In order to avoid this in your reality however, you'll have to learn about loops. I'm talking about for loops and while loops. We'll also take a look at the control statements If and Else. We'll check out List Comprehension, Ternary Operators and Switch (Match) Statements . Don't fret if this all sounds like German to you; it'll all be clear soon.
Control Statements
Python is well loved because there honestly couldn't be a programming language that sounds as much as the English language. The if and else statements do exactly what you'd expect them to do. It just means if condition do A else do B. The snippet below would explain better.
x = "Italian"
if x == "German":
print("Hey! I'm so efficient! watch me print German!")
else:
print("No...I guess I'll print the next language!")
The variable x houses the string "Italian". We then try to find out if x is "German". If x is what we want we print the first sentence but if it isn't, we print the second (what else here means). In other words, do something if the condition is obeyed. If not, do the other thing. Pretty straightforward.
But there's more. There is a third control statement and the reason for this is that sometimes we may need to have more than one condition. So we say if*condition, do A else ifanother condition, do B else do C. The *else statement is like a catch-all here. if all the other conditions fail, do the one associated with else. Check below.
x = "Italian"
if x == "German":
print("Hey! I'm so efficient! watch me print German!")
elif x == "Italian":
print("Whoa! Italian is here and I can print it. I'm the best Dev!")
else:
print("No...I guess I'll print the next language!")
Python is the "cool" language apparently, so while PHP goes with elseif and JavaScript else if, Python goes with elif...just a thought.
You should note that if/else statements can be nested; that is, one can be in another that can be in another, that can be...you get the idea.
x = 6
y = 12
if x == 9:
if y == 8
print ("Not sure what I'm doing here")
else:
print("I need some sleep, still not sure")
# The the first statment would be printed only if the two if conditions
# are true; else, the second statment is printed. That's how nested
# if statments run.
Switch (Match) Statements
Another way to handle control in code is to use what's known as a Switch Statement. Switch statements however have been used in languages like JavaScript for a long time, and the concept only just arrived in Python version 3.10 so...didn't take long I guess. Instead of a switch-case which many programmers are accustomed to, python uses a match-case statement. Like the if...else statements it checks for conditions and runs code accordingly.
At first glance however you may notice that the match-case statement evaluates a single expression, while if...else may have multiple conditions. Below is a typical example of a match-case statement.
x = "Italian"
match(x):
case "German":
print("Hallo!")
case "Spanish":
print("Hola!")
case "Idoma":
print("Nma Ochi!")
case _:
print("I guess the language isn't here...")
The code above checks each case for the condition (looking for "Italian"). If it fails to find the condition obeyed, it simply runs the default (case _).
Ternary Operators
Sometimes a short form of a function or statement is important for easy readability. This is the case with Ternary Operators. Check below.
# Using if/else statments;
x = "I am smart"
if x == "I am smart":
print ("Yes, you are")
else:
print ("Are you sure?")
# Using Ternary Operators;
x = "I am smart" ? "yes you are" : "Are you sure?"
print(x)
Did you see the magic? We successfully took a multi-line piece of code and condensed it into a single. That's what a ternary operator does but before you go rushing off to implement this in all your code, you've got to remember that they are quite limited. For instance, ternary operators can only handle one expression, whereas if/else statements can handle multiple conditions. In addition, a lot of Developers tend to prefer if/else statements because they are clearer to understand especially when dealing with more complex logic, and they are more flexible.
Looping with For Loops
Remember that bad dream I had at the beginning of this article and your (hopefully not) reality? I'll explain why the Senior Developer was amused. You see, in programming we often have to deal with a lot of data and in order to make our programs run more efficiently, it is important that we write code that is readable, fast and efficient. You'll learn more about this when we talk about algorithms soon enough (Don't worry about that now).
Imagine I ask you to pick up every grain of sand at a beach, you'll probably try to give me a black eye. It's the same with picking up each piece of data one by one. Loops help us with this by running through data so that we can have faster computations and won't have to type each item one after the...other... (sorry I dozed off there). Take a look at how a for-loop runs below.
# Printing each item
languageList = ["Italian", "German", "Chinese", "Idoma", "Hausa"]
print(languageList[0])
print(languageList[1])
# And you could keep going...
# Using a for-loop
for i in languageList:
print(i)
#The two code snippets do exactly the same thing, however, the
#for-loop saves us time. 'i' in the code refers to each item in the
#list. You are simply saying, 'for each i in this list, print the i'
#so all the data is printed.
You could combine if statements and for loops if you want to.
someNumbers = [1,2,3,4,5,6]
for i in someNumbers:
if i < 4:
print(i)
# for each item in someNumbers (i) print i if and only if i is less
# than 4. It will therefore print 1, 2 and 3.
Note also that for loops can be nested, like if statements.
languages = ["Italian", "German", "Igbo"]
takeOverWorldScale = ["possibly", "want to", "definitely"]
for i in languages:
for j in takeOverWorldScale:
print(f"The {i} man would {j} take over the world \n")
print("\n")
#"\n" means new line.
Break, Continue and Pass
The break statement is used when you want to stop a loop process before it is finished.
someNumbers = [1,2,3,4,5,6]
for i in someNumbers:
print(i)
if i == 4:
break
# What happens here is that the code stops printing the values
# after i becomes equal to 4. 1,2,3 and 4 are printed.
someNumbers = [1,2,3,4,5,6]
for i in someNumbers:
if i == 4:
break
print(i)
# In this case, 4 is excluded completely and 1,2 and 3 are printed.
The continue statement is important when we would like to pause on some value and continue from some point.
someNumbers = [1,2,3,4,5,6]
for i in someNumbers:
if i == 4:
continue
print(i)
#The code skips 4 and continues printing the rest of the list;
#so it prints 1,2,3,5,6.
The pass statement is used in python in the absence of code. Since loops cannot be empty, if you have nothing to put in a loop and leave it hanging, Python would get really mad at you. Placing a 'pass' statement is like having a placeholder.
for i in "Indonesian":
pass
Looping with While Loops
While loops are quite similar to for loops but there are differences. Look at the code snippet here.
inTheEnterprise = ["Russia", "USA", "Scottish", "Vulcan"]
#with a for loop
for i in inTheEnterprise:
print(i)
#with a while loop
x = 0
while x < len(inTheEnterprise):
print(inTheEnterprise[x])
x = x + 1
Both loops do the same thing, however we must initialize a variable (x in this case) that we have to iterate over. It is most important that we have a condition in a while loop that brings it to a stop (x = x + 1, here) else the condition would run forever if permitted. In the code, we make x become equal to 0; while x is less than the length of the list, we print each item in the list and increase x by 1 at each turn. When x is just below the length before exceeding the condition, it stops; so as long as x does not go over the length of the list of nationalities in the Star Trek Enterprise, the code is safe from running forever.
All we have discussed regarding break, continue and pass also apply to while statements.
List Comprehension
The last thing we would be looking at here is List Comprehension. Just as we saw ternary operators as some sort of short-form of if else statements, the same may be said about list comprehension as it relates to loops.
starTrekCrew = ["Kirk", "Spock", "Uhura", "Chekov", "MCcoy"]
beamingDown = []
#without List comprehension
for i in starTrekCrew:
if "o" in starTrekCrew:
beamingDown.append(i)
print(beamingDown)
#with List comprehension
starTrekCrew = ["Kirk", "Spock", "Uhura", "Chekov", "MCcoy"]
beamingDown = [i for i in starTrekCrew if "o" in starTrekCrew]
print(beamingDown)
#In running the code, you'd find that only Spock, Chekov and MCcoy
#beam down to the planet surface.
We can see that list comprehension makes the code more concise. We essentially form a new list with a short-form that just means that the list consists of i (each item) in the list, if "o" is in the item. We loop through the list with a for statement.
This...was a pretty information dense post but remember to take your time and go through the concepts. Arrivederci!
Subscribe to my newsletter
Read articles from Amanda Ene Adoyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amanda Ene Adoyi
Amanda Ene Adoyi
I am a Software Developer from Nigeria. I love learning about new technologies and talking about coding. I talk about coding so much that I genuinely think I could explode if I don't . I have been freelance writing for years and now I do the two things I love the most: writing and talking about tech.